Demystifying React State and Props: Understanding their usage, differences, and similarities

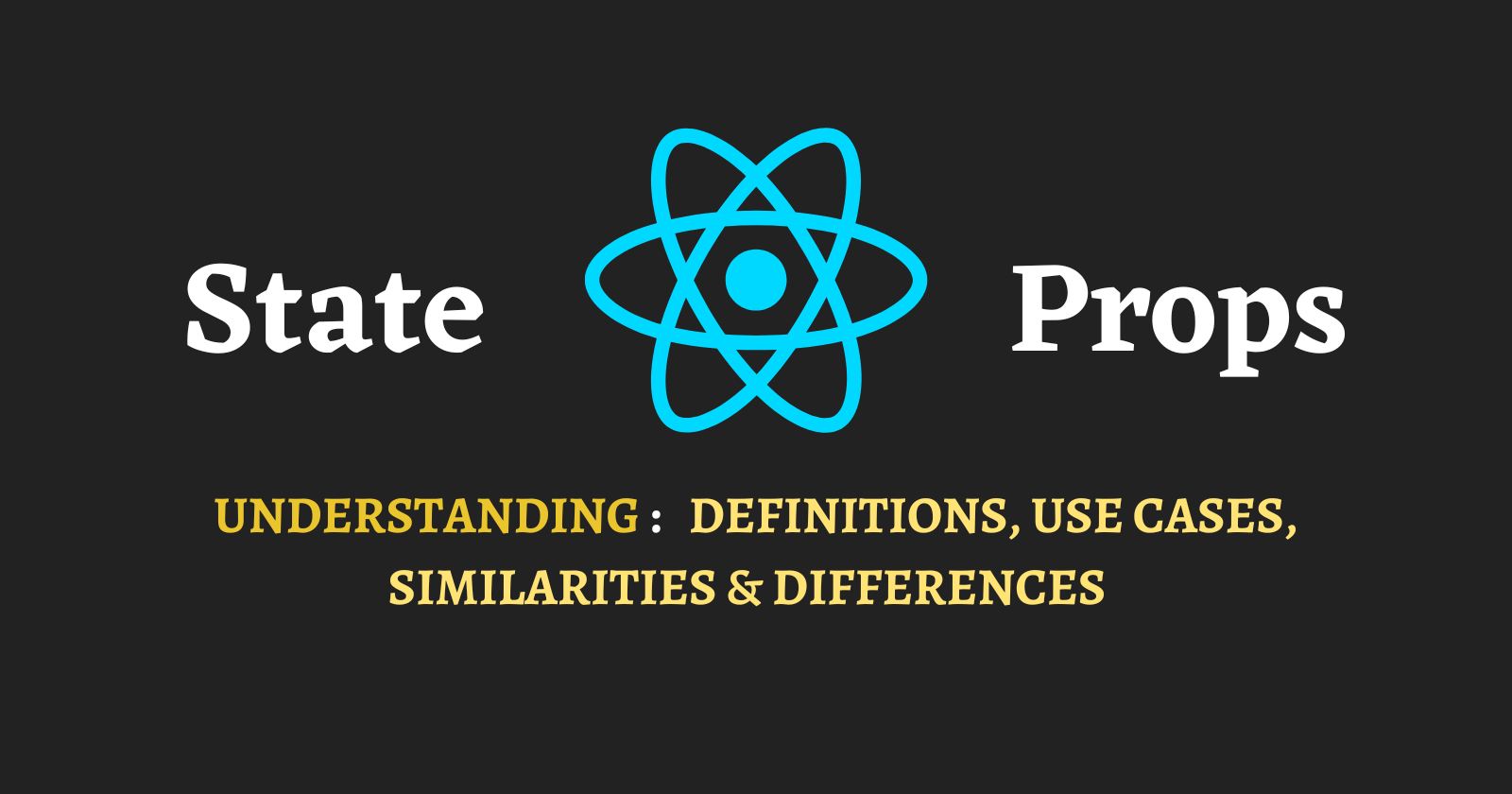
Introduction:
React is a popular JavaScript library used for building user interfaces. It introduces two important concepts for managing and passing data between components: state and props. In this beginner-friendly article, we'll explore the usage, differences, and similarities of React state and props. By the end, you'll have a solid understanding of these concepts and how to utilize them effectively in your React applications.
Section 1: Understanding React State
Keywords: React state, managing state, useState hook, updating state
The first concept we'll explore is React state. State represents the data that a component maintains and can change over time. It enables components to have dynamic behavior and reflect changes in the user interface.
In React functional components, we use the useState
hook to introduce and manage state. This hook provides a way to define and update state in a concise manner. Here's an example:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
In the above example, we initialize the state count
to 0 using the useState
hook. The count
variable represents the current value of the state, and setCount
is a function provided by useState
to update the state. Whenever setCount
is called, React will re-render the component with the updated value.
Section 2: Exploring React Props
Keywords: React props, passing data, component communication, component composition
Now, let's dive into React props. Props (short for properties) are inputs to a component that are passed from its parent component. They provide a way to pass data and behavior from parent components to their children.
Here's an example to illustrate how props are used:
import React from 'react';
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
function App() {
return <Greeting name="Alice" />;
}
In this example, the Greeting
component receives a prop called name
from its parent component, App
. Props are accessed using the props
object within the component. In this case, the component renders "Hello, Alice!" as the output.
Props allow components to be reusable and compose with other components to build complex UI structures. They create a unidirectional flow of data and facilitate communication between components in a React application.
Section 3: Differences and Similarities
Keywords: React state vs props, comparison, component ownership
Now that we understand state and props individually, let's examine their differences and similarities.
Location and Ownership:
State is defined and managed within a component, making it local to that component.
Props are passed from parent components down to child components and are owned and controlled by the parent.
Mutability:
State can be updated and modified within the component using the appropriate state updating methods.
Props are read-only and cannot be modified within the component receiving them.
Communication:
State allows components to manage their internal data and reflect changes in the user interface.
Props enable communication between components, as they provide a way to pass data and behavior from parent to child components.
Conclusion:
React state and props are essential concepts for building robust and dynamic user interfaces. State enables components to manage and update their internal data, while props facilitate communication and data flow between components. Understanding the differences and similarities between state and props is crucial for writing maintainable and reusable React components.
By grasping these concepts, you'll be equipped to utilize state and props effectively, leading to more efficient React development and enhanced user experiences.
Remember, state and props are fundamental building blocks of React, and mastering them will empower you to create powerful and interactive applications.
Subscribe to my newsletter
Read articles from Debmalya Sen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Debmalya Sen
Debmalya Sen
I'm a frontend web developer, learning MERN stack and DSA ! My main programming language is JavaScript. I love JavaScript and learning Backend! I would love to work on Open Source Projects and do Freelancing Works! I'm from Kolkata, West Bengal , India. I'm currently a 12th grade High School commerce Student, but I love Programming and Web Development so much & wanna make a career in it! I have a Web & Digital Marketing Agency "OCEANEEK" (www.oceaneek.com/) I love Web Development and exploring other Tech Stacks ! I have a Youtube channel "debmalya sen" and I run a podcast show "The SenCast " there on Youtube! Thanks for bearing me :)