Enhancing Laravel Scout Search with a Stop Words Removal Helper
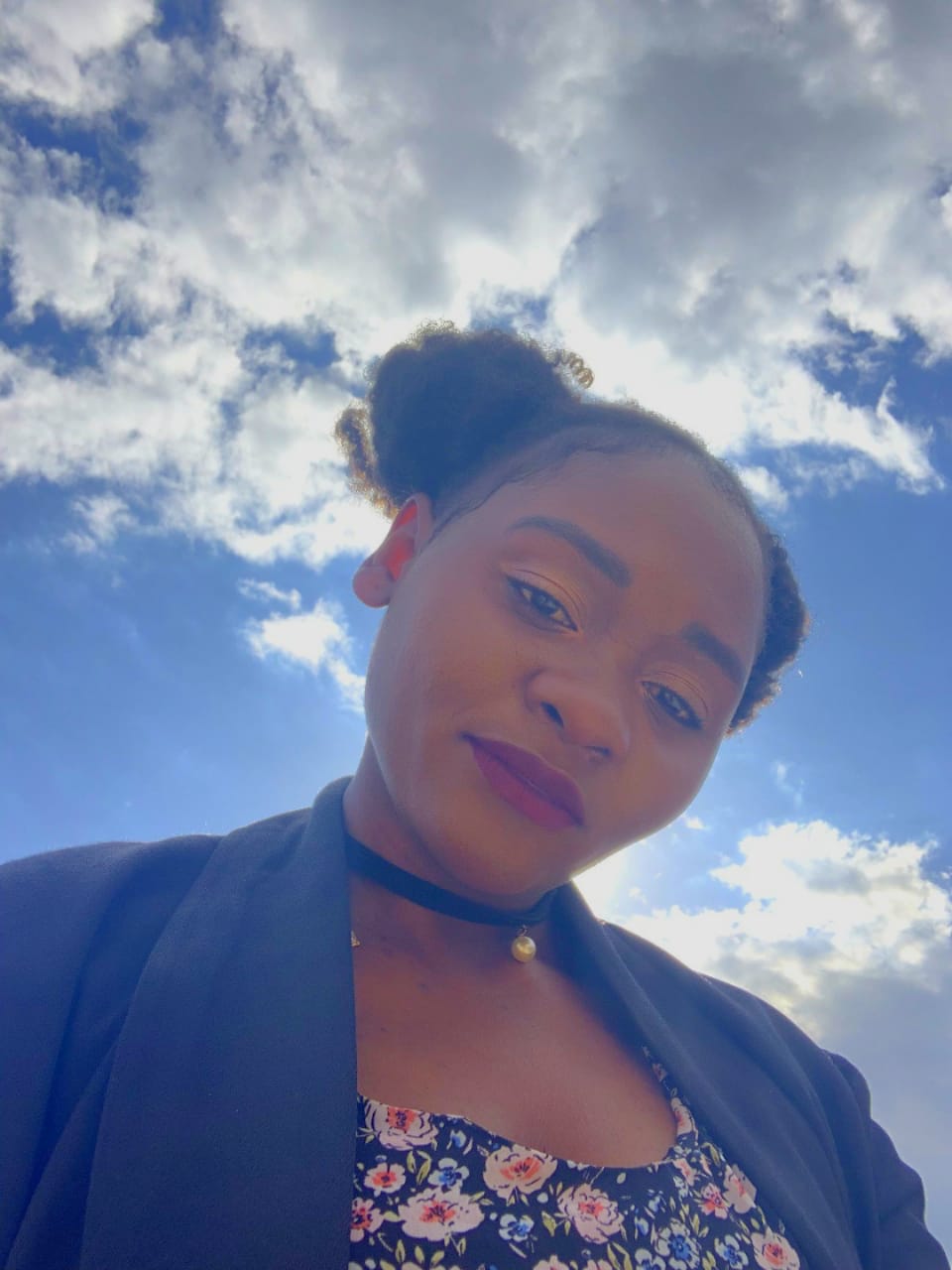
Introduction
When building search functionality with Laravel Scout, it's essential to provide users with accurate and relevant results. One common obstacle in search algorithms is the presence of stop words, which are commonly used words that carry little or no significance in the search context. In this blog post, we will explore how to create a helper function in Laravel Scout that removes stop words from a given text, enhancing the search experience for your users.
Understanding Stop Words
Stop words are words that are frequently used in natural language but do not contribute significantly to the meaning or relevance of a text. Examples of common stop words include "a," "an," "the," "and," and "in." Including these words in search queries can lead to less accurate results, as they are often found in almost every document and don't provide meaningful insights into the content.
Creating the Stop Words Removal Helper
To address the issue of stop words in Laravel Scout, we can create a helper function that removes these words from a given text. Let's walk through the steps to implement this helper function:
Open your Laravel project and navigate to the
app\Helpers
directory.Create a new file called
ScoutHelper.php
(or any other name you prefer) inside theapp\Helpers
directory.Define a new helper function,
removeStopWords
within theScoutHelper
class. This function will take text input and remove any stop words from it. Here's an example implementation:
<?php
namespace App\Helpers;
class ScoutHelper
{
public static function removeStopWords($text)
{
// Define your list of stop words
$stopWords = [
'a', 'an', 'and', 'the', 'or', 'but', 'in', 'on', 'at', 'to', 'from',
// Add more stop words as needed
];
// Split the text into individual words
$words = explode(' ', $text);
// Filter out the stop words
$filteredWords = array_filter($words, function ($word) use ($stopWords) {
return ! in_array(strtolower($word), $stopWords);
});
// Join the filtered words back into a single string
$filteredText = implode(' ', $filteredWords);
return $filteredText;
}
}
- Save the
ScoutHelper.php
file. Implementing the Helper Function
Now that we have our removeStopWords helper function, we can integrate it into our code to enhance search functionality. Let's take a look at an example implementation:
<?php
use App\Helpers\ScoutHelper;
use App\Models\Blog;
public function search()
{
$text = 'This is a sample text to be filtered';
// Remove stop words from the text
$filteredText = ScoutHelper::removeStopWords($text);
// Perform the search using the filtered text
$results = Blog::search($filteredText)->get();
// Process and display the search results
}
In this example, we start by defining a sample text that we want to search within. We then call the removeStopWords
helper function, passing in the text to be filtered. The function removes any stop words from the text, resulting in a filtered version.
Next, we use the filtered text to perform the search operation using Laravel Scout. By excluding the stop words, we improve the relevance and accuracy of the search results
Subscribe to my newsletter
Read articles from Imali Susan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
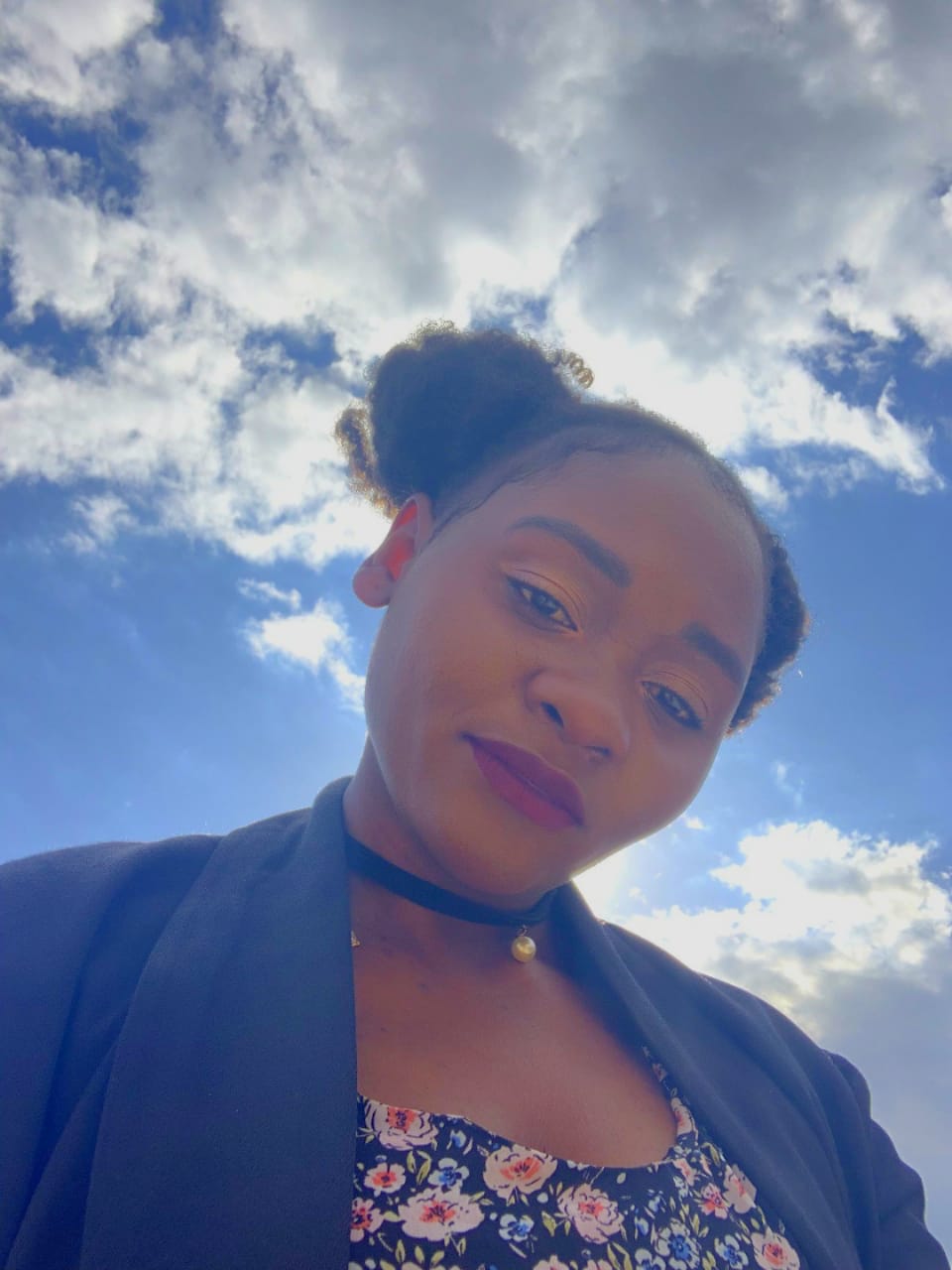
Imali Susan
Imali Susan
A Laravel Lover