understanding javascript as a programming language

Table of contents
- Imagine the web pages without Javascript
- How javascript achieves dynamicity
- How to include Javascript in our HTML
- Internal Javascript with the script tag
- Javascript Data Types
- Variables in Javascript
- Useful tips on when to use var, let and const declarations
- Comments in Javascript
- Functions in Javascript
- Conclusion
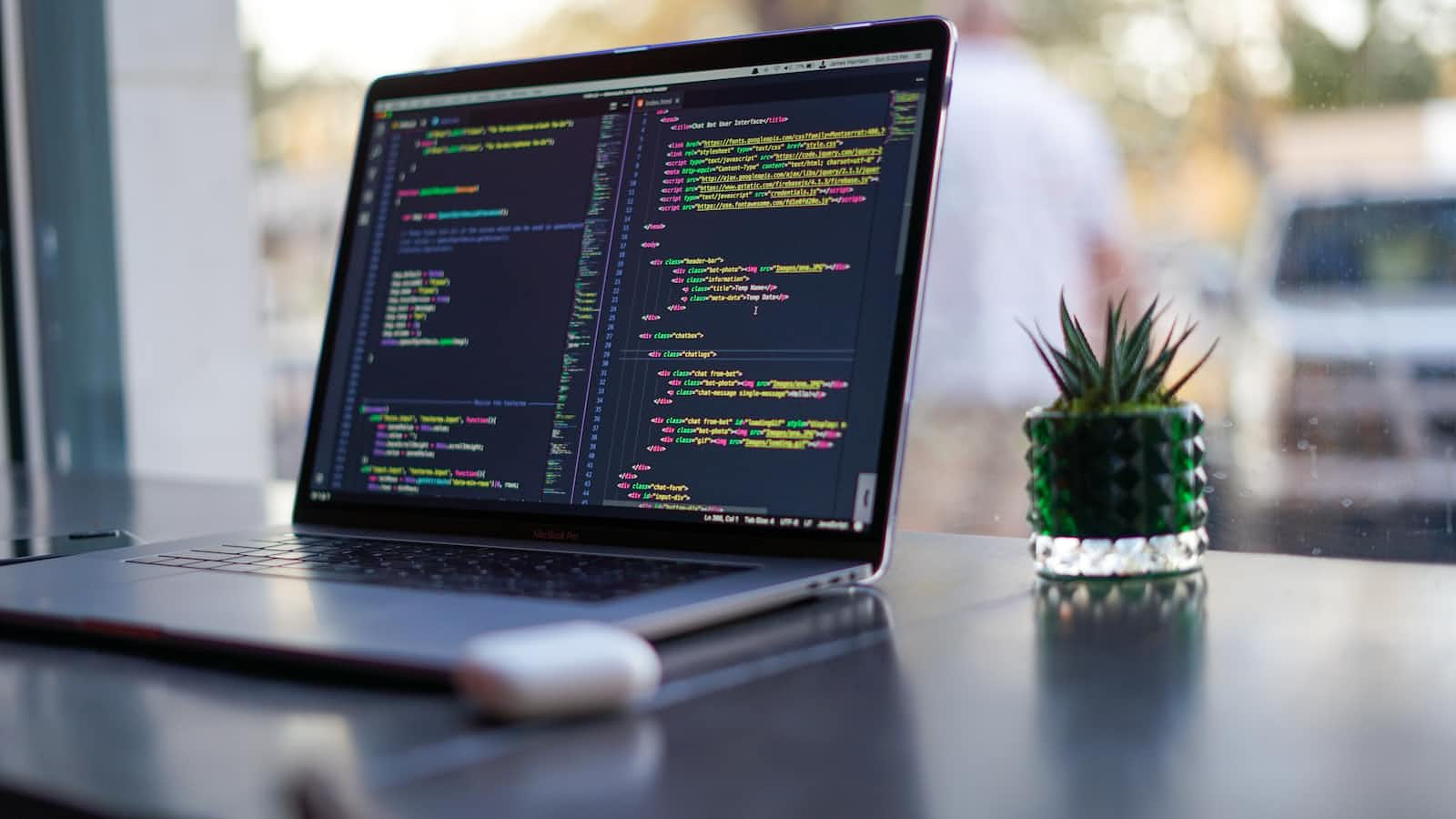
Well, have been coding on javascript for about 3 years now but I wouldn't be confident enough to explain what javascript is as a programming language. If you can explain easily what Javascript is within a few minutes allocated for the question, then I will call you a champ, you deserve a pat on the back.
Javascript has been around for some time now, It has gained popularity from its ability to build lighting fast applications supporting both the client and server side. But for sure, how would you define javascript pretty much good without beating around the bush? Well, don't tense, I got your precise answer.
Javascript is a dynamic programming language that is mostly used in building interactive responsive web applications, in game development and many more. It is dynamic in a manner that allows the implementation of features that cannot be achieved with pure CSS and HTML.
Behaviors such as button clicks, dynamic content rendering and changing of color elements, just to mention a few, are the work of javascript. Most web browsers use javascript as a scripting tool to achieve interactive dynamic behavior.
Imagine the web pages without Javascript
A basic web application is crafted from HTML, CSS and Javascript. With that noted, HTML and CSS contribute to 90%(if not more) of the static content of the application and can only achieve a few dynamic behaviors provided by CSS animations. A web page without javascript would mean a static website that is not interactive and undynamic.
How javascript achieves dynamicity
Without javascript, HTML, and CSS cannot achieve the full potential of a fully functional, interactive, and dynamic website. HTML is just a structured markup language that organizes the page content while CSS is a styling markup language that styles the elements in the page according to your need.
JavaScript, on the other hand, is a dynamic programming language that allows you to dynamically add HTML contents to the DOM, conditionally add CSS styling, data fetching from external websites, and lots more.
In this article, I will only cover a little more about JavaScript that can get you started. we will talk about.
How to include javascript in our HTML
Various Data Types
Variables
Comments
functions
How to include Javascript in our HTML
There is probably a bunch of ways to embed javascript in your code. Whichever you choose depends on your preference for code structure. Here is a list of ways to achieve it
Inline Javascript
In this method, javascript code is wrapped within special attributes
For example, HTML elements have event attributes attached to them that can be triggered in case of an event occurring such as button clicks, mouseovers and so on. I have clearly shown this within the code below
<button onclick="alert('You just clicked a button')">Click me!</button>
This is often referred to as inline JavaScript.
Internal Javascript with the script tag
The
script
tag provided by javascript functions the same way as thestyle
tag by allowing one to fully write javascript base code within the script tags.<script> function(){ alert("I am inside a script tag") } </script>
External Javascript
This method provides the flexibility of defining your Javascript code in a completely different file and using its src path within the HTML code
Due to its flexibility, its mostly used in large projects with tones of files to keep things organised and easy to walk through
<!-- index.html --> <script src="./script.js"></script>
// script.js alert("I am inside an external file");
The
src
attribute of thescript
tag allows you to apply a source for the JavaScript code. That reference is important because it notifies the browser to also fetch the content ofscript.js
.It's now time to study some of the features of javascript, now that we already have the basics of including javascript in our HTML code.
Javascript Data Types
In Javascript, data types can store one or more values. Here the value assigned to a variable determines the data type of that variable, This is why most often javascript is referred to as loosely typed. How different data types interact with each other is left for Javascript to handle. There are 7 different data types in javascript, eg
Number Type (for example,
6
,7
,8.9
): supports arithmetic operations (like addition/subtraction) and so onString Type (like
"hello, world"
,'My blog'
: here anything defined within either double("..."
) or single('...'
) quotes are considered as a String. There's no difference between single and double quotesBoolean Type (either
true
orfalse
): just like you can say yes (true
) or no (false
)Array Type (for example,
[1, 2, "hello", false]
): a collection of data (can be of different data types or even include an array ) separated by a comma. By default, its indexing starts from 0. Accessing the content of such a group can be like so:array[0]
, which for this example will return1
, since it's the first item.Object Type (for example
{name: 'javascript', age: 5}
): stores its data as akey: value
pair. Thekey
is defined as a string, and its associated value can be of any data type including the object itself. To access the values associated with the specific key, we can either use a dot notation soobj. age
or bracket notation like soobj["age"]
, this returns5.
Undefined Type (
undefined
like its name suggests): Is a variable declared without any value assigned to it. The assignment can be done either implicitly by javascript or explicitly by a developer. Later in this article, we'll look at variable declaration and value assignment.Null Type (
null
denotes a value that is null/empty): Null means there is no value or is empty. It stores an empty value ( more precisely-not real value) – rather, null.Function Type (for example,
function(){ console.log("function") }
): A function is a data type that invokes a block of code when called. We will discuss more on functions later in this article
JavaScript data types can be a bit complicated to understand. You may have heard that arrays and functions are also objects, and that's true.
Data types in javascript sometimes sound confusing and might be hard to understand. Do you know functions and arrays are also objects, Wait a minute, already i have started the confusion too early right? This is an advanced level, and I will cover it in future articles, With the basics well said and discussed, I think is time to learn how variables work in javascript right?
Variables in Javascript
Variables are containers for values of any data type. They hold values such that when the variables are used, JavaScript uses the value they represent for that operation.
Variables are container stores for values of any data type. They store values that will be used whenever an operation involves them. Javascript uses the value they represent to perform a given operation
Terms that are associated with variables are( declaration and assignment)- a variable can be declared and can be assigned a value. When a variable is declared, then it means doing this:
let name;
In the above example, name
is declared, but is not assigned a value.
Here javascript, as you may have learned in the data types section, does an implicit assignment of undefined
to the variable a name
so that whenever you try to use this variable in an operation, the undefined value will be assigned to that variable in that particular operation
Here's what it means to assign a value to a variable:
let name;
name = "JavaScript";
Here, when you use name
variable, it will no longer represent undefined
but the value javascript
assigned to it
Declarations and assignments can be done on one line like so:
let name = "JavaScript";
As you may have noticed in the above examples, I have constantly used let
keyword for variable declarations right? I will explain shortly why the consistency. In javascript, the variable declaration can be done in 3 different ways as follows:
- the
var
operator: This has been in javascript since its creation. It supports variable redeclarations and reassignment. To be precise, a variable can be reassigned to another value as well it can be redeclared with the same name without any errors. Here's what I mean:
//first declaration and variable assignment
var name = "JavaScript";
//variable reassigment
name = "Language";
//redeclaration of the variable name, doesnt cause any error
var name="Javascript"
- the
let
operator: most often works the same asvar
with little difference– It allows reassignment of variables and not redeclaration, This can be a major difference. In Javascript variables declared withvar
keywords are hoisted while thelet
keyword is not hoisted, This is also another difference. The code below briefly explains the concept of hoisting
function print() {
console.log(name);
console.log(age);
var name = "JavaScript";
let age = 5;
}
print();
When the print
function(print()
) is invoked/called, the first console.log
returns undefined
while the second console.log
fires an error that reads "Cannot access age
before initialization".
This is because variables declared with the var
keywords are hoisted to the top of the function and assigned undefined
by javascript at the same line
Here's how the above code is compiled:
function print() {
var name;
console.log(name);
console.log(age);
name = "JavaScript";
let age = 5;
}
print();
Hoisting problems do occur unexpectedly, and that's why you should use let
instead of var
. I now hope I have answered why I constantly used let
in the above examples
- the
const
operator: Is never hoisted, and doesn't support reassignment or redeclarations, but it does one more thing: It creates an immutable variable binding that doesn't allow any kind of mutation on the variable
For example:
let name = "JavaScript"
name = "Language" // no errors
const age = 5
age = 6 // error, assignment to a constant
Useful tips on when to use var
, let
and const
declarations
Avoid using
var
, stick withconst
andlet
due to hoisting and scope issuesuse
const
unless you need to reassign valuesuse
let
if you know, if you will reassign the value
Comments in Javascript
Comments in javascript are texts that are not meant to be executed by javascript. It is mostly used by developers to document clear explanations of how the code executes.
We can comment our comment in javascript in two ways:
a single-line comment, like so:
// a single-line comment
or with multi-line comments, like this:
/* a multi line comment */
Now let's talk about functions!!!!
Functions in Javascript
With functions, you can store a block of code that can be used in other places in your code. Say you wanted to print "JavaScript" and "Language" at different places in your code. Instead of doing this:
Functions in javascript are used to store blocks of code that might be executed later in our code. For example, assuming you wanted to log "Javascript" and "Language" in different places, Instead of performing this,
console.log("JavaScript")
console.log("Language")
// some things here
console.log("JavaScript")
console.log("Language")
// more things here
console.log("JavaScript")
console.log("Language")
You can do this:
function print() {
console.log("JavaScript")
console.log("Language")
}
print()
// some things here
print()
// more things here
print()
By doing this, we have eliminated repeated code that pollutes our global scope by storing them within one place as a block of code, This is probably one of the benefits of functions. We can still perform several fancy things with functions, take a look here. Assuming we wanted to get the average of three numbers. We could have naively done this:
let num1 = 5
let num2 = 6
let num3 = 8
let average = (num1 + num2 + num3) / 3
Doing this outside the functions may be considered bad practice, Why- you are polluting the global scope which might get worse while working in a team. You can always do this within functions if all you care about is good practices
function findAverage(n1, n2, n3) {
let aver = (n1 + n2 + n3) / 3
return aver
}
let num1 = 5
let num2 = 6
let num3 = 8
let average = findAverage(num1, num2, num3)
// later on, somewhere else
let average2 = findAverage(...)
// later on, somewhere else
let average3 = findAverage(...)
You may have noticed the n1,n2,n3
are provided to the findAverage
function. In javascript, they are parameters that act as a placeholder for the values that will be given to the function during invocation later in our code
Conclusion
Javascript is a vast language with a lot more features we couldn't cover in this article, but am glad to have provided the basics to get you going. Now it's about time to explore every feature in javascript and how it can help you build interactive, dynamic webs
In this article, we've looked at how to add JavaScript code to our HTML files, the different types of data that JavaScript supports, variables that serve as containers for values, how to write comments in JavaScript, and a little bit about how to declare and use functions.
In this article, we have discussed how to add Javascript code to your HTML files, The data types associated with javascript, variables, Comments and some basics into function use and declarations
There are so many places to go from here, but I'd recommend learning about The DOM and how JavaScript interacts with it next which I will cover in a future article.
Subscribe to my newsletter
Read articles from Deno Abonda directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Deno Abonda
Deno Abonda
Am a passionate full stack developer from Kenya having 3 years of experience in building robust, scalable applications that are user friendly and meets clients objectives. I would love to start writing technical content that resonates with my audience, If that sound interesting, don't be too shy to get in touch.