What I learn today coding with ChatGPT
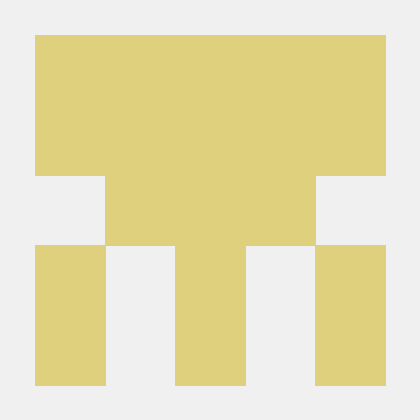
Struggle: You were unsure about how to filter the FAQs based on the selected types and display only the relevant FAQs.
Solution: To address this struggle, I suggested using the some array method in the selectedTypes.filter
method. The filter
method creates a new array by filtering elements based on a specified condition. In this case, we want to filter the FAQs based on the selected types.
In the code, we iterate over the faqList?.data?.rows
array using the filter
method and provide a callback function. Inside the callback function, we use the some
method to check if at least one element in the selectedTypes
array matches the type
of the FAQ. If the condition is met, the FAQ is included in the filtered array.
const result = faqList?.data?.rows?.filter((faq) => {
return selectedTypes.some((type) => faq.type === type);
});
setSearchResult(result || []);
By using the some
method, we ensure that only the types with matching FAQs are included in the result
array, which is then set as the new searchResult
.
Important concept: The some
method is a powerful tool for array manipulation. It iterates over an array and returns true
if at least one element satisfies the specified condition in the callback function, or false
if none of the elements match the condition. It allows you to check if any element in an array meets a particular condition.
Struggle: You faced difficulty in scrolling to the selected FAQ type section when it was clicked.
Solution: To overcome this struggle, I recommended using the scrollIntoView
function. This function is a built-in browser API that scrolls the specified element into the viewport. In our case, we want to scroll to the FAQ section based on the selected type.
In the code, I introduced a new function called scrollToType
, which uses document.getElementById
to get the element with the corresponding type
and then calls scrollIntoView
on that element. By providing the behavior: 'smooth'
option, the scrolling animation appears smooth and visually appealing.
const scrollToType = () => {
const element = document.getElementById(type);
if (element) {
element.scrollIntoView({ behavior: 'smooth' });
}
};
I then updated the handleFaqTypeClick
function to call scrollToType
to scroll to the selected FAQ type section. If the type is already selected, we scroll immediately; otherwise, we update the selected types, update the search results, and then scroll to the section.
const handleFaqTypeClick = (type) => {
const isSelected = selectedTypes.includes(type);
if (isSelected) {
scrollToType();
} else {
setSelectedTypes([type]);
setSearchResult(faqList?.data?.rows || []);
scrollToType();
}
};
Important concept: The scrollIntoView
function is a useful method to navigate to specific elements within a webpage. It is commonly used for smooth scrolling to improve user experience and provide visual feedback when navigating to different sections of a page.
Summary
By understanding and implementing these concepts, you were able to enhance the search and filtering functionality of your FAQ page, improving the user experience and overall performance. The use of array methods like filter
and some
allowed you to filter and manipulate data effectively, while the scrollIntoView
function helped you navigate to specific sections on the page smoothly.
Subscribe to my newsletter
Read articles from Seongeun Jo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
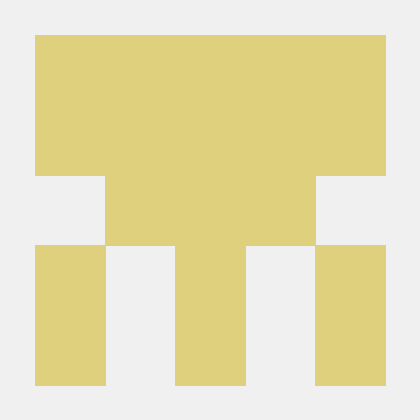