Annotation in Java
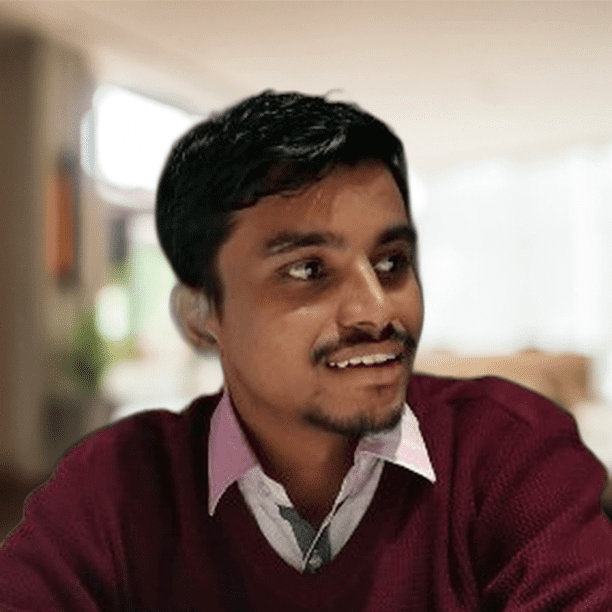
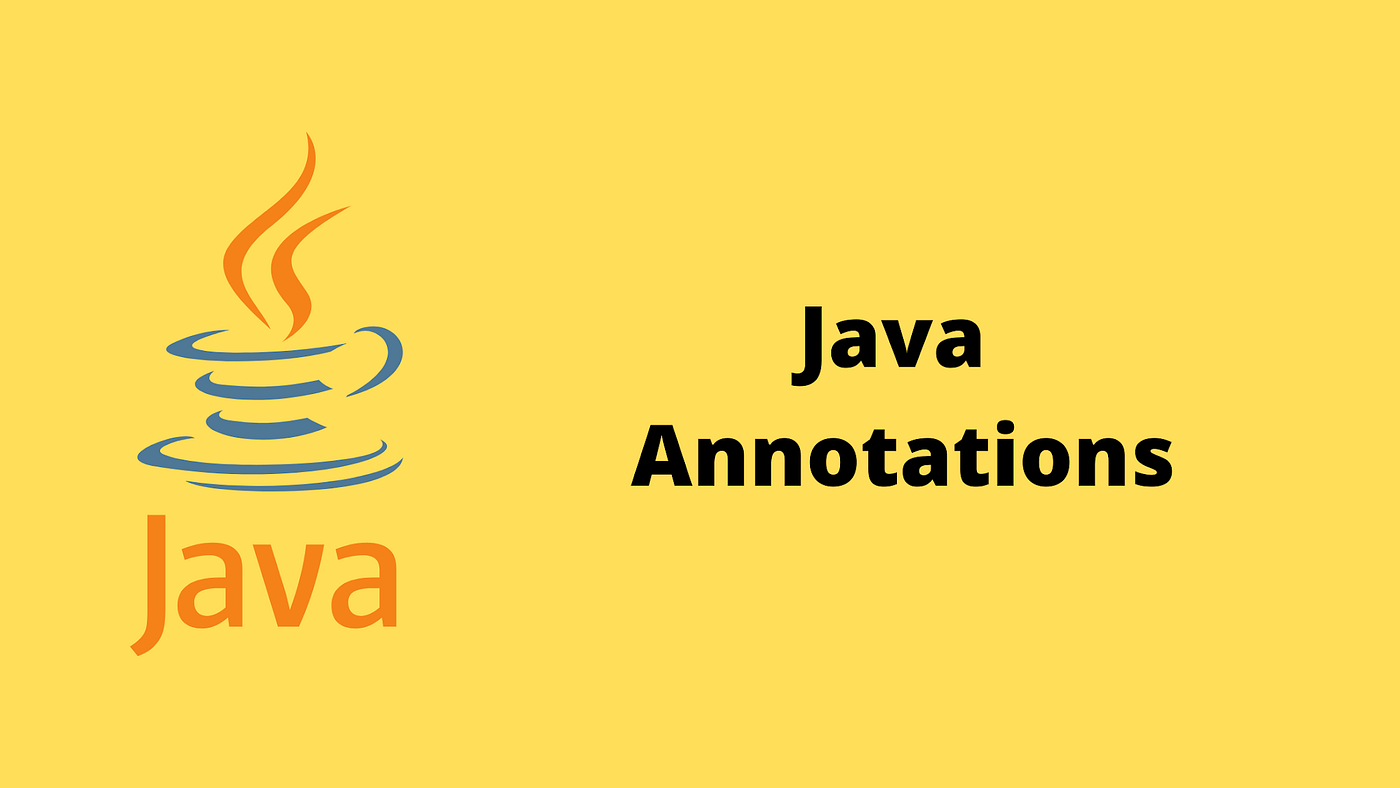
Before reading this article you have a basic understanding of annotations in Java
In this article, I will explain the following things
What is an annotation?
why do we need annotation?
How can we use an annotation?
Can we create a custom annotation?
Different Types of annotations
What is an annotation?
Annotation supplies additional information to code which can be used at the Class level, method level, field level, and interface level it depending on the Target of that annotation. Target is nothing but annotation scope.
Annotations in Java are denoted by the @
symbol followed by the annotation name,
@AnnotationName
public class MyClass {
// Class implementation
}
why do we need annotation? How can we use an annotation? Can we create a custom annotation?
In some cases, we need to supply some data or information to the class or method we can do this by just adding the annotation to that class or method.
here is the example
import java.lang.annotation.Annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@interface CricketPlayer{
int age();
String country();
}
@CricketPlayer(age=24,country="india")
public class App {
int runs;
int innings;
public void setRuns(int runs) {
this.runs=runs;
}
public void setInnings(int innings) {
this.runs=runs;
}
public int getRuns() {
return runs;
}
public int getInnings() {
return innings;
}
public static void main( String[] args ) {
App a = new App();
a.setRuns(23);
a.setInnings(12);
System.out.println(a.getInnings());
System.out.println(a.getRuns());
Class c= a.getClass();
Annotation an = c.getAnnotation(CricketPlayer.class);
CricketPlayer cp = (CricketPlayer)an;
System.out.println(cp.age());
System.out.println(cp.country());
}
}
//output
0
23
24
india
In the above example, we add @CricketPlayer
an annotation to the class App it provides age and country to the class and we can use that information.
@CricketPlayer
is a custom annotation that I created, we can create custom annotations also.
Different Types of annotations
In Java, there are several built-in annotations that serve different purposes and are used in various contexts. Here are some commonly used types of annotations in Java
Marker Annotations:
@Override
: Indicates that a method in a subclass is intended to override a method from the superclass.@Deprecated
: Marks a method, class, or field as deprecated, indicating that it is no longer recommended for use.
Single-Value Annotations:
@SuppressWarnings
: Suppresses compiler warnings for a specific element or a whole code section.@SafeVarargs
: Indicates that a method with a var args parameter is safe to use with non-reifiable types.
Multi-Value Annotations:
@Author
: Indicates the author of a class or method.@SuppressWarnings
: Suppresses multiple types of compiler warnings.
Meta-Annotations:
@Retention
: Specifies the retention policy for annotation (e.g.,@Retention(RetentionPolicy.RUNTIME)
).@Target
: Specifies the target elements (e.g., classes, methods) to which an annotation can be applied.@Documented
: Indicates that annotation should be documented by tools.
Functional Interface Annotations:
@FunctionalInterface
: Indicates that an interface is intended to be a functional interface, which has a single abstract method.
Type Annotations:
@NonNull
: Indicates that a parameter, field, or method return value should not be null.@Nullable
: Indicates that a parameter, field, or method return value can be null.
Subscribe to my newsletter
Read articles from Chimata Manohar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
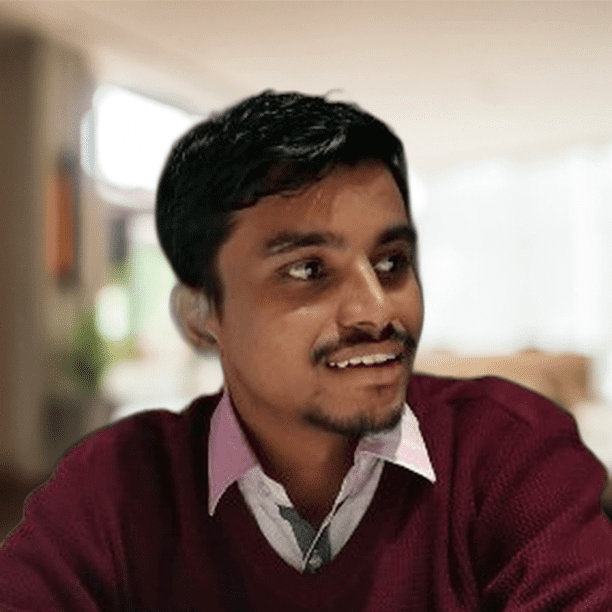
Chimata Manohar
Chimata Manohar
I am a developer from Arshaa Technologies Pvt.Ltd