JavaScript Closures Made Easy: Simple and Effective Examples for Rapid Learning
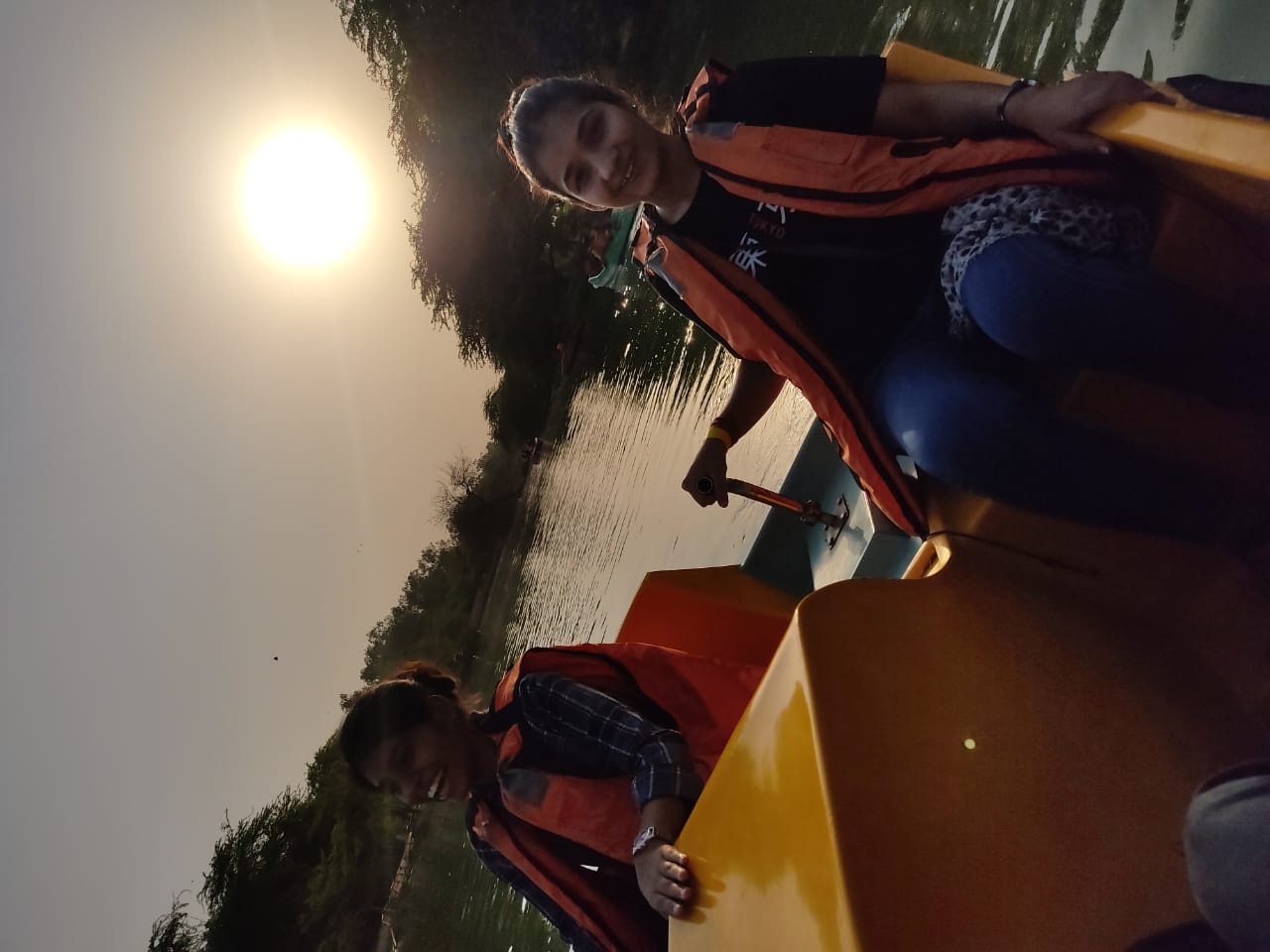
JavaScript is a versatile and powerful programming language that is widely used for web development. One of the key concepts in JavaScript that developers often encounter is closures. Closures can be a bit challenging to understand initially, but once you grasp the concept, they can become a powerful tool in your JavaScript arsenal. In this article, we will explore closures in JavaScript and provide you with simple and effective examples to help you rapidly learn and apply closures in your own code.
To start with, let's define what closure is in the context of JavaScript. A closure is created when a function is defined inside another function and has access to variables defined in the outer function, even after the outer function has finished executing. In simpler terms, a closure "remembers" its lexical scope, including the variables, even when it is executed outside of that scope.
To better understand closures, let's dive into some practical examples.
Example 1: Basic Closure
javascriptCopy codefunction outerFunction() {
let outerVariable = 'I am from the outer function';
function innerFunction() {
console.log(outerVariable);
}
return innerFunction;
}
const closure = outerFunction();
closure(); // Output: I am from the outer function
In this example, outerFunction
contains a variable called outerVariable
and a nested function called innerFunction
. The innerFunction
has access to the outerVariable
, even after the outerFunction
has finished executing. When we call outerFunction
, it returns the innerFunction
, which we then assign to the variable closure
. Finally, when we invoke closure
, it prints the value of outerVariable
, demonstrating that the closure has retained access to the outer variable.
Example 2: Counter using Closure
javascriptCopy codefunction createCounter() {
let count = 0;
return function() {
return ++count;
};
}
const counter1 = createCounter();
console.log(counter1()); // Output: 1
console.log(counter1()); // Output: 2
const counter2 = createCounter();
console.log(counter2()); // Output: 1
In this example, we have a createCounter
function that returns an anonymous function. The anonymous function has access to the count
variable defined in the outer function. Every time we call the returned function, it increments the count
variable and returns the updated value. We create two separate counters (counter1
and counter2
) using createCounter
, and each counter maintains its own independent count. This showcases how closures can be used to create private variables in JavaScript.
Example 3: Data Encapsulation with Closures
javascriptCopy codefunction createPerson(name, age) {
return {
getName: function() {
return name;
},
getAge: function() {
return age;
},
setName: function(newName) {
name = newName;
},
setAge: function(newAge) {
age = newAge;
}
};
}
const person = createPerson('John Doe', 30);
console.log(person.getName()); // Output: John Doe
console.log(person.getAge()); // Output: 30
person.setName('Jane Smith');
person.setAge(25);
console.log(person.getName()); // Output: Jane Smith
console.log(person.getAge()); // Output: 25
In this example, we use closures to encapsulate data within a function. The createPerson
the function takes in the name and age as parameters and returns an object with four methods: getName
, getAge
, setName
, and setAge
. These methods have access to the name
and age
variables due to closures. The getName
and getAge
methods return the corresponding values, while the setName
and setAge
methods allow us to update the values of name
and age
, respectively. This encapsulation ensures that the data is protected and can only be accessed or modified through the defined methods, providing a level of abstraction and control over the object's properties.
Example 4: Event Handling with Closures
javascriptCopy codefunction addClickHandlers() {
const buttons = document.querySelectorAll('button');
for (let i = 0; i < buttons.length; i++) {
const button = buttons[i];
button.addEventListener('click', function() {
console.log('Button ' + (i + 1) + ' clicked!');
});
}
}
addClickHandlers();
In this example, we have a function addClickHandlers
that adds click event handlers to a collection of buttons on a web page. Inside the for
loop, we define a click event handler function using an anonymous function. The closure created by this anonymous function allows us to access the loop variable i
inside the event handler, even after the loop has finished executing. As a result, when a button is clicked, it logs the corresponding button number to the console. Without closures, each event handler would log the value of i
as the final value after the loop.
Closures are not only powerful but also essential for many JavaScript patterns and techniques. They enable us to create private variables, encapsulate data, and provide a level of abstraction and control over our code. By understanding closures, you can enhance your JavaScript skills and write more flexible and maintainable code.
In conclusion, closures are a fundamental concept in JavaScript that allows functions to retain access to variables defined in their lexical environment, even after the outer function has finished executing. Through simple and effective examples, we have explored how closures work and how they can be used in practical scenarios such as creating private variables, encapsulating data, and handling events. By mastering Javascript closures, you will have a powerful tool at your disposal to write cleaner, more modular, and more efficient JavaScript code.
Remember, practice is key when it comes to mastering closures. Experiment with different examples and scenarios to deepen your understanding of how closures work and their practical applications. With time and practice, closures will become second nature, and you will be able to leverage your power to write more robust and elegant JavaScript code.
References:
Subscribe to my newsletter
Read articles from Krishna Kumari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
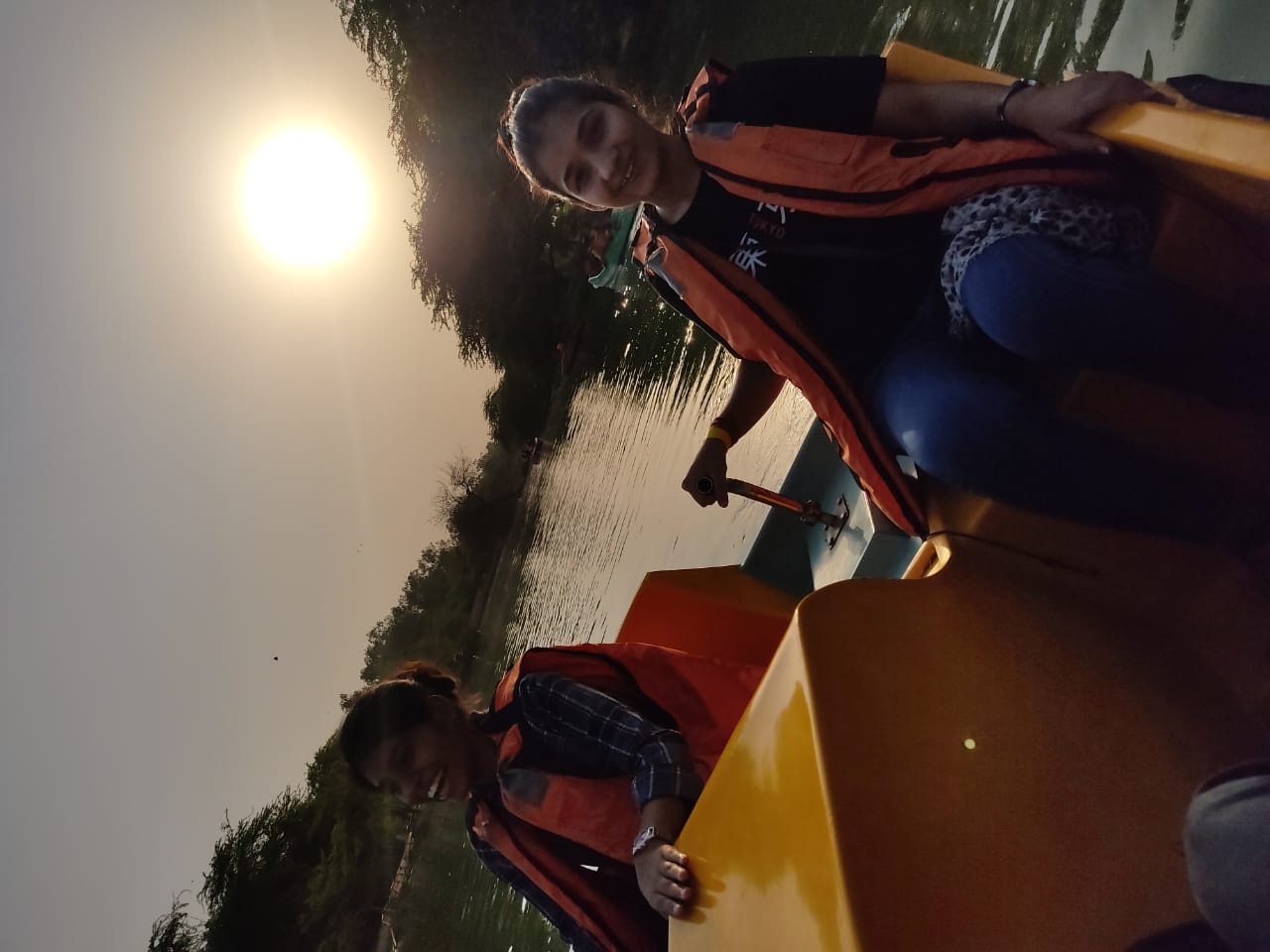
Krishna Kumari
Krishna Kumari
I am a content writer and social media analyst.