Dagger2 with Java Example: Employee and Attendance Tracker
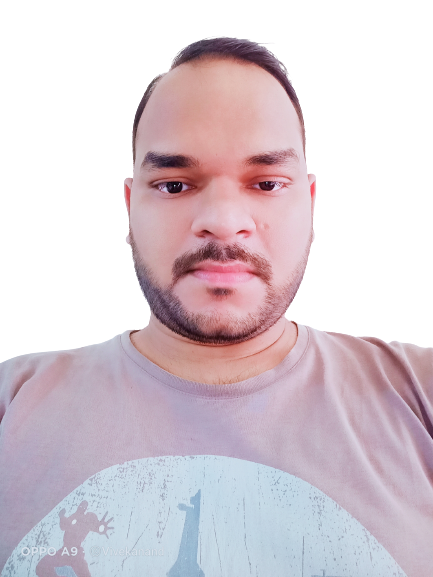
Table of contents
Introduction: In this cheatsheet, we'll explore how to use Dagger, a dependency injection framework, to build an Employee and Attendance Tracker application in Java. We'll cover the essential concepts and provide code examples to help you understand how Dagger can simplify dependency management in your project.
Setting up Dagger:
Add the Dagger dependencies to your project's build.gradle file.
Create a new Java class called
AppModule
and annotate it with@Module
.Inside the module, define methods annotated with
@Provides
to provide instances of dependencies, such as the EmployeeRepository and AttendanceTracker.
Define Dependencies:
Create a Java interface called
EmployeeRepository
to handle employee-related data operations, such as fetching employee details.Create a Java class called
AttendanceTracker
to handle attendance tracking functionalities, such as marking employee attendance.
Inject Dependencies:
Annotate the constructor of the
AttendanceTracker
class with@Inject
to indicate that it requires an instance ofEmployeeRepository
.Annotate the
EmployeeRepository
interface with@Inject
to indicate that it should be injected.
Create a Component:
Create a Java interface called
AppComponent
and annotate it with@Component
.Specify the modules to be used by the component using the
modules
attribute, including theAppModule
.Declare an injection method to inject dependencies into the
AttendanceTracker
class.
Build the Component and Inject Dependencies:
In your main application class, create an instance of
AppComponent
usingDaggerAppComponent.builder().build()
.Use the
AppComponent
instance to obtain an instance ofAttendanceTracker
with injected dependencies.
Example Code:
// AppModule.java
@Module
public class AppModule {
@Provides
public EmployeeRepository provideEmployeeRepository() {
return new EmployeeRepositoryImpl();
}
@Provides
public AttendanceTracker provideAttendanceTracker(EmployeeRepository employeeRepository) {
return new AttendanceTracker(employeeRepository);
}
}
// EmployeeRepository.java
public interface EmployeeRepository {
List<Employee> getAllEmployees();
Employee getEmployeeById(int id);
// Other employee-related methods
}
// AttendanceTracker.java
public class AttendanceTracker {
private EmployeeRepository employeeRepository;
@Inject
public AttendanceTracker(EmployeeRepository employeeRepository) {
this.employeeRepository = employeeRepository;
}
// Other methods for attendance tracking
}
// AppComponent.java
@Component(modules = AppModule.class)
public interface AppComponent {
void inject(AttendanceTracker attendanceTracker);
}
// Main.java
public class Main {
public static void main(String[] args) {
AppComponent appComponent = DaggerAppComponent.builder().build();
AttendanceTracker attendanceTracker = new AttendanceTracker();
appComponent.inject(attendanceTracker);
// Use the attendanceTracker instance with injected dependencies
attendanceTracker.trackAttendance();
}
}
Conclusion: Dagger is a powerful tool for managing dependencies in Java applications. In this cheatsheet, we explored how to set up Dagger, define dependencies, and inject them using a simple example of an Employee and Attendance Tracker application. By leveraging Dagger's capabilities, you can make your code more modular, maintainable, and testable. Remember to refer to the official Dagger documentation for more advanced features and use cases.
Happy coding and enjoy exploring Dagger in your projects!
Subscribe to my newsletter
Read articles from Vivekanand R Yadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
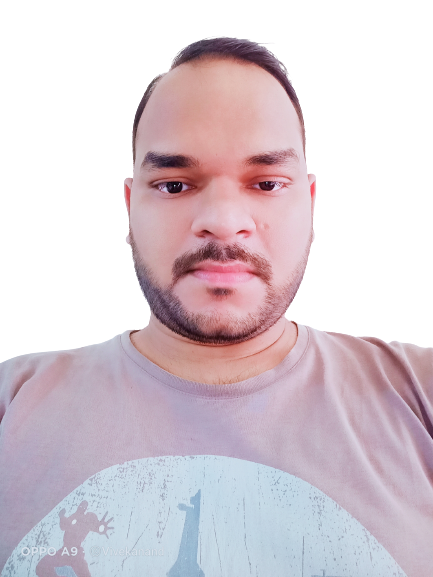
Vivekanand R Yadav
Vivekanand R Yadav
I am a skilled software engineer with expertise in automation engineering, specializing in infrastructure, Test Framework Development and DevOps. With a strong command of Golang, Java, and Python, I have worked across diverse industries including healthcare, human resources, auditing and assurance, manufacturing, and banking. My experience in these industries has allowed me to design and implement efficient automated solutions tailored to their specific needs. As an effective problem-solver and collaborator, I drive automation initiatives and deliver high-quality results across various industry domains.