Python Operators.
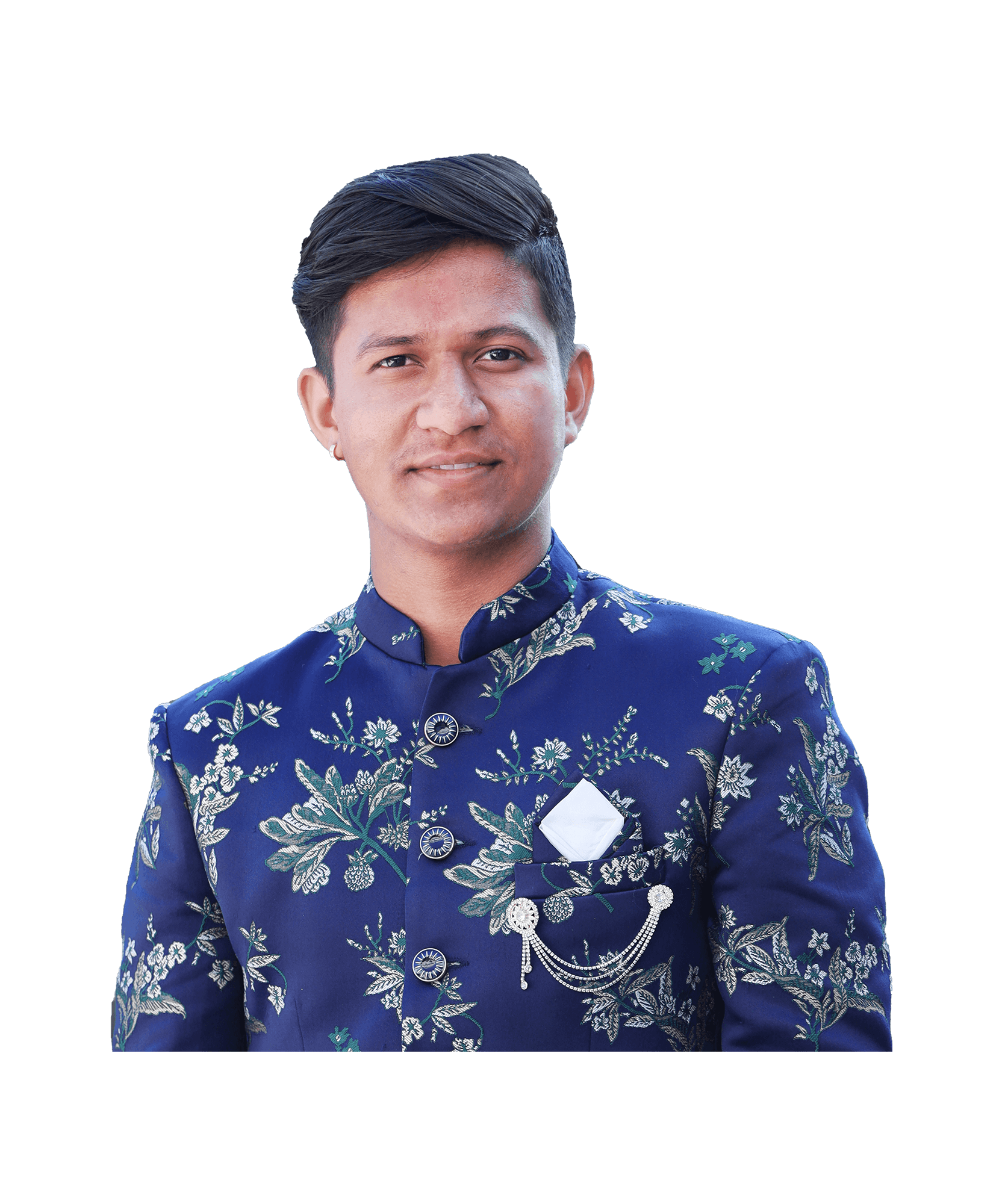
Table of contents
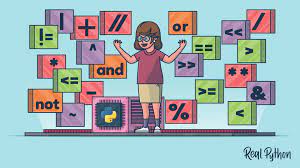
What are the Python Operators?
In Python, operators are symbols or special symbols that perform operations on one or more operands to produce a result. They are used to manipulate data and perform various computations. Python supports a wide range of operators, including arithmetic, comparison, assignment, logical, bitwise, and more. These operators allow you to perform tasks such as mathematical calculations, comparisons, logical operations, and manipulating bits.
Types of Python Operators.
Arithmetic Operators: +, -, , /, %, *, //
Comparison Operators: ==, !=, >, <, >=, <=
Assignment Operators: =, +=, -=, \=, /=, %=, *=, //=
Logical Operators: and, or, not
Bitwise Operators: &, |, ^, ~, <<, >>
Membership Operators: in, not in
Identity Operators: is, is not
Unary Operators: +, -
Ternary Operator: expr1 if condition else expr2
Arithmetic Operators:
Addition (+): Performs the addition of two operands.
Subtraction (-): Performs subtraction of the second operand from the first.
Multiplication (*): Performs multiplication of two operands.
Division (/): Performs division of the first operand by the second.
Modulo (%): Returns the remainder after the division of the first operand by the second.
Exponentiation (**): Raises the first operand to the power of the second.
Floor Division (//): Performs division and returns the integer quotient without the remainder.
Example:
x = 10 y = 3 addition = x + y subtraction = x - y multiplication = x * y division = x / y modulo = x % y exponentiation = x ** y floor_division = x // y print(addition) # Output: 13 print(subtraction) # Output: 7 print(multiplication) # Output: 30 print(division) # Output: 3.3333333333333335 print(modulo) # Output: 1 print(exponentiation) # Output: 1000 print(floor_division) # Output: 3
Comparison Operators:
Equal to (==): Checks if the operands are equal.
Not equal to (!=): Checks if the operands are not equal.
Greater than (>): Checks if the first operand is greater than the second.
Less than (<): Checks if the first operand is less than the second.
Greater than or equal to (>=): Checks if the first operand is greater than or equal to the second.
Less than or equal to (<=): Checks if the first operand is less than or equal to the second.
Example:
x = 5 y = 3 is_equal = x == y is_not_equal = x != y is_greater_than = x > y is_less_than = x < y is_greater_than_or_equal = x >= y is_less_than_or_equal = x <= y print(is_equal) # Output: False print(is_not_equal) # Output: True print(is_greater_than) # Output: True print(is_less_than) # Output: False print(is_greater_than_or_equal) # Output: True print(is_less_than_or_equal) # Output: False
Assignment Operators:
Assign (=): Assigns the value of the right operand to the left operand.
Add and assign (+=): Adds the value of the right operand to the left operand and assigns the result to the left operand.
Subtract and assign (-=): Subtracts the value of the right operand from the left operand and assigns the result to the left operand.
Multiply and assign (*=): Multiplies the left operand by the right operand and assigns the result to the left operand.
Divide and assign (/=): Divides the left operand by the right operand and assigns the result to the left operand.
Modulo and assign (%=): Computes the modulus of the left operand with the right operand and assigns the result to the left operand.
Exponentiation and assign (**=): Raises the left operand to the power of the right operand and assigns the result to the left operand.
Floor divide and assign (//=): Divides the left operand by the right operand and assigns the integer quotient to the left operand.
Example:
x = 5 x += 3 print(x) # Output: 8 x -= 2 print(x) # Output: 6 x *= 4 print(x) # Output: 24 x /= 3 print(x) # Output: 8.0 x %= 5 print(x) # Output: 3.0 x **= 2 print(x) # Output: 9.0 x //= 2 print(x) # Output: 4.0
Logical Operators:
Logical AND (and): Returns True if both operands are True.
Logical OR (or): Returns True if at least one of the operands is True.
Logical NOT (not): Returns the opposite of the operand's logical value.
Example:
x = True y = False logical_and = x and y logical_or = x or y logical_not_x = not x print(logical_and) # Output: False print(logical_or) # Output: True print(logical_not_x) # Output: False
Bitwise Operators:
Bitwise AND (&): Performs a bitwise AND operation on the operands.
Bitwise OR (|): Performs a bitwise OR operation on the operands.
Bitwise XOR (^): Performs a bitwise XOR (exclusive OR) operation on the operands.
Bitwise NOT (~): Performs a bitwise NOT operation, which flips the bits of the operand.
Left Shift (<<): Shifts the bits of the left operand to the left by the number of positions specified by the right operand.
Right Shift (>>): Shifts the bits of the left operand to the right by the number of positions specified by the right operand.
Example:
x = 5 y = 3 bitwise_and = x & y bitwise_or = x | y bitwise_xor = x ^ y bitwise_not_x = ~x left_shift = x << 1 right_shift = x >> 1 print(bitwise_and) # Output: 1 print(bitwise_or) # Output: 7 print(bitwise_xor) # Output: 6 print(bitwise_not_x) # Output: -6 print(left_shift) # Output: 10 print(right_shift) # Output: 2
Membership Operators:
Membership Operators:
in
,not in
Example:
fruits = ['apple', 'banana', 'cherry'] print('banana' in fruits) # Output: True print('orange' not in fruits) # Output: True
Identity Operators:
Identity Operators:
is
,is not
Example:
x = 5 y = 5 print(x is y) # Output: True print(x is not y) # Output: False list1 = [1, 2, 3] list2 = [1, 2, 3] print(list1 is list2) # Output: False print(list1 is not list2) # Output: True
Unary Operators:
Unary Operators:
+
,-
Example:
x = 5 print(+x) # Output: 5 print(-x) # Output: -5 y = -3 print(+y) # Output: -3 print(-y) # Output: 3
Ternary Operator:
Ternary Operator:
expr1 if condition else expr2
Example:
x = 10 y = 5 result = 'x is greater' if x > y else 'y is greater' print(result) # Output: 'x is greater'
These examples demonstrate the usage of each operator in Python and their corresponding results.
Subscribe to my newsletter
Read articles from Aksh Darji directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
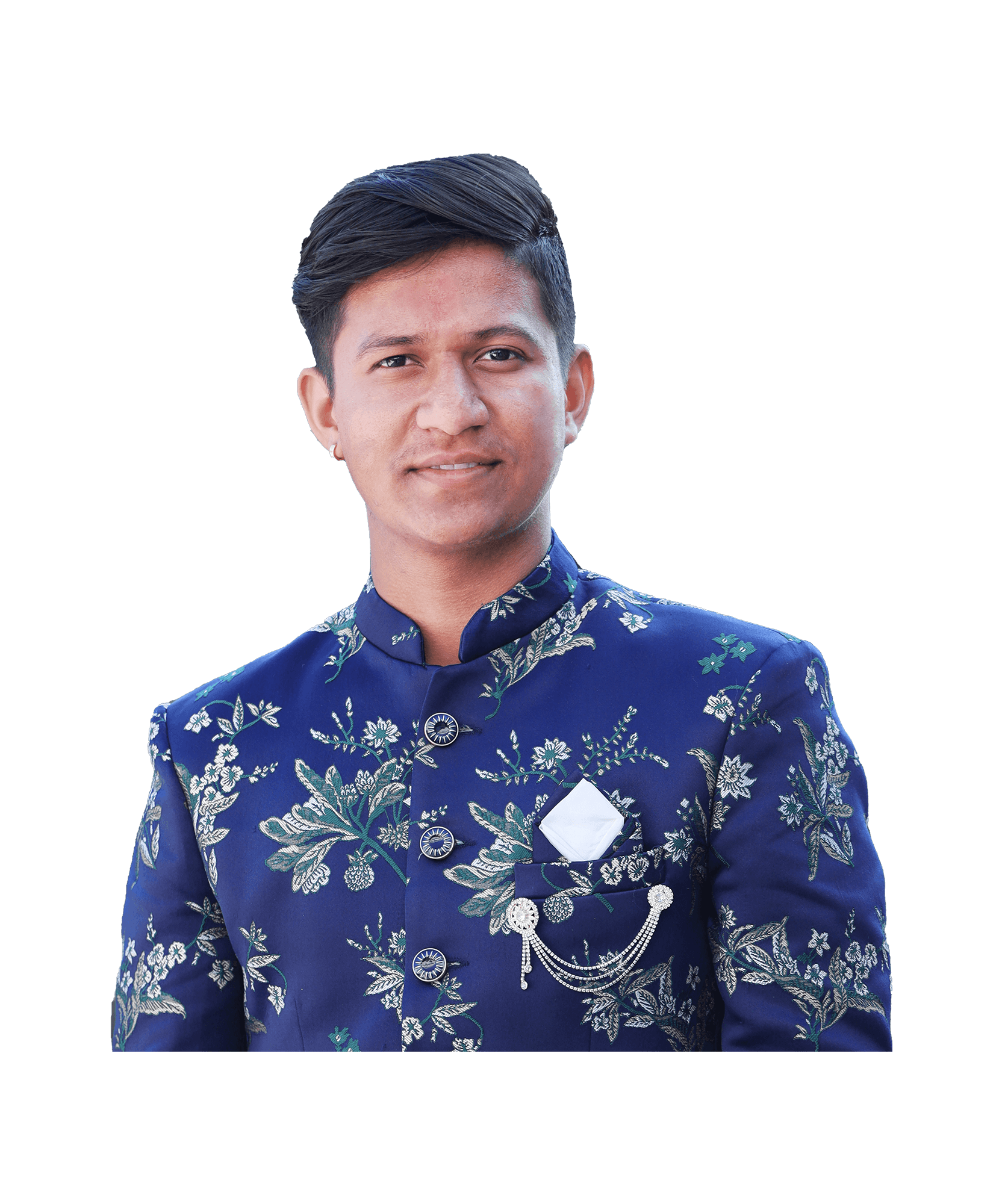