Mastering JavaScript: Write Code Like Senior Developers
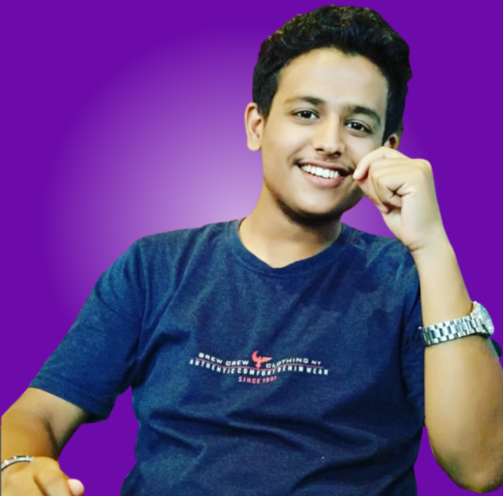
Table of contents
- Introduction
- Understanding the Importance of JavaScript Code Practices
- Writing Readable and Maintainable Code
- Effective Use of Variables and Functions
- Handling Errors and Exceptions
- Optimizing Performance
- Security Considerations
- Testing and Debugging
- Conclusion
- Understanding the Importance of JavaScript Code Practices
- Writing Readable and Maintainable Code
- Effective Use of Variables and Functions
- Handling Errors and Exceptions
- Optimizing Performance
- Security Considerations
- Testing and Debugging
- Conclusion
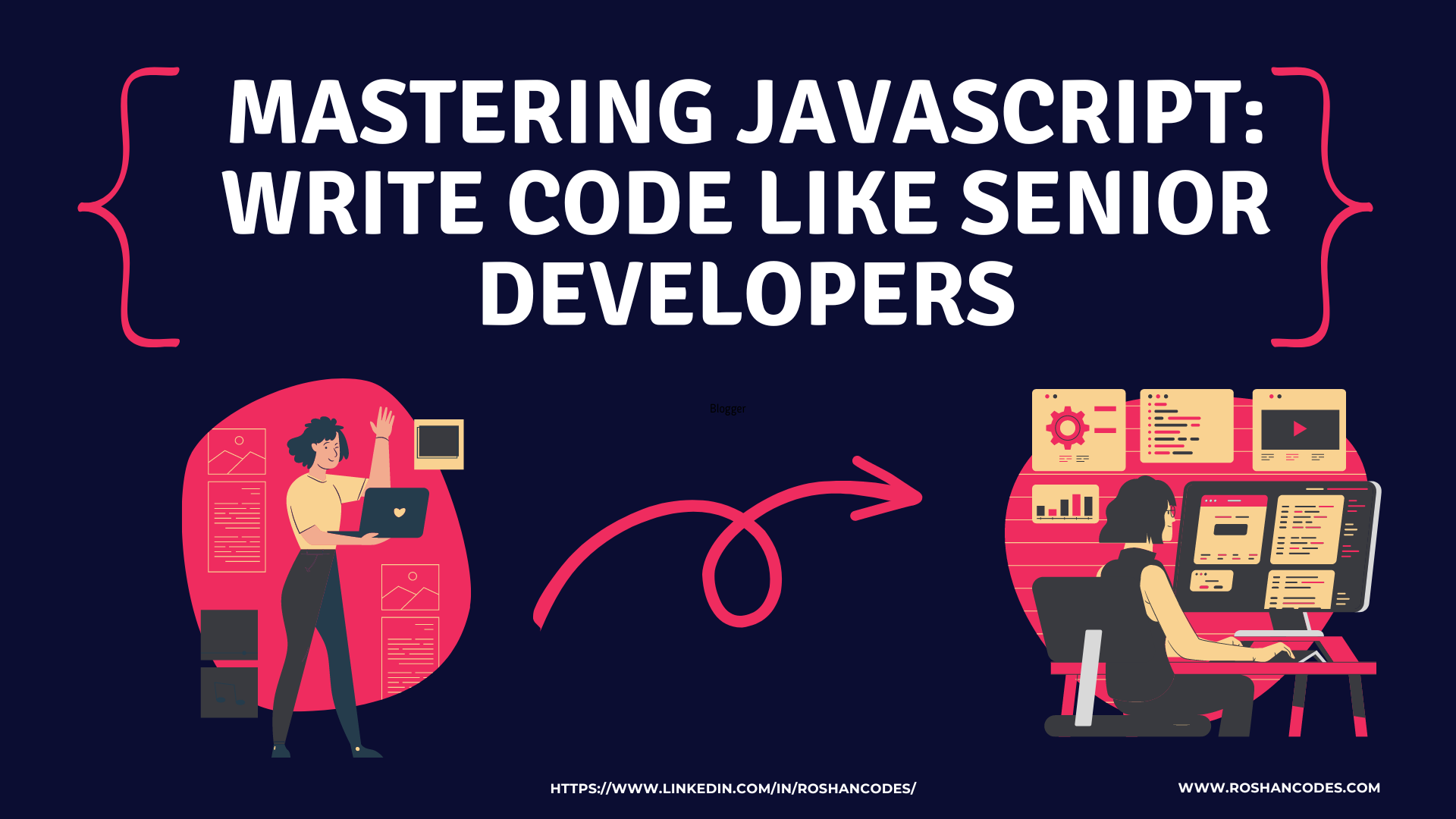
As a senior JavaScript developer, it is essential to not only write code that functions correctly but also to ensure that it is maintainable, efficient, and secure. By following best practices and adhering to established code conventions, you can enhance code readability, reduce errors, and optimize performance. This article will explore the top JavaScript code practices that senior developers should consider when working on projects.
Introduction
JavaScript is a powerful programming language that enables developers to create dynamic and interactive web applications. However, without proper coding practices, JavaScript codebases can quickly become difficult to manage, leading to increased development time, bugs, and a lack of scalability. By adopting the right practices, senior developers can produce high-quality code that is both efficient and maintainable.
Understanding the Importance of JavaScript Code Practices
Effective JavaScript code practices provide numerous benefits. They improve code readability, making it easier for developers to understand and maintain the codebase. Consistent code formatting and indentation help with this, ensuring that code is visually organized and accessible.
Writing Readable and Maintainable Code
Consistent Indentation and Formatting
Consistency in code indentation and formatting is crucial for readability. By using consistent indentation levels and formatting conventions, code becomes more organized and easier to follow. It is recommended to use a standard style guide, such as the one provided by the JavaScript Standard Style, to ensure consistency across the codebase.
Naming Conventions
Choosing meaningful and descriptive names for variables, functions, and classes is essential for code maintainability. By using clear and concise names, other developers can understand the purpose and functionality of each element without needing to dive into the implementation details.
Commenting and Documentation
Documenting code with comments and proper documentation is vital
for long-term maintenance. Comments can explain complex algorithms or provide additional context for future developers. Additionally, generating documentation using tools like JSDoc can create comprehensive references for the codebase.
Effective Use of Variables and Functions
Proper Variable Declarations
Always declare variables using let
or const
to ensure proper scoping and prevent global namespace pollution. Explicitly declaring variables improves code clarity and helps catch potential issues like accidental variable shadowing.
Avoiding Global Variables
Minimizing the use of global variables is a good practice as it reduces the risk of naming conflicts and makes code more modular and reusable. Encapsulating variables within functions or modules prevents unintended side effects and improves code maintainability.
Function Structure and Modularity
Breaking down complex tasks into smaller functions promotes code reusability and maintainability. Each function should have a single responsibility, making it easier to test, debug, and understand. Embracing modular design patterns like the module pattern or the revealing module pattern can enhance code organization.
Handling Errors and Exceptions
Error Handling Best Practices
Implementing proper error handling mechanisms ensures that your application gracefully handles unexpected situations. Use meaningful error messages and consider logging errors to aid in debugging. Handling errors consistently and gracefully prevents crashes and enhances user experience.
Try-Catch Blocks
Wrap code that may throw exceptions in try-catch blocks to handle specific errors and prevent application crashes. By catching and handling exceptions at appropriate levels, you can recover from errors gracefully and display relevant error messages to users.
Error Logging and Reporting
Implement error logging to capture and track errors that occur in the application. Error logs provide valuable insights into potential issues and help identify areas that require improvement. Utilize logging frameworks or error tracking services to streamline the process.
Optimizing Performance
Minimizing DOM Manipulation
Frequent updates to the Document Object Model (DOM) can impact performance. Minimize DOM manipulations by caching frequently accessed elements, utilizing batch operations, and avoiding unnecessary reflows and repaints. Manipulate the DOM efficiently to improve rendering speed.
Efficient Loops and Iterations
In JavaScript, loops can be a significant performance bottleneck. Optimize loops by reducing unnecessary iterations, utilizing appropriate loop constructs, and avoiding operations that impact performance, such as DOM manipulation within loops.
Caching Repeatedly Accessed Elements
Repeatedly accessing the same elements in the DOM can result in performance degradation. Cache frequently accessed elements in variables to minimize DOM queries and improve code efficiency. This practice reduces the overhead of searching and accessing elements repeatedly.
Security Considerations
Input Validation and Sanitization
Validate and sanitize all user input to prevent security vulnerabilities such as cross-site scripting (XSS) attacks. Implement server-side validation and client-side validation to ensure data integrity and protect against malicious input.
Protecting Against Cross-Site Scripting (XSS) Attacks
Preventing XSS attacks is crucial for web application security. Utilize proper output encoding techniques, such as escaping special characters, to prevent user input from being interpreted as code. Employ security libraries and frameworks to assist in mitigating XSS vulnerabilities.
Secure Data Handling and Storage
Safeguard sensitive data by following security best practices, including secure transmission over HTTPS and proper data encryption. Hash passwords and sensitive information before storing them in databases to protect user privacy and prevent unauthorized access.
Testing and Debugging
Writing Unit Tests
Unit testing is a crucial aspect of software development. Write comprehensive unit tests to validate the functionality and behavior of individual units of code. Utilize testing frameworks like Jest or Mocha to automate the testing process.
Debugging Techniques
Mastering debugging techniques is essential for identifying and fixing issues in JavaScript code. Use browser developer tools, console logging, breakpoints, and error tracking tools to efficiently debug and troubleshoot code.
Conclusion
Adopting top JavaScript code practices is paramount for senior developers aiming to excel in their craft. By prioritizing readability and maintainability, developers can create codebases that are not only functional but also comprehensible to their peers. Consistent indentation, naming conventions, and documentation contribute to code clarity, making it easier to understand and maintain. Effective variable and function usage, along with proper error handling, enhance code quality and stability. Optimizing performance through efficient DOM manipulation and loop iterations ensures smooth execution. Furthermore, considering security measures, testing, and debugging techniques guarantees robust and secure applications. By following these best practices, senior developers can elevate their JavaScript proficiency and produce high-quality code that stands the test of time.Introduction
JavaScript is a powerful programming language that enables developers to create dynamic and interactive web applications. However, without proper coding practices, JavaScript codebases can quickly become difficult to manage, leading to increased development time, bugs, and a lack of scalability. By adopting the right practices, senior developers can produce high-quality code that is both efficient and maintainable.
Understanding the Importance of JavaScript Code Practices
Effective JavaScript code practices provide numerous benefits. They improve code readability, making it easier for developers to understand and maintain the codebase. Consistent code formatting and indentation help with this, ensuring that code is visually organized and accessible.
Writing Readable and Maintainable Code
Consistent Indentation and Formatting
Consistency in code indentation and formatting is crucial for readability. By using consistent indentation levels and formatting conventions, code becomes more organized and easier to follow. It is recommended to use a standard style guide, such as the one provided by the JavaScript Standard Style, to ensure consistency across the codebase.
Naming Conventions
Choosing meaningful and descriptive names for variables, functions, and classes is essential for code maintainability. By using clear and concise names, other developers can understand the purpose and functionality of each element without needing to dive into the implementation details.
Commenting and Documentation
Documenting code with comments and proper documentation is vital
for long-term maintenance. Comments can explain complex algorithms or provide additional context for future developers. Additionally, generating documentation using tools like JSDoc can create comprehensive references for the codebase.
Effective Use of Variables and Functions
Proper Variable Declarations
Always declare variables using let
or const
to ensure proper scoping and prevent global namespace pollution. Explicitly declaring variables improves code clarity and helps catch potential issues like accidental variable shadowing.
Avoiding Global Variables
Minimizing the use of global variables is a good practice as it reduces the risk of naming conflicts and makes code more modular and reusable. Encapsulating variables within functions or modules prevents unintended side effects and improves code maintainability.
Function Structure and Modularity
Breaking down complex tasks into smaller functions promotes code reusability and maintainability. Each function should have a single responsibility, making it easier to test, debug, and understand. Embracing modular design patterns like the module pattern or the revealing module pattern can enhance code organization.
Handling Errors and Exceptions
Error Handling Best Practices
Implementing proper error handling mechanisms ensures that your application gracefully handles unexpected situations. Use meaningful error messages and consider logging errors to aid in debugging. Handling errors consistently and gracefully prevents crashes and enhances user experience.
Try-Catch Blocks
Wrap code that may throw exceptions in try-catch blocks to handle specific errors and prevent application crashes. By catching and handling exceptions at appropriate levels, you can recover from errors gracefully and display relevant error messages to users.
Error Logging and Reporting
Implement error logging to capture and track errors that occur in the application. Error logs provide valuable insights into potential issues and help identify areas that require improvement. Utilize logging frameworks or error tracking services to streamline the process.
Optimizing Performance
Minimizing DOM Manipulation
Frequent updates to the Document Object Model (DOM) can impact performance. Minimize DOM manipulations by caching frequently accessed elements, utilizing batch operations, and avoiding unnecessary reflows and repaints. Manipulate the DOM efficiently to improve rendering speed.
Efficient Loops and Iterations
In JavaScript, loops can be a significant performance bottleneck. Optimize loops by reducing unnecessary iterations, utilizing appropriate loop constructs, and avoiding operations that impact performance, such as DOM manipulation within loops.
Caching Repeatedly Accessed Elements
Repeatedly accessing the same elements in the DOM can result in performance degradation. Cache frequently accessed elements in variables to minimize DOM queries and improve code efficiency. This practice reduces the overhead of searching and accessing elements repeatedly.
Security Considerations
Input Validation and Sanitization
Validate and sanitize all user input to prevent security vulnerabilities such as cross-site scripting (XSS) attacks. Implement server-side validation and client-side validation to ensure data integrity and protect against malicious input.
Protecting Against Cross-Site Scripting (XSS) Attacks
Preventing XSS attacks is crucial for web application security. Utilize proper output encoding techniques, such as escaping special characters, to prevent user input from being interpreted as code. Employ security libraries and frameworks to assist in mitigating XSS vulnerabilities.
Secure Data Handling and Storage
Safeguard sensitive data by following security best practices, including secure transmission over HTTPS and proper data encryption. Hash passwords and sensitive information before storing them in databases to protect user privacy and prevent unauthorized access.
Testing and Debugging
Writing Unit Tests
Unit testing is a crucial aspect of software development. Write comprehensive unit tests to validate the functionality and behavior of individual units of code. Utilize testing frameworks like Jest or Mocha to automate the testing process.
Debugging Techniques
Mastering debugging techniques is essential for identifying and fixing issues in JavaScript code. Use browser developer tools, console logging, breakpoints, and error tracking tools to efficiently debug and troubleshoot code.
Conclusion
Adopting top JavaScript code practices is paramount for senior developers aiming to excel in their craft. By prioritizing readability and maintainability, developers can create codebases that are not only functional but also comprehensible to their peers. Consistent indentation, naming conventions, and documentation contribute to code clarity, making it easier to understand and maintain. Effective variable and function usage, along with proper error handling, enhance code quality and stability. Optimizing performance through efficient DOM manipulation and loop iterations ensures smooth execution. Furthermore, considering security measures, testing, and debugging techniques guarantees robust and secure applications. By following these best practices, senior developers can elevate their JavaScript proficiency and produce high-quality code that stands the test of time.
Thank you for visiting my blog! Stay connected and let's keep things interesting on social media. Follow me on Twitter (@**RoshanCodes_**) for the latest updates, Instagram (@roshan.codes) for some behind-the-scenes fun, and LinkedIn (@roshancodes) for a more professional connection. Let's connect and keep the conversation going beyond the blogosphere. See you there!
Subscribe to my newsletter
Read articles from Roshan Kumar Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
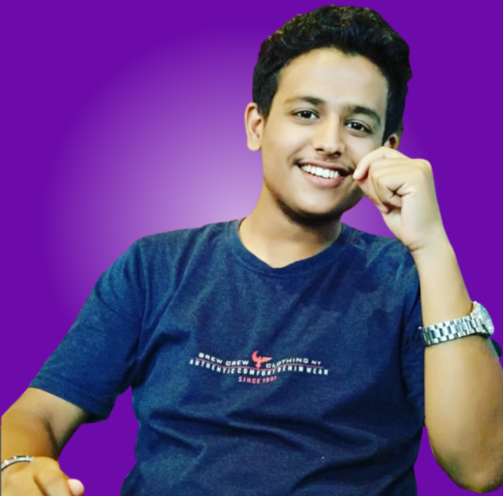