Send your first SMS with Node.js & Twilioπ
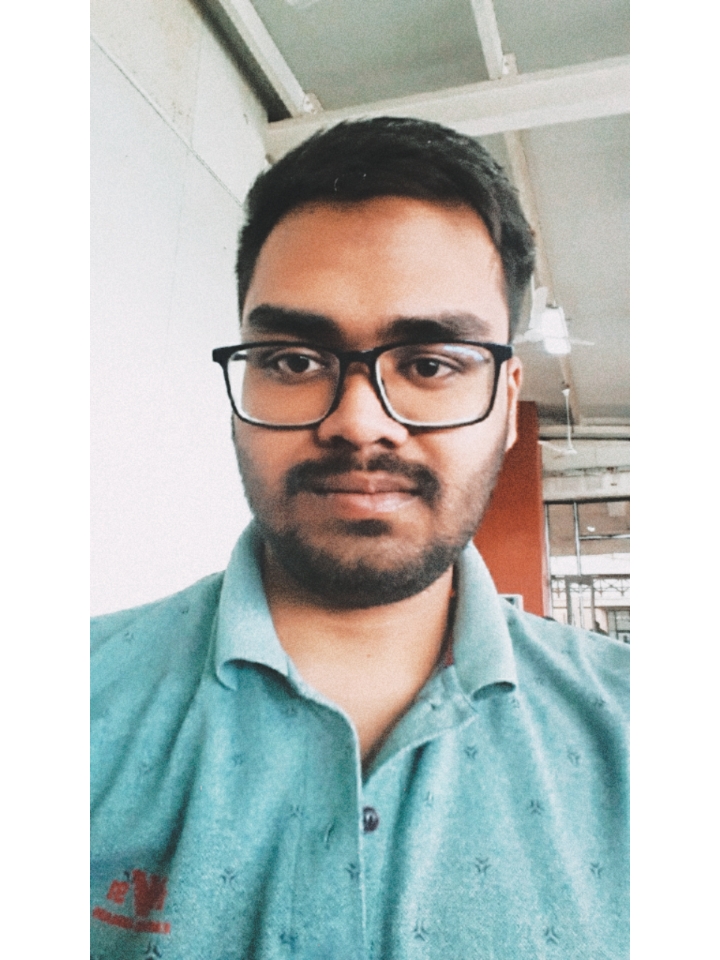
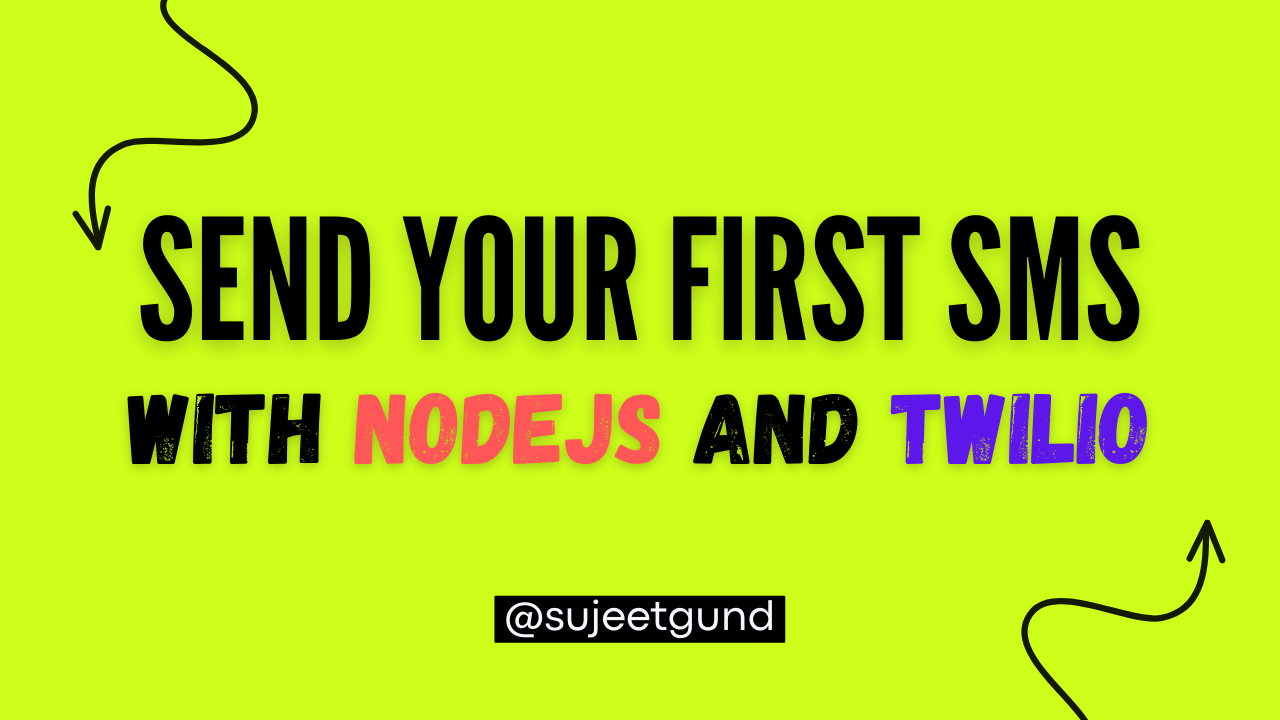
In today's tutorial, we will explore how to send your first SMS using Node.js and Twilio. SMS (Short Message Service) has become an integral part of modern applications that authenticate users' mobile number. By the end of this tutorial, you will have a good understanding of how to incorporate SMS functionality into your Node.js applications using Twilio's API.
To get started, make sure you have Node.js installed on your machine. If not, you can download it from the official Node.js website.
Next, you will need to sign up for a Twilio account if you don't have one already. Twilio offers a powerful API for sending and receiving SMS, as well as making and receiving phone calls. Upon signing up, you will receive your Twilio credentials, including an Account SID and an Auth Token, which you will use to authenticate your requests.
Once you have your Twilio account set up, you can begin by installing the Twilio Node.js module using npm:
npm install twilio
With the Twilio Node.js module installed, you can now proceed to write your first SMS-sending code. Below is a simple example of how to send an SMS using Twilio and Node.js:
const accountSid = 'your_account_sid';
const authToken = 'your_auth_token';
const client = require('twilio')(accountSid, authToken);
client.messages
.create({
body: 'This is your first SMS sent from Node.js & Twilio! π',
from: 'your_twilio_number',
to: 'recipient_number'
})
.then(message => console.log('SMS sent with SID:', message.sid));
Replace 'your_account_sid', 'your_auth_token', 'your_twilio_number', and 'recipient_number' with your actual Twilio credentials and phone numbers.
Run the above code in your Node.js environment, and if everything is set up correctly, you should see a message in the console confirming that your SMS has been sent. Here's the sample output in the console:
And there you have it! You've successfully sent your first SMS using Node.js and Twilio. This is just the beginning of what you can accomplish with Twilio's powerful API. Not only the integration of SMS functionality but also WhatsApp texts and phone calls for verification can be integrated, and with Twilio, it's easy to implement.
I hope you found this tutorial helpful in getting started with sending SMS using Node.js and Twilio. Stay tuned for more exciting tutorials on leveraging technology for building great applications. Happy coding!
Subscribe to my newsletter
Read articles from Sujeet Gund directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
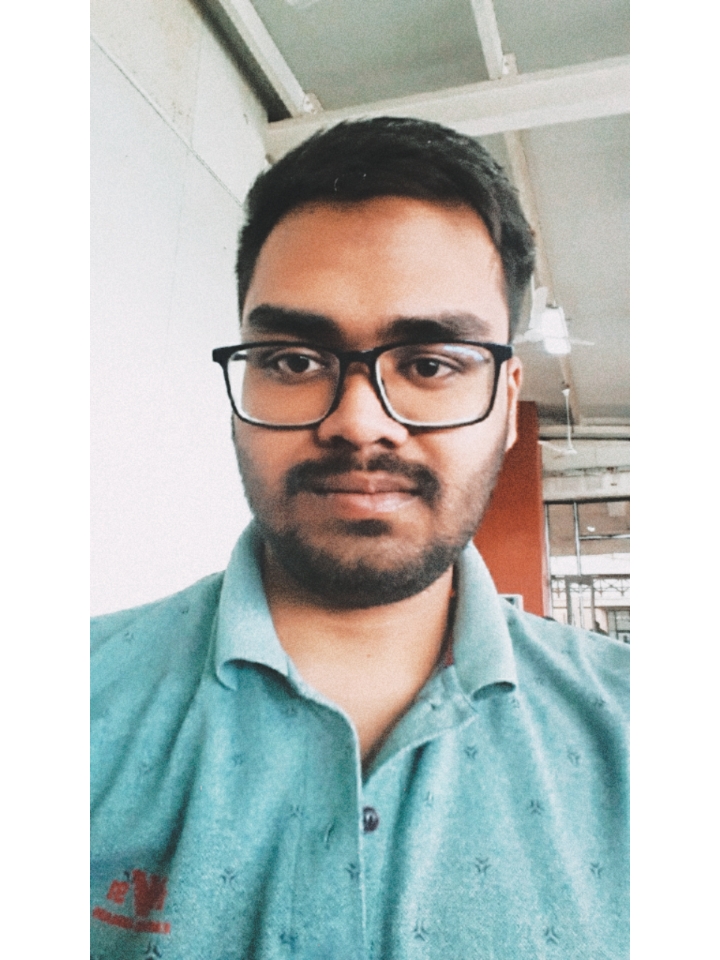
Sujeet Gund
Sujeet Gund
I am a Data Science student pursuing an Integrated MTech in AI at VIT Bhopal University, with over 3 years of coding experience in Python, JavaScript, and C++. Proficient in Git, Docker, AWS, Azure, and MongoDB, I develop robust solutions and am passionate about leveraging data for insights and innovation. Eager to connect and collaborate on exciting AI and data science projects.