Foundation CSS JavaScript Utilities
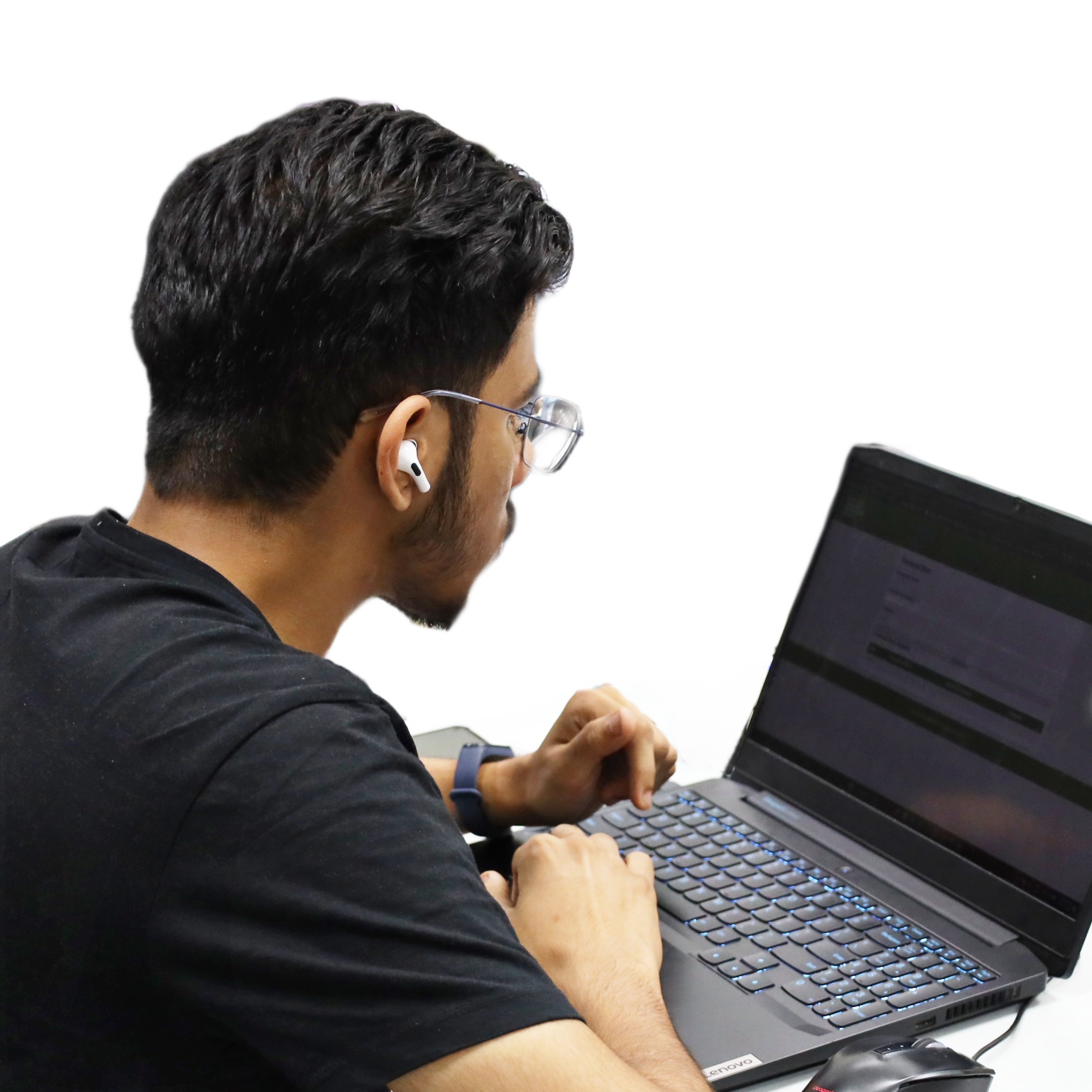
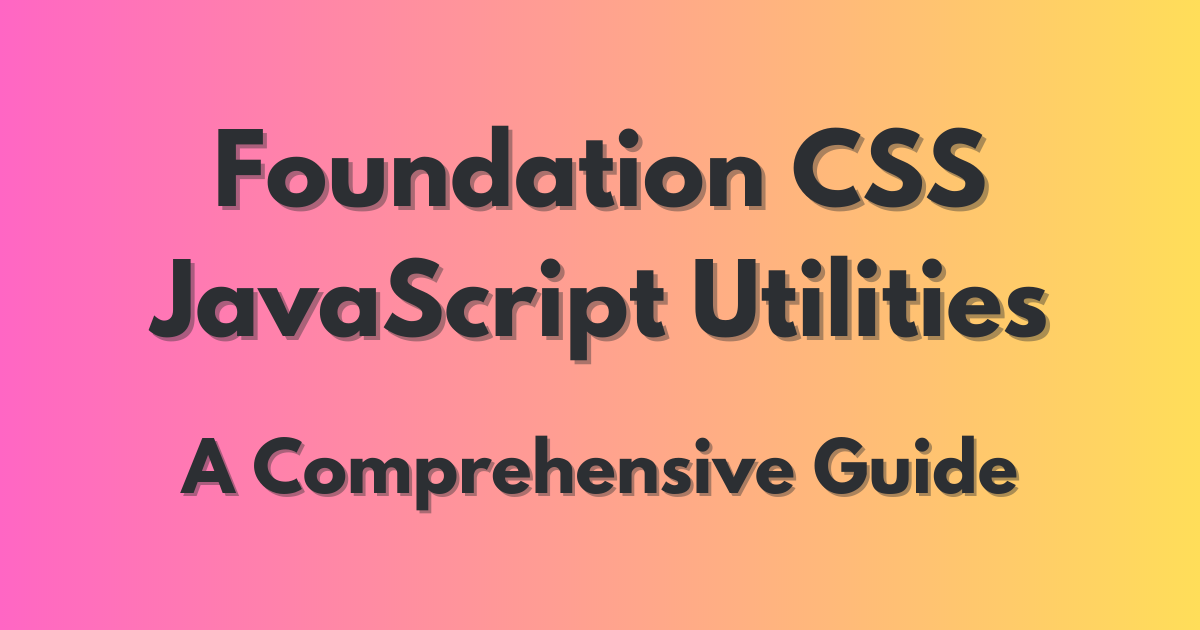
Foundation CSS is a responsive front-end framework that provides a robust toolkit for developers to create responsive designs with ease. It includes a set of JavaScript utilities that are used to add common functionalities, making it very helpful and easy to use. These utilities can be found in the folder Your_folder_name/node_modules/foundation-sites/js.
JavaScript Utilities in Foundation CSS
Box
The Foundation.Box
library consists of a couple of methods that are designed to make your life easier. You can pass either jQuery objects or plain JavaScript elements to both. The Foundation.Box
.GetDimensions(element)
method returns an object that contains the dimensions of the element.
var dims = Foundation.Box.GetDimensions(element);
The returned object specifies the dimension of the element as:
{
height: 54,
width: 521,
offset: {
left: 198,
top: 1047
},
parentDims: {
height: ... //The same format is share for parentDims and windowDims as the element dimensions.
},
windowDims: {
height: ...
}
}
The Foundation.Box
.ImNotTouchingYou
function returns a boolean based on whether the passed element is colliding with the edge of the window (default), or a parent element. The remaining two options are booleans for limiting collision detection to only one axis.
var clear = Foundation.Box.ImNotTouchingYou(element [, parent, leftAndRightOnly, topAndBottomOnly]);
Keyboard
The Foundation.Keyboard
library has several methods to make keyboard event interaction easier for all. The Foundation.Keyboard.keys
object contains key/value pairs of the most frequently used keys in the framework. The Foundation.Keyboard.parseKey(event)
function is called to get a string of what key was pressed, e.g. 'TAB' or 'ALT_X'.
var focusable = Foundation.Keyboard.findFocusable($('#content'));
The Foundation.Keyboard.handleKey
function is a main function of this library. This method is used to handle the keyboard event; it can be called whenever any plugin is registered with the utility.
Foundation.Keyboard.register('pluginName', {
'TAB': 'next'
});
//in event callback
Foundation.Keyboard.handleKey(event, 'pluginName', {
next: function(){
//do stuff
}
});
MediaQuery
The Foundation.MediaQuery
library is the backbone of all responsive CSS techniques. It has two publicly accessible functions and two properties:
Foundation.MediaQuery.get('medium'); // returns the minimum pixel value for the `medium` breakpoint.
Foundation.MediaQuery.atLeast('large'); // returns a boolean if the current screen size is, you guessed it, at least `large`.
Foundation.MediaQuery.queries; // an array of media queries Foundation uses for breakpoints.
Foundation.MediaQuery.current; // a string of the current breakpoint size.
Also included is an event emitter for breakpoint changes. You can hook into this event with:
$(window).on('changed.zf.mediaquery', function(event, newSize, oldSize){});
Motion & Move
The Foundation.Motion
JavaScript is similar to the Motion UI library, which is included in Foundation 6. It is used to create custom CSS transitions and animations. Foundation.Move
is used to make CSS3 backed animation simple and elegant.
Foundation.Move(durationInMS, $element, function() {
//animation logic
});
When the animation is completed, finished.zf.animate
is fired.
Timer & Images Loaded
The Foundation.Timer
function is similar to setInterval
, except youcan pause and resume where you left off. The Foundation.onImagesLoaded
method runs a callback function in your jQuery collection when images are completely loaded.
var timer = new Foundation.Timer($element, {duration: ms, infinite: bool}, callback);
Foundation.onImagesLoaded($images, callback);
Touch
The Foundation.Touch
methods are used for adding pseudo-drag events and swipe to elements. The addTouch
method is used to bind elements to touch events in the Slider plugin for mobile devices. The spotSwipe
method binds the elements to swipe events in the Orbit plugin for mobile devices.
$('selector').addTouch().on('mousemove', handleDrag);
$('selector').spotSwipe().on('swipeleft', handleLeftSwipe);
Triggers
The Foundation.Triggers
utility provides a number of event listeners and triggers your script can hook into. Most are self-explanatory, and used in many Foundation plugins.
$('selector').on('open.zf.trigger', handleOpen);
$('selector').on('close.zf.trigger', handleClose);
$('selector').on('toggle.zf.trigger', handleToggle);
Throttle
Throttle prevents a function from being executed more than once every n milliseconds. Throttling is often used in cases where it's disadvantageous to trigger a callback every time an event is triggered (during a continuous action), but you still want to trigger a reaction while the event is occurring.
// Throttled resize function
$(window).on('resize', Foundation.util.throttle(function(e){
// Do responsive stuff
}, 300));
Miscellaneous
Foundation includes a couple of useful features in the core library that are used in many places. Foundation.GetYoDigits([number, namespace])
returns a base-36, pseudo-random string with a hyphenated namespace (if you include one). Both arguments are optional; by default, it will return a string six characters long. Foundation.getFnName(fn)
returns a string representation of a named function. Foundation.transitionend()
is a function that returns the string of the properly vendor-prefixed version of transitionend events.
Code Examples
Here are two examples of how to use multiple utilities to create a functional application. You can run these on your own computer to see the output.
Example 1: Using Keyboard Utilities
<!DOCTYPE html>
<html>
<head>
<title>Foundation CSS JavaScript Utilities Example</title>
<link
rel="stylesheet"
href="https://cdn.jsdelivr.net/npm/foundation-sites@6.6.3/dist/css/foundation.min.css"
/>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/foundation-sites@6.6.3/dist/js/foundation.min.js"></script>
</head>
<body>
<button style="margin: 50px" class="button" data-open="exampleModal">
Open Modal
</button>
<div class="reveal" id="exampleModal" data-reveal>
<h1>Awesome. I Have It.</h1>
<p class="lead">Your couch. It is mine.</p>
<p>
I'm a cool paragraph that lives inside of an even cooler modal. Wins!
</p>
<button
class="close-button"
data-close
aria-label="Close modal"
type="button"
>
<span aria-hidden="true">×</span>
</button>
</div>
<script>
$(document).foundation();
// Register a keyboard event
Foundation.Keyboard.register("exampleModal", {
ENTER: "open",
ESCAPE: "close",
});
// Handle a keyboard event
$(document).on("keydown", function (e) {
Foundation.Keyboard.handleKey(e, "exampleModal", {
open: function () {
$("#exampleModal").foundation("open");
},
close: function () {
$("#exampleModal").foundation("close");
},
});
});
</script>
</body>
</html>
In this example, we have a button that opens a modal when clicked. Users can also open the modal by pressing the Enter key and close it by pressing the Escape key. When you open this HTML file in a web browser, you'll see a button. Try clicking the button to open the modal, and press the Enter and Escape keys to open and close the modal.
Output
Example 2: Using MediaQuery and Motion Utilities
<!DOCTYPE html>
<html>
<head>
<title>Foundation CSS JavaScript Utilities Example 2</title>
<link
rel="stylesheet"
href="https://cdn.jsdelivr.net/npm/foundation-sites@6.6.3/dist/css/foundation.min.css"
/>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/foundation-sites@6.6.3/dist/js/foundation.min.js"></script>
</head>
<body>
<div
id="myElement"
style="
margin: 100px;
width: 100px;
height: 100px;
background-color: #008000;
"
></div>
<script>
$(document).foundation();
// Check if the screen is at least as wide as a breakpoint
if (Foundation.MediaQuery.atLeast("large")) {
console.log("Screen is at least `large`.");
}
// Animate an element
Foundation.Move(500, $("#myElement"), function () {
$("#myElement").css({
transform: "translateX(1000px)",
transition: "transform 5000ms ease",
});
});
</script>
</body>
</html>
In this example, we have a div
element with the id 'myElement'. When you open this HTML file in a web browser, if your screen size is at least 'large', you'll see the message 'Screen is at least large
.' in your console. Additionally, the div
element will move 1000 pixels to the right over 5 seconds.
You can open the console in your web browser to see any messages logged by the JavaScript code. In most browsers, you can open the console by right-clicking on the webpage, selecting 'Inspect' or 'Inspect Element', and then clicking on the 'Console' tab.
Output
Conclusion
The JavaScript utilities provided by Foundation CSS are a powerful set of tools that can greatly enhance your web development process. They offer a wide range of functionalities, from handling keyboard events to managing media queries, and from creating animations to controlling timers. By understanding and utilizing these utilities, you can create more interactive and responsive web applications.
References
Subscribe to my newsletter
Read articles from Anay Sinhal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
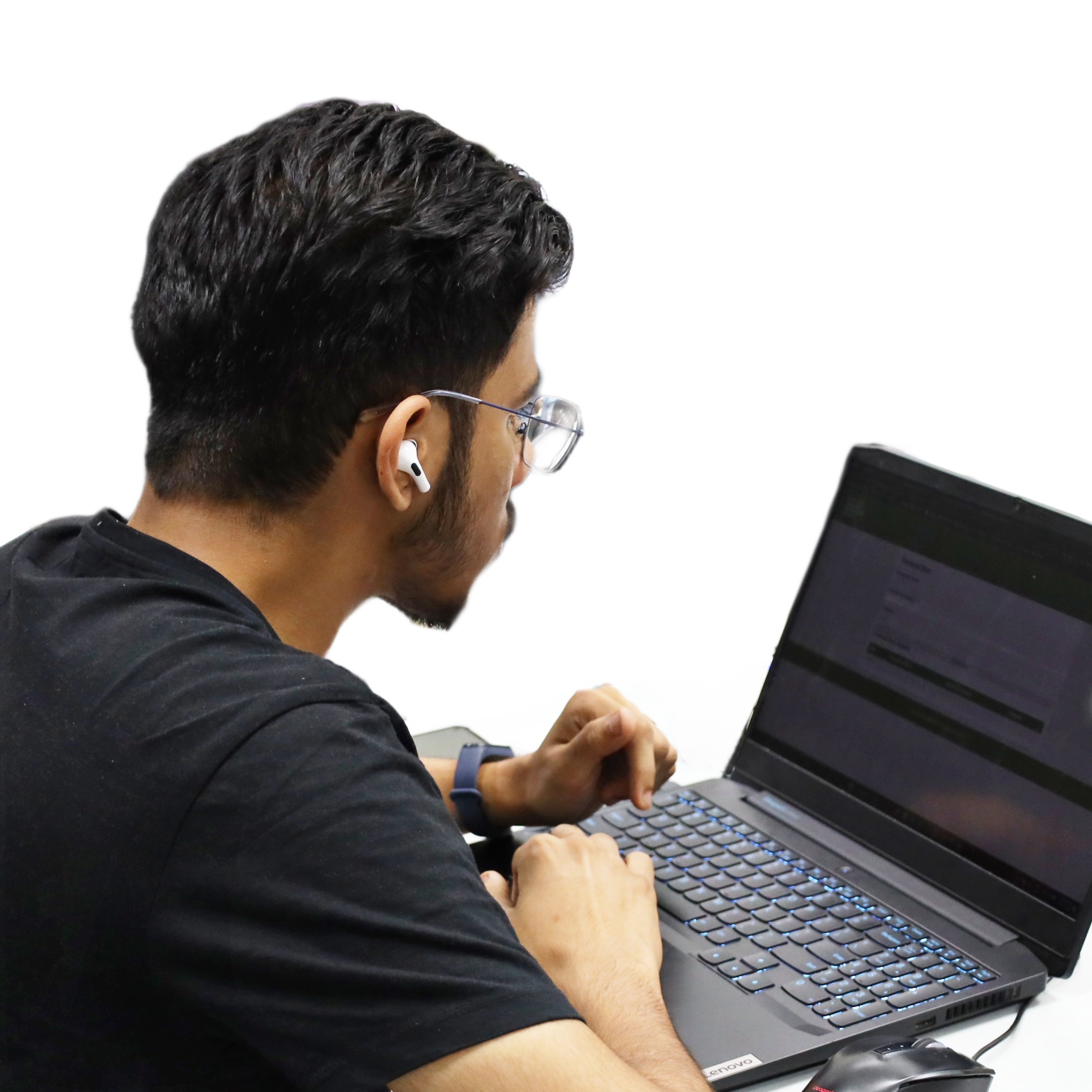
Anay Sinhal
Anay Sinhal
I am a CSE undergrad pursuing B.Tech from JKLU, Jaipur, and have completed a semester at IIT Gandhinagar. Also, I am currently a Software Development Intern. My professional interest lies in Full Stack Development, UI/UX Design, and I am proficient in programming languages such as Python, C++, and Java.I have also worked with Java Spring Boot and Zoho Creator. I have achieved a 2-star rating on Codechef and am committed to continuously improving my skills.