Unit Test : Angular Series : Mocking a service in components
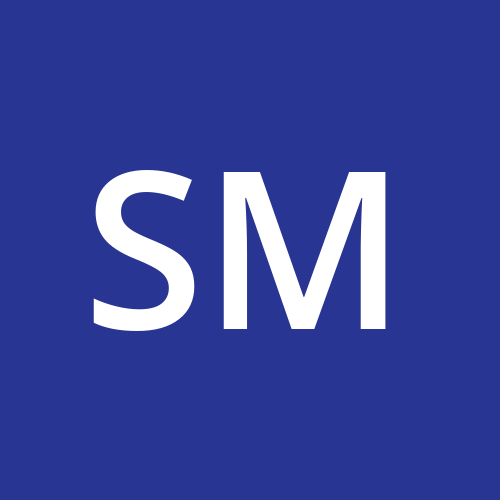
1 min read
This article will explain how to mock a service call while trying to cover a method in a component.
Consider the following component,
export class HelloComponent {
constructor(private helloSvs: HelloService) {
}
serviceCall() {
this.helloSvs.run().subscribe(val => {
if(val) { console.log("Printing True"); }
else { console.log("Printing False"); }
});
}
}
The service used there will be similar to this,
@Injectable({
providedIn: 'root'
)
export class HelloService {
run() {
// some operations and assume the method returns boolean value
if (true) {
return false;
}
return true;
}
}
Then the test case for the above component,
describe('AppComponent', () => {
beforeEach(async () => {
await TestBed.configureTestingModule({
declarations: [ HelloComponent ],
providers: [ HelloService ] /** Add the service **/
}).compileComponents();
});
beforeEach(() => {
fixture = TestBed.createComponent(HelloComponent );
component = fixture.componentInstance;
fixture.detectChanges();
});
it('should mock the service with true as return', () => {
/** Inject the service into fixture **/
const service = fixture.debugElement.injector.get(HelloService);
/**
Spy on the method present in the service and return the mock response
Here the mock calls will give a response as true.
**/
spyOn(service, 'run').and.returnValue(of(true));
/** While the call is made actually, the subscribe will get the response as true and corresponding lines will be executed **/
component.serviceCall();
.................
});
});
0
Subscribe to my newsletter
Read articles from Senbagaraman M directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
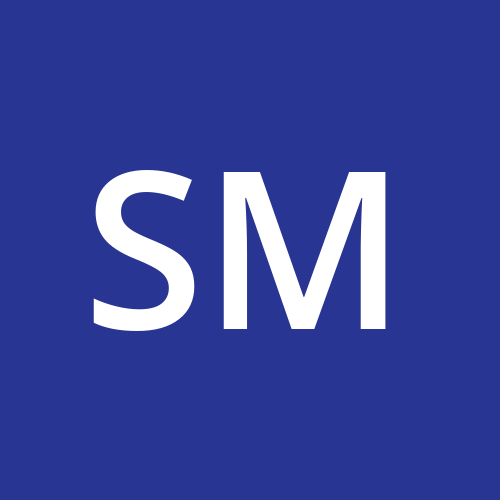