Loops in Python.
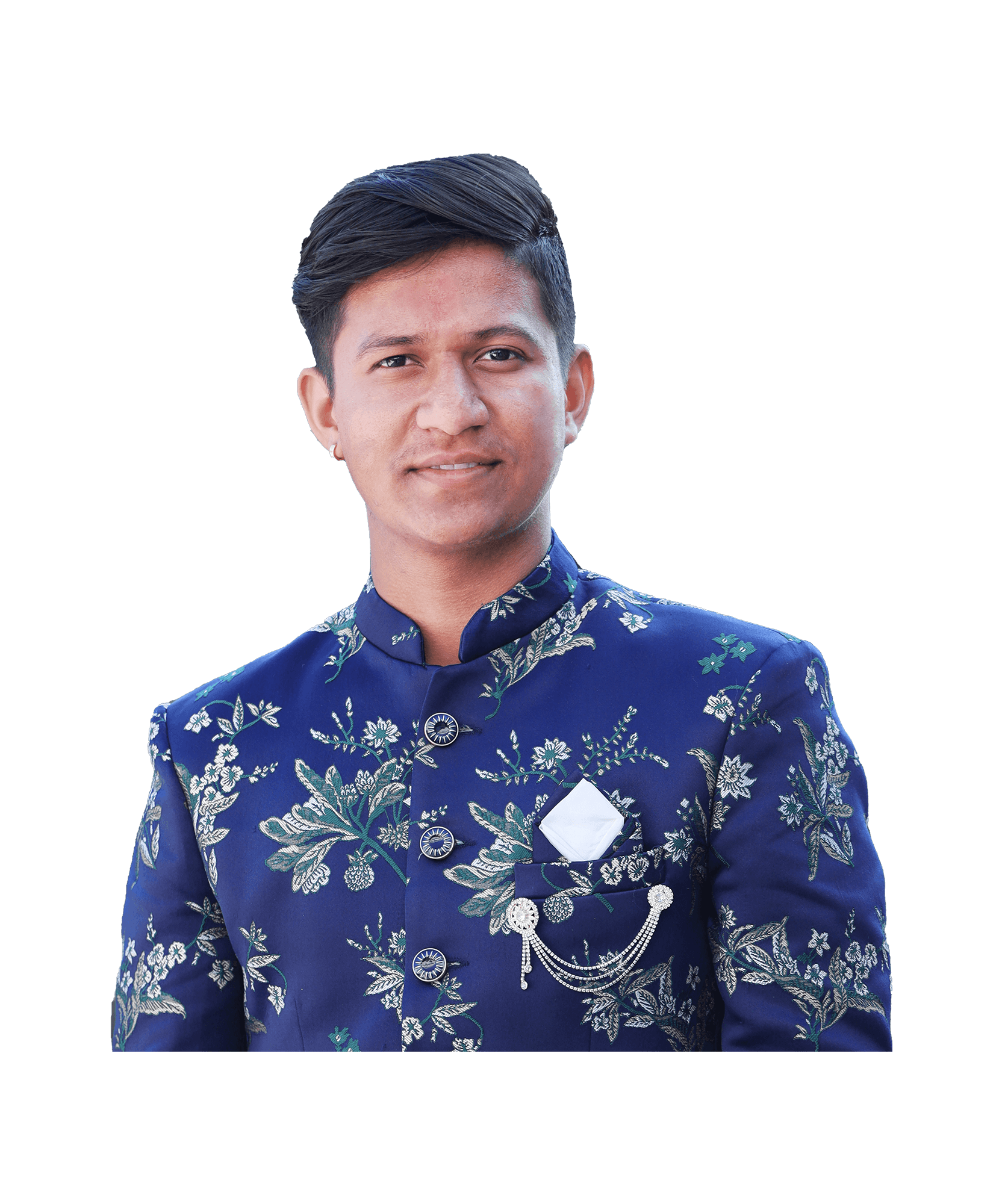
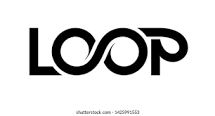
What are the Loops in Python?
In Python, a loop is a programming construct that allows you to repeat a block of code multiple times. It enables you to automate repetitive tasks and iterate over collections of data. Python provides two main types of loops: the for
loop and the while
loop.
For Loop.
A for
loop is a control flow statement in Python that allows you to iterate over a sequence of elements, such as a list, tuple, string, or range. It enables you to execute a block of code for each item in the sequence.
Here's the general syntax of a for
loop in Python:
for item in sequence:
# code block to be executed
Let's break down the components of the for
loop:
item
: This is a loop variable that takes the value of each item in the sequence, one at a time, during each iteration of the loop. You can choose any valid variable name to represent the current item.sequence
: It represents the collection of elements over which you want to iterate. It can be any iterable object like a list, tuple, string, or even a range of numbers.code block to be executed
: This is the indented block of code that will be executed for each item in the sequence. You can perform any desired operations or calculations using the loop variableitem
within this block.
During each iteration, the loop variable item
will take the value of the current element in the sequence. The loop will continue iterating until all items in the sequence have been processed.
Here's an example that demonstrates a for
loop in action:
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
Output:
Copy codeapple
banana
cherry
In this example, the for
the loop iterates over the list fruits
, and the loop variable fruit
takes the value of each item in the list. The print(fruit)
the statement within the loop block prints each fruit name on a separate line.
While Loop.
A while
loop is a control flow statement in Python that allows you to repeatedly execute a block of code as long as a specified condition is true. It is useful when you want to repeat a task until a particular condition is met.
The basic syntax of a while
loop in Python is as follows:
while condition:
# code block to be executed
Here's how the while
loop works:
The
condition
is a Boolean expression that is evaluated before each iteration of the loop. If the condition is true, the code block inside the loop is executed. If the condition is false, the loop is exited, and program execution continues with the next statement after the loop.The code block inside the loop is indented and contains the statements that you want to repeat. You can perform any desired operations or calculations within this block.
After executing the code block, the program goes back to the beginning of the loop and re-evaluates the condition. If the condition is still true, the code block is executed again. This process continues until the condition becomes false.
It is important to ensure that the condition eventually becomes false; otherwise, the loop may run indefinitely, resulting in an infinite loop. To avoid infinite loops, make sure that the condition is updated within the loop so that it can eventually become false.
Here's an example that demonstrates a while
loop in action:
count = 0
while count < 5:
print(count)
count += 1
Output:
Copy code0
1
2
3
4
In this example, the while
loop continues executing the code block as long as the count
variable is less than 5. The loop block prints the value of count
and increments it by 1 in each iteration. Once the count
reaches 5, the condition becomes false, and the loop is exited.
DO While Loop.
In Python, there is no built-in "do-while" loop construct like in some other programming languages. However, you can achieve a similar behavior using a combination of a while
loop and a control variable.
A "do-while" loop is a loop that executes a block of code at least once and then continues to repeat the loop as long as a specified condition is true. In contrast to a regular while
loop, the "do-while" loop guarantees that the code block will be executed at least once before checking the condition.
Here's an example of how you can emulate a "do-while" loop in Python:
condition = True
while condition:
# code block to be executed
# ...
# update the condition
# set condition to False to exit the loop or modify it based on certain conditions
condition = False # or condition = some_condition()
In this approach:
The loop is initially set to execute because the condition variable is set to
True
.The code block inside the loop is executed.
After executing the code block, you have the opportunity to update the condition variable. If the condition variable is set to false, the loop will exit. Otherwise, the loop will continue and execute the code block again.
By setting the condition variable appropriately within the loop, you can control the behavior of the loop and emulate a "do-while" loop.
Keep in mind that it's essential to ensure that the condition eventually becomes false to prevent an infinite loop.
Conclusion.
In conclusion, loops are an essential part of Python programming, allowing you to automate repetitive tasks and iterate over collections of data. The for
loop is ideal for iterating over sequences, executing a block of code for each item. On the other hand, the while
loop is useful when you want to repeat a task until a specific condition is met. By understanding how to use loops effectively, you can enhance the efficiency and flexibility of your Python programs.
Subscribe to my newsletter
Read articles from Aksh Darji directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
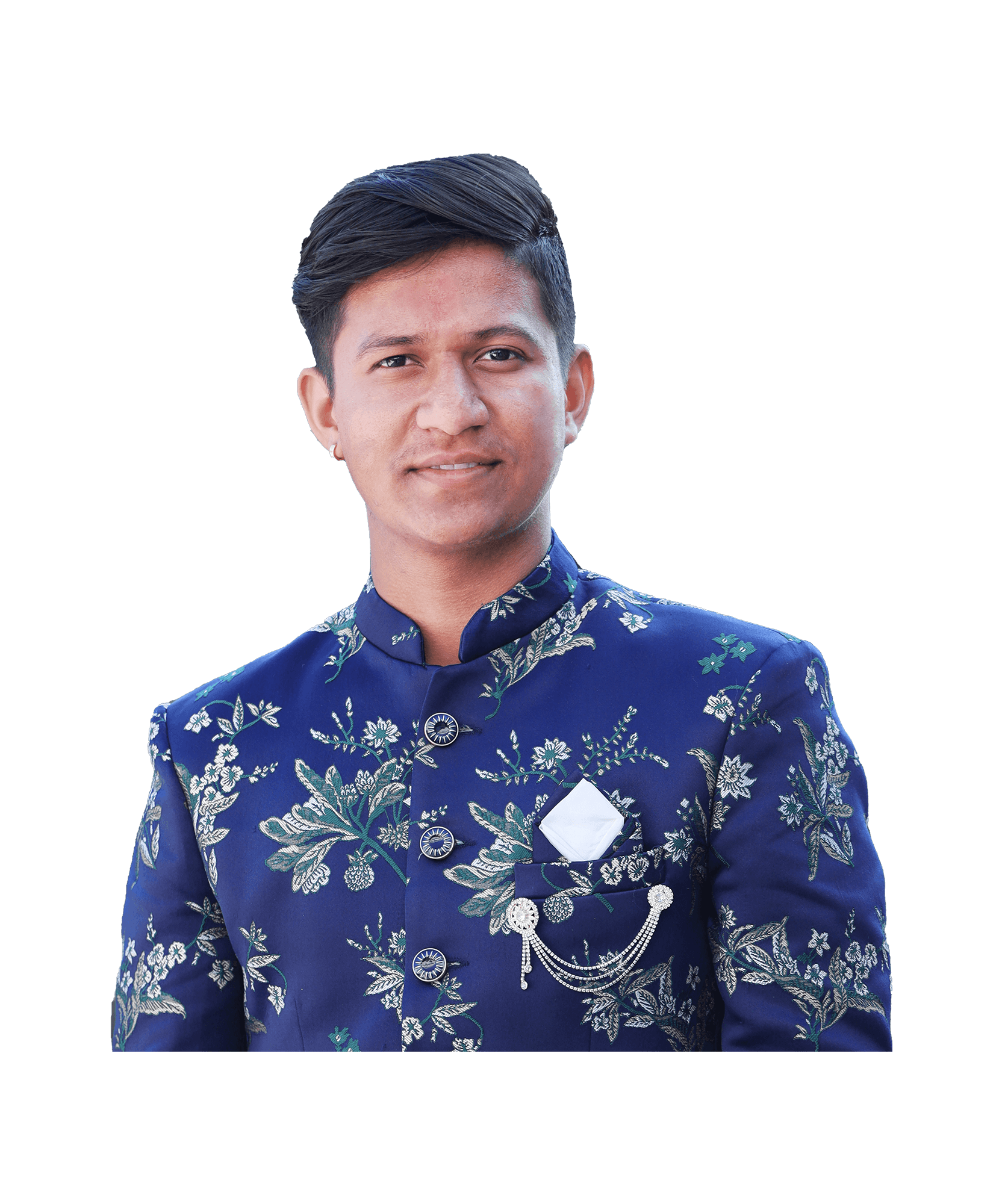