Getting Real-Time Notifications in React with Pusher
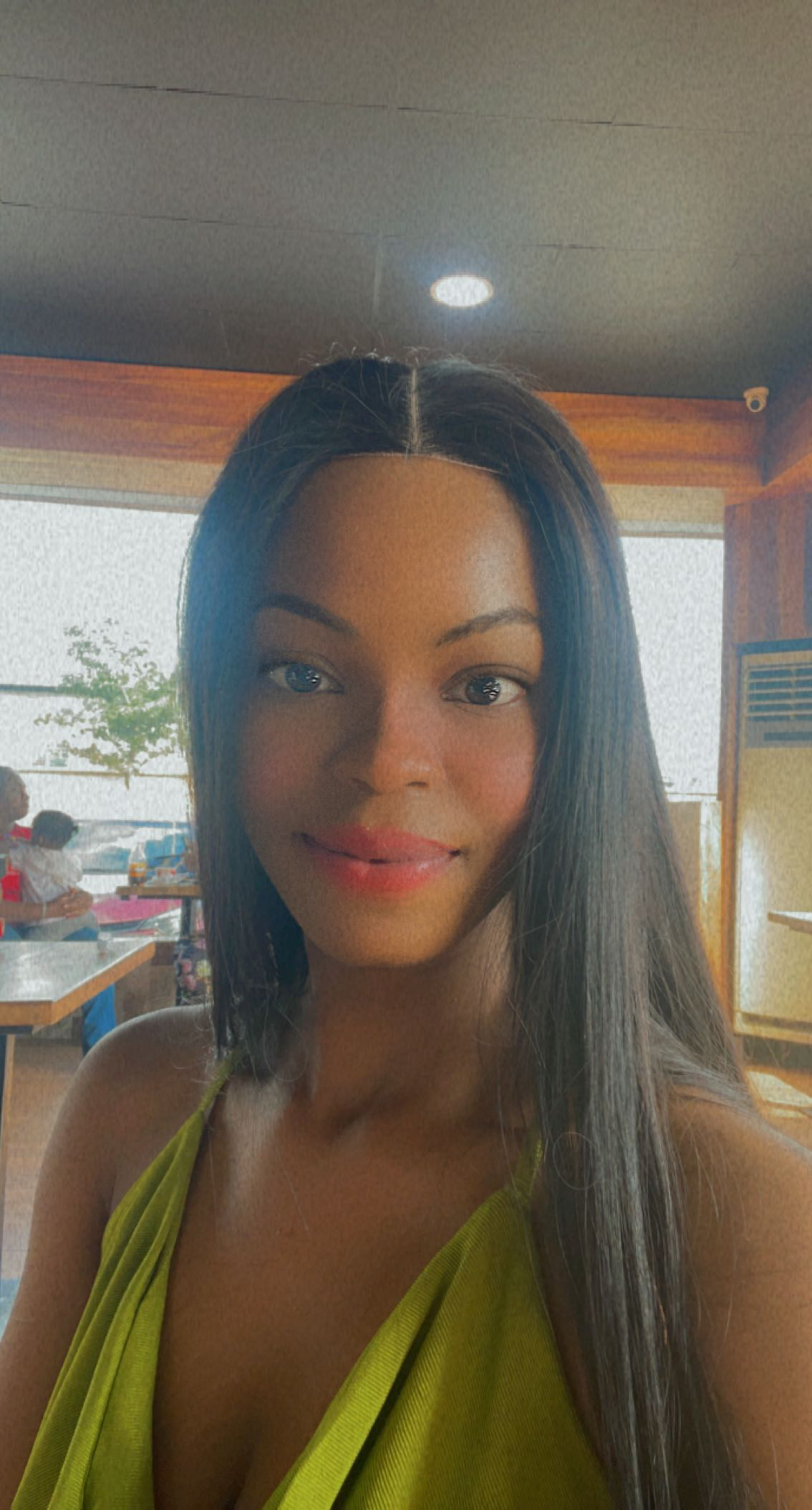
Introduction
Real-time notifications play a crucial role in keeping users engaged and informed within web applications. With the combination of React, a popular JavaScript library for building user interfaces, and Pusher, a real-time messaging service, developers can easily implement real-time notification features. In this article, we will explore how to integrate Pusher with React to achieve real-time notifications and keep users up to date with dynamic content.
Table Of Content
What is Pusher?
Setting up a Pusher Account
Creating a React Application
Installing and Configuring Pusher
Handling Real-Time Notifications
Displaying Real-Time Notifications in React
Conclusion
What is Pusher?
Pusher is a cloud-based service that enables real-time communication and event-driven updates in web applications. React, on the other hand, is a popular JavaScript library for building user interfaces. By combining these technologies, we can create dynamic and interactive applications that provide real-time notifications to users.
Setting up a Pusher Account
To begin, visit the Pusher website (https://pusher.com) and sign up for a free account. Once registered, create a new application within your Pusher dashboard. This process will provide you with API keys (app ID, key, and secret) necessary for configuring your React application to use Pusher.
Creating a React Application
Before integrating Pusher, let's set up a React application as our development environment. We'll utilize Create React App, a popular tool for quickly setting up React projects. Open your terminal and execute the following command:
npx create-react-app pusher-react-app
This command creates a new directory named pusher-react-app
with the basic structure of a React application.
Installing and Configuring Pusher
Within the pusher-react-app
directory, install the Pusher JavaScript client library using npm or yarn:
npm install pusher-js
or
yarn add pusher-js
Next, open the src
directory and create a new file named PusherComponent.js
. Import the Pusher library in this file:
import Pusher from 'pusher-js';
Within the PusherComponent.js
file, create a new function-based component named PusherComponent
. In the useEffect()
hook, establish a connection to Pusher, and subscribe to a channel:
import React, { UseEffect } from 'react';
import Pusher from 'pusher-js';
function PusherComponent {
useEffect() {
const pusher = new Pusher('<your-app-key>', {
cluster: '<your-cluster>',
encrypted: true,
forceTLS: true,
channelAuthorization: {
endpoint: `<your-backend-endpoint>`,
transport: 'ajax',
headers: {
Accept: 'application/json',
Authorization: `Bearer <your-token>`,
},
},
});
const channel = pusher.subscribe('<channel-name>');
// Bind to an event
channel.bind('<event-name>', (data) => {
// Handle the new notification
console.log(data);
});
// Clean up the Pusher instance when the component unmounts
return () => {
pusher.unsubscribe('<event-name>');
pusher.disconnect();
};
}, []);
return <div>PusherComponent</div>;
}
export default PusherComponent;
Replace <your-app-key>
with your Pusher app key, <your-cluster>
with the Pusher cluster, your app is hosted on, <channel-name>
with the name of the channel you want to subscribe to, and <event-name>
with the name of the event, you want to listen to.
Handling Real-Time Notifications
To handle the real-time notifications received from Pusher, we need to store them in our application state or dispatch them to a global state management solution like Redux. Let's update our PusherComponent.js
file to handle notifications using React's useState
hook:
import React, { useEffect, useState} from 'react';
import Pusher from 'pusher-js';
function PusherComponent {
const [notifications, setNotifications] = useState([]);
useEffect() {
const pusher = new Pusher('<your-app-key>', {
cluster: '<your-cluster>',
encrypted: true,
forceTLS: true,
channelAuthorization: {
endpoint: `<your-backend-endpoint>`,
transport: 'ajax',
headers: {
Accept: 'application/json',
Authorization: `Bearer <your-token>`,
},
},
});
const channel = pusher.subscribe('<channel-name>');
// Bind to an event
channel.bind('<event-name>', (data) => {
// Update notifications state with the new notification
setNotifications((prevNotifications) => [
...prevNotifications,
data,
]);
});
// Clean up the Pusher instance when the component unmounts
return () => {
pusher.unsubscribe('<event-name>');
pusher.disconnect();
};
}, []);
return (
<div>
<h1>Real-Time Notifications</h1>
{notifications.map((notification, index) => (
<div key={index}>{notification.message}</div>
))}
</div>
);
}
export default PusherComponent;
The updated code uses the useState
hook to store the notifications array and updates it whenever a new notification is received.
Displaying Real-Time Notifications in React
In the main App.js
file of your React application, import the PusherComponent
:
import React from 'react';
import PusherComponent from './PusherComponent';
const App = () => {
return (
<div>
<h1>My App</h1>
<PusherComponent />
</div>
);
};
export default App;
The PusherComponent
is now rendered within the App
component, displaying real-time notifications as they are received.
Conclusion
In this article, we explored how to integrate Pusher into a React application to enable real-time notifications. By leveraging Pusher's capabilities and React's hooks, we established a connection to Pusher, subscribed to a notification channel, and displayed real-time notifications as they were received. Real-time notifications are a powerful way to engage users and enhance the interactivity of your React applications, opening up possibilities for various real-time use cases.
Subscribe to my newsletter
Read articles from Onyenekwe Elizabeth directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
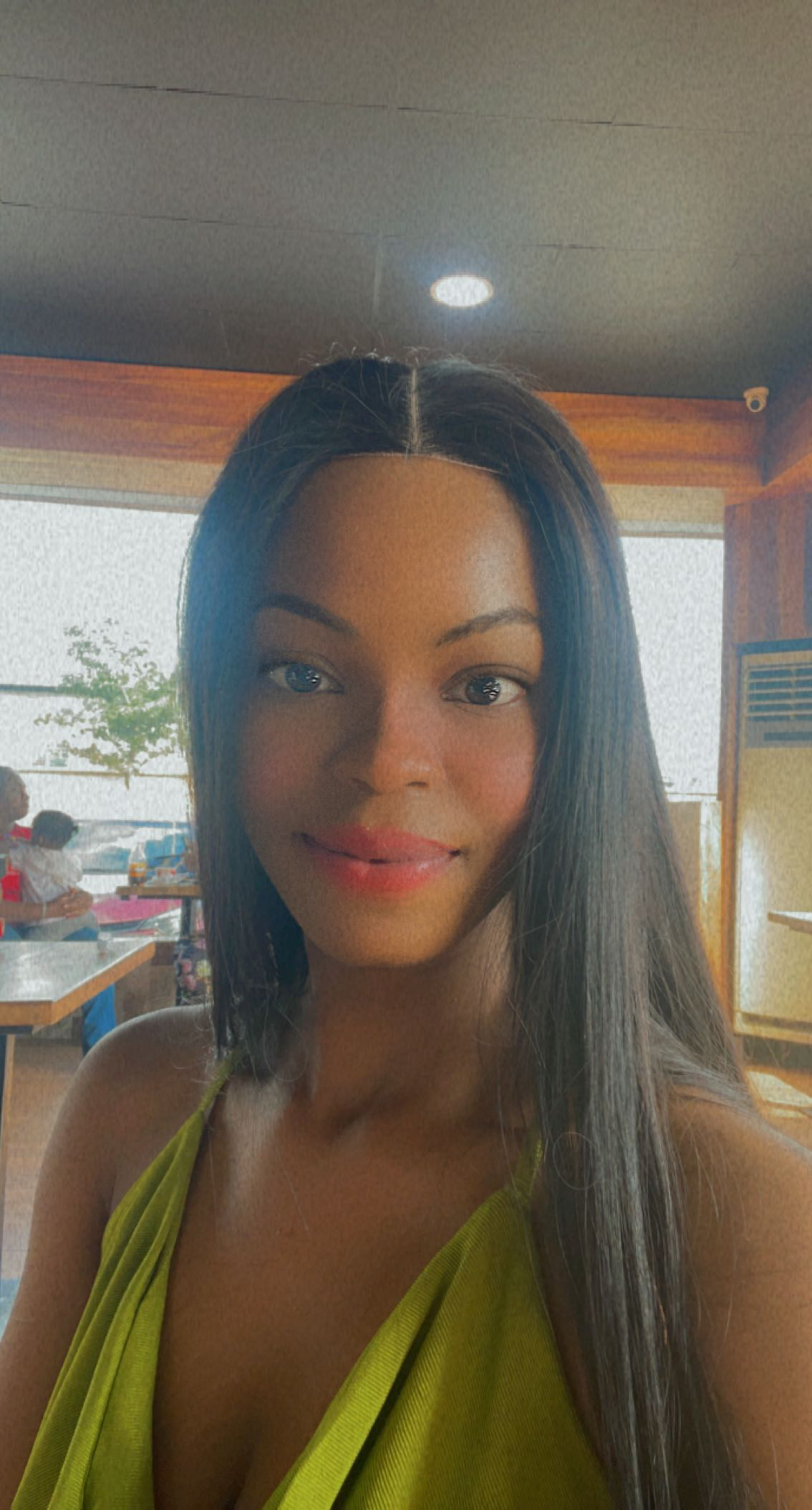
Onyenekwe Elizabeth
Onyenekwe Elizabeth
Experienced Frontend Developer with a passion for creating engaging and user-friendly websites and applications. Skilled in HTML, CSS, JavaScript, Vue, React, and React native. Proven track record of delivering high-quality projects on time.