How to Use Angular to Build Dynamic Forms in Your Web Application

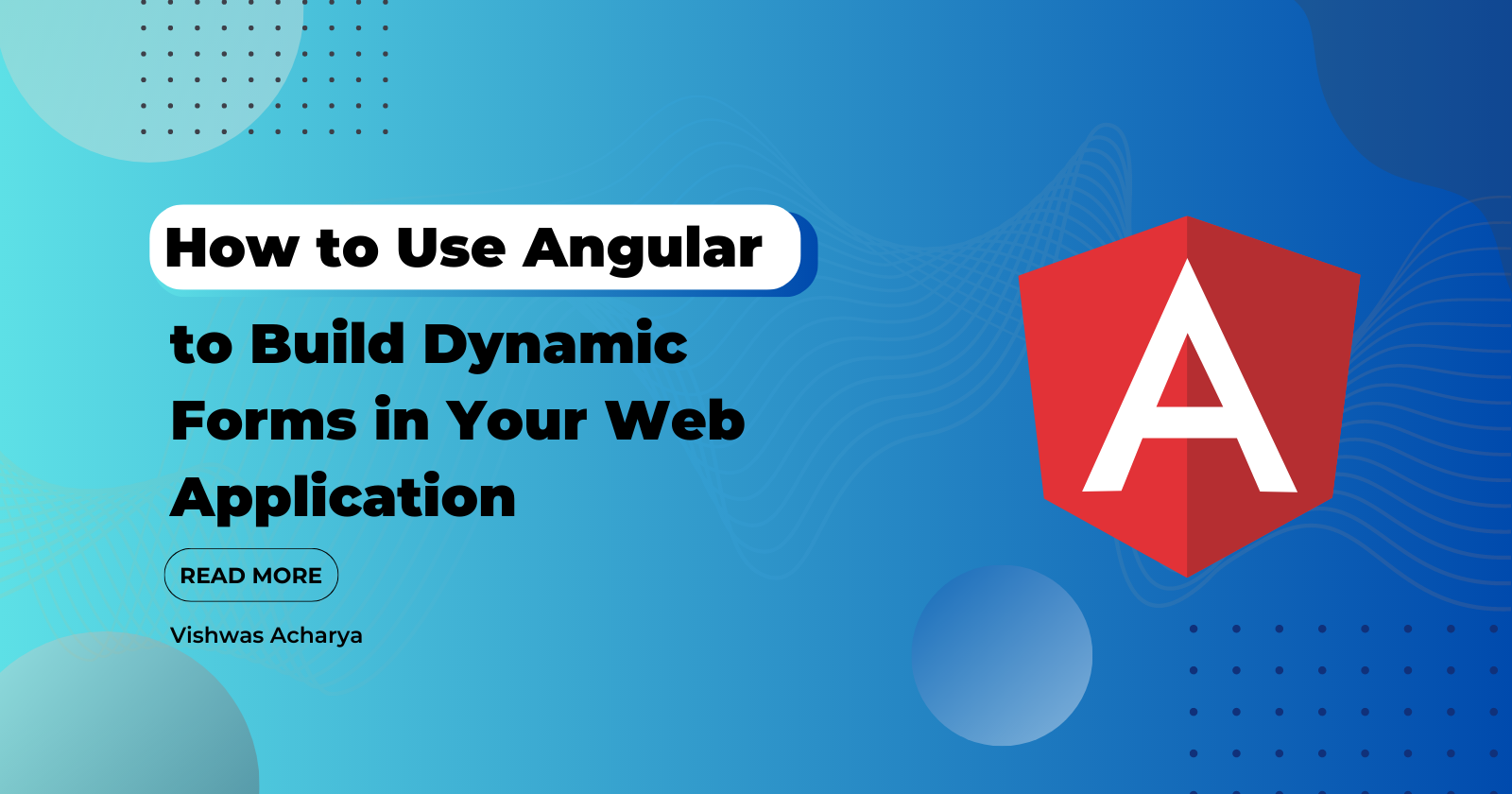
As a web developer, building dynamic forms is an essential part of creating user-friendly and efficient web applications. In this article, we will explore how to use Angular to build dynamic forms that are easy to use, efficient, and visually appealing. We will cover everything from the basics of building forms with Angular to more advanced techniques for creating complex forms.
Introduction
In this section, we will discuss the importance of building dynamic forms and why Angular is a great tool for achieving this goal.
Getting Started with Angular Forms
In this section, we will cover the basics of building forms with Angular, including template-driven forms and reactive forms. We will also discuss the pros and cons of each approach and when to use each one.
Template-Driven Forms
In this subsection, we will explore how to build template-driven forms in Angular. We will cover how to create a form, how to add form controls, how to validate form input, and how to submit form data.
Reactive Forms
In this subsection, we will explore how to build reactive forms in Angular. We will cover how to create a form, how to add form controls, how to validate form input, and how to submit form data. We will also discuss the benefits of using reactive forms over template-driven forms.
Advanced Form Techniques with Angular
In this section, we will explore more advanced techniques for building dynamic forms with Angular. We will cover topics such as form arrays, nested forms, and custom form validators.
Form Arrays
In this subsection, we will explore how to use form arrays in Angular to build dynamic forms with multiple form inputs. We will cover how to add form inputs dynamically, how to remove form inputs, and how to validate form input in a form array.
Nested Forms
In this subsection, we will explore how to use nested forms in Angular to build complex forms with multiple levels of input. We will cover how to create a nested form, how to add form controls, how to validate form input, and how to submit form data.
Custom Form Validators
In this subsection, we will explore how to create custom form validators in Angular. We will cover how to create a custom validator function, how to add it to a form control, and how to use it to validate form input.
Example:
Creating the Form Component
import { Component } from '@angular/core';
import { FormGroup, FormControl, Validators } from '@angular/forms';
@Component({
selector: 'app-form',
templateUrl: './form.component.html',
styleUrls: ['./form.component.css']
})
export class FormComponent {
form: FormGroup;
constructor() {
this.form = new FormGroup({
name: new FormControl('', [Validators.required]),
email: new FormControl('', [Validators.required, Validators.email]),
age: new FormControl('', [Validators.required, Validators.min(18)]),
gender: new FormControl('', [Validators.required]),
interests: new FormControl([], [Validators.required])
});
}
onSubmit() {
if (this.form.valid) {
console.log('Form submitted:', this.form.value);
}
}
}
Creating the Form Template
<form [formGroup]="form" (ngSubmit)="onSubmit()">
<div>
<label for="name">Name:</label>
<input id="name" type="text" formControlName="name">
<div *ngIf="form.get('name').invalid && (form.get('name').dirty || form.get('name').touched)">
<div *ngIf="form.get('name').hasError('required')">Name is required.</div>
</div>
</div>
<div>
<label for="email">Email:</label>
<input id="email" type="email" formControlName="email">
<div *ngIf="form.get('email').invalid && (form.get('email').dirty || form.get('email').touched)">
<div *ngIf="form.get('email').hasError('required')">Email is required.</div>
<div *ngIf="form.get('email').hasError('email')">Email is invalid.</div>
</div>
</div>
<div>
<label for="age">Age:</label>
<input id="age" type="number" formControlName="age">
<div *ngIf="form.get('age').invalid && (form.get('age').dirty || form.get('age').touched)">
<div *ngIf="form.get('age').hasError('required')">Age is required.</div>
<div *ngIf="form.get('age').hasError('min')">Age must be at least 18.</div>
</div>
</div>
<div>
<label for="gender">Gender:</label>
<select id="gender" formControlName="gender">
<option value="">Select gender</option>
<option value="male">Male</option>
<option value="female">Female</option>
<option value="other">Other</option>
</select>
<div *ngIf="form.get('gender').invalid && (form.get('gender').dirty || form.get('gender').touched)">
<div *ngIf="form.get('gender').hasError('required')">Gender is required.</div>
</div>
</div>
<div>
<label for="interests">Interests:</label>
<div>
<label><input type="checkbox" formControlName="interests" value="sports"> Sports</label>
<label><input type="checkbox" formControlName="interests" value="music"> Music</label>
<label><input type="checkbox" formControlName="interests" value="movies"> Movies</label>
</div>
</div>
<button type="submit">Submit</button>
</form>
Custom Validators
import { AbstractControl, ValidatorFn } from '@angular/forms';
export function forbiddenNameValidator(name: string): ValidatorFn {
return (control: AbstractControl): { [key: string]: any } | null => {
const forbidden = control.value === name;
return forbidden ? { 'forbiddenName': { value: control.value } } : null;
};
}
export function forbiddenEmailValidator(email: string): ValidatorFn {
return (control: AbstractControl): { [key: string]: any } | null => {
const forbidden = control.value === email;
return forbidden ? { 'forbiddenEmail': { value: control.value } } : null;
};
}
Using the Custom Validators
import { Component } from '@angular/core';
import { FormGroup, FormControl, Validators } from '@angular/forms';
import { forbiddenNameValidator, forbiddenEmailValidator } from './validators';
@Component({
selector: 'app-form',
templateUrl: './form.component.html',
styleUrls: ['./form.component.css']
})
export class FormComponent {
form: FormGroup;
constructor() {
this.form = new FormGroup({
name: new FormControl('', [
Validators.required,
forbiddenNameValidator('admin')
]),
email: new FormControl('', [
Validators.required,
Validators.email,
forbiddenEmailValidator('admin@example.com')
]),
age: new FormControl('', [Validators.required, Validators.min(18)]),
gender: new FormControl('', [Validators.required]),
interests: new FormControl([], [Validators.required])
});
}
onSubmit() {
if (this.form.valid) {
console.log('Form submitted:', this.form.value);
}
}
}
Conclusion
In conclusion, Angular provides a powerful and flexible way to build dynamic forms for your web application. With Angular's built-in form directives and validators, you can easily create complex forms with validation, error handling, and custom input components. By following the best practices outlined in this article, you can ensure that your Angular forms are performant, scalable, and maintainable. Happy coding!
FAQs
What is Angular?
Angular is a TypeScript-based open-source web application framework developed by Google.What are dynamic forms?
Dynamic forms are forms that are generated dynamically at runtime, based on some external data source or user input.What are validators in Angular?
Validators are functions that can be used to validate the values of form controls. They can be used to enforce rules such as required fields, minimum and maximum lengths, and custom validation rules.How can I create custom validators in Angular?
You can create custom validators by defining a function that takes a control as input and returns either null (if the control is valid) or an object with validation errors.How can I handle form submissions in Angular?
You can handle form submission in Angular by binding to the (ngSubmit) event of the form element and defining a function that handles the form submission. This function can access the form values through the form object, and perform any necessary validation or processing before submitting the form.
By Vishwas Acharya 😉
Checkout my other content as well:
YouTube:
Podcast:
Book Recommendations:
Subscribe to my newsletter
Read articles from Vishwas Acharya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vishwas Acharya
Vishwas Acharya
Embark on a journey to turn dreams into digital reality with me, your trusted Full Stack Developer extraordinaire. With a passion for crafting innovative solutions, I specialize in transforming concepts into tangible, high-performing products that leave a lasting impact. Armed with a formidable arsenal of skills including JavaScript, React.js, Node.js, and more, I'm adept at breathing life into your visions. Whether it's designing sleek websites for businesses or engineering cutting-edge tech products, I bring a blend of creativity and technical prowess to every project. I thrive on overseeing every facet of development, ensuring excellence from inception to execution. My commitment to meticulous attention to detail leaves no room for mediocrity, guaranteeing scalable, performant, and intuitive outcomes every time. Let's collaborate and unleash the power of technology to create something truly extraordinary. Your dream, my expertise—let's make magic happen! Connect with me on LinkedIn/Twitter or explore my work on GitHub.