Create a social dApp using Spheron Storage SDK
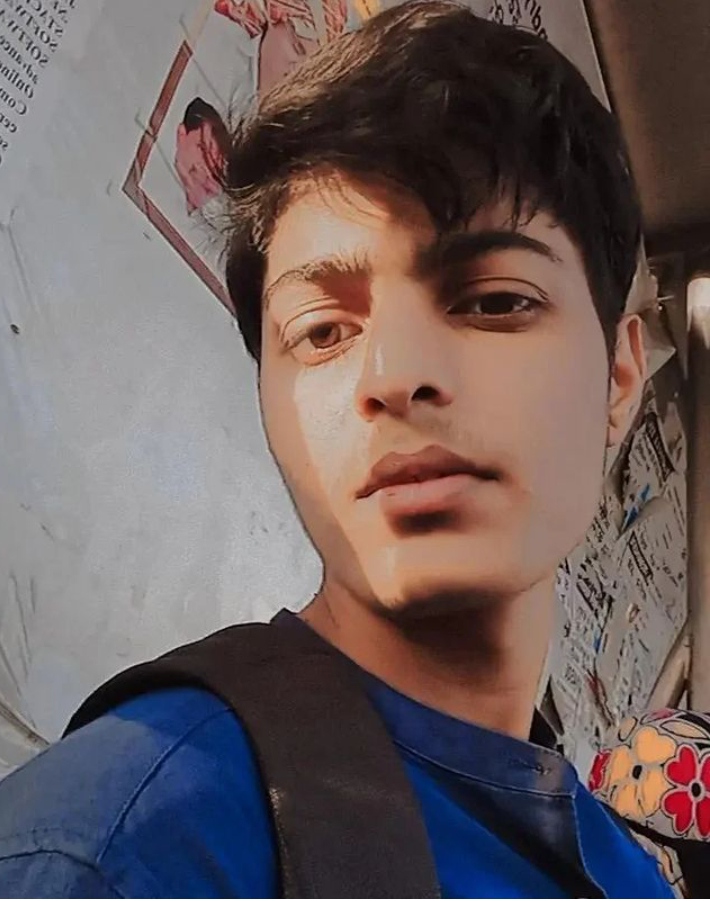

In this tutorial, we will explore how to create a social decentralized application (dApp) using the Spheron Storage SDK. Spheron Storage provides a robust and secure backend solution for storing and managing data in a decentralized manner. By leveraging the power of Spheron Storage, we can build a social dApp with features like user registration, authentication, profile management, posting, and social interactions.
Setup Environment:
Install the necessary development tools, such as Node.js and npm (Node Package Manager).
Create a new directory for your project and navigate to it using the command line.
Refer to Spheron CLI for the setup.
Initialize a new project:
Run the following command to initialize a new project and create a package.json file:
npm init -y
Install Spheron Storage SDK:
Use the following command to install the Spheron Storage SDK package:
npm i @spheron/storage
Import the SDK:
Create a new JavaScript file, such as
app.js
, and import the Spheron Storage SDK at the top of the file:const spheron = require('@spheron/spheron-sdk');
Connect to Spheron Storage:
Initialize the Spheron Storage SDK by providing your API key and secret:
const storage = new spheron.Storage({ apiKey: 'YOUR_API_KEY', apiSecret: 'YOUR_API_SECRET', });
you are ready to go.
Implement user authentication using the Spheron Storage SDK:
Install the necessary dependencies:
You'll need the
express
framework and thebcrypt
library for password hashing.Run the following command to install them:
npm install express bcrypt
Create a new file, e.g.,
auth.js
, and import the required modules:const express = require('express'); const bcrypt = require('bcrypt'); const spheron = require('@spheron/spheron-sdk'); const router = express.Router(); const storage = new spheron.Storage({ apiKey: 'YOUR_API_KEY', apiSecret: 'YOUR_API_SECRET', }); // Define your routes and authentication logic here
Implement the user registration route:
router.post('/register', async (req, res) => { const { username, password } = req.body; // Check if the username is already taken const existingUser = await storage.findOne('users', { username }); if (existingUser) { return res.status(409).json({ error: 'Username already exists' }); } // Hash the password const hashedPassword = await bcrypt.hash(password, 10); // Create a new user const newUser = { username, password: hashedPassword, }; try { const result = await storage.create('users', newUser); res.status(201).json(result); } catch (error) { console.error(error); res.status(500).json({ error: 'Failed to create user' }); } });
Implement the user login route:
router.post('/login', async (req, res) => { const { username, password } = req.body; // Find the user by username const user = await storage.findOne('users', { username }); if (!user) { return res.status(404).json({ error: 'User not found' }); } // Compare the provided password with the stored hashed password const passwordMatch = await bcrypt.compare(password, user.password); if (!passwordMatch) { return res.status(401).json({ error: 'Invalid password' }); } // Generate a token or session for authenticated user (you can use a library like jsonwebtoken) res.json({ message: 'Login successful' }); });
Add the authentication routes to your application:
// Assuming you have an instance of the express app app.use('/auth', router);
Remember to replace 'YOUR_API_KEY'
and 'YOUR_API_SECRET'
with your actual Spheron Storage API credentials.
Implementing user profiles in the social dApp using the Spheron Storage SDK:
Set up the server:
const express = require('express'); const spheron = require('@spheron/spheron-sdk'); const app = express(); const port = 3000; const storage = new spheron.Storage({ apiKey: 'YOUR_API_KEY', apiSecret: 'YOUR_API_SECRET', }); app.use(express.json()); // Start the server app.listen(port, () => { console.log(`Server is running on port ${port}`); });
Implement the route for creating or updating a user's profile:
app.post('/profile', async (req, res) => { const { userId, name, profilePicture, bio } = req.body; try { // Check if the user exists const user = await storage.findOne('users', { id: userId }); if (!user) { return res.status(404).json({ error: 'User not found' }); } // Update user profile information user.name = name; user.profilePicture = profilePicture; user.bio = bio; const result = await storage.update('users', user.id, user); res.json(result); } catch (error) { console.error(error); res.status(500).json({ error: 'Failed to update profile' }); } });
Implement the route for retrieving a user's profile:
app.get('/profile/:userId', async (req, res) => { const userId = req.params.userId; try { // Retrieve the user profile const user = await storage.findOne('users', { id: userId }); if (!user) { return res.status(404).json({ error: 'User not found' }); } res.json(user); } catch (error) { console.error(error); res.status(500).json({ error: 'Failed to retrieve profile' }); } });
Start the server and listen for incoming requests:
app.listen(port, () => { console.log(`Server is running on port ${port}`); });
Implement social interactions like likes, comments, and shares in the social dApp using the Spheron Storage SDK:
Add fields for tracking likes, comments, and shares to the post schema
in your Spheron Storage schema for the "posts" collection, add the following fields:
const postSchema = { userId: String, content: String, timestamp: Number, likes: Number, comments: Number, shares: Number, };
Implement routes for handling likes, comments, and shares:
// Like a post app.post('/posts/:postId/like', async (req, res) => { const postId = req.params.postId; try { // Find the post const post = await storage.findOne('posts', { id: postId }); if (!post) { return res.status(404).json({ error: 'Post not found' }); } // Update the like count post.likes = post.likes ? post.likes + 1 : 1; const result = await storage.update('posts', postId, post); res.json(result); } catch (error) { console.error(error); res.status(500).json({ error: 'Failed to like the post' }); } }); // Comment on a post app.post('/posts/:postId/comment', async (req, res) => { const postId = req.params.postId; const { userId, comment } = req.body; try { // Find the post const post = await storage.findOne('posts', { id: postId }); if (!post) { return res.status(404).json({ error: 'Post not found' }); } // Update the comment count post.comments = post.comments ? post.comments + 1 : 1; const result = await storage.update('posts', postId, post); res.json(result); } catch (error) { console.error(error); res.status(500).json({ error: 'Failed to comment on the post' }); } }); // Share a post app.post('/posts/:postId/share', async (req, res) => { const postId = req.params.postId; const { userId, sharedPlatform } = req.body; try { // Find the post const post = await storage.findOne('posts', { id: postId }); if (!post) { return res.status(404).json({ error: 'Post not found' }); } // Update the share count post.shares = post.shares ? post.shares + 1 : 1; const result = await storage.update('posts', postId, post); // Implement the logic to share the post to the specified platform // e.g., call an external API or perform necessary actions res.json(result); } catch (error) { console.error(error); res.status(500).json({ error: 'Failed to share the post' }); } });
Implement a route for retrieving a single post with likes, comments, and shares:
app.get('/posts/:postId', async (req, res) => { const postId = req.params.postId; try { // Retrieve the post const post = await storage.findOne('posts', { id: postId }); if (!post) { return res.status(404).json({ error: 'Post not found' }); } res.json(post); } catch (error) { console.error(error); res.status
Conclusion:
That's it... this is a basic code to create a social dApp using Spheron Storage SDK. This app consists of user authentication, creating and retrieving a post and new profiles, and likes, comments, and shares to it.
Reference:
Spheron Storage SDK Docs: https://docs.spheron.network/sdk/
Spheron Docs: https://docs.spheron.network/
Subscribe to my newsletter
Read articles from Yogiraj Patil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
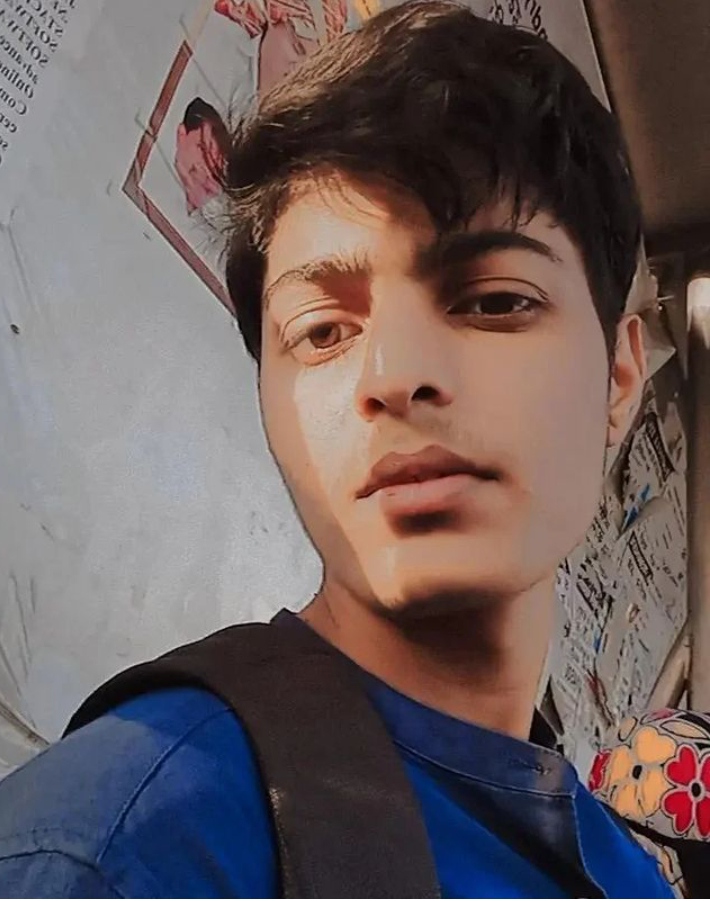