Python for Beginners: Part 3 - Exploring Data Types
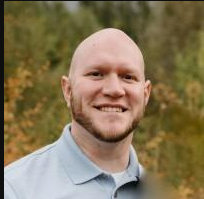
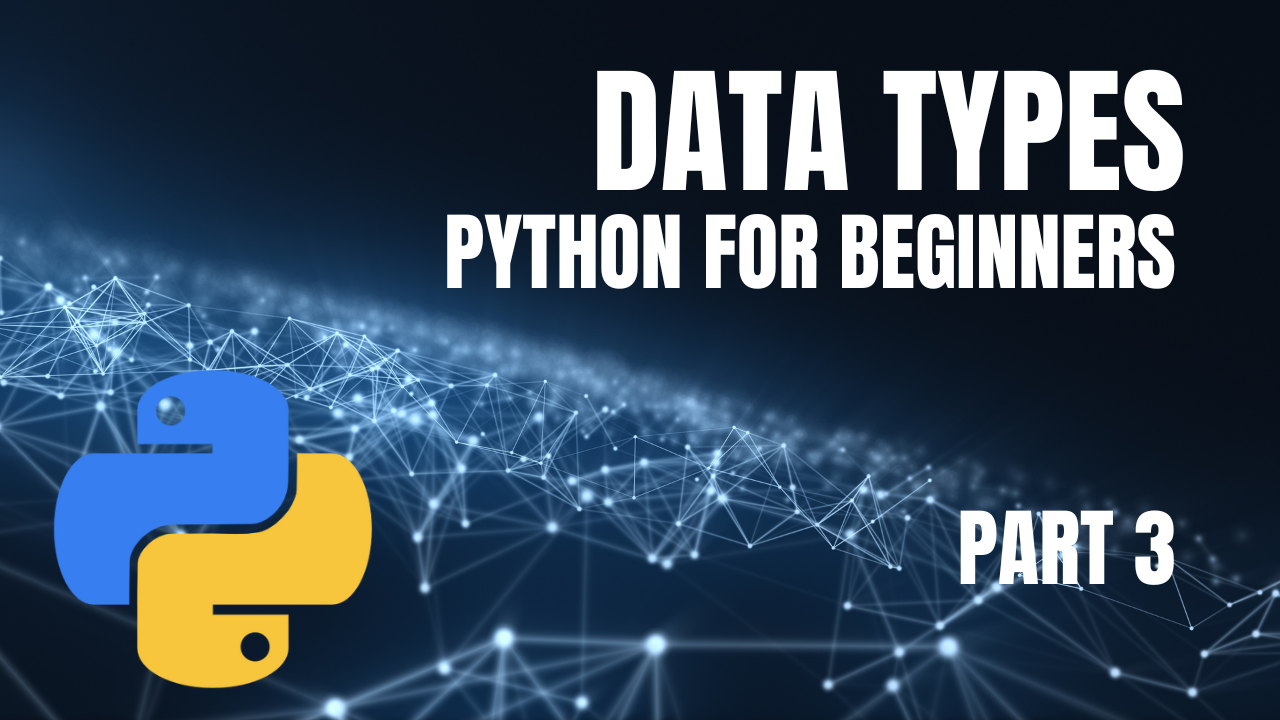
Welcome back, everyone! Today, we're going to dive a little deeper into the world of Python programming by exploring data types. Just like different tools for building a house have different uses and purposes, data types in Python help us organize and work with different kinds of information. So, let's get with Python data types!
Understanding Data Types
In Python, there are several primary data types we use to represent different kinds of information. Let's explore three common data types: numbers, text, and boolean.
1. Numbers
Numbers in Python can be of two types: integers (whole numbers) and floating-point numbers (numbers with decimal points). Here are some examples:
Integer: 7, 15, 42
Floating-Point: 3.14, 2.5, 0.5
We can perform mathematical operations on numbers, such as addition, subtraction, multiplication, and division. Let's take a look at an example:
# Performing arithmetic operations
x = 10
y = 4
addition = x + y
subtraction = x - y
multiplication = x * y
division = x / y
# Displaying the results
print("Addition:", addition)
print("Subtraction:", subtraction)
print("Multiplication:", multiplication)
print("Division:", division)
2. Text (Strings)
Text in Python is represented by a data type called a string. Strings are enclosed in single quotes ('') or double quotes (""). Here are some examples:
"Hello, world!"
'Python is fun!'
"I'm learning coding."
We can perform various operations on strings, such as concatenation (joining strings together) and accessing individual characters. Let's see an example:
# Working with strings
name = "Alice"
greeting = "Hello, " + name + "!"
character = name[0] # Accessing the first character of the name
# Displaying the results
print(greeting)
print("The first character of my name is:", character)
3. Boolean
Boolean data type represents truth values: either true or false. Booleans are often used in decision-making and control structures. Here are some examples:
True
False
We can use comparison operators to compare values and obtain boolean results. Let's see an example:
# Working with booleans
x = 5
y = 10
is_greater = x > y
is_equal = x == y
# Displaying the results
print("Is x greater than y?", is_greater)
print("Is x equal to y?", is_equal)
Conclusion
Congratulations, young coders! You've learned about different data types in Python: numbers, text (strings), and booleans. You've seen how we can perform operations on numbers, manipulate strings, and use booleans for decision-making.
Data types are like building blocks that help us work with various types of information in our programs. In the next post, we'll continue our coding journey by exploring loops, a powerful concept that allows us to repeat actions.
Stay curious and keep coding!
Subscribe to my newsletter
Read articles from Matthew Hard directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
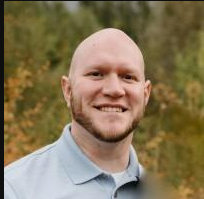
Matthew Hard
Matthew Hard
I'm Matthew, a cybersecurity enthusiast, programmer, and networking specialist. With a lifelong passion for technology, I have dedicated my career to the world of cybersecurity, constantly expanding my knowledge and honing my skills. From a young age, I found myself captivated by the intricate workings of computers and networks. This fascination led me to pursue in-depth studies in the fields of networking and cybersecurity, where I delved deep into the fundamental principles and best practices. Join me on this exciting journey as we explore the multifaceted world of technology together. Whether you're a beginner or a seasoned professional, I am here to share my knowledge, discuss the latest trends, and engage in insightful discussions. Together, let's embrace the ever-changing world of tech and navigate the complexities of cybersecurity with confidence and expertise.