Guess the Number Game Using JavaScript

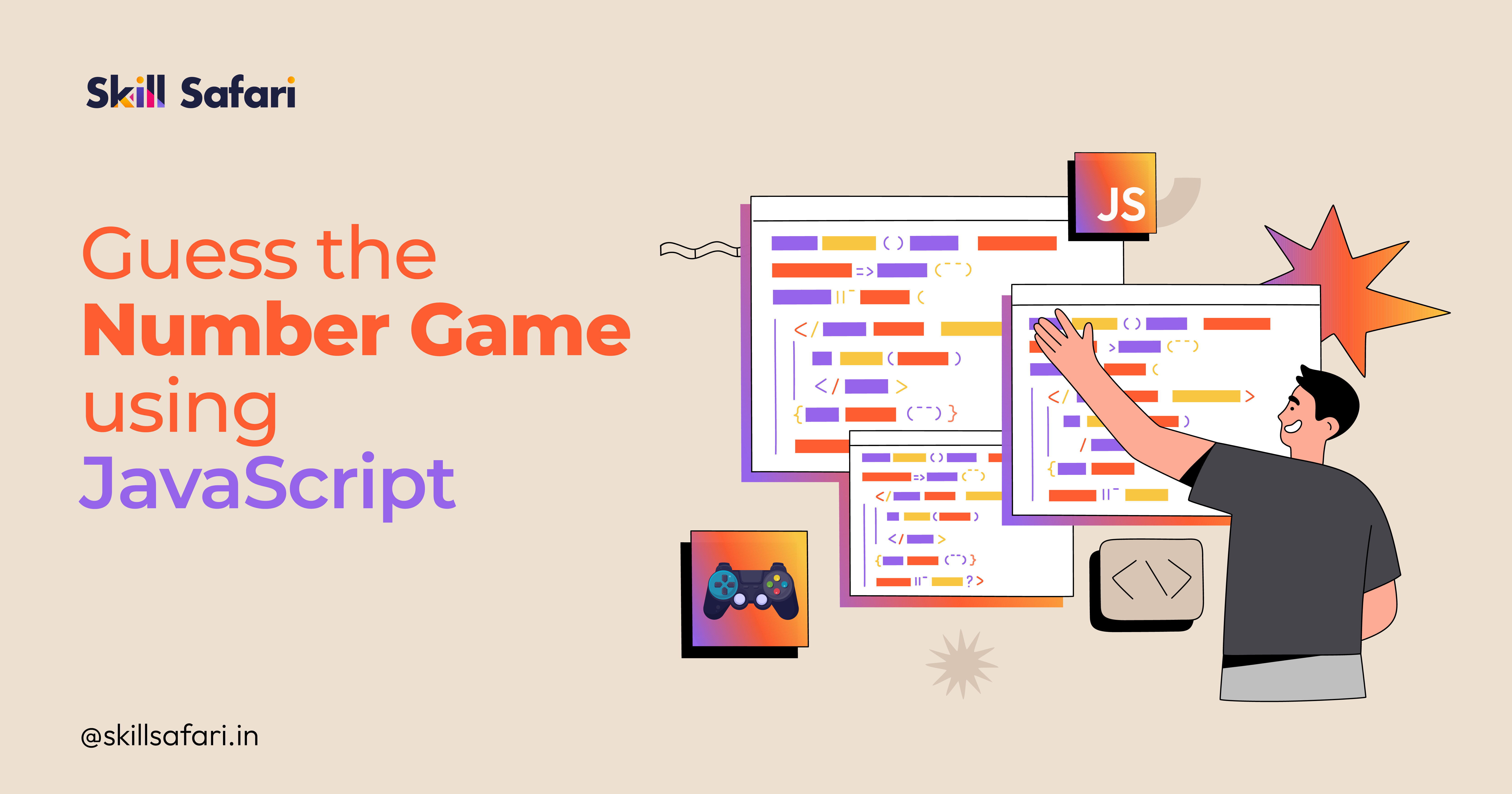
JavaScript is used in our day-to-day applications, from Facebook to Netflix. It is a functional programming language with lots of advantages. Any browser can run it, making it possible to develop dynamic, interactive websites. Because of its adaptability can be used for front-end and back-end development, improving the user experience. JavaScript effectively manages several tasks at once because it supports asynchronous programming. Its extensive ecosystem of libraries and frameworks makes products more accessible and encourages code reuse. The adaptability of JavaScript makes it possible to develop web applications that are mobile-friendly and responsive.
Additionally, it seamlessly integrates with HTML and CSS, making web page manipulation easier. JavaScript is vital for contemporary web development due to its wide adoption, comprehensive documentation, and active community support. In this article, we'll detail how to play Guess the Number Game with JavaScript.
Let’s start splitting the task into several points. We will learn the game by achieving the following points:
Prompt the player to enter their name. Use their name to print greetings.
The prompt() method in JavaScript displays a dialog box that prompts the user for input. It allows you to get user input interactively while running a script.Here in the Console log, we use a string console.log('Welcome to the Guess Game ')
Our first task is to get the name, so by getting the variable name inside the console log, we can be able to achieve it.
<html>
<head>
<title> Guess Game</title>
</head>
<body>
<script>
var name = prompt ("Enter Your Name")
console.log('Welcome to the Guess Game ' + name )
</script>
</body>
</html>
2.Generate a random number from 1 to 100 and store it as a target number for the players to guess.
The first step of this game is to find a random number using math.random( ) method, Math.random ( )is used to return a random number between 0 and 1
By applying a random method, we can get a random number, and the given task for us is to generate a random number from 1 to 100. We use a method called Math. round, 1 to implement it. Math. round(x) returns the value of x rounded to its nearest integer.
var targetNumber = Math.round(Math.random() * 100)
console.log(targetNumber)
3. Check whether the guess is high, low, or correct.
Start off with assigning the maximum guess count of the player, where we can assign the variable maxGuessCount as 3 and use a parseInt JavaScript function that converts a string into an integer data type.
var maxGuess =3, playerGuessCount = 0
var guess = parseInt (prompt("Guess my number"))
//console.log( typeof guess)
By using the if and else if functions, we can guess if the targetNumber is low, high, or correct.
var maxGuess = 3, playerGuessCount = 0
while (playerGuessCount < maxGuess){
var guess = parseInt (prompt("Guess my number"))
//console.log( typeof guess)
if (guess < targetNumber) {
console.log('Your guess is low')
}
else if (guess > targetNumber) {
console.log(' your guess is high')
} else if( guess == targetNumber) {
console.log(' Good Job ' + name + ' Your Guess Is Correct')
break
}
Full SourceCode:
<html>
<head>
<title> Guess Game</title>
</head>
<body>
<script>
var name = prompt(" Enter your name")
console.log(' Welcome to the guess game ' + name )
var targetNumber = Math.round(Math.random() * 100)
console.log(targetNumber)
var maxGuess = 3, playerGuessCount = 0
while (playerGuessCount < maxGuess){
var guess = parseInt (prompt("Guess my number"))
//console.log( typeof guess)
if (guess < targetNumber) {
console.log('Your guess is low')
}
else if (guess > targetNumber) {
console.log(' your guess is high')
} else if( guess == targetNumber) {
console.log(' Good Job ' + name + ' Your Guess Is Correct')
break
}
}
</script>
</body>
</html>
Conclusion :
Following the 3 step process mentioned above, we can guess the number using Javascript. Javascript has emerged as a mandatory skill for anyone who is looking to start a career in IT. At Skillsafari, we teach students how to build a web application from scratch and offer a 100% placement guarantee. If you are interested in learning more about the program click on the link below:
Subscribe to my newsletter
Read articles from Arun R directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
