Abstract contracts and Interfaces in solidity
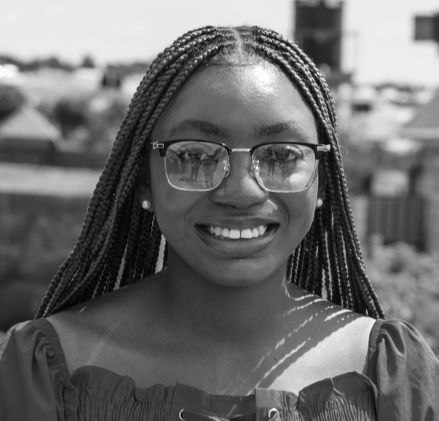
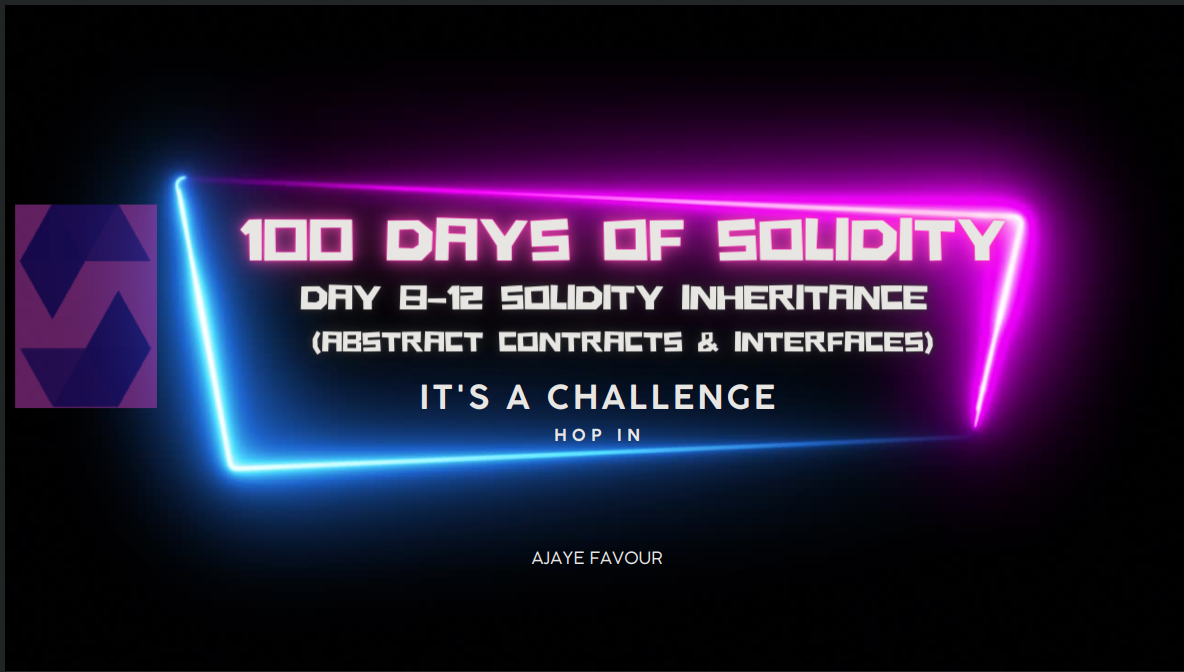
Abstract Contracts
Now, I'm sitting here thinking of a way to explain abstract contracts in their simplest form. First, let's start by knowing what an abstract contract is.
What is abstract contract?
An abstract contract in Solidity is a contract that cannot be instantiated directly. It is used as a base contract and defines a set of functions that must be implemented by any derived contracts. Abstract contracts are useful for defining common functionality that can be shared across multiple contracts.
From solidity documentation...
Contracts must be marked as abstract when at least one of their functions is not implemented or when they do not provide arguments for all of their base contract constructors.
Understanding abstract contract using an example
I'm hoping we can make it easy with this example.
Alice and her friends are planning a big event, like a music festival, and they want to create a set of rules that all the performers must follow. However, they realize that the rules can vary depending on the type of performer (e.g., singer, dancer, or guitarist).
To simplify things, they create an abstract contract called "Performer
" that outlines common rules and requirements for all types of performers. This abstract contract serves as a blueprint or template for the specific contracts that each performer will have.
Think of the abstract contract as a general outline or skeleton. It defines certain functions or properties that every performer contract must have, but it doesn't provide the full implementation. It's like having a checklist of rules and expectations for all performers, without specifying how each rule is carried out.
Each specific performer (e.g., SingerContract
, DancerContract
) then inherits from the abstract contract. They provide their own implementation of the abstract functions and may have additional functions or properties specific to their role.
By using abstract contracts, you can ensure consistency and enforce certain behaviors across different performers'
contracts. It allows you to define a common structure and set of rules that all performers must adhere to, while still allowing flexibility for performers to have their own unique features.
An abstract contract is like a blueprint or set of rules that all performers must follow. It outlines common functions and properties without providing full details. Each specific performer contract then inherits from the abstract contract and adds their own implementation. It helps ensure consistency and allows for customization
Here is another example for clarification:
Bob is building a car factory, and he has a blueprint for creating different types of cars. Each car has certain functions it can perform, like starting the engine or accelerating.
Now, let's say Bob has a blueprint for a specific type of car called "SportsCar
." This blueprint includes all the necessary functions for a car, like starting the engine, but it doesn't provide full details on how to implement some of these functions. It's like having a partial blueprint where some instructions are missing.
In this case, you need to mark the "SportsCar
" blueprint as "abstract
" because it's not complete. It's like a placeholder or reminder that certain functions still need to be implemented.
By marking a contract as "abstract
," you're basically saying, "Hey, this blueprint is not complete or it's missing some crucial information, so it cannot be used directly to create an actual object."
Marking a contract as "abstract" means that the blueprint is not fully finished or lacks important details. It's like a placeholder or reminder that certain functions are missing or that required arguments are not provided. It's a way of indicating that the blueprint cannot be used directly to create an actual object until those missing parts are completed or filled in.
Abstract contracts are similar to Interfaces but an interface is more limited in what it can declare.
An abstract contract is declared using the abstract keyword.
Here is an example;
The contract Performer
is defined as abstract, because the function rules()
is declared, but no implementation is provided (no implementation body {} is given).
If a contract inherits from an abstract contract and does not implement all non-implemented functions by overriding, it needs to be marked as abstract as well.
Abstract contracts cannot override an implemented virtual function with an unimplemented one. Abstract contracts are useful in the same way that defining methods in an interface is useful. It is a way for the designer of the abstract contract to say “any child of mine must implement this method”.
Interfaces
Interfaces are similar to abstract contracts, but they cannot have any functions implemented.
What is interface in solidity?
In Solidity, an interface is a way to define the external-facing functions of a contract without providing the implementation details. It serves as a blueprint or contract template for interacting with other contracts.
Interfaces are typically used when you want to interact with functions of other contracts without having to include the entire contract's code or inherit from it. It provides a way to specify the function names, arguments, return types, and visibility without including the actual code implementation.
Understanding abstract contract using an example
Using real life examples... Picture this;
You're organizing a language exchange event, where people from different countries come together to practice speaking different languages.
An interface is like a language dictionary or guidebook that defines a set of functions and their expected behavior. It specifies the functions that a contract must implement in order to be considered compatible with the interface.
See interface as a common language that everyone participating in the language exchange event must understand. It provides a set of rules or guidelines for contracts to follow, ensuring they have certain functions and behaviors.
For example, you can create an interface called "LanguageExchange" that includes functions like "speak" and "listen." The interface defines what these functions should do, but it doesn't provide the actual implementation.
Different contracts can now choose to "implement" this interface by including the required functions with their own specific implementation. It's like saying, "Hey, if you want to participate in our language exchange event, your contract needs to be able to speak and listen."
Using interfaces, you can ensure that contracts have certain functions and behaviors, even if they are created by different developers. It allows for interoperability and ensures that contracts can interact with each other using a common set of functions.
Further restrictions of interfaces
Interfaces have other restrictions aside from not having any functions implemented. They are;
They cannot inherit from other contracts, but they can inherit from other interfaces.
All declared functions must be external in the interface, even if they are public in the contract.
They cannot declare a constructor.
They cannot declare state variables.
They cannot declare modifiers.
Interfaces are denoted by their own keyword:
Contracts can inherit interfaces as they would inherit other contracts.
In case you missed solidity data types, click here.
Read about Solidity's fallback function and function overloading here.
Also, click here to learn more about variables and control structures in solidity and here for solidity functions.
Know a lot more about inheritances here
Click here to see the Github repo for this 100 days of solidity challenge.
Click here for guidelines for becoming a Solidity developer.
To learn more about blockchain, click here.
To learn more about Web3, click here.
Follow me for more knowledge on Solidity, Ethereum, and blockchain.
Subscribe to my newsletter
Read articles from Favour Ajaye directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
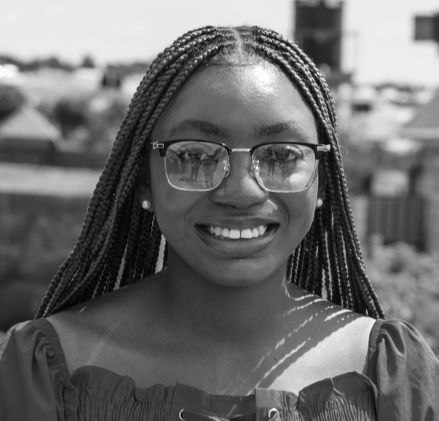
Favour Ajaye
Favour Ajaye
smart contract developer