Understanding Variables in JavaScript: let, const, and var

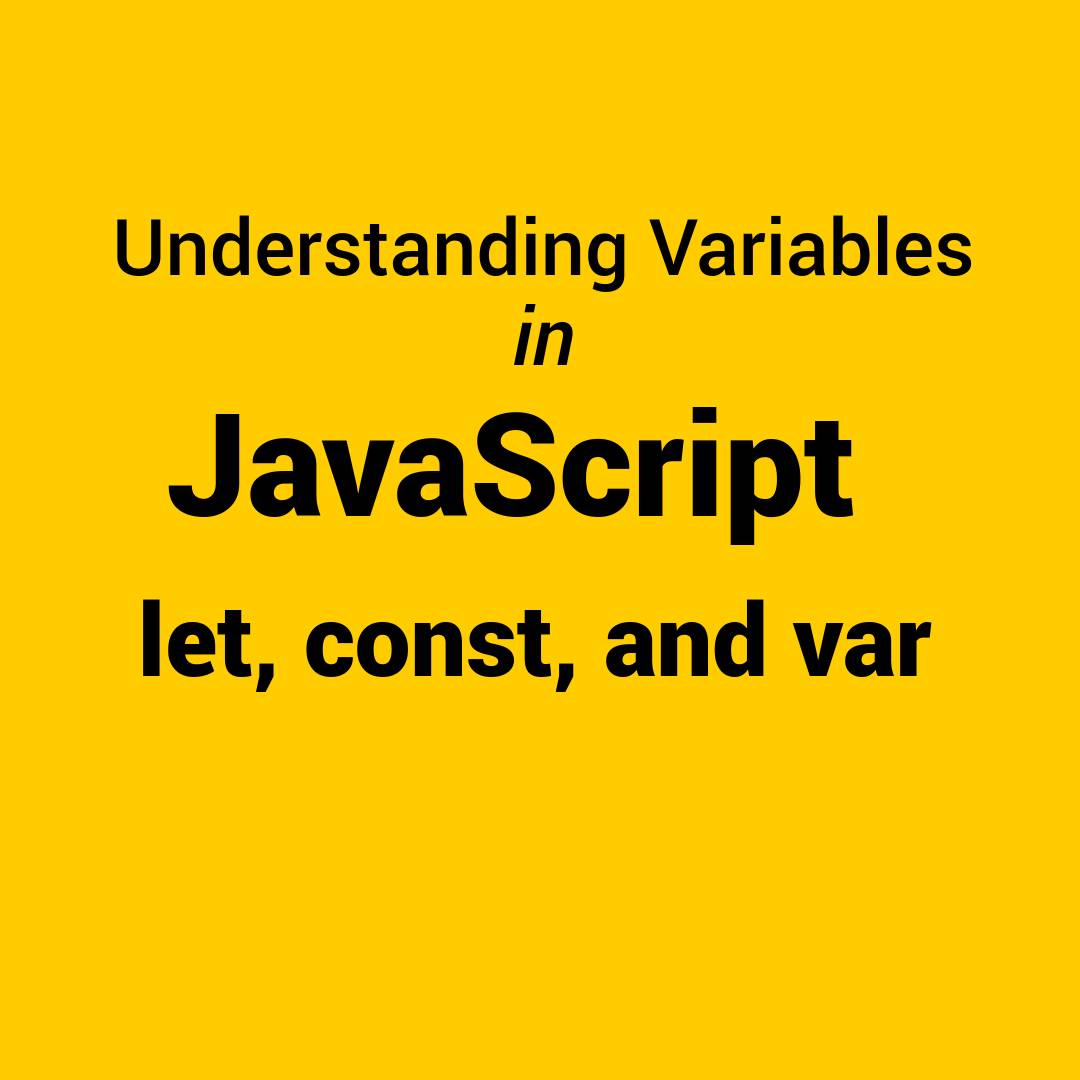
In JavaScript, variables are fundamental elements used for storing and manipulating data. With the introduction of ECMAScript 6 (ES6), new variable declaration keywords were introduced, namely let
and const
, alongside the existing var
keyword. In this blog post, we will explore the differences between let
, const
, and var
, and understand their appropriate use cases with code examples.
The var
Keyword:
The var
keyword was the primary variable declaration keyword in JavaScript before ES6. It has function scope or global scope, allowing the variable to be accessed from anywhere within the function or globally.
function exampleFunction() {
var x = 10;
if (true) {
var x = 20;
console.log(x); // Output: 20
}
console.log(x); // Output: 20
}
In the above example, the variable x
is redeclared and reassigned within the if statement, affecting its value outside the block scope as well.
The let
Keyword:
The let
keyword was introduced in ES6 to address the shortcomings of var
by providing block scope.
function exampleFunction() {
let x = 10;
if (true) {
let x = 20;
console.log(x); // Output: 20
}
console.log(x); // Output: 10
}
In this example, the variable x
is declared using let
inside the if statement. As a result, the value of x
inside the if block remains separate from the value outside the block.
The const
Keyword:
The const
keyword is also introduced in ES6 and is used for declaring constants, which are variables that cannot be reassigned.
function exampleFunction() {
const PI = 3.14159;
console.log(PI); // Output: 3.14159
PI = 3; // Error: Assignment to constant variable
}
In the above example, the constant PI
is assigned a value and cannot be changed afterward. If an attempt is made to reassign it, an error will occur.
Conclusion:
Understanding the differences between let
, const
, and var
is crucial for writing clean and maintainable JavaScript code. While var
is still valid, it's generally recommended to use let
and const
to benefit from block scoping and immutability. Use let
when you need a mutable variable with block scope and const
for variables that should remain constant throughout the program.
Remember, let
, const
, and var
are just tools in your JavaScript toolbox. Choose the right tool for the right job!
Happy coding! ๐๐๐
Note: The code examples provided in this blog post are simplified for the sake of illustration and may not cover all edge cases. It's recommended to consult the official JavaScript documentation for a comprehensive understanding of variable declarations.
Subscribe to my newsletter
Read articles from Sakil Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sakil Khan
Sakil Khan
I'm a Software Engineer specialized in Nodejs/Reactjs ecosystem with professional experience. I always look forward to enhancing my knowledge and skills to give my best for real-world problems through technology. The primary interest is JavaScript stack but open enough for other languages if needed. I love to work with edge technologies & enjoy the challenges.