useState Hook In react
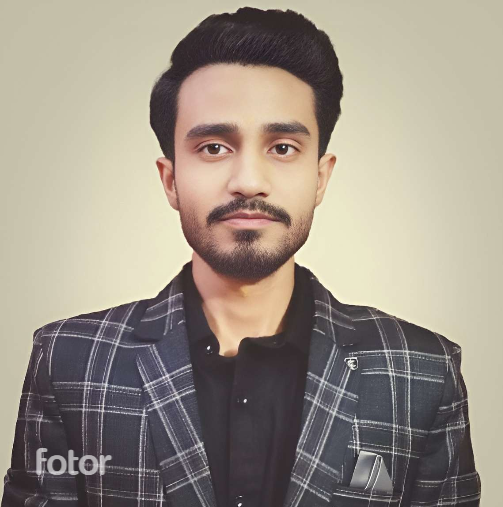
The useState
hook is a fundamental feature in React that allows you to add state to functional components. It provides a way to manage and update state without the need for class components. Here are the details of the useState
hook, along with two examples:
Syntax:
scssCopy codeconst [state, setState] = useState(initialState);
The
useState
function is called with an initial state value, which can be of any data type.It returns an array with two elements: the current state value (
state
) and a function (setState
) to update the state value.
Example 1 - Managing a Counter:
jsxCopy codeimport React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; const decrement = () => { setCount(count - 1); }; return ( <div> <h2>Counter: {count}</h2> <button onClick={increment}>Increment</button> <button onClick={decrement}>Decrement</button> </div> ); }
In this example, we initialize the state
count
with an initial value of 0 usinguseState(0)
.We define two functions,
increment
anddecrement
, which update thecount
state by callingsetCount
with the new value.The current state value is displayed in the JSX using
{count}
, and theincrement
anddecrement
functions are attached to button click events.
Example 2 - Handling Input:
jsxCopy codeimport React, { useState } from 'react'; function InputExample() { const [text, setText] = useState(''); const handleChange = (e) => { setText(e.target.value); }; return ( <div> <input type="text" value={text} onChange={handleChange} /> <p>You entered: {text}</p> </div> ); }
In this example, we initialize the state
text
with an empty string usinguseState('')
.We define the
handleChange
function, which is called whenever the input value changes.The
setText
function is used to update thetext
state with the new input value.The current state value
text
is displayed below the input field using{text}
.
In both examples, the useState
hook enables functional components to maintain and update their own state. By using useState
, you can easily manage stateful data within your React applications.
Hope I was able to clear all basics of useState hook, if you have further doubts please comment I will try to reply as soon as possible. Thanks for reading!
Subscribe to my newsletter
Read articles from Jayesh Chauhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
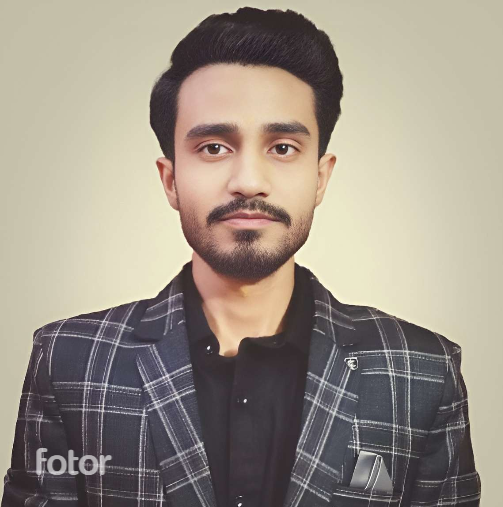
Jayesh Chauhan
Jayesh Chauhan
Hey I am Full Stack Developer.