Prototypes and Inheritance in JavaScript
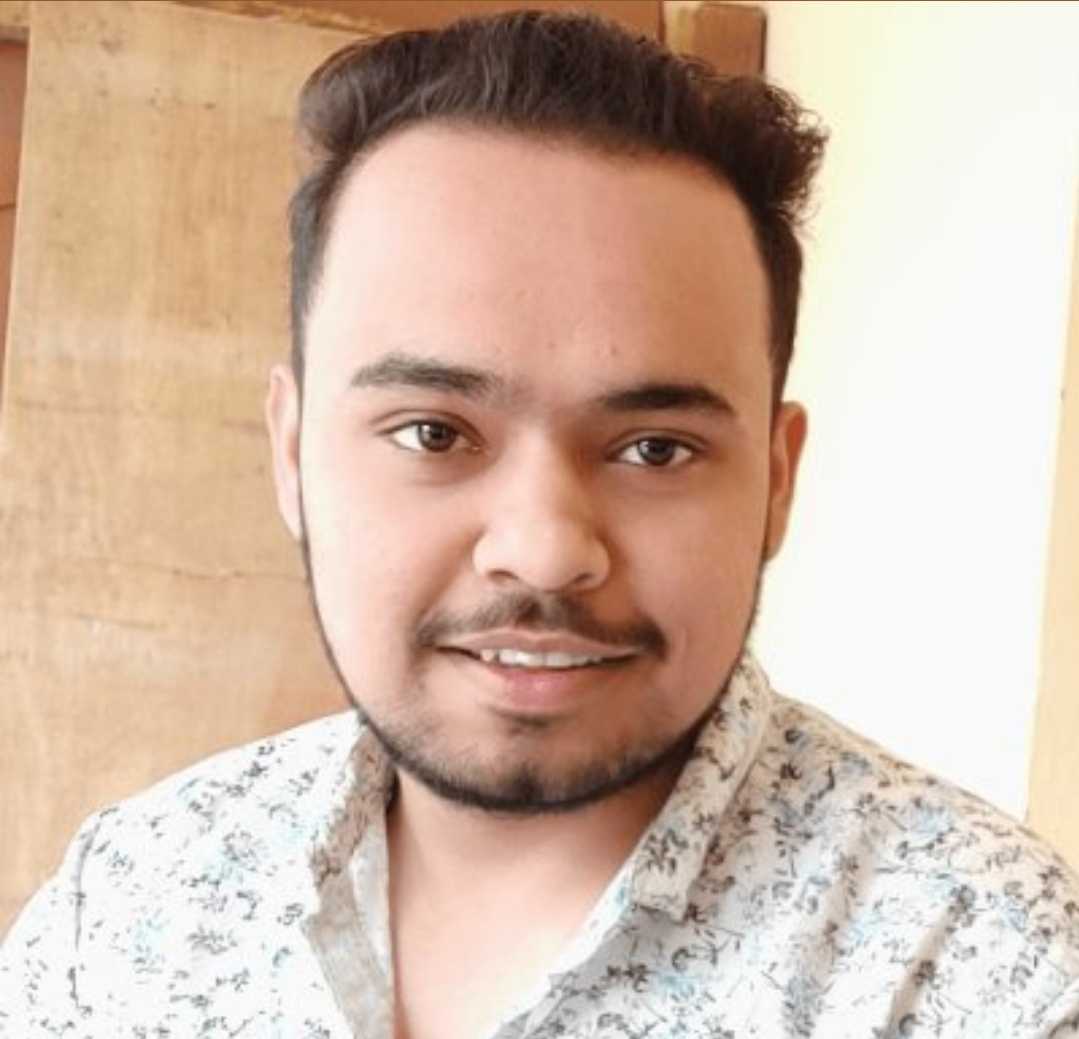
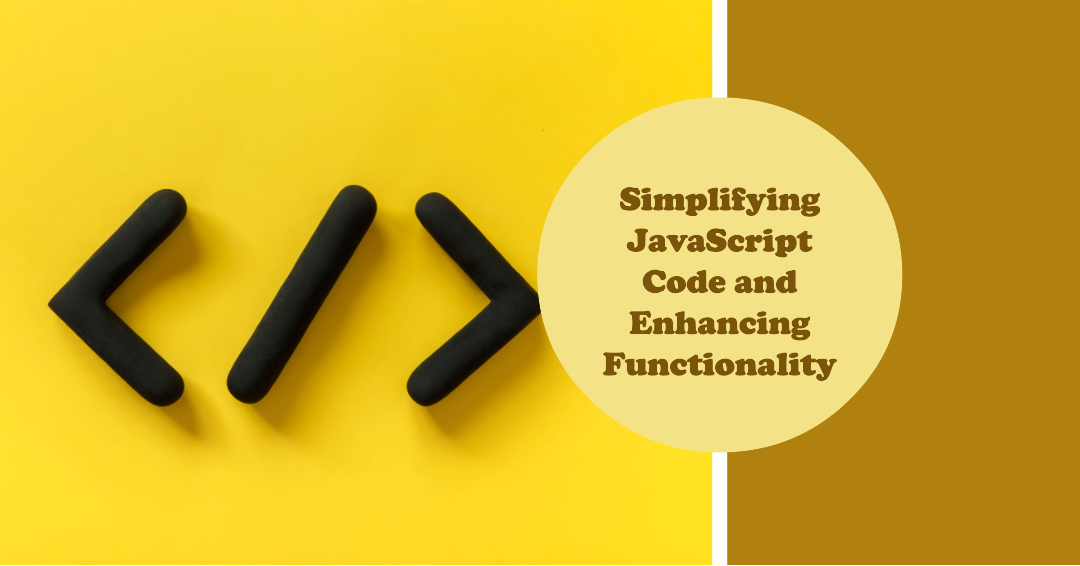
JavaScript is a versatile and powerful programming language widely used for web development. One of its fundamental concepts is prototypes and inheritance, which provide a mechanism for code reuse and object-oriented programming. In this blog post, we will explore prototypes, inheritance, and how they work together in JavaScript.
What are Prototypes?
In JavaScript, every object has a prototype, which serves as a blueprint for that object. It defines the object's properties and methods. When you access a property or method on an object, JavaScript first checks if it exists on the object itself. If not, it looks for it in the object's prototype. This chain of prototypes is called the prototype chain.
Creating Objects with Prototypes: JavaScript provides several ways to create objects with prototypes. One commonly used approach is through constructor functions. Let's consider an example:
function Person(name) {
this.name = name;
}
Person.prototype.greet = function() {
console.log(`Hello, my name is ${this.name}.`);
};
const ankit = new Person("Ankit");
ankit.greet(); // Output: Hello, my name is Ankit.
In the above code, we define a Person
constructor function and add a greet
method to its prototype. When we create a new instance of Person
using the new
keyword, the name
property is set on the instance. We can then call the greet
method on the ankit
object, and it prints the personalized greeting.
Inheritance in JavaScript:
Inheritance allows objects to inherit properties and methods from a prototype, enabling code reuse and hierarchical relationships between objects. In JavaScript, inheritance is achieved through prototype chaining.
Let's expand on our previous example and introduce a Teacher
constructor function that inherits from the Person
prototype:
function Teacher(name, subject) {
Person.call(this, name);
this.subject = subject;
}
Teacher.prototype = Object.create(Person.prototype);
Teacher.prototype.constructor = Teacher;
Teacher.prototype.teach = function() {
console.log(`I am teaching ${this.subject}.`);
};
const ankit = new Teacher("Ankit", "Math");
ankit.greet(); // Output: Hello, my name is Ankit.
ankit.teach(); // Output: I am teaching Math.
In the code above, we create a Teacher
constructor function that calls the Person
constructor using Person.call
(this, name)
. This allows the Teacher
instance to inherit the name
property from the Person
prototype. We then create a new object and set its prototype to Person.prototype
using Object.create(Person.prototype)
. Finally, we define a teach
method on the Teacher
prototype.
The ankit
object created from the Teacher
constructor can now access both the greet
method inherited from Person
and the teach
method defined in Teacher
.
Conclusion:
Understanding prototypes and inheritance is crucial for effective JavaScript development. Prototypes provide a way to define shared properties and methods for objects, while inheritance allows objects to inherit and extend functionality from prototypes. By utilizing these concepts, you can write more modular and reusable code.
In this blog post, we covered the basics of prototypes and inheritance in JavaScript. There is much more to explore, such as prototypal inheritance patterns and ES6 classes. By delving deeper into these concepts, you can enhance your JavaScript skills and build more robust applications.
Subscribe to my newsletter
Read articles from Ankit Bajpai directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
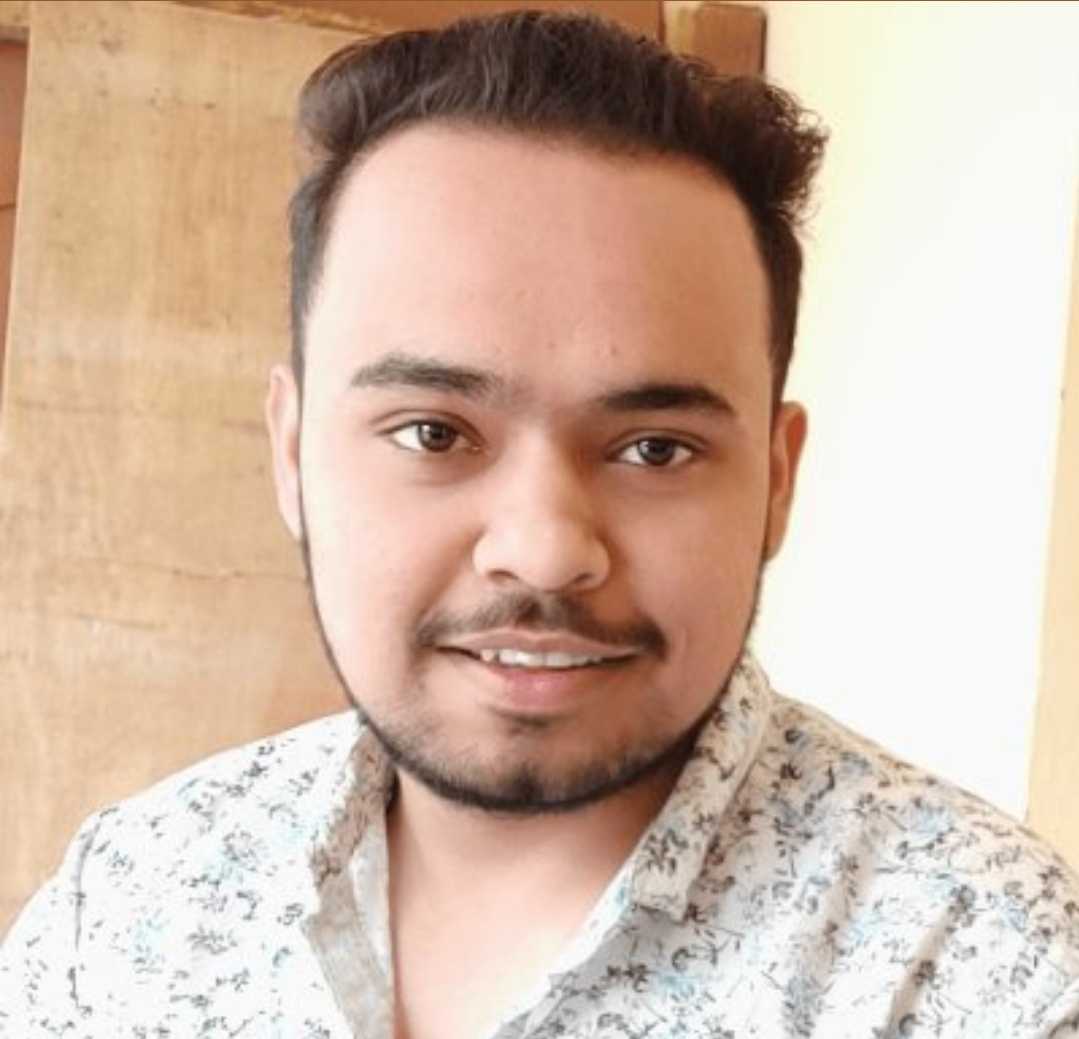
Ankit Bajpai
Ankit Bajpai
I am an innovative and observant full stack developer with expertise in JavaScript, React, Redux, Express, and Node. My passion lies in utilizing my analytical and engineering skills to create practical solutions and enhance my knowledge in the field. I thrive in a collaborative team environment and believe in leveraging my skills to contribute to the success of projects. With a strong background in full stack development, I bring a comprehensive understanding of frontend and backend technologies. I am proficient in JavaScript and have hands-on experience with popular frameworks and libraries such as React and Redux. On the backend, I am skilled in working with Express and Node.js to build robust and scalable applications. As a team player, I find great joy in working with people to align our efforts with the business objectives. Collaborative processes inspire me, as they pave the way for innovative ideas to flourish. I embrace the challenges that come with leadership roles, and I thrive when fostering an environment that encourages creativity and open communication. Beyond my technical expertise, I am truly passionate about my work and always eager to connect with like-minded professionals. I believe in continuous learning and strive to stay updated with the latest industry trends and advancements. This drive for growth motivates me to explore new opportunities and push the boundaries of my capabilities. If you are interested in connecting or discussing potential collaborations, I would be delighted to connect and explore how we can mutually benefit from our expertise and experiences.