π’ Using Web3.js to Interact with Smart Contracts: A Beginner's Guide!

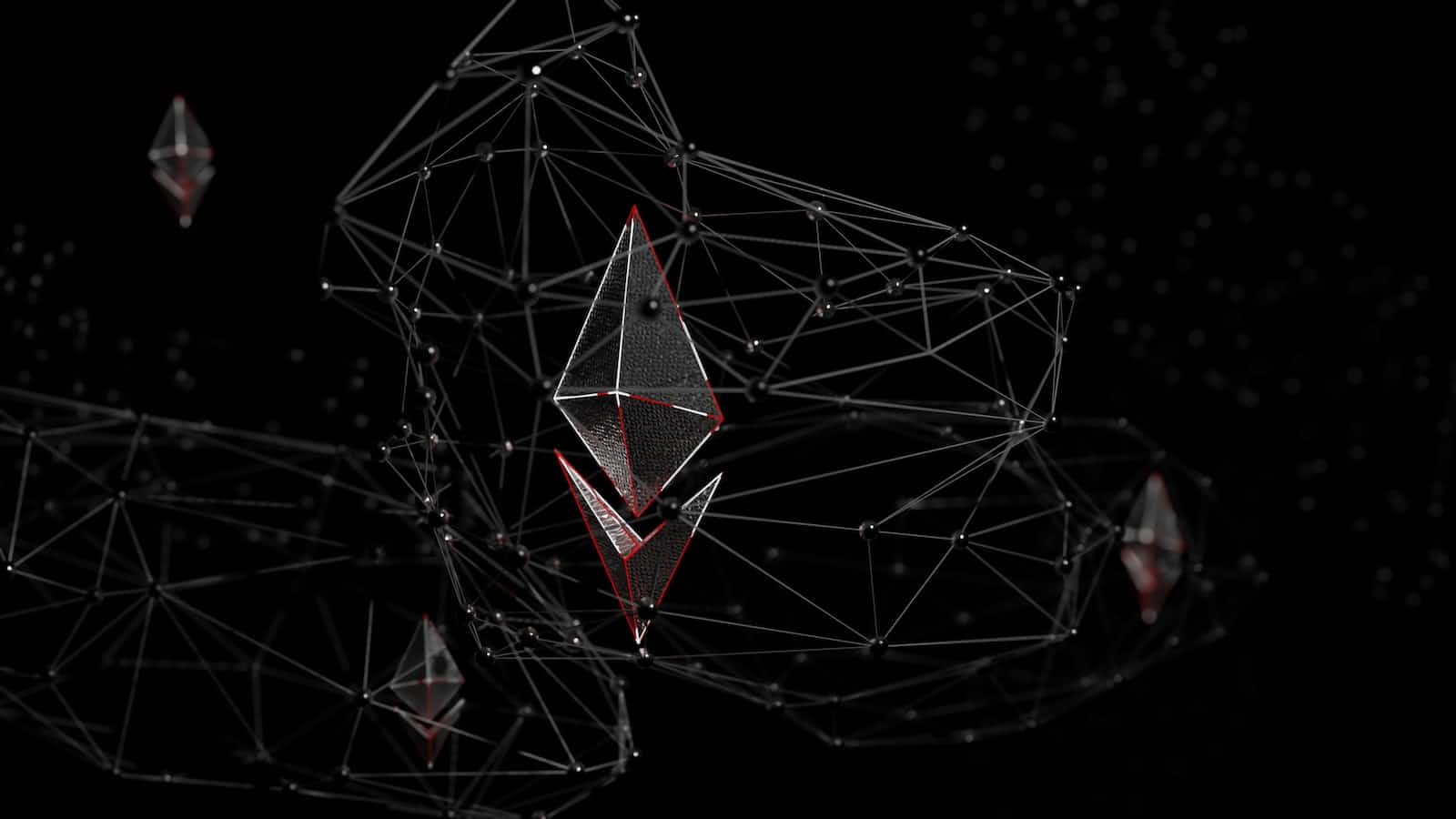
Imagine walking into a secure, automated store πͺ - where you don't need a human cashier. Instead, you select your items, and when you're ready, you go to the checkout, and the system knows exactly what items you picked up. Now, imagine this happening on the Internet - that's what a smart contract on the blockchain is like. It's an agreement that automatically verifies and executes transactions according to predefined rules.
In this post, we're going to learn how to interact with these smart contracts using a JavaScript library called Web3.js. π―
Setting Up Web3.js
First, you need to include web3.js in your project. It's as easy as adding the following line to your HTML file:
<script src="https://cdn.jsdelivr.net/gh/ethereum/web3.js/dist/web3.min.js"></script>
Or installing via npm:
npm install web3
Connecting to Ethereum Node
Just like how you need to connect to a WiFi network to access the Internet, you need to connect to an Ethereum node to interact with the Ethereum network. One popular service is Infura.
var Web3 = require('web3');
var web3 = new Web3('https://mainnet.infura.io/YOUR_INFURA_KEY');
Interacting with Smart Contracts
Now we're ready to start interacting with smart contracts. Imagine you're renting a digital locker in our virtual store. There's a smart contract that handles this process.
First, you need the smart contract's ABI (Application Binary Interface), which is like a menu of the contract's functions you can call, and its address.
var contractABI = [{"constant":true,
"inputs":[],
"name":"get",
"outputs":[{
"name":"",
"type":"uint256"
}],
"payable":false,
"type":"function"},
{"constant":false,
"inputs":[{"name":"x",
"type":"uint256"
}],
"name":"set",
"outputs":[],
"payable":false,
"type":"function"}];
// This is just a sample
var contractAddress = '0xYourContractAddress';
Next, we instantiate the contract using our previously defined web3 instance:
var contract = new web3.eth.Contract(contractABI, contractAddress);
Now you're all set! You can start interacting with the smart contract.
Say you want to rent a locker. The contract might have a rentLocker
function that you could call like this:
contract.methods.rentLocker(5).send({from: 'Your-Ethereum-Address'})
.then(function(receipt){
console.log(receipt);
});
Just like that, you've rented a locker using a smart contract!
Remember, this is a very basic example. Interacting with smart contracts can get complex, but web3.js makes it much easier. Be sure to read the [official web3.js documentation](https://web3js.readthedocs.io/) to learn more.
Stay tuned for our next post on creating and deploying your own smart contracts! πΌπ»
Subscribe to my newsletter
Read articles from Joshua Obafemi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Joshua Obafemi
Joshua Obafemi
Software Developer || Web3 Advocate