strcpy in C: A Comprehensive Guide

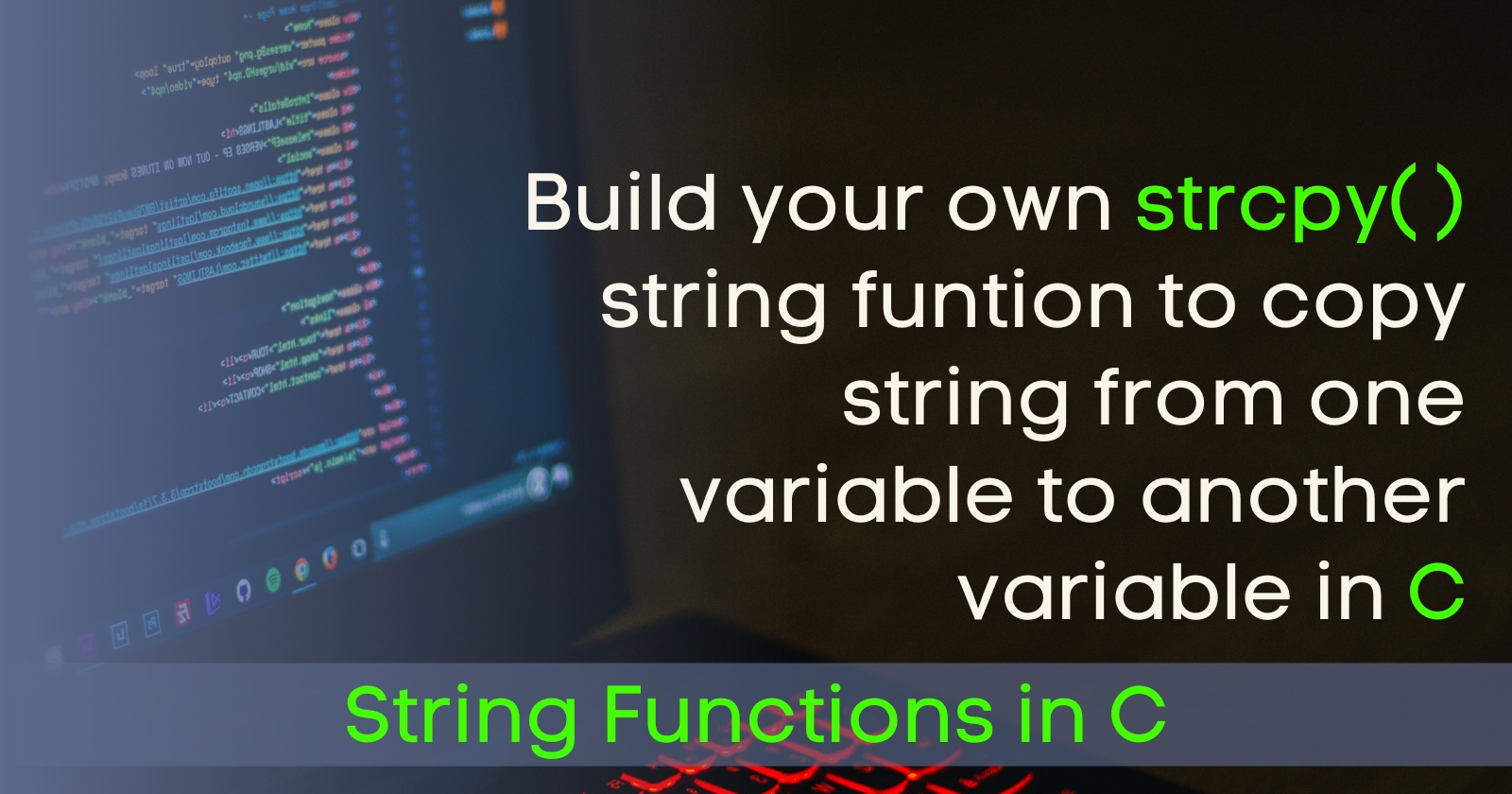
Introduction to strcpy( )
The strcpy( ) inbuilt string function that is contained in the string.h header file is a function that copies a string from one variable to another variable. It copies all the characters from the variable including the null character (\0) to the other variable.
We will be looking at how to use the built-in strcpy function as well as build our strcpy from scratch with enough illustration and an example each to show how the two work.
The Built-in strcpy( ) Function
The Built-in strcpy( ) string function is found in the string.h header file, just like any other of the string functions.
To make use of this function, you just need to pass two arguments into the function. Of these two arguments, the first argument will be the destination and the second argument will be the source.
The destination is where the string is to be copied, while the source is the string that is to be copied.
One other very important thing about the strcpy( ) function is that the variable that will act as the destination argument needs to have an array size that is enough to accommodate the string from the source including the null character '\0'.
Let us take this example:
#include <stdio.h>
#include <string.h>
int main(void)
{
/* declared the destination variable - dest as an array with size 50
to have enough space to take all the string from the source variable */
char dest[50];
// declared the source variable - src.
char *src;
// assigned a string to the source variable - src.
src = "I Love Programming in C";
// print out the destination before applying the strcpy function.
printf("Before strcpy = %s\n", dest);
// apply the strcpy to copy from the src to the dest variable.
strcpy(dest, src);
// print out the destination after applying the strcpy function
printf("After strcpy = %s\n", dest);
return (0);
}
This is how to apply and use the inbuilt strcpy string function. Always remember to include the string.h header file at the beginning of the file as that is the file that contains the function declaration.
Building a Custom strcpy( ) Function
To build a custom strcpy function, here we will be using the name _strcpy for the custom function.
The main concept behind this function is that we will take advantage of the null character that terminates the string we will be copying from the source. It will copy the string from the first character down until it gets to the null terminating character \0
and then finally, it will then append the null terminating character \0
to mark the end of the string we just copied to the destination variable.
Here is an algorithm to build this function:
Declare the destination variable as an array, that is the variable we will be copied to, and the size should be sufficient enough to take the entire string including a terminating null character that will be appended manually at the end.
Loop through the string from the source variable, that is the string we intend to copy.
Keep looping from the first character, which will be at index zero, until it gets to the terminating null character.
As it loops from one character to another, copy the character to the destination variable, that is to the place we are copying the string to.
Below is the implementation of the above algorithm:
#include <string.h>
char *_strcpy(char *dest, char *src)
{
// declare a count for the loop
int i;
/* using the for loop to iterate through all the characters
of the string contained in the src variable */
for (i = 0; src[i] != '\0'; i++)
{
/* for every character from the src, set it as a corresponding
character in the dest variable */
dest[i] = src[i];
}
// append the terminating null character to the end of the string
dest[i] = '\0';
/* always return the variable pointer of the string set to the
destination variable, which in this case is the dest */
return (dest);
}
Let us now test this code by including our main function:
#include <stdio.h>
#include <string.h>
char *_strcpy(char *dest, char *src);
int main(void)
{
char dest[10];
char *src;
src = "Copy me";
printf("Before the strcpy = %s\n", dest);
_strcpy(dest, src);
printf("After the strcpy = %s\n", src);
return (0);
}
char *_strcpy(char *dest, char *src)
{
int i;
for (i = 0; src[i] != '\0'; i++)
{
dest[i] = src[i];
}
dest[i] = '\0';
return (dest);
}
This is how to build your strcpy function, known as string copy.
Conclusion
This is how you can build your strcpy (string copy) string function from scratch and implement it.
Thank you for reading, you can connect with me on Twitter and LinkedIn.
Subscribe to my newsletter
Read articles from Gideon Bature directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Gideon Bature
Gideon Bature
A Seasoned Software Engineer and Technical Writer.