Demystifying Confusing Topics in JavaScript
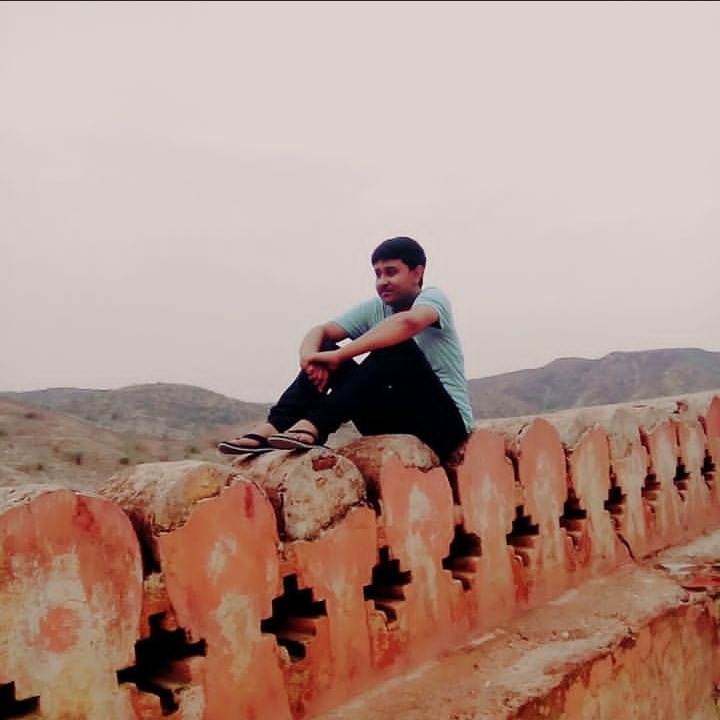
When I began my journey of learning JavaScript, one aspect that often left me perplexed was some of the confusing topics of JavaScript. I soon realized that I wasn't alone in this confusion, many beginners stumble upon this issue and find themselves wondering about their purpose and how they differ from one another.
Concat()
Starting with the easiest method concat. This method is used to merge two or more arrays. This method does not change the current array but returns a new array.
const arr1 = ['a','b']
const arr2 = [1, 2]
const arr = arr1.concat(arr2)
//output => ['a','b', 1, 2]
When learning this concept, I was confused with the '+' operator, which is commonly referred to as an arithmetic operator. Interestingly, the '+' operator can also be used to join two or more arrays, but it behaves differently from the concat
method. While the concat
method returns a new array by combining the original arrays, the '+' operator converts the arrays to strings and concatenates their string representations. Let me provide an example to illustrate this distinction.
const arr1 = ['a','b']
const arr2 = [1, 2]
const arr = arr1 + arr2
//output => "a,b1,2"
every()
The every()
method tests whether all elements in the array pass the test implemented by the provided function. It returns a Boolean value. The every()
method is an iterative method. It calls a provided callbackFn
function once for each element in an array, until the callbackFn
returns a false value. The example of every()
is as follows
const numbers = [1, 2, 3, 4, 5];
const allEven = numbers.every(num => num % 2 === 0);
console.log(allEven); // Output: false (not all numbers are even)
filter()
The JavaScript filter()
Method is used to create a new array from a given array consisting of only those elements from the given array which satisfy a condition set by the argument method. The filter()
method is an iterative method. It calls a provided callbackFn
function once for each element in an array, and constructs a new array of all the values for which callbackFn
returns a truthy value. Array elements that do not pass the callbackFn
tests are not included in the new array.
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(num => num % 2 === 0);
console.log(evenNumbers); // Output: [2, 4] (filtered array with even numbers)
What is the difference between every() and filter()?
While both every()
and filter()
methods involve the use of a callback function and iterate through each element of an array while evaluating a condition, they have distinct purposes and outcomes.
The every()
method tests whether all elements in an array satisfy a given condition. It iterates through each element and stops as soon as it encounters an element that doesn't meet the condition, returning false. If all elements pass the condition, it returns true. In other words, it checks if every element in the array satisfies the condition.
On the other hand, the filter()
method creates a new array with all elements that pass a specific condition. It iterates through each element and includes elements in the resulting array if the condition returns true. It does not modify the original array. It returns a new array containing the filtered elements, based on the condition.
forEach()
The forEach()
method executes a provided function once for each array element. The forEach()
method in JavaScript is used to execute a provided callback function once for each element in an array, in ascending order.
It iterates through each element in the array, sequentially executing the provided callback function for each element. The callback function can access the current element, its index, and the original array if needed.
const arr1 = ['a', 'b', 'c'];
arr1.forEach(element => console.log(element));
// Expected output: "a"
// Expected output: "b"
// Expected output: "c"
map()
The map()
method is used to create a new array by applying a provided callback function to each element of an existing array. It iterates over the elements of the array, executes the callback function for each element, and returns a new array with the results.
const array1 = [1, 4, 9, 16];
// Pass a function to map
const map1 = array1.map(x => x * 2);
console.log(map1);
// Expected output: Array [2, 8, 18, 32]
What is the difference between map() and forEach()?
The map()
method creates a new array by applying a provided callback function to each element of the original array. It returns a new array containing the results of the callback function applied to each element. The length and order of the new array will be the same as the original array. map()
is commonly used when you want to transform or map each element of an array into a new value or object.
On the other hand, the forEach()
method iterates over each element of the array and executes a callback function for each element. It does not return a new array; instead, it is typically used for performing side effects or operations on the elements, such as logging or modifying in place. forEach()
is useful when you need to perform an action on each element without requiring a transformed or filtered result.
rest parameter
The rest parameter is a useful feature that lets a function accept any number of arguments. It's represented by three dots (...) followed by a parameter name. The rest parameter gathers all the extra arguments passed to the function and stores them in an array. This makes it easy to work with functions that can handle different amounts of input. Instead of listing each argument separately, the rest parameter simplifies the process by collecting them all in one place. It's like having a basket to gather any number of items.
function printItems(...items) {
console.log(items); //output => ["Apple","Banana","Orange"]
}
printItems("Apple", "Banana", "Orange");
spread Parameter
The spread syntax (...) is a powerful tool that lets you easily work with arrays. It allows you to take elements from an array and spread them out into different places. You can use it to copy arrays, merge arrays, or even pass multiple elements as arguments to functions. It simplifies working with arrays and gives you more flexibility in manipulating and combining data.
const array = [1, 2, 3];
const newArray = [...array];
console.log(newArray)//output => [1, 2, 3]
What is the difference between the spread and rest parameters?
The spread syntax, denoted by the three dots (...
), is used to expand elements of an iterable (like an array or string) into individual elements. It allows you to create copies of arrays, merge arrays, or pass individual elements as function arguments. Essentially, it "spreads" the elements into a new context. For example, [...array]
creates a new array with the elements of the array spread out.
On the other hand, the rest parameter, also denoted by the three dots (...
), is used in function declarations to gather multiple arguments into an array. It allows functions to accept a variable number of arguments and treat them as an array. The rest parameter collects the "rest" of the arguments into an array, making it easier to work with variable-length argument lists within a function.
Subscribe to my newsletter
Read articles from akshit bhati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
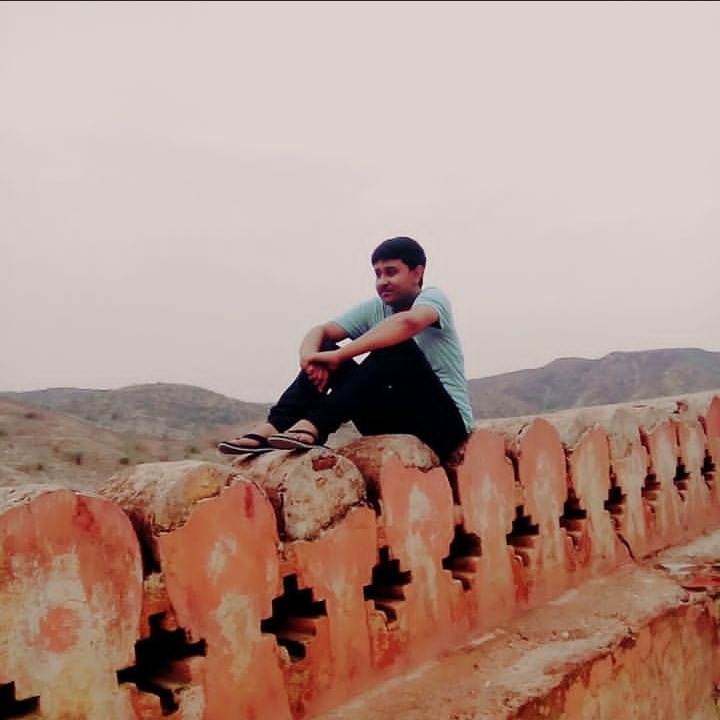