Understanding Callback Functions and Avoiding Callback Hell in JavaScript
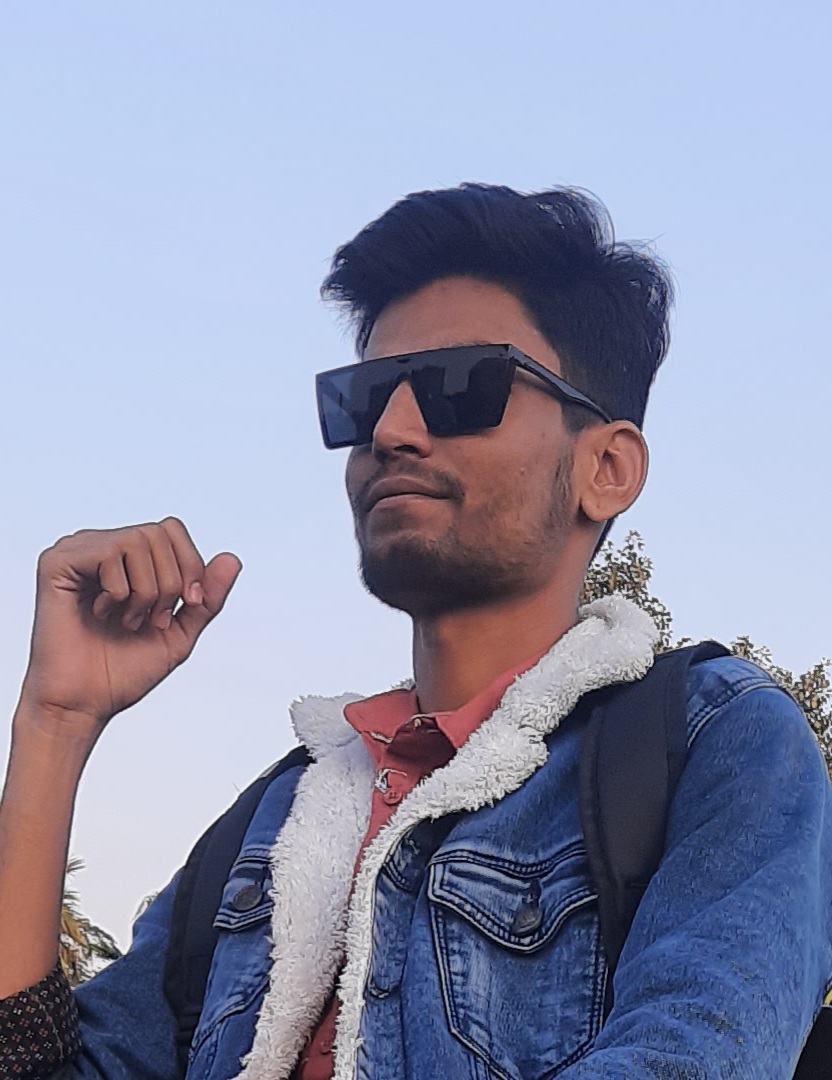
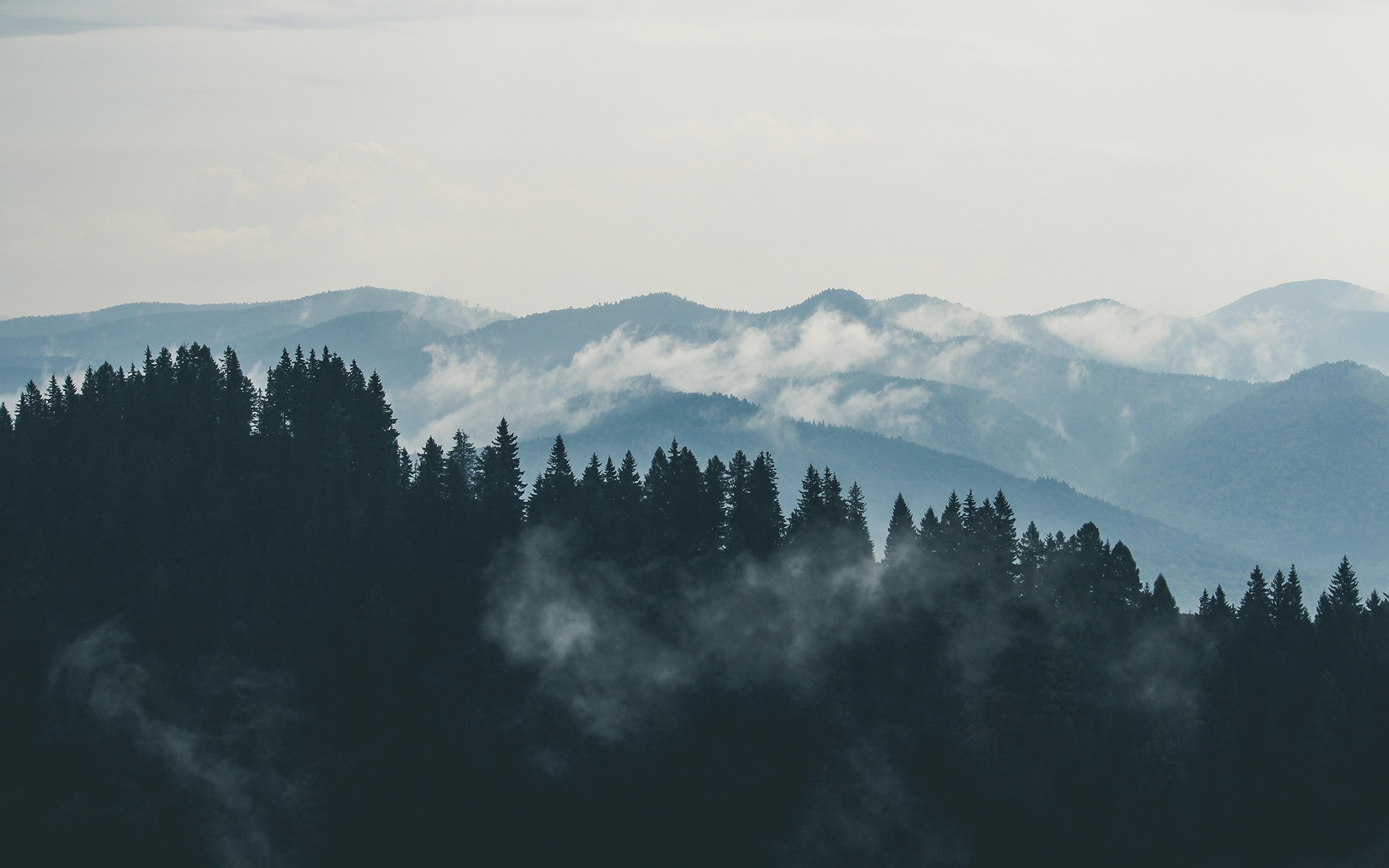
Introduction
JavaScript is a versatile programming language that employs various techniques to handle asynchronous operations. One of the essential concepts in JavaScript for managing asynchronous code is callback functions. In this blog post, we will delve into the world of callback functions, their purpose, and how to avoid the notorious "callback hell" phenomenon.
What are Callback Functions?
A callback function, in simple terms, is a function that is passed as an argument to another function and gets executed at a later point in time. Callback functions are commonly used in JavaScript to handle asynchronous operations, such as reading and writing files, making API requests, and handling events.
How Callback Functions Work
In JavaScript, functions are first-class citizens, meaning they can be assigned to variables, passed as arguments, and returned from other functions. This flexibility allows us to pass functions as arguments to other functions, including callback functions. When an asynchronous operation is completed, the callback function is called with the result.
javascriptCopy codefunction fetchData(callback) {
// Simulating an asynchronous API request
setTimeout(() => {
const data = { name: 'John', age: 30 };
callback(data);
}, 1000);
}
function processData(data) {
console.log('Received data:', data);
}
fetchData(processData);
In the example above, we have a `fetchData`
function that simulates an asynchronous API request using `setTimeout`
. It takes a callback function as an argument, and after one second, it calls the callback function with the retrieved data. The `processData`
function is passed as the callback function, and when the data is received, it logs the data to the console.
Common Use Cases for Callback Functions
Callback functions are prevalent in JavaScript programming. Some common use cases include event handling, AJAX requests, and setTimeout/setInterval functions. They allow us to define what should happen after an asynchronous operation completes successfully or encounters an error.
javascriptCopy code// Event handling
document.addEventListener('click', function(event) {
console.log('Clicked!', event);
});
// AJAX request with callback
function makeRequest(url, callback) {
// Simulating an AJAX request
setTimeout(() => {
const response = { data: 'Some data' };
callback(response);
}, 1000);
}
makeRequest('https://api.example.com', function(response) {
console.log('Received response:', response);
});
Introducing Callback Hell
Callback hell refers to a situation where multiple nested callback functions make the code difficult to read, understand, and maintain. When handling complex asynchronous operations, the callback functions tend to pile up, resulting in deeply nested code structures that are hard to follow.
javascriptCopy codeasyncOperation1(function(result1) {
asyncOperation2(result1, function(result2) {
asyncOperation3(result2, function(result3) {
// More nested callbacks...
});
});
});
Pitfalls and Challenges of Callback Hell
Callback hell can lead to several issues, including code duplication, decreased readability, and increased chances of introducing bugs. It becomes challenging to manage error handling, handle control flow, and maintain the codebase as the complexity grows.
Solving Callback Hell
a) Using Named Functions:
Breaking down complex callback structures by using named functions can enhance code readability and maintainability. Instead of defining anonymous functions inline, named functions can be declared and passed as callback arguments.
javascriptCopy codefunction asyncOperation1(callback) {
// Async operation 1
callback(result1);
}
function asyncOperation2(result, callback) {
// Async operation 2 using result
callback(result2);
}
function asyncOperation3(result, callback) {
// Async operation 3 using result
callback(result3);
}
function finalOperation(result) {
// Perform final operation using result
}
asyncOperation1(function(result1) {
asyncOperation2(result1, function(result2) {
asyncOperation3(result2, function(result3) {
finalOperation(result3);
});
});
});
In the code snippet above, we have defined named functions (`asyncOperation1`
, `asyncOperation2`
, `asyncOperation3`
, and `finalOperation`
) to handle different stages of the asynchronous operations. By using named functions, the code structure becomes clearer, and it is easier to understand the flow of execution.
b) Modularization and Code Organization:
Breaking down large blocks of code into smaller, modular functions can help mitigate callback hell. Separating concerns and organizing code into manageable functions allows for better code comprehension and maintainability.
javascriptCopy codefunction asyncOperation1(callback) {
// Async operation 1
callback(result1);
}
function asyncOperation2(result, callback) {
// Async operation 2 using result
callback(result2);
}
function asyncOperation3(result, callback) {
// Async operation 3 using result
callback(result3);
}
function finalOperation(result) {
// Perform final operation using result
}
function handleAsyncOperations() {
asyncOperation1(function(result1) {
asyncOperation2(result1, function(result2) {
asyncOperation3(result2, function(result3) {
finalOperation(result3);
});
});
});
}
handleAsyncOperations();
By encapsulating the async operations and their respective callbacks within a modular function (`handleAsyncOperations`
), the code becomes more organized and easier to manage.
c) Promises and Asynchronous JavaScript:
Promises provide an alternative approach to managing asynchronous code. Promises allow for more structured and readable code by chaining functions using `.then()`
and `.catch()`
methods. Promises enable a more linear flow and simplify error handling.
javascriptCopy codefunction asyncOperation1() {
return new Promise(function(resolve, reject) {
// Async operation 1
resolve(result1);
});
}
function asyncOperation2(result) {
return new Promise(function(resolve, reject) {
// Async operation 2 using result
resolve(result2);
});
}
function asyncOperation3(result) {
return new Promise(function(resolve, reject) {
// Async operation 3 using result
resolve(result3);
});
}
function finalOperation(result) {
// Perform final operation using result
}
asyncOperation1()
.then(asyncOperation2)
.then(asyncOperation3)
.then(finalOperation)
.catch(function(error) {
// Handle errors
});
In the above example, each async operation returns a promise object. The .then()
method is used to chain the operations together, ensuring that each operation is executed in sequence. The `finalOperation`
function is called after all the promises are resolved. Error handling is done using the `.catch()`
method.
d) Async/Await:
Introduced in ECMAScript 2017, the async/await syntax offers a more elegant way to write asynchronous code. By using the `async`
keyword to define an asynchronous function and `await`
to pause execution until a promise settles, developers can write code that appears synchronous while maintaining the benefits of asynchronous operations.
javascriptCopy codeasync function asyncOperation1() {
// Async operation 1
return result1;
}
async function asyncOperation2(result) {
// Async operation 2 using result
return result2;
}
async function asyncOperation3(result) {
// Async operation 3 using result
return result3;
}
function finalOperation(result) {
// Perform final operation using result
}
async function handleAsyncOperations() {
try {
const result1 = await asyncOperation1();
const result2 = await asyncOperation2(result1);
const result3 = await asyncOperation3(result2);
finalOperation(result3);
} catch (error) {
// Handle errors
}
}
handleAsyncOperations();
In the code snippet above, the async
keyword is used to define asynchronous functions (`asyncOperation1`
, `asyncOperation2`
, `asyncOperation3`
, and `handleAsyncOperations`
). The await
keyword is used to pause execution until each async operation is resolved. This allows the code to appear more sequential and readable, resembling synchronous code. Error handling is done using a `try-catch`
block.
Best Practices for Using Callback Functions
To effectively use callback functions, it is essential to follow some best practices:
Keep your code modular and organized.
Avoid deeply nested callback structures.
Handle errors properly within callback functions.
Leverage control flow libraries like async.js or use modern JavaScript features like Promises or async/await.
Consider using libraries or frameworks that provide utilities for managing asynchronous code, such as Axios for HTTP requests or Node.js `
fs`
module for file operations.
Conclusion
Callback functions are a crucial part of JavaScript when dealing with asynchronous operations. However, if not handled properly, they can lead to callback hell, making the code difficult to read and maintain. By using techniques like named functions, modularization, Promises, and async/await, developers can avoid callback hell and write cleaner, more readable asynchronous code. Following best practices and leveraging modern JavaScript features will result in more maintainable and efficient codebases.
Subscribe to my newsletter
Read articles from Sawai Singh Rajpurohit directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
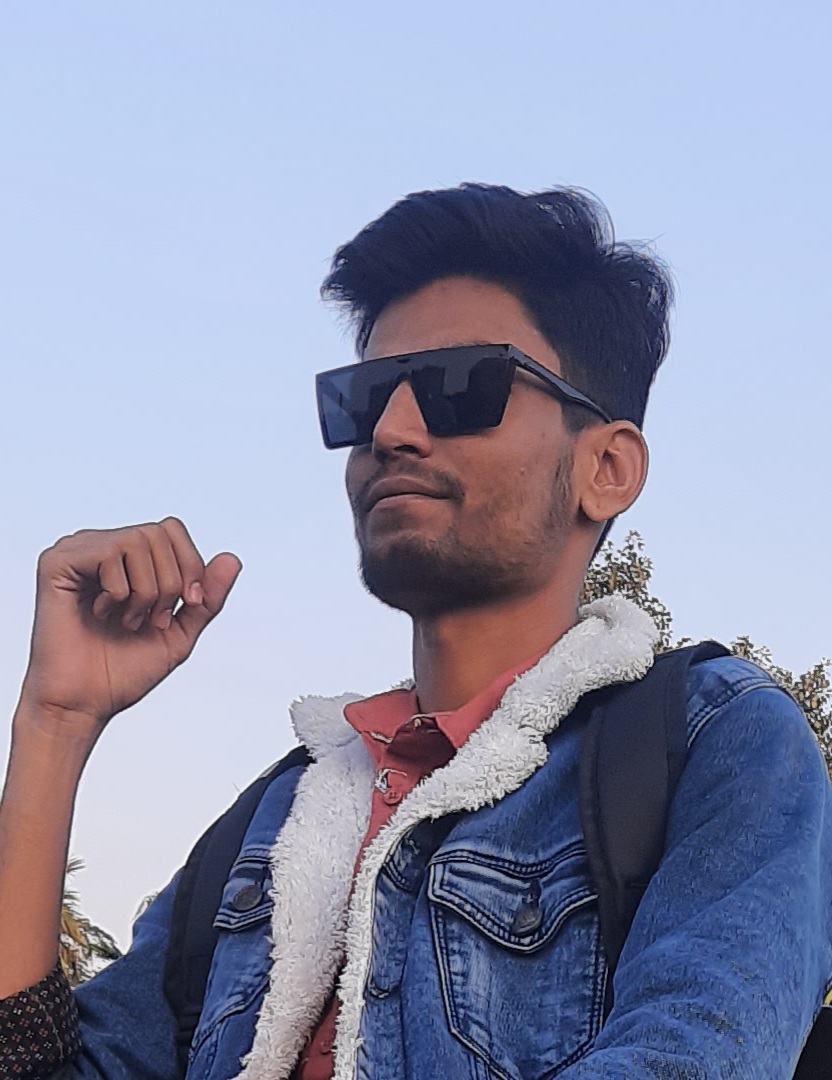
Sawai Singh Rajpurohit
Sawai Singh Rajpurohit
I am a curiosity-driven software engineer who likes to write. This blog is a constant work in progress. ๐