Unraveling the Java Mysteries: Unveiling the Gotchas of Java Programming
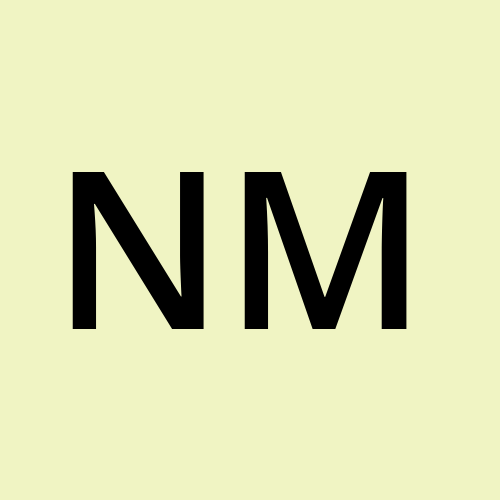
Introduction:
Welcome, curious programmers, to a thrilling journey into the realm of Java programming! Java, a widely used and versatile programming language, has been embraced by developers across the globe. While it boasts a reputation for its simplicity and robustness, some hidden surprises can trip up even the most experienced Java wizards. In this article, we will delve into the intriguing world of Java's "gotchas" – those unexpected quirks and pitfalls that can leave you scratching your head. Fasten your seatbelts and prepare for an exhilarating exploration of the Gotchas of Java!
The Case of the Infamous Floating-Point Arithmetic:
One of the most notorious Java gotchas lies within the realm of floating-point arithmetic. Due to the inherent imprecision of floating-point numbers, seemingly straightforward calculations may yield unexpected results. Consider the following code snippet:
double result = 0.1 + 0.2;
System.out.println(result);
The expected result might be 0.3, but brace yourself for a surprise. Java's binary representation of floating-point numbers can introduce rounding errors, resulting in a value like 0.30000000000000004. To mitigate this, it is advisable to use the BigDecimal class when precise decimal calculations are required.
The Enigma of String Equality:
Strings are fundamental in Java programming, but comparing them can be trickier than meets the eye. The == operator in Java compares the memory addresses of objects, rather than their contents. Consider this puzzling code snippet:
String str1 = "Hello";
String str2 = new String("Hello");
System.out.println(str1 == str2);
Surprisingly, the result of this comparison is false! Why? When using the new keyword, Java creates a new object, resulting in different memory addresses for str1 and str2. To compare string content, use the equals() method instead:
System.out.println(str1.equals(str2));
The Cryptic Switch Statement:
Ah, the humble switch statement, known for its ability to simplify complex branching. However, Java's switch statement has some peculiar behaviors. Consider the following code:
int day = 5;
switch (day) {
case 1:
System.out.println("Monday");
case 5:
System.out.println("Friday");
break;
case 7:
System.out.println("Sunday");
break;
default:
System.out.println("Invalid day");
}
You might expect only "Friday" to be printed, right? Wrong! Without explicit break statements, Java's switch statement falls through to subsequent cases. In this example, "Friday" and "Invalid day" will be printed. To prevent this, remember to include break statements after each case.
The Mystical Loop: Loops are vital for iterative tasks, but one particular loop construct in Java can be mystifying. Consider this code snippet:
int i = 0;
while (i < 5) {
System.out.println("Loop!");
i++;
continue;
System.out.println("Unreachable");
}
What will happen? Surprisingly, the line System.out.println("Unreachable"); is indeed unreachable! The continue statement causes control to immediately jump to the next iteration of the loop, skipping any subsequent code within the loop block. This unexpected behavior can lead to confusion, so be cautious when using continue within a loop.
The Conundrum of Null References: Ah, the dreaded null reference—a common source of frustration for Java developers. Consider the following snippet:
String message = null;
System.out.println(message.length());
You might expect a null pointer exception, right? Surprisingly, the code will indeed throw a NullPointerException. Remember that accessing methods or properties of a null reference will result in this exception. Always check for null values before performing operations to avoid such mishaps.
Conclusion:
Java, with all its power and versatility, hides a few surprises up its sleeve. By familiarizing ourselves with these Java gotchas, we can avoid the pitfalls and become more proficient programmers. Remember to tread carefully when it comes to floating-point arithmetic, string equality, switch statements, loops, and null references. The journey through Java's intricacies is a thrilling one, and with a little caution, you'll be well-equipped to tackle any Java puzzle that comes your way. Happy coding!
Subscribe to my newsletter
Read articles from Naveen Metta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
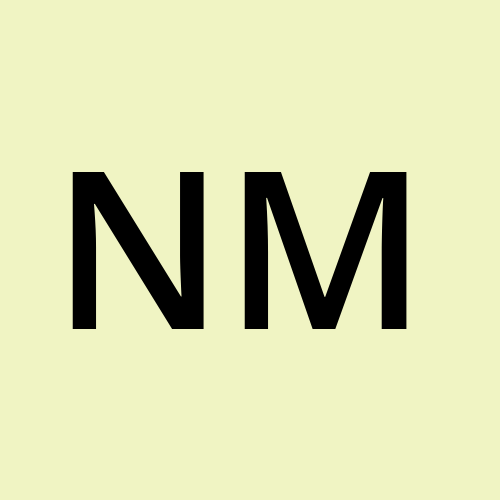