Intermediate Python: Part 0 - Recursion
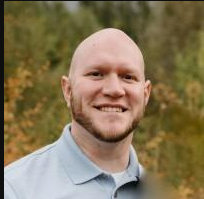
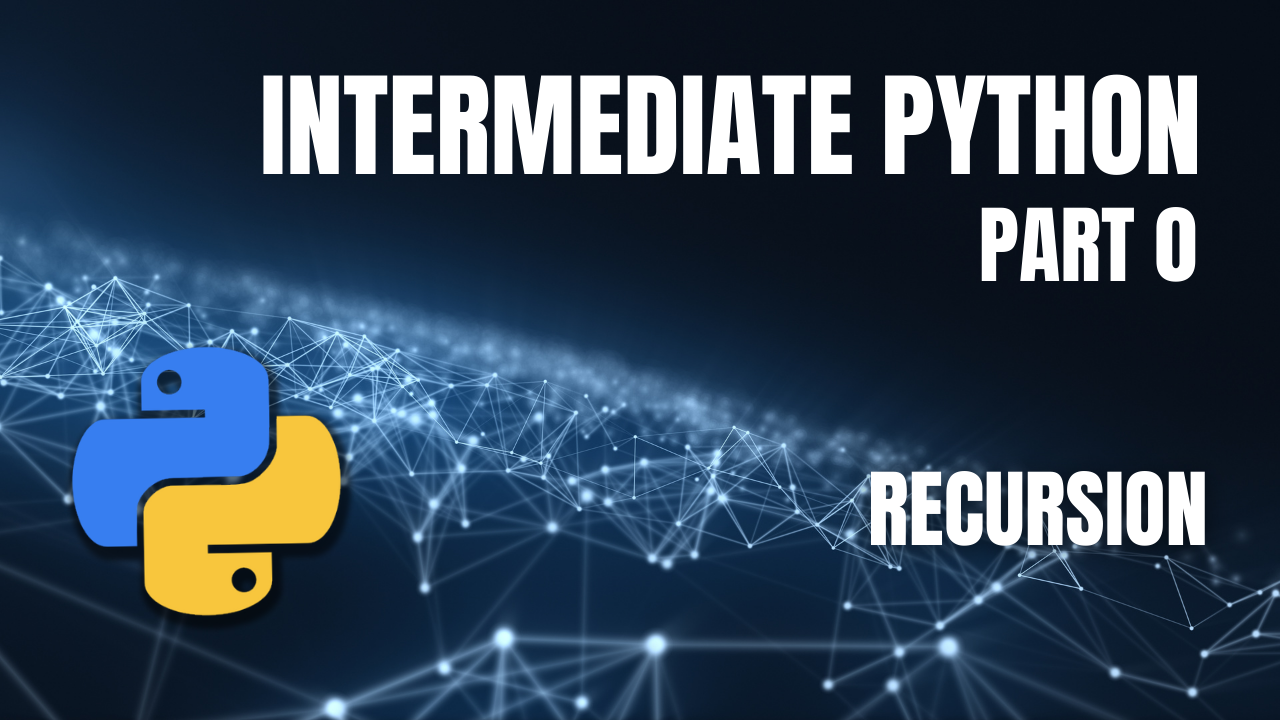
Welcome, aspiring Python programmers, to the prelude of our intermediate programming series! Today, we embark on a captivating exploration of the fascinating world of recursion. While it was not originally on our list, I believe it is a crucial concept to cover as we transition from basic code to embracing quality programming practices in the intermediate landscape.
Understanding Recursion: Descending into the Abyss
Recursion, often hailed as a powerful sorcery, allows a function to call itself from within its own definition. Just like a never-ending tunnel leading to the unknown, recursive functions descend deeper and deeper into their own execution, repeatedly solving smaller subproblems until a base case is reached.
The Power of Base Cases: Breaking the Spell
In the realm of recursion, base cases act as the ultimate spell breakers. They provide the necessary condition for a recursive function to stop its descent and return a result. Without a properly defined base case, the function would spiral into an infinite loop, consuming all available resources.
Recursive Cases: Navigating the Recursive Depths
Recursive functions are not complete without recursive cases. These are the magical incantations that call the function again, but with a modified input, allowing it to solve a smaller version of the original problem. The recursive cases ensure progress towards the base case, gradually unraveling the problem's complexity.
A Simple Example: The Power of Counting
Let's delve into a simple example to experience the captivating nature of recursion. Consider the task of counting down from a given number to zero. We can harness the power of recursion to elegantly solve this problem:
def countdown(n):
if n <= 0:
print("Blastoff!")
else:
print(n)
countdown(n - 1)
countdown(5)
In this spellbinding code, the countdown()
function takes an integer n
as its input. If n
is less than or equal to 0, it exclaims "Blastoff!" and terminates. Otherwise, it prints the current value of n
and invokes itself with n - 1
. The recursive calls continue until the base case is reached, enchanting us with a countdown from the initial value to zero.
The Spellbinding Potential: Unlocking Complex Problems
Recursion possesses immense power, capable of unraveling even the most complex problems. Its ability to break down a task into smaller, more manageable subproblems paves the way for elegant solutions. By harnessing the recursive magic, you can conquer challenges like traversing intricate data structures, solving complex algorithms, and exploring the realm of fractals.
However, it's essential to approach recursion with caution. The absence of a proper base case or the misuse of recursive calls can lead to infinite loops and stack overflows. Understanding the flow of recursive functions and ensuring progress towards the base case is crucial for success.
TIME OUT
But wait... I was told recursion was bad. Well, using recursion is not inherently bad. However, it does require careful consideration and understanding of its implications. Recursion can actually be a powerful tool for solving certain problems, particularly those that exhibit a natural recursive structure. Nonetheless, it's important to be aware that improper use of recursion can result in performance issues and potential stack overflows.
Here are some points to consider regarding recursion:
Clarity and Readability: Recursion can sometimes lead to more concise and elegant code, making it easier to understand and maintain. It can capture the essence of a problem by breaking it down into smaller subproblems.
Efficiency: Recursive solutions may not always be the most efficient, especially for problems with large input sizes. Recursive function calls involve additional overhead in terms of memory allocation and stack management. Iterative solutions are often more efficient in such cases.
Stack Limitations: Recursion relies on the call stack to keep track of function calls. If a recursive function goes too deep without reaching a base case, it can exceed the stack's capacity, resulting in a stack overflow error. Iterative approaches or tail recursion optimization can be used to mitigate this issue.
Base Cases and Termination: Defining proper base cases is essential to ensure that the recursion terminates. Without well-defined base cases, a recursive function can run indefinitely, leading to unexpected results or program crashes.
Space Complexity: Recursive solutions may require additional memory to store function call frames on the stack. For problems with deep recursion or large input sizes, this can lead to high memory usage. Iterative solutions often have better space efficiency.
Alternative Approaches: In some cases, iterative or dynamic programming techniques may offer more efficient and straightforward solutions compared to recursion. It's important to consider different approaches and choose the one that best suits the problem at hand.
Recursion can be a powerful and elegant approach for solving certain problems. However, it is important to assess its suitability based on factors such as performance, clarity, and potential limitations. By understanding the strengths and weaknesses of recursion, you can make informed decisions about when and how to use it effectively.
TIME IN
As you embark on this Python journey with recursion, remember to define clear base cases, navigate the recursive depths with caution, and marvel at the spellbinding capabilities of recursion.
In the upcoming chapters of our intermediate programming series, we shall explore further realms of Python's enchanting abilities. Brace yourselves for exciting concepts, empowering your journey towards becoming true Python sorcerers!
May your recursive spells be well-defined, your base cases be reachable, and your coding adventures be filled with magic!
Subscribe to my newsletter
Read articles from Matthew Hard directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
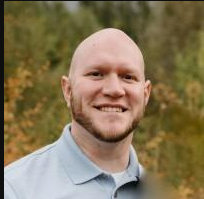
Matthew Hard
Matthew Hard
I'm Matthew, a cybersecurity enthusiast, programmer, and networking specialist. With a lifelong passion for technology, I have dedicated my career to the world of cybersecurity, constantly expanding my knowledge and honing my skills. From a young age, I found myself captivated by the intricate workings of computers and networks. This fascination led me to pursue in-depth studies in the fields of networking and cybersecurity, where I delved deep into the fundamental principles and best practices. Join me on this exciting journey as we explore the multifaceted world of technology together. Whether you're a beginner or a seasoned professional, I am here to share my knowledge, discuss the latest trends, and engage in insightful discussions. Together, let's embrace the ever-changing world of tech and navigate the complexities of cybersecurity with confidence and expertise.