Mastering Python: Essential Tips and Tricks for Efficient Programming

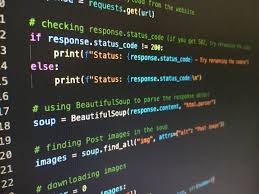
Python has become one of the most widely used programming languages across various domains, from web development and data analysis to artificial intelligence and scientific computing. Its simplicity, readability, and extensive ecosystem of libraries make it a popular choice for both beginners and experienced programmers. In this article, we will explore essential tips and tricks to help you master Python and become a more efficient programmer.
Introduction
Python is a high-level, interpreted programming language that was created by Guido van Rossum and first released in 1991. It is known for its clean and readable syntax, which makes it easy to understand and write code quickly. Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming, making it versatile for different types of projects.
Understanding Python Programming
What is Python?
Python is a dynamically-typed language that emphasizes code readability and simplicity. It uses indentation and whitespace to define code blocks, eliminating the need for braces or semicolons. Python's philosophy, often referred to as "The Zen of Python," promotes a clear and explicit coding style that prioritizes readability and ease of maintenance.
Why Python is Popular?
Python's popularity can be attributed to several factors. Firstly, its ease of learning and use makes it an excellent choice for beginners. Python's syntax resembles natural language, which helps new programmers grasp programming concepts more easily.
Secondly, Python has a vast ecosystem of libraries and frameworks that enable developers to build complex applications efficiently. Libraries such as NumPy, pandas, and Matplotlib are widely used in data analysis and scientific computing, while frameworks like Django and Flask are popular for web development.
Lastly, Python's versatility allows it to be used in various domains, including web development, data analysis, machine learning, and automation. Its flexibility makes it an attractive option for developers who need a language that can adapt to different tasks and projects.
Python Versatility and Use Cases
Python can be used for a wide range of applications, some of which include:
Web Development: Python web frameworks like Django and Flask provide powerful tools for building dynamic and scalable web applications.
Data Analysis: Python's libraries, such as NumPy and pandas, make it a preferred language for data analysis, manipulation, and visualization.
Machine Learning: Libraries like TensorFlow, PyTorch, and scikit-learn enable developers to implement machine learning algorithms efficiently.
Scientific Computing: Python is widely used in scientific research for simulations, data modeling, and visualization.
Automation and Scripting: Python's simplicity and versatility make it ideal for automating repetitive tasks and scripting.
Essential Python Tips and Tricks
To become a proficient Python programmer, it's essential to familiarize yourself with various tips and tricks that can improve your coding efficiency. Let's explore some of these techniques:
Utilizing Python Libraries
Python has a rich collection of libraries that extend its capabilities and provide pre-built functions and tools. Leveraging these libraries can significantly enhance your productivity. Some popular Python libraries include:
NumPy: A library for numerical computing that provides efficient data structures and functions for working with large arrays and matrices.
pandas: A data analysis library that offers powerful data manipulation and analysis tools, including data frames and SQL-like operations.
Matplotlib: A plotting library that allows you to create a wide range of static, animated, and interactive visualizations.
requests: A library for making HTTP requests, enabling you to interact with web APIs and retrieve data from remote servers.
Beautiful Soup: A library for web scraping, which allows you to extract data from HTML and XML documents.
Efficient Coding Techniques
Writing clean and efficient code is crucial for Python development. Here are some techniques to enhance your coding skills:
List Comprehensions: Use list comprehensions to create concise and readable one-liners for transforming and filtering lists.
Generator Expressions: Instead of creating large lists, use generator expressions to produce values on-the-fly, saving memory.
Context Managers: Employ the
with
statement and context managers to ensure proper resource management, such as file handling.Function Decorators: Use decorators to modify the behavior of functions, such as adding logging or caching functionality.
Exception Handling: Implement proper exception handling to gracefully handle errors and prevent program crashes.
Python Debugging and Testing
Bugs are an inevitable part of software development, but effective debugging and testing techniques can help you identify and fix issues quickly. Consider the following:
Debugging Tools: Familiarize yourself with Python's built-in debugger,
pdb
, and other third-party tools likepdb++
oripdb
to step through your code and identify problems.Unit Testing: Write unit tests using frameworks like
unittest
orpytest
to verify the correctness of individual components of your code.Test-Driven Development (TDD): Embrace TDD practices by writing tests before implementing code, promoting code quality and maintainability.
Linting and Code Analysis: Utilize tools like
flake8
orpylint
to enforce coding standards, detect potential errors, and ensure code consistency.Pythonic Idioms and Best Practices
Pythonic code follows the idioms and best practices established by the Python community. Adhering to these conventions improves code readability and helps you write more maintainable programs. Consider the following guidelines:
PEP 8: Follow the recommendations outlined in PEP 8, the official Python style guide, for consistent code formatting and naming conventions.
Naming Conventions: Use descriptive variable and function names that convey their purpose and avoid single-letter names or ambiguous abbreviations.
Docstrings: Document your code using docstrings to provide clear explanations of functions, classes, and modules.
Code Reviews: Engage in code reviews with peers to receive feedback and ensure code quality.
Version Control: Utilize a version control system, such as Git, to track changes, collaborate with others, and revert code if needed.
Advanced Python Concepts
Once you have a solid foundation in Python, exploring advanced concepts can take your programming skills to the next level. Let's delve into some advanced topics:
Object-Oriented Programming (OOP)
Python supports object-oriented programming, allowing you to create classes and objects to encapsulate data and behavior. Key OOP concepts in Python include:
Classes and Objects: Define classes to create objects with attributes and methods.
Inheritance: Use inheritance to create subclasses that inherit properties and behaviors from a parent class.
Polymorphism: Employ polymorphism to define methods with the same name but different implementations in different classes.
Functional Programming (FP)
Functional programming is another paradigm supported by Python. It emphasizes immutability, pure functions, and higher-order functions. Some FP concepts in Python include:
Higher-Order Functions: Functions that can accept other functions as arguments or return functions as results.
Lambda Functions: Anonymous functions defined using the
lambda
keyword for concise and one-time use.Map, Filter, and Reduce: Built-in functions for transforming, filtering, and aggregating data using functional programming techniques.
Python Generators and Iterators
Generators and iterators allow you to work with large datasets and streams of data efficiently. They provide a memory-friendly and iterative approach to processing data. Consider the following:
Generators: Create generators using the
yield
keyword to produce values on-demand, preserving memory.Iterators: Implement iterators by defining classes that implement the
__iter__
and__next__
methods for iterable objects.
Decorators and Metaclasses
Decorators and metaclasses are powerful features of Python that enable you to modify or extend the behavior of functions and classes. Understand these concepts to leverage their capabilities:
Decorators: Decorators are functions that wrap other functions, allowing you to add additional functionality or modify behavior.
Metaclasses: Metaclasses are classes that define the behavior and structure of other classes. They enable advanced customization and control over class creation.
Python Performance Optimization
Writing efficient and performant Python code is essential, especially when dealing with large-scale projects or computationally intensive tasks. Consider the following optimization techniques:
Profiling and Benchmarking
Use profiling tools to identify performance bottlenecks in your code. Python provides built-in modules like cProfile
and timeit
for profiling and benchmarking.
Memory Management and Optimization
Python's automatic memory management can sometimes lead to unnecessary memory consumption. Optimize memory usage by:
Avoiding Unnecessary Object Creation: Reuse objects where possible to reduce memory allocation overhead.
Garbage Collection: Understand Python's garbage collection mechanism and consider using libraries like
gc
to optimize memory usage.
Using Compiled Extensions
Python allows you to leverage compiled extensions, written in languages like C or C++, to optimize performance-critical parts of your code. Consider using tools like Cython or writing Python bindings for existing libraries.
Parallel Computing with Python
Leverage parallel processing to speed up computationally intensive tasks. Python provides libraries such as multiprocessing
and concurrent.futures
for parallelism and concurrency.
Python Tools and Resources
As a Python programmer, it's crucial to be aware of the tools and resources available to support your development process. Consider the following:
Integrated Development Environments (IDEs)
IDEs provide powerful features like code auto-completion, debugging, and project management. Some popular Python IDEs include PyCharm, Visual Studio Code, and Jupyter Notebook.
Package and Dependency Management
Use tools like pip
or conda
to manage packages and dependencies in your Python projects. Virtual environments, such as venv
or conda env
, help isolate project-specific dependencies.
Online Communities and Forums
Engage with the Python community to learn, share knowledge, and seek help. Websites like Stack Overflow, Reddit, and Python.org provide forums for discussion and support.
Learning Resources and Tutorials
Numerous online resources can help you improve your Python skills. Websites, tutorials, and documentation from sources like Python.org, Real Python, and YouTube tutorials offer valuable learning materials.
Conclusion
Mastering Python is an ongoing journey that requires continuous learning, practice, and exploration of its vast ecosystem. By understanding the fundamentals, adopting best practices, and utilizing advanced techniques, you can become a more efficient and effective Python programmer. Remember to stay engaged with the Python community and leverage available tools and resources to enhance your skills further.
Frequently Asked Questions (FAQs)
What are the main advantages of using Python for programming? Python offers advantages such as easy syntax, extensive libraries, cross-platform compatibility, and versatility across various domains.
How can I improve my Python coding skills? Practice regularly, read code from experienced developers, engage in coding challenges, and explore new libraries and techniques.
Are there any recommended Python libraries for specific domains? Yes, Python has numerous libraries catering to specific domains. For example, Flask and Django are popular for web development, while TensorFlow and PyTorch are widely used for machine learning.
What are some good resources for learning Python online? Python.org, Real Python, Codecademy, Coursera, and YouTube tutorials are excellent resources for learning Python online.
Can Python be used for web development? Yes, Python is widely used for web development. Frameworks like Django and Flask provide robust tools for building web applications efficiently.
Subscribe to my newsletter
Read articles from Krishna Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
