How to give your program commands.
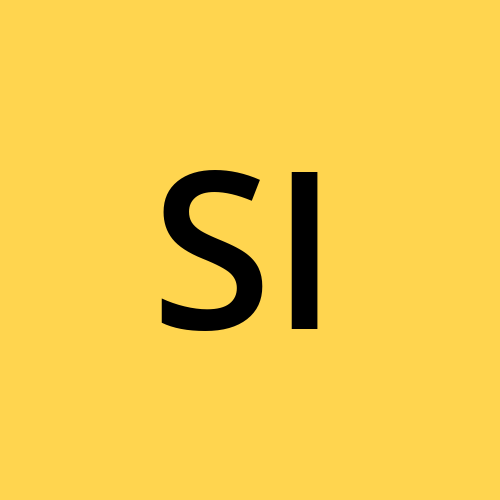
Commandline arguments in C.
While writing programs that give out values is great, user interaction is key to making your program usable. Imagine 'grep' without the ability to choose files to give it to ( see the manual pages for grep in GNU/Linux) to learn more). This would not be ideal to understate the matter. The ubiquitousness and usability of grep show how important it is for your program to enable the user to give commands to it. It is axiomatic that the commands given to the program will be strings. It is also important that the program be able to interpret this input into whatever data it needs.
The C programming language enables this feature by using the main prototype,
This prototype shows the command line taking two arguments, an integer and an array of character pointers. The integer argument, argc ( short for argument count), gives the number of arguments passed to the program. The array of character pointers will hold the commands the user passes as strings. Since C does not have a string data type, strings are given as arrays of characters which are usually passed by reference using character pointers. The argv (short for argument vectors) is an array of strings(character pointers) which means it could also be passed by reference as a pointer; hence the prototype is equivalent to:
Manipulating the commands.
Once the main function is written like that, the program can interpret the commands by manipulating the strings in argv. Argv can be treated as a 2D array to manipulate it. Of course, when passing arguments, each argument is taken as a string, and a string in quotes is treated as a single string. Since argc counts the number of arguments, it is used as the limiting number in the program when manipulating the arrays. argc gives the size of the array argv. The first value in the argv array is the program name, i.e., argv[0] = name of program, while argv[argc] is a NULL pointer. Of course, the count of arguments when manipulating the commands will still be those passed by the user ( See how to manipulate pointers and arrays for a deeper understanding of how it works.)
Examples.
Now this is a contrived example of a code that multiplies two numbers. It does not represent good coding practice (due to the hard-coded values when manipulation of argc values would achieve the same result. This code takes two arguments and multiplies them, returning the result. It ensures 2 values by checking the values of argc. The conversion using the atoi function converts the entered string to an integer.
While contrived, it shows that the value entered is taken to be a string and thus can be manipulated as such. Another example is a code that adds the numbers given. This example shows how to treat argv as a multidimensional array. It checks the entered arguments for if they are numbers before converting. This shows how to treat and manipulate the arguments as strings and how to check for each argument using array and pointer manipulation.
These examples show how to manipulate the given arguments in the code.
Unused variables
Sometimes when writing code, some of its variables may be unused but have to be there. An example is a code that counts the given arguments but still uses the argv array. An unused attribute should be added to the variable to compile such code. This is shown in the example below.
This will allow the code to compile without the unused variable warning, when you're ready you'll use the variable in the code. As an exercise on compiling with unused variables, look at how to use void to achieve the same.
Note
The examples are written in C, using the GNU/Emacs text editor.
Subscribe to my newsletter
Read articles from Samuel Ingosi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
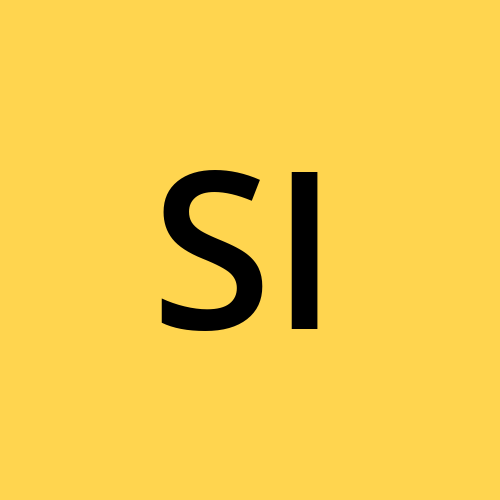
Samuel Ingosi
Samuel Ingosi
I am an aspiring software engineer coming to change the world.