AIDL : IPC, The Android way
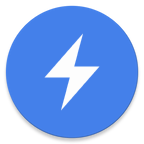
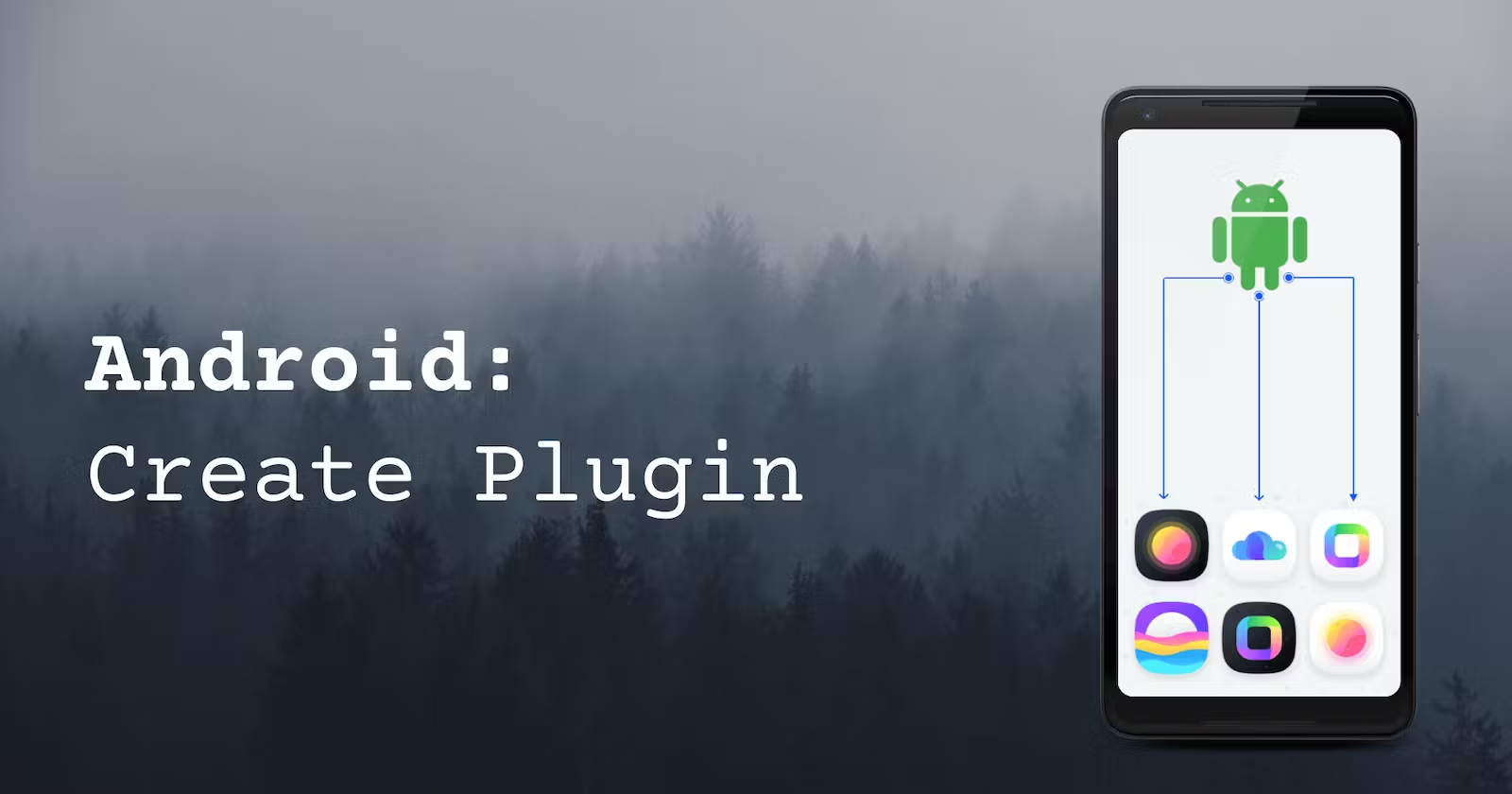
The Android Interface Definition Language (AIDL) is similar to other IDLs: it lets you define the programming interface that both the client and service agree upon in order to communicate with each other using interprocess communication (IPC).
Aidl consists of 3 parts:
AIDL file
Client application
Server application
Creating AIDL file
Enable build feature in your
build.gradle
.buildFeatures { aidl = true }
Create a new AIDL file using
File
->New
->AIDL
->AIDL File
Let's name it
ICalculator.aidl
Declare aidl methods:
interface IExampleAidlInterface { int add(int a, int b); }
Create an AIDL server
An AIDL server is an app that does the actual work and sends back the result to requesting clients. One service can connect with multiple clients via Binders.
Steps:
Create a service, e.g.
CalculatorService extends Service
Create an Implementation of
ICalculator.Stub
, lets name itCalculatorImpl
Implement methods:
object CalculatorImpl : ICalculator.Stub() { override fun add(a: Int, b: Int): Int { return a + b } }
Expose your stub implementation with a service binder:
class CalculatorService : Service() { override fun onBind(intent: Intent): IBinder { return CalculatorImpl } }
Now your service is ready to be consumed by your clients, now let's create a client application.
Create an AIDL client
An AIDL client is an app that binds to an AIDL service, calls API, and shows a response inside its views.
Steps:
Create a service connection:
private var aidlService: ICalculator? = null private val serviceConnection = object : ServiceConnection { override fun onServiceConnected(name: ComponentName?, service: IBinder?) { aidlService = ICalculator.Stub.asInterface(service) Toast.makeText(applicationContext, "Service Connected", Toast.LENGTH_LONG).show() } override fun onServiceDisconnected(name: ComponentName?) { aidlService = null Toast.makeText(applicationContext, "Service Disconnected", Toast.LENGTH_LONG).show() } }
Connect to AIDL service via Binders.
val serviceIntent = Intent() serviceIntent.component = ComponentName(BuildConfig.AIDL_PACKAGE, BuildConfig.AIDL_SERVICE) bindService(serviceIntent, serviceConnection, BIND_AUTO_CREATE)
Call methods of AIDL after successful connection:
aidlService?.add(2, 2)?.let { Toast.makeText( applicationContext, String.format(Locale.getDefault(), "Sum is: %d", it), Toast.LENGTH_LONG ).show() }
Limitations
There is one BIG gotcha with AIDL services, which is when the system is in doze mode, your service app might not start to respond to requests.
Here is an example application using AIDL in real life ๐
Subscribe to my newsletter
Read articles from Tej Pratap Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
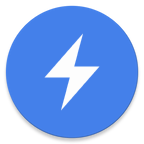
Tej Pratap Singh
Tej Pratap Singh
Code, Movies and code for movies