How to use the useMemo and useCallback hooks in React to optimize performance by avoiding unnecessary re-renders
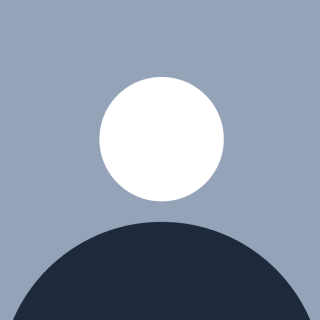
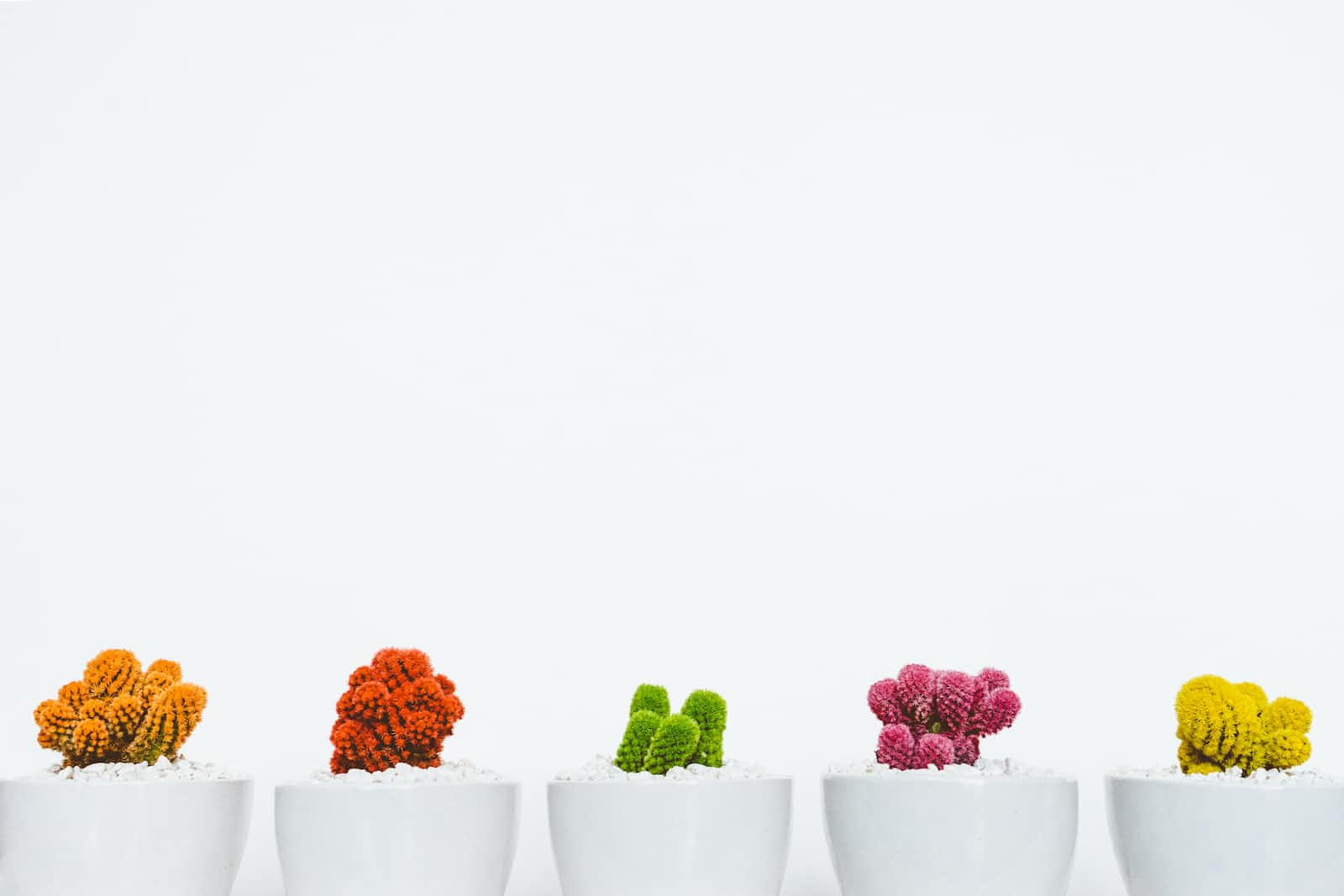
React is a powerful web development framework that lets you build complex user interfaces with ease. However, when dealing with large amounts of data or complex calculations, you may find yourself needing to optimize the performance of your components. One way to do this is by using the useMemo
and useCallback
hooks.
useMemo
allows you to memoize the result of a function call, so that it only re-runs if its dependencies change. This can be useful for expensive calculations that don't need to be run on every render. Here's an example of how to use useMemo
:
const memoizedValue = useMemo(() => expensiveFunction(a, b), [a, b]);
Copy
In this example, expensiveFunction
is an expensive function that processes the values of a
and b
. By using useMemo
, we can ensure that it only runs when either a
or b
changes.
On the other hand, useCallback
is similar to useMemo
, but it memoizes the function itself instead of its result. This can be useful for functions that are passed down as props, since it ensures that they don't trigger unnecessary re-renders. Here's an example of how to use useCallback
:
const memoizedFunction = useCallback(() => onClick(memoizedData), [onClick, memoizedData]);
Copy
In this example, onClick
is a function that is passed down as a prop, and memoizedData
is a value calculated using useMemo
. By using useCallback
, we can ensure that the onClick
function doesn't change unnecessarily and trigger a re-render of the component.
Here's an example of using useMemo
and useCallback
together in a React component to avoid unnecessary re-renders:
const MyComponent = ({ data, onClick }) => {
const memoizedData = useMemo(() => processData(data), [data]);
const memoizedFunction = useCallback(() => {
onClick(memoizedData);
}, [onClick, memoizedData]);
return (
<button onClick={memoizedFunction}>
Click me!
</button>
);
};
Copy
In this example, processData
is an expensive function that processes the data
prop. By using useMemo
, we can ensure that it only runs when the data
prop changes. Similarly, by using useCallback
, we can ensure that the onClick
function doesn't change unnecessarily and triggers a re-render of the component.
In conclusion, React provides several hooks that can help you optimize the performance of your components. By using useMemo
and useCallback
, you can ensure that expensive calculations and function calls are only executed when necessary, which can lead to significant performance improvements in your application.
Subscribe to my newsletter
Read articles from Preeti samuel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Preeti samuel
Preeti samuel
I am Kamilla Preeti Samuel, a Fullstack Developer with a strong command of JavaScript, Node.js, MongoDB, MySQL, CSS, and HTML. Over the years, I have built and worked on a range of applications, gaining valuable hands-on experience in both backend and frontend development. My professional journey includes working as a Junior Software Engineer at Bytestrum, where I focused on software development, and at NUK9 as a UX and UI Designer, contributing to creating user-centered design solutions. I thrive on building efficient, scalable, and user-friendly applications, combining technical expertise with a keen eye for design. I enjoy collaborating with cross-functional teams to create seamless digital experiences, and I am passionate about continuously exploring new tools and frameworks to stay ahead in the fast-evolving tech landscape. I am Kamilla Preeti Samuel, a full-stack developer with a strong command of JavaScript, Node.js, MongoDB, MySQL, CSS, and HTML. Over the years, I have built and worked on various applications, gaining valuable hands-on experience in both backend and frontend development. My professional journey includes working as a Junior Software Engineer at Bytestrum, where I focused on software development, and at NUK9 as a UX and UI Designer, contributing to creating user-centered design solutions. I thrive on building efficient, scalable, and user-friendly applications, combining technical expertise with a keen eye for design. I enjoy collaborating with cross-functional teams to create seamless digital experiences, and I am passionate about continuously exploring new tools and frameworks to stay ahead in the fast-evolving tech landscape.