round() in python

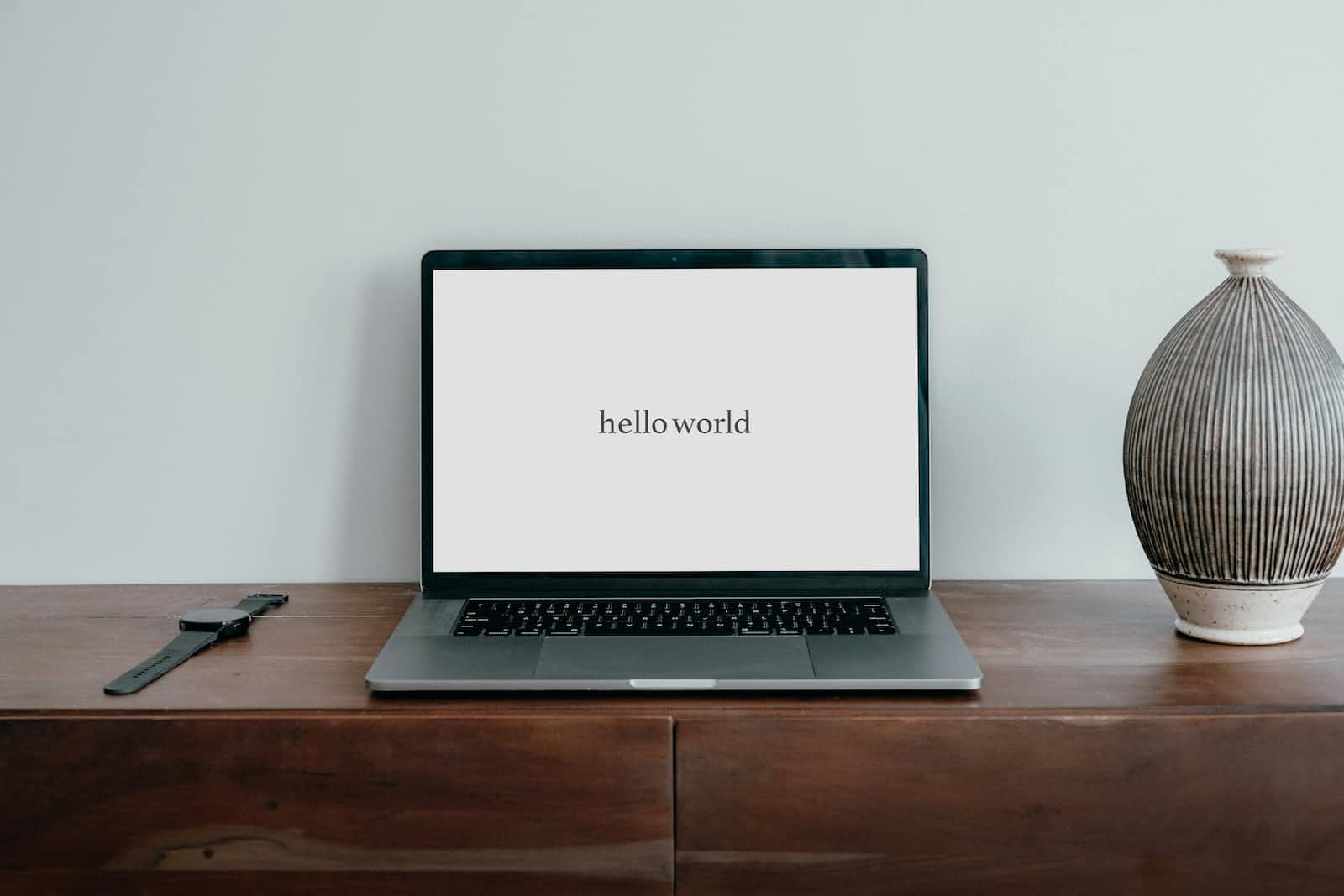
The round() function is used for rounding off numbers up to a specified number of digits after the decimal point.
Syntax:
round(number, digits)
number - the number to be rounded. It is a required parameter.
digits - the number of decimals to use when rounding the number. This is an optional parameter. The default is 0.
Return value:
The round() function's return value depends upon the value of digits. It always returns a number that is either a float or an integer.
x = 3.14159
rounded_1 = round(x) # Rounds to the nearest whole number
print(rounded_1) # returns 3
rounded_2 = round(x, 2) # Rounds to 2 decimal places
print(rounded_2) # returns 3.14
rounded_3 = round(x, 4) # Rounds to 4 decimal places
print(rounded_3) # returns 3.1416
If the input is an integer, the return value is also an integer.
If the input is float, the output depends on digits.
If digits is not specified, an integer is returned, else a floating-point number is returned.
Note:
If there are two equally close values to round to, python will round to the nearest even value.
y = 2.5
rounded_4 = round(y) # Rounding 2.5 to the nearest whole number
print(rounded_4) # returns 2
Here, round() rounds 2.5 to the nearest whole number, which is 2. Since 2 and 3 are equally close, it rounds down to the nearest even number. This behaviour is known as "round half to even" or "ties to even."
Subscribe to my newsletter
Read articles from Parvathi Somasundaram directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
