useMemo Hook?

Table of contents
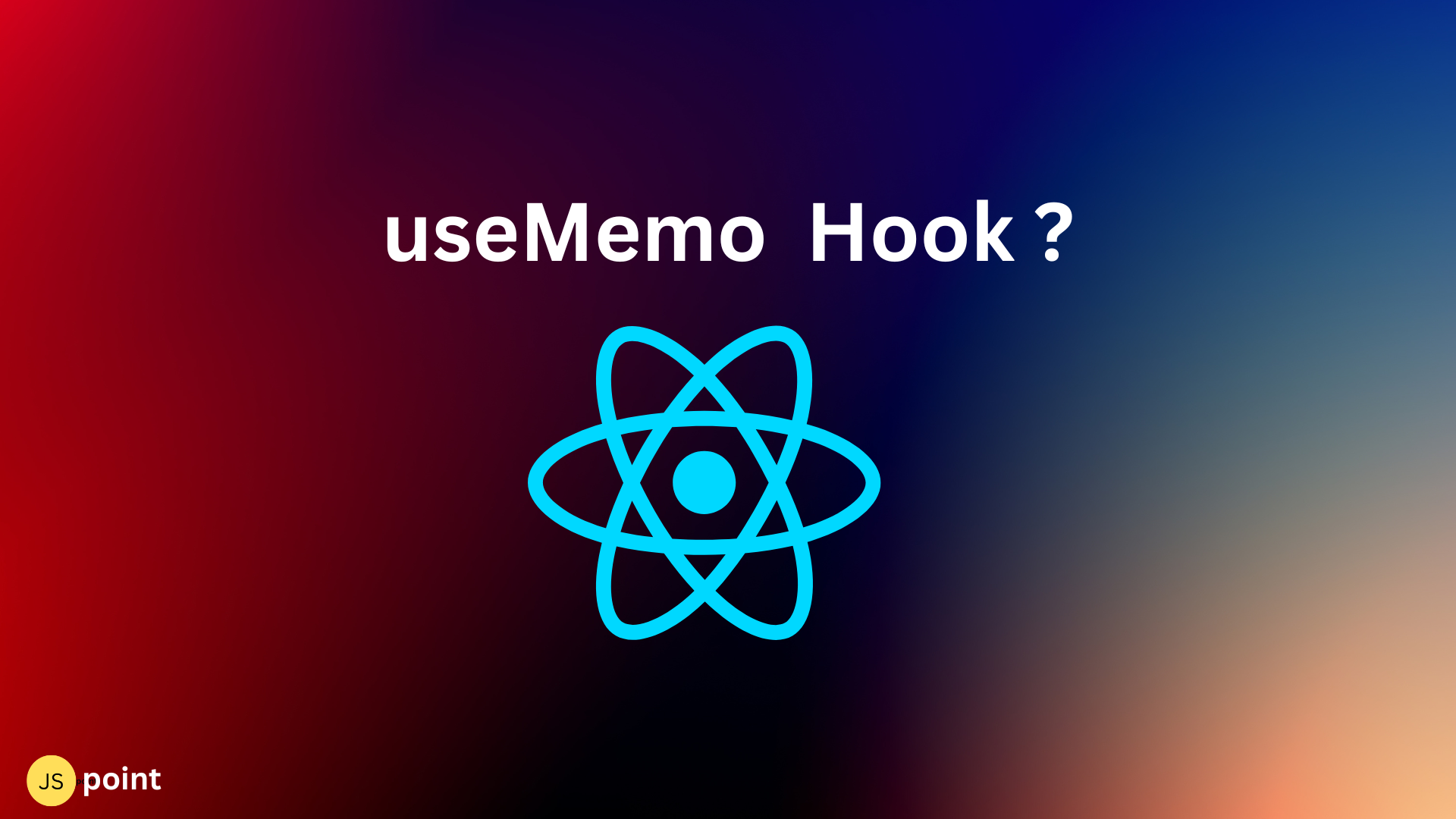
In this article, we will learn about useMemo Hook
It works similarly to the useCallback hook
The React
useMemo
Hook returns a memoized value.
What is useMemo hook?
useMemo()
is a built-in React hook that accepts ✌️two arguments — a function
and the dependencies array
:
const memoizedResult = useMemo(compute, dependencies);
The function provided to useMemo
is only re-evaluated if any of the dependencies in the dependencies array have changed. If the dependencies have not changed, the previously memoized value is returned without re-computing the function.
The syntax for using useMemo
is as follows:
import React, { useMemo } from 'react';
const Component = () => {
const memoizedValue = useMemo(() => {
// computation or expensive operation
return someValue;
}, [dependency1, dependency2]);
// Rest of the component logic
return (
// JSX rendering using the memoized value
);
};
Here's an example to illustrate the usage of useMemo
:
import React, { useState, useMemo } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
const doubleCount = useMemo(() => {
console.log('Computing double count...');
return count * 2;
}, [count]);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<p>Double Count: {doubleCount}</p>
<button onClick={increment}>Increment</button>
</div>
);
};
In the above example, we have a Counter
component that uses the useMemo
Hook. The doubleCount
variable is memoized using useMemo
. It computes the double of the count
value but will only recompute if the count
value changes.
Whenever the increment
button is clicked, the count
state is updated using the setCount
function, triggering a re-render of the component. However, the doubleCount
value is memoized, and it will not be re-computed if the count
value remains the same. This helps to optimize the performance of the component by avoiding unnecessary re-computations.
You can use useMemo
in scenarios where you have expensive calculations or computations that depend on certain values but don't need to be recomputed on every render. By memoizing the value, you can ensure that the computation is only performed when necessary, reducing unnecessary work and improving the performance of your React components.
Let's take another example:
import { useState, useMemo } from "react";
import ReactDOM from "react-dom/client";
const App = () => {
const [count, setCount] = useState(0);
const [todos, setTodos] = useState([]);
const calculation = useMemo(() => expensiveCalculation(count), [count]);
const increment = () => {
setCount((c) => c + 1);
};
const addTodo = () => {
setTodos((t) => [...t, "New Todo"]);
};
return (
<div>
<div>
<h2>My Todos</h2>
{todos.map((todo, index) => {
return <p key={index}>{todo}</p>;
})}
<button onClick={addTodo}>Add Todo</button>
</div>
<hr />
<div>
Count: {count}
<button onClick={increment}>+</button>
<h2>Expensive Calculation</h2>
{calculation}
</div>
</div>
);
};
In this example, the useMemo
hook is used to memoize the result of the expensiveCalculation
function based on the count
state. Whenever the count
state changes, the expensiveCalculation
function is re-evaluated, and the memoized result is stored in the calculation
variable.
The component has two state variables: count
and todos
. The count
state is incremented when the "+" button is clicked, and the todos
state is updated when the "Add Todo" button is clicked.
The expensiveCalculation
function represents a computationally expensive operation that takes the count
as input. It performs a loop for a large number of iterations, simulating a time-consuming calculation. By using useMemo
, the result of this calculation is memoized and only re-computed when the count
state changes.
The rendered JSX displays a list of todos, an "Add Todo" button, the current count
, an increment button, and the result of the calculation
. Whenever the count
state changes, the calculation
is re-evaluated and displayed.
Overall, useMemo
optimizes the performance of the component by avoiding unnecessary re-evaluations of the expensive calculation when the dependencies don't change, resulting in a smoother user experience.
Summary
The useMemo
Hook is a built-in hook in React that allows you to memoize the result of a computation and cache it for future use.
The useMemo
Hook only runs when one of its dependencies update.This can improve performance.
The useMemo
and useCallback
Hooks are similar. The main difference is that useMemo
returns a memoized value and useCallback
returns a memoized function
To fix this performance issue, we can use the useMemo
Hook to memoize the expensiveCalculation
function. This will cause the function to only run when needed.We can wrap the expensive function call with useMemo
.
The useMemo
Hook accepts a second parameter to declare dependencies. The expensive function will only run when its dependencies have changed.
Subscribe to my newsletter
Read articles from tarunkumar pal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

tarunkumar pal
tarunkumar pal
I'm a recent graduate with a degree in Mechanical Engineering but I have a passion for web development. I have hands on in ReactJS and I have experience building responsive, user-friendly web applications using this framework. I gained hands-on experience in web development through several projects . I'm proficient in HTML, CSS, JavaScript, and I have experience working with various front-end technologies such as ReactJS, Redux, and Bootstrap. I'm also familiar with back-end development such as Node.js and Express.js.