Regularization Techniques
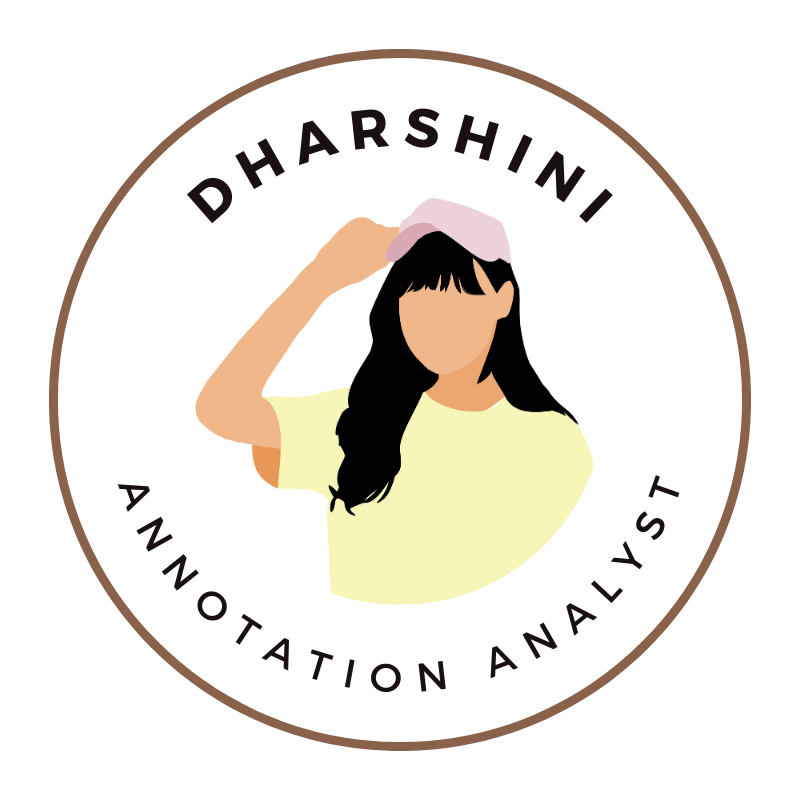
Overfitting is a common challenge in deep learning, where a neural network becomes excessively specialized in the training data and fails to generalize well to unseen data. To combat overfitting, various regularization techniques have been developed. In this article, we will explore some effective regularization techniques for deep learning neural networks and understand how they help in reducing overfitting.
L1 and L2 Regularization: L1 and L2 regularization, also known as weight decay, are commonly used techniques to control overfitting. These techniques add a regularization term to the loss function, penalizing the model for large weight values. L1 regularization encourages sparsity in the model by promoting some weights to become exactly zero, while L2 regularization encourages small weights without enforcing sparsity. By reducing the magnitude of the weights, L1 and L2 regularization help prevent the model from overemphasizing specific features or patterns in the training data.
Dropout: Dropout is a popular regularization technique that randomly sets a fraction of the neuron outputs to zero during training. This forces the network to learn redundant representations and prevents the reliance on specific neurons. By introducing randomness, dropout reduces the interdependence between neurons, making the network more robust and less prone to overfitting. During testing or inference, the dropout is typically turned off, and the full network is used for making predictions.
Batch Normalization: Batch normalization is a technique that normalizes the intermediate outputs of a network's layers. By normalizing the inputs to each layer, batch normalization reduces the internal covariate shift, which can help stabilize and speed up the training process. Additionally, batch normalization acts as a form of regularization by adding a small amount of noise to the inputs. This noise injection can prevent the network from relying too heavily on specific activations and improve its generalization capability.
Early Stopping: Early stopping is a simple yet effective regularization technique. It involves monitoring the model's performance on a validation set during training and stopping the training process when the model's performance starts deteriorating. By preventing the model from continuing to learn from the training data, early stopping helps avoid overfitting. The idea behind early stopping is to strike a balance between model complexity and generalization, stopping the training process at an optimal point.
Data Augmentation: Data augmentation is a technique that artificially increases the size of the training set by applying various transformations to the existing data. These transformations can include rotations, translations, scaling, and flipping of images, for example. Data augmentation introduces additional variations to the training data, making the model more robust and less likely to overfit on specific instances. It helps the model learn invariant features and improves its ability to generalize to unseen data.
from keras.models import Sequential
from keras.layers import Dense, Dropout
# Define the neural network architecture
model = Sequential()
model.add(Dense(64, activation='relu', input_dim=100))
model.add(Dropout(0.5))
model.add(Dense(64, activation='relu'))
model.add(Dropout(0.5))
model.add(Dense(10, activation='softmax'))
# Compile the model
model.compile(loss='categorical_crossentropy',
optimizer='adam',
metrics=['accuracy'])
# Train the model with dropout regularization
model.fit(x_train, y_train,
epochs=10,
batch_size=128,
validation_data=(x_val, y_val))
# Evaluate the model
score = model.evaluate(x_test, y_test, batch_size=128)
print("Test loss:", score[0])
print("Test accuracy:", score[1])
In this example, we create a neural network model with two hidden layers, each followed by a dropout layer. The dropout layer is specified using the Dropout
class in Keras. The parameter 0.5
represents the fraction of input units to drop during training, meaning 50% of the inputs will be randomly set to 0 at each update during training.
By applying dropout regularization, we introduce randomness to the network, which helps prevent overfitting by reducing the interdependence between neurons. The dropout layers act as a regularizer, forcing the model to learn more robust representations and improving its generalization ability.
During training, the model is fitted to the training data using the fit
function. We specify the number of epochs, batch size, and validation data. The model is then evaluated on the test data using the evaluate
function, providing us with the test loss and accuracy.
Subscribe to my newsletter
Read articles from Dharshini Sankar Raj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
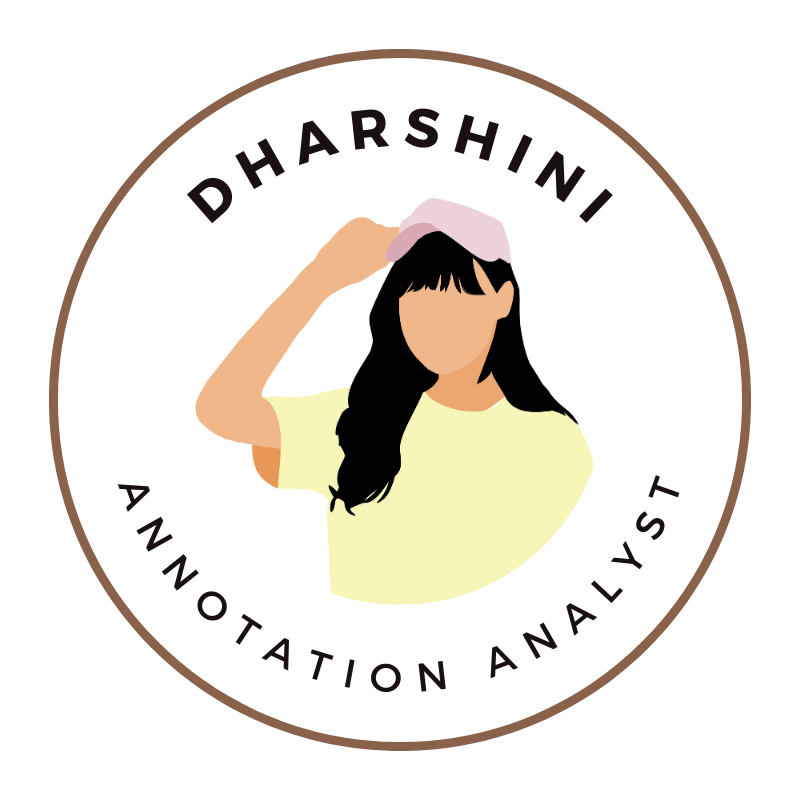
Dharshini Sankar Raj
Dharshini Sankar Raj
Driven by an intense desire to understand data and fueled by the opportunities presented during the COVID-19 pandemic, I enthusiastically ventured into the vast world of Python, Machine Learning, and Deep Learning. Through online courses and extensive self-learning, I immersed myself in these areas. This led me to pursue a Master's degree in Data Science. To enhance my skills, I actively engaged in data annotation while working at Biz-Tech Analytics during my college years. This experience deepened my understanding and solidified my commitment to this field.