Python for Beginners: Part 7 - Exercises
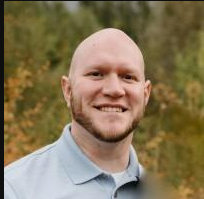
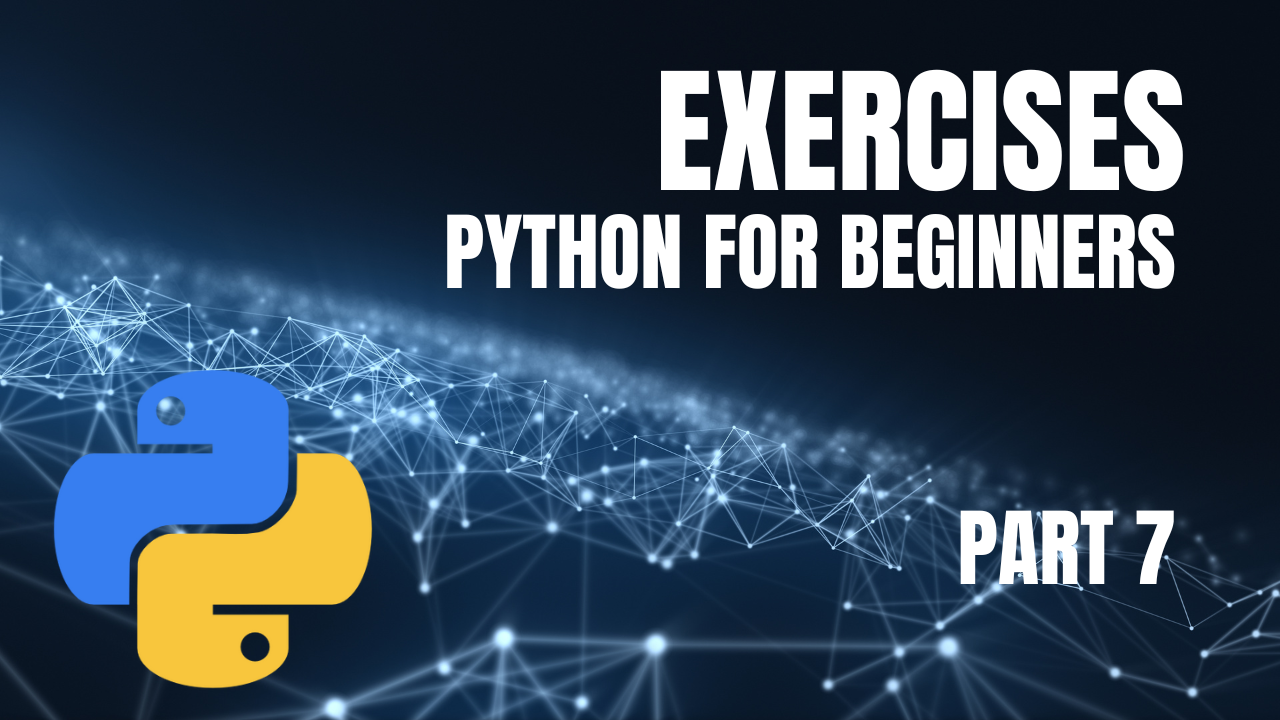
Exercise 1: Greeting Program
Write a program that asks the user for their name and then prints a personalized greeting. The program should display a message like "Hello, [name]!" where [name] is the name entered by the user.
Exercise 2: Even or Odd
Write a program that asks the user to enter a number and determines whether the number is even or odd. The program should display a message indicating whether the number is even or odd.
Exercise 3: Multiplication Table
Write a program that asks the user to enter a number and prints the multiplication table for that number. The program should display the multiplication table from 1 to 10 for the given number.
Exercise 4: Temperature Converter
Write a program that asks the user to enter a temperature in Celsius and converts it to Fahrenheit. The program should display the converted temperature in Fahrenheit.
Exercise 5: Guessing Game
Write a program that generates a random number between 1 and 10 and asks the user to guess the number. The program should provide feedback to the user, indicating whether their guess is too high or too low. The game should continue until the user guesses the correct number.
If you get stuck, here is the code for all 5 exercises.
Exercise 1: Greeting Program
name = input("Enter your name: ")
print("Hello, " + name + "!")
Exercise 2: Even or Odd
number = int(input("Enter a number: "))
if number % 2 == 0:
print("The number is even.")
else:
print("The number is odd.")
Exercise 3: Multiplication Table
number = int(input("Enter a number: "))
for i in range(1, 11):
print(number, "x", i, "=", number * i)
Exercise 4: Temperature Converter
celsius = float(input("Enter temperature in Celsius: "))
fahrenheit = (celsius * 9/5) + 32
print("Temperature in Fahrenheit:", fahrenheit)
Exercise 5: Guessing Game
import random
random_number = random.randint(1, 10)
guess = 0
while guess != random_number:
guess = int(input("Guess a number between 1 and 10: "))
if guess > random_number:
print("Too high!")
elif guess < random_number:
print("Too low!")
else:
print("Congratulations! You guessed the number!")
Feel free to use these code snippets and modify them as needed. Happy coding!
Subscribe to my newsletter
Read articles from Matthew Hard directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
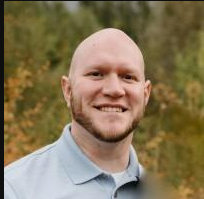
Matthew Hard
Matthew Hard
I'm Matthew, a cybersecurity enthusiast, programmer, and networking specialist. With a lifelong passion for technology, I have dedicated my career to the world of cybersecurity, constantly expanding my knowledge and honing my skills. From a young age, I found myself captivated by the intricate workings of computers and networks. This fascination led me to pursue in-depth studies in the fields of networking and cybersecurity, where I delved deep into the fundamental principles and best practices. Join me on this exciting journey as we explore the multifaceted world of technology together. Whether you're a beginner or a seasoned professional, I am here to share my knowledge, discuss the latest trends, and engage in insightful discussions. Together, let's embrace the ever-changing world of tech and navigate the complexities of cybersecurity with confidence and expertise.