Shell Scripting Mastery: Exploring Conditionals, Loops, Functions, and Useful Commands with a Mini Project
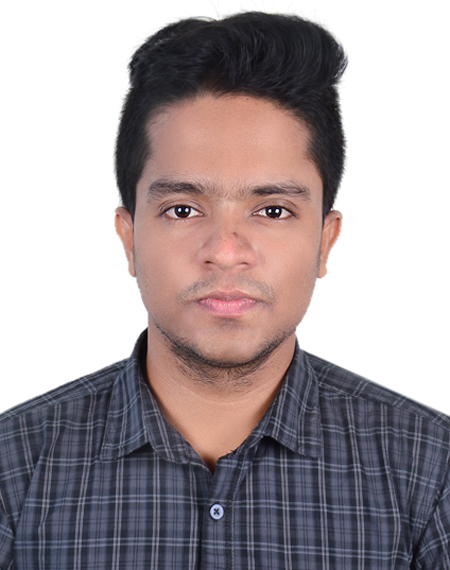
This article covers everything you need to know about user input, conditional statements (if-else), case statements, loops (for and while), functions, and passing arguments in shell scripting.
We will introduce essential commands like date, whoami, uptime, disk and RAM space checks, and last login details, which will be used in our mini project. The project includes welcoming users with their usernames, displaying the current date and time, showing server uptime and the last two or three login information (based on user input), providing server utilization statistics, and presenting details about the top two or three CPU processes(based on user input).
Create a file name whatever you want. But, make sure it ends with .sh. Open that file in your favorite text editor and write the first line to begin your shell script journey.
We execute .sh file and we will provide execute permission by below commands.
Chmod 700 ./filename.sh
#!/bin/bash
The #! /bin/bash line is called the shebang or hashbang, and it is used to specify the interpreter to be used for executing the script. In this case, #!/bin/bash indicates that the script should be interpreted and executed using the Bash shell.
Open your terminal and go to the directory where you created the script file. Then run the below command to execute the script
./my_script.sh
At this stage, you won't see any output since we have not written anything other than the shebang line. Let's add some fun stuff
You can use the echo command to print statements or messages in the terminal. For example:
echo welcome to shell scripting
You will see “ welcome to shell scripting ” in your terminal
You can assign values to variables in Bash using the assignment operator (=). Here is example
name="nahid"
echo "my name is $name"
Now to execute the script and look at the ouput
User input:
To read user input in Bash, you can use the read command. It prompts you to enter a value and stores it in a variable. For example:
echo "Enter your name: "
read name
echo "Hello $name, nice to meet you bro! "
Conditional Statements:
Bash supports various conditional statements to control the flow of your script. Here are a few examples:
Simple If Statement:
name="nahid"
if [ "$name" == "nahid" ];
then
echo "Your name is nahid"
fi
#!/bin/bash
echo "Enter your name: "
read name
if [ "$name" == "nahid" ];
then
echo "Your name is nahid"
else
echo "Your name is not nahid"
fi
If Statement with Else:
name="nahid"
if [ "$name" == "nahid" ];
then
echo "Your name is nahid"
else
echo "Your name is not nahid"
fi
Else If Statement:
#!/bin/bash
echo "Enter your name: "
read name
if [ "$name" == "nahid" ]; then
echo "Your name is nahid"
elif [ "$name" == "jannat" ]; then
echo "Your name is Jannat"
else
echo "Your name is not nahid or Jannat"
fi
Below is an example of comparing two numbers using -gt operator in the shell script
NUM1=3
NUM2=5
if [ "$NUM1" -gt "$NUM2" ]
then
echo "$NUM1 is greater than $NUM2"
else
echo "$NUM1 is less than $NUM2"
fi
You can use any of the following operators to do comparisons in a shell script.
Equal to: == or =
Example: [ "$var1" == "$var2" ]
Checks if $var1 is equal to $var2.
Not equal to: !=
Example: [ "$var1" != "$var2" ]
Checks if $var1 is not equal to $var2.
Greater than: >
Example: [ "$var1" -gt "$var2" ]
Checks if $var1 is greater than $var2.
Greater than or equal to: -ge
Example: [ "$var1" -ge "$var2" ]
Checks if $var1 is greater than or equal to $var2.
Less than: <
Example: [ "$var1" -lt "$var2" ]
Checks if $var1 is less than $var2.
Less than or equal to: -le
Example: [ "$var1" -le "$var2" ]
Checks if $var1 is less than or equal to $var2.
Following is the example to check whether a file exists with the given or not
FILE="test.txt"
if [ -e "$FILE" ]
then
echo "$FILE exists"
else
echo "$FILE does not exist"
fi
Case Statement:
The case statement allows you to evaluate the value of a variable against multiple patterns and execute different blocks of code based on the matching pattern.
Following is the example:
#!/bin/bash
echo "Enter a fruit: "
read FRUIT
case "$FRUIT" in
"apple")
echo "You selected: Apple"
;;
"banana")
echo "You selected: Banana"
;;
"orange")
echo "You selected: Orange"
;;
*)
echo "Invalid fruit selection"
;;
esac
For loop
The for loop allows you to iterate over a list of values and perform a set of actions for each value.
#!/bin/bash
echo "Enter your name: "
read NAME
for ((i=1; i<=5; i++))
do
echo "Hello, $NAME!"
done
While Loop:
A while loop in a shell script allows you to repeatedly execute a block of code as long as a certain condition is true. Here is an example of for loop.
#!/bin/bash
echo "Enter your name: "
read NAME
counter=1
while [ $counter -le 5 ]
do
echo "Hello, $NAME!"
counter=$((counter+1))
done
Functions:
In shell scripting, you can define and use functions to encapsulate a block of code that can be executed multiple times throughout your script.
Function with parameters:
#!/bin/bash
greet() {
echo "Hello, I am $1 and I am $2 years old"
}
# Call the function and pass arguments
greet "nahid" "27"
Shell Scripting Mastery: Exploring Conditionals, Loops, Functions, and Useful Commands with a Mini Project
In this mini project, I have written a shell script that provides the details of the server based on the below criteria.
Welcoming the user with the username
Information regarding the Current date and time
Show the uptime of the server along with the last 5 login information.
Showing the server utilization depicting total and available space.
Information regarding top 5 CPU processes.
You can add further instructions/commands according to your needs.
Below Commands used in our project
whoami | used to display the current user. |
date | used to display the date. |
uptime | used to find out how long the system is active (running) |
head | used to print a particular number of lines of script based on argument. |
last | used to find the last login details. |
$df -h | used to get details about the disk space of the Linux System. |
free | used to get details about RAM. |
top | used to show which processes are consuming processor and memory resources. |
echo | used to display lines of text or string passed as argument. |
Date Command:
Last Login Command:
Free Space Command:
Top CPU processes Command:
Let's write the project script in our scripting file:
#!/bin/bash
echo "***************************** Welcome $(whoami) ***************************"
echo "Hi! Welcome to mastering of shell scripting."
echo "***************************************************************************"
echo "Current date : " && date |awk '{print $3 "-" $2 "-" $6}'
echo "Current time and Day:" && date | awk '{print $4, $5 "," $1}'
echo "***************************************************************************"
echo "The uptime of the server is $(uptime)"
echo "Last login details: $(last -a | head -5)"
echo "***************************************************************************"
echo "My server Utilization:"
echo "Free space is $(df -h | xargs | awk '{print $11}')"
echo "Total space is $(df -h | xargs | awk '{print $9}')"
echo "***************************************************************************"
echo "Top CPU Process: $(top | head -5)"
echo "***************************************************************************"
"Shell Scripting Mastery: Exploring Conditionals, Loops, Functions, and Useful Commands with a Mini Project" is a comprehensive guide that equips you with the skills to master shell scripting. Through practical examples and step-by-step instructions, you'll learn about user input, various types of condition statements, loops, and functions in shell scripting.
The mini project included in this article demonstrates the application of these concepts. You'll utilize useful commands like date, whoami, uptime, disk and RAM space checks, and last login details. The project covers welcoming users, displaying the current date and time, showcasing server uptime and login information, presenting server utilization, and providing insights into CPU processes based on user input.
By delving into the depths of shell scripting, this article empowers you to automate tasks and enhance your scripting abilities. Whether you're a beginner or have prior experience, this guide will expand your knowledge and enable you to leverage the power of shell scripting for better automation and efficiency.
Thank you for reading this blog. If you found this blog helpful, please like, share, and follow me for more blog posts like this in the future.
— Happy Learning !!!
Let’s connect !!!
Github
Subscribe to my newsletter
Read articles from Nahidul Islam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
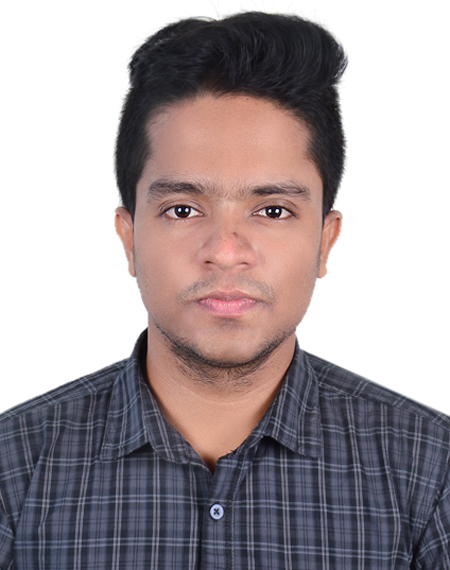
Nahidul Islam
Nahidul Islam
I am actively seeking a DevOps role and am currently available for new opportunities. With hands-on experience and project involvement in Docker, Kubernetes (K8s), CI/CD, Jenkins, AWS, and DevOps practices, I am equipped with the skills to drive efficient and scalable infrastructure solutions. I am eager to contribute my expertise and continue learning in a dynamic DevOps environment. If you have any openings or know of relevant positions, feel free to connect with me on LinkedIn to explore opportunities and learn more about my skills and experiences.