Simplify Your Codebase with Absolute Imports in React: A Guide for JavaScript/TypeScript Programmers

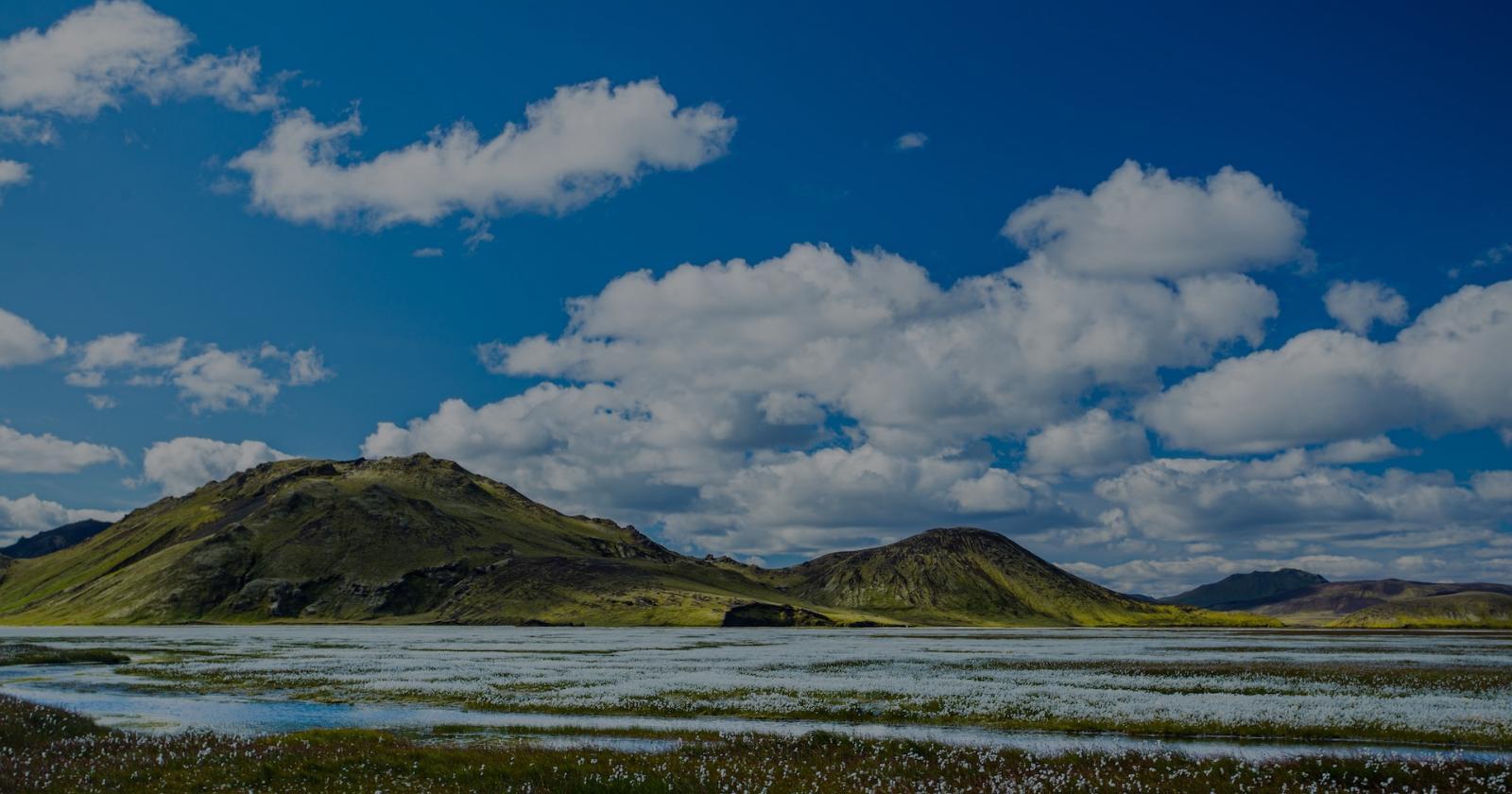
Introduction
As a JavaScript/TypeScript programmer, working on a large codebase means importing a lot of things from different places. Most times your import section looks like this:
import CartModal from "../../../components/cart/CartModal";
import Actions from "../../../store/actions/types";
This can lead to messy code, which can be particularly troublesome for React developers when it comes to maintenance. For instance, let's say we want to reuse some of the components imported in one feature component in another feature component. This would require us to rewrite the import statement, meaning we will have to retrace the location of the component we're importing. Such manual adjustments can be stressful and difficult to manage.
Absolute Imports
Using absolute imports in TypeScript and JavaScript makes it easier for us to write code that we can easily copy and paste. With absolute imports, we can have cleaner import statements like this:
import CartModal from "components/cart/CartModal";
import Actions from "store/actions/types";
It is highly recommended to use absolute imports instead of relative imports. The latter often requires navigating through various folders, which can become cumbersome. One significant drawback of relative imports is the need to retrace our steps when moving component content around.
For more information, you can check out this page from the Create React App documentation: https://create-react-app.dev/docs/importing-a-component/#absolute-imports
To configure absolute imports in your React project,
Create a `jsconfig.json` or `tsconfig.json` (if you're working with TypeScript) file
Add the code below to the file created in Step 1
If your project is already running, terminate it in the terminal
Start your project
{
"compilerOptions": {
"baseUrl": "src"
},
"include": ["src"]
}
Once you've completed these steps, you can now import your modules into your project using the absolute path format. If you want to import a module located at /src/components/Sidebar
you can import the component like this:
import Sidebar from "components/Sidebar";
Please ensure that you perform steps 3 and 4. Neglecting these steps may result in your changes not being applied.
I hope you find this information helpful.
Thank you for joining me on this technical journey. Your support and interest mean the world to me. I look forward to sharing more insightful content with you in the future. Until then, stay curious and keep exploring!
Subscribe to my newsletter
Read articles from Chilo Maximillian directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Chilo Maximillian
Chilo Maximillian
I am a Software Engineer from Nigeria. I have over 5 years of experience in Frontend Engineering and I have 2 years of experience working with AWS and GCP.