Python for Beginners: Part 2 - Understanding Variables
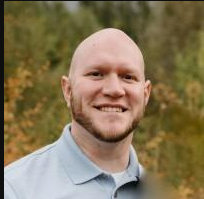
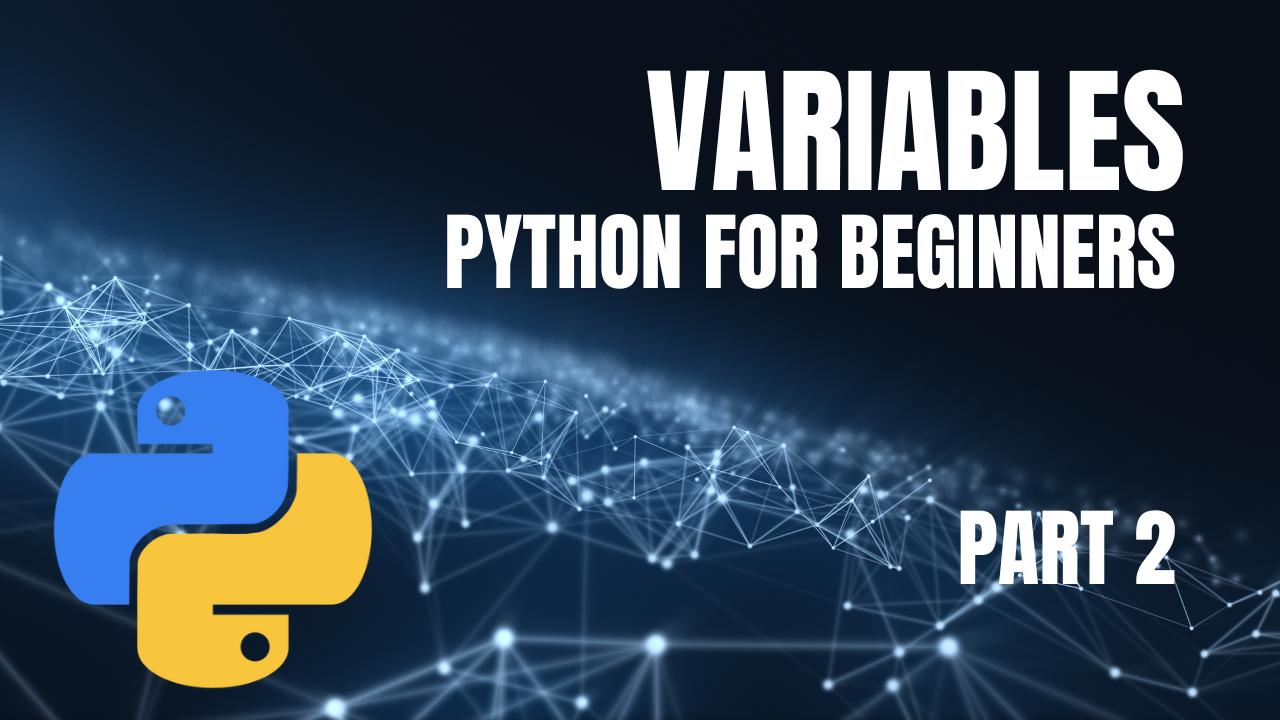
Welcome back! Today, we're going to take our first step in programming by exploring variables. Our goal is to familiarize ourselves with them and learn how to use them in our code. Then, we'll build a program to see how variables work in practice.
Understanding Variables
Imagine variables as containers that hold different things. They can store numbers, names, or any other information you can think of. Each variable has a unique name, like a label on a box. Let's explore some real-world examples to better understand variables:
Age: Think of a variable called "age" that stores your age. For example, if you're 10 years old, you can assign the value 10 to the variable "age." Later, you can update it as you grow older.
Favorite Color: Imagine having a variable called "favorite_color" that stores your favorite color. You can assign the value "blue" to it and change it whenever your preferences change.
Building Our First Python Program
Now, let's dive into Python coding and build a simple program that uses variables. Follow these steps:
Open your preferred Python development environment (e.g., Thonny, Mu, or any text editor).
Create a new Python file and save it with a meaningful name, such as "my_first_program.py".
In your Python file, write the following code:
# Creating variables
name = "Alice"
age = 12
favorite_color = "blue"
# Displaying information
print("My name is", name)
print("I am", age, "years old")
print("My favorite color is", favorite_color)
- Save the file and run the program. You should see the output displaying your information.
Explanation of the Code
The lines starting with a "#" symbol are comments. They provide information about the code but are ignored by the computer.
We create three variables: "name," "age," and "favorite_color." We assign values to them using the "=" sign.
The "print" function is used to display information on the screen. We use it to print our name, age, and favorite color, along with some additional text.
Let's Break It Down
In the first line,
name = "Alice"
, we create a variable called "name" and assign the value "Alice" to it. You can replace "Alice" with your name.The second line,
age = 12
, creates a variable called "age" and assigns the value 12 to it. You can change the value to your age.Similarly, the third line,
favorite_color = "blue"
, creates a variable called "favorite_color" and assigns the value "blue" to it. You can replace "blue" with your favorite color.Finally, the
print
statements display the stored information on the screen. You can add or modify the print statements to show additional information.
Conclusion
Congratulations! You've just built your first Python program using variables. You've learned that variables are like containers that store different types of information. They help us keep track of things in our programs.
In the next post, we'll explore different data types in Python, such as numbers and text. Get ready to expand your coding skills even further!
Subscribe to my newsletter
Read articles from Matthew Hard directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
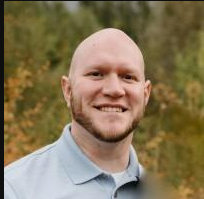
Matthew Hard
Matthew Hard
I'm Matthew, a cybersecurity enthusiast, programmer, and networking specialist. With a lifelong passion for technology, I have dedicated my career to the world of cybersecurity, constantly expanding my knowledge and honing my skills. From a young age, I found myself captivated by the intricate workings of computers and networks. This fascination led me to pursue in-depth studies in the fields of networking and cybersecurity, where I delved deep into the fundamental principles and best practices. Join me on this exciting journey as we explore the multifaceted world of technology together. Whether you're a beginner or a seasoned professional, I am here to share my knowledge, discuss the latest trends, and engage in insightful discussions. Together, let's embrace the ever-changing world of tech and navigate the complexities of cybersecurity with confidence and expertise.