A Simple Way to Convert Word to PDF in C#
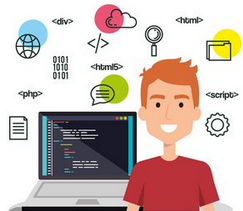
PDF is a file format that is widely used for sharing documents on different platforms. It is a popular choice for businesses, educational institutions and individuals. After you have created a Word document, you may need to convert it to PDF in many cases. This article will focus on how to convert Word to PDF in C# using a .NET Word library.
Convert Doc or Docx to PDF
Convert Word to Password-Protected PDF
Install the Library
Before we begin, you will need to download Spire.Doc for .NET from the link or follow the below steps to install it via NuGet:
1. Open Visual Studio and create a new project.
2. Right-click on the project in the Solution Explorer and select “Manage NuGet Packages”.
3. Search for “Spire.Doc” and install the latest version.
Convert a Doc or Docx Document to PDF
With only three lines of code, we can easily convert a .doc/.docx document to PDF with C#. First we need to create an instance of Document class and load an input Word document. Then we can convert the document to PDF using the Document.SaveToFile(string fileName, FileFormat.PDF) method.
Sample Code:
using Spire.Doc;
namespace ToPDF
{
class Program
{
static void Main(string[] args)
{
//Create a Document object
Document document = new Document();
//Load a sample Word document
document.LoadFromFile("REPORT.docx");
//Save the document to PDF
document.SaveToFile("ToPDF.pdf", FileFormat.PDF);
}
}
}
Convert Word to Password-Protected PDF
During conversion, we are also allowed to set both open password and permission password for the output PDF document and encrypt it using the ToPdfParameterList.PdfSecurity.Encrypt() method.
Sample Code:
using Spire.Doc;
namespace ToPDFWithPassword
{
class Program
{
static void Main(string[] args)
{
//Create a Document object
Document document = new Document();
//Load a sample Word document
document.LoadFromFile("REPORT.docx");
//Create a ToPdfParameterList instance
ToPdfParameterList parameters = new ToPdfParameterList();
//Set open password and permission password for PDF
string openPsd = "123";
string permissionPsd = "abc";
parameters.PdfSecurity.Encrypt(openPsd, permissionPsd, PdfPermissionsFlags.Default, PdfEncryptionKeySize.Key128Bit);
//Save the Word document to PDF with password
document.SaveToFile("ToPDFWithPassword.pdf", parameters);
}
}
}
In addition to the above two examples, Spire.Doc for .NET is also capable of converting Word to PDF with bookmarks, with fonts embedded or set image quality. Check the below link for a comprehensive guide:
Other Conversion Features Supported by the .NET Word API:
Convert Word to Images (JPG, PNG and SVG) in C#/VB.NET
Convert Word to Excel in C#/VB.NET
Subscribe to my newsletter
Read articles from Jonathon directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
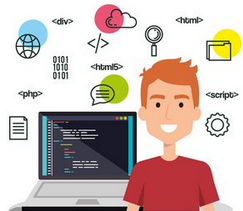