Interactive Command Line Interfaces using Inquirer.js
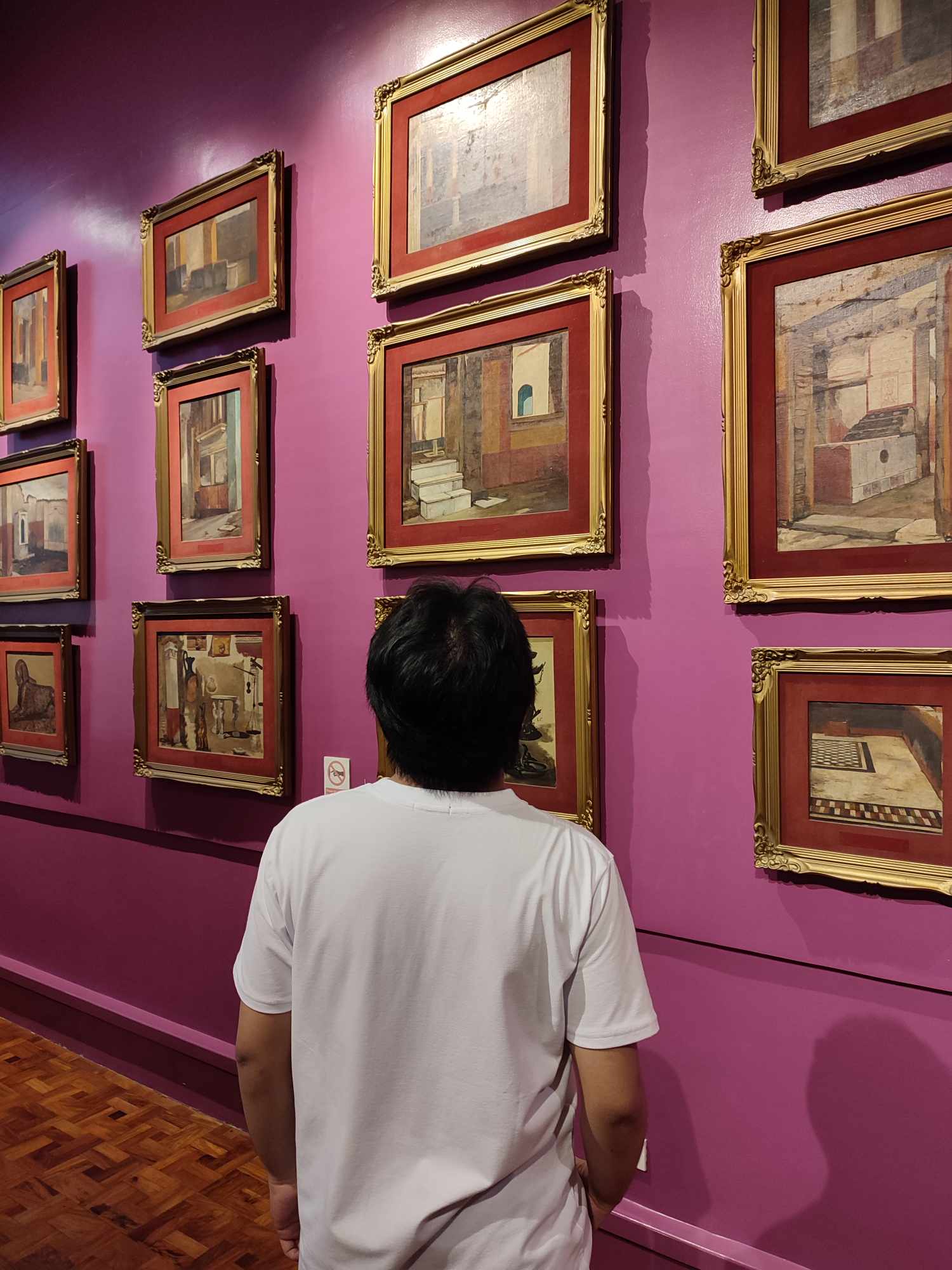
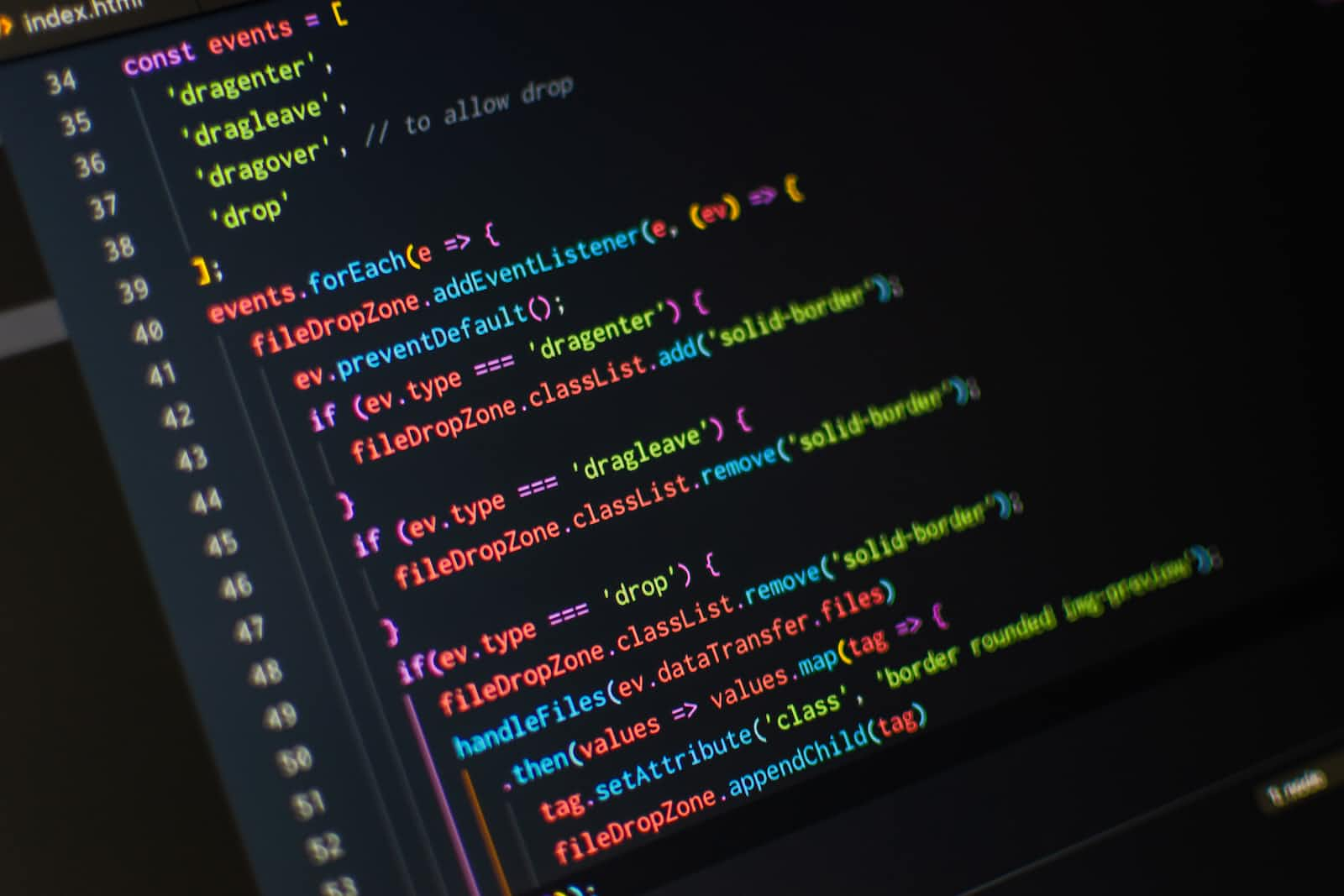
Introduction
In this blog, we will discuss how to use Node.js to enable us to create console-based applications with the help of Inquirer.js, a third-party package library.
Node.js has also a built-in module (readline
) which provides functionality to accept user input in the console, but the main difference is that Inquirer.js
gives you more flexibility to provide appropriate questions such as:
Instead of asking a user to type about their favorite programming languages repeatedly, you can use a checkbox.
Instead of asking a user to type their password and letting it be displayed on the screen, you can use a password prompt type to mask the input and give that kind of
password
functionality.
As you can see, by having that kind of feature and functionalities, we can also build a console-based app and many more.
Without further due, let's get started!
Prerequisites โ
๐๐ป Installed Node.js in your local machine.
๐๐ป Basic Knowledge of JavaScript and Node.js.
๐๐ป Basic knowledge of NPM.
๐๐ป Inquirer.js package.
What you will learn ๐ง
Built-in prompt() function from Inquirer.js.
Basic Prompt or Question Types.
What is Inquirer.js? ๐ป
๐๐ป Inquirer.js is a third-party package that enables us to do console applications.
1๏ธโฃ First Step
๐๐ป Make sure you have installed Node.js on your personal computer.
2๏ธโฃ Second Step (Initialize a package.json)
๐๐ป Open your terminal and initialize a package.json file in your project by running this npm command:
npm init
or
npm init -y
3๏ธโฃ Third Step (Installing the Inquirer.js)
npm install inquirer
4๏ธโฃ Fourth Step (Setting it up)
๐๐ป import the package on your file using CommonJS module
const inquirer = require('inquirer');
Here's the basic structure of setting up the inquirer:
inquirer.prompt(questions).then(answers => {
});
prompt()
function needs an Array of Questions and it returns a promise since it is an asynchronous function.questions
is an array containing all of the question objects.answer
is an object containing all of the answers for every prompt/question.
Example:
Here's the basic structure of thequestions
array.
const questions = [
{
type: typeOfPrompt,
name: nameOfPrompt,
message: textToBePrompted
},
]
For each question
object, we need to provide the first 3 required properties:
type
property
- It is the type of prompt or question to be displayed on the console.
name
property
- Since the
answer
is an object, it is the key for every question that is going to be stored in theanswer
object.
message
property
- It is the message to be prompted on the screen.
Question Types โ
I will only tackle the basic or core question types in Inquirer.js, so that it can help you to get started with it.
typeinput
Use this type if you want to accept data as plain text.
The value of this prompt is a string.
Example:
const questions = [
{
type: 'input',
name: 'given-name',
message: 'Enter your given name: '
},
]
// inquirer
inquirer.prompt(questions)
.then(answers => {
console.log(answers);
});
Output:
typepassword
In some cases, you want to accept a password from the user, and you don't want to display it while the user providing it on the console. This prompt provides that kind of functionality.
The value of this prompt is a string.
Let's add it to our questions
array:
const questions = [
{
type: 'input',
name: 'given-name',
message: 'Enter your given name: '
},
{
type: 'password',
name: 'password',
message: 'Enter your password: ',
mask: true
}
]
// inquirer
inquirer.prompt(questions)
.then(answers => {
console.log(answers);
})
The
mask
property is needed to hide the input.
Here's the output of it:
type list
and rawlist
list
and rawlist
helps you to create questions with choices. An example of that is if you want to create a console-based quiz app, and those two are a great fit for that.
Don't get tricked by the value of
list
andrawlist
on theanswers
object. Since these two only accept one input, the value of it in theanswers
object is still in a type ofstring
.
Here's an example of the list
and rawlist
type:
const questions = [
{
type: 'input',
name: 'given-name',
message: 'Enter your given name: '
},
{
type: 'password',
name: 'password',
message: 'Enter your password: ',
mask: true
},
{
type: 'list', // or type: 'rawlist'
name: 'favorite-color',
message: 'Your favorite color: ',
choices: ['Blue', 'Red', 'Green']
},
]
Here's thelist
type
Here's therawlist
type
As you can see the difference between the two is that the
rawlist
type is numbered and it can also accept input from a range of the choices such as 1, 2, or 3.
Here's the output:
typecheckbox
If you want your user to select multiple values, let's say their favorite programming languages, checkbox
will become handy.
It accepts multiple inputs, and the value of it is in
array
type.
Here's an example of using the checkbox
prompt:
const questions = [
// input type
{
type: 'input',
name: 'given-name',
message: 'Enter your given name: '
},
// password type
{
type: 'password',
name: 'password',
message: 'Enter your password: ',
mask: true
},
// rawlist type
{
type: 'rawlist',
name: 'favorite-color',
message: 'Your favorite color: ',
choices: ['Blue', 'Red', 'Green']
},
// checkbox type
{
type: 'checkbox',
name: 'fave-prog-language',
message: 'Favorite Programming Languages: ',
choices: ['Python', 'Java', 'JavaScript']
},
]
Similar to other list types, the checkbox also needs to have a
choices
property.
Here's the output of it:
The result will be saved in an array because the user can select multiple choices.
That's all! These are some of the basic Inquirer.js prompt/question types! ๐
You've reached the end! ๐๐๐
To sum up, with Inquirer.js, we can also do a console-based app in Node.js, and unlike other console-based support APIs from other programming languages, it gives us flexible UI-like prompts.
There are other prompt types that I haven't covered in this article, but with this, you are given a head start to further explore Inquirer.js. I hope you have learned from this post and might share this also to help other developers who are also starting to delve into the world of Node.js
References
You can check other advanced prompt types and other plugins in their documentation.
If you are new to Node.js, this documentation will help you to understand some of the core fundamentals of it.
Socials
Subscribe to my newsletter
Read articles from Nads directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
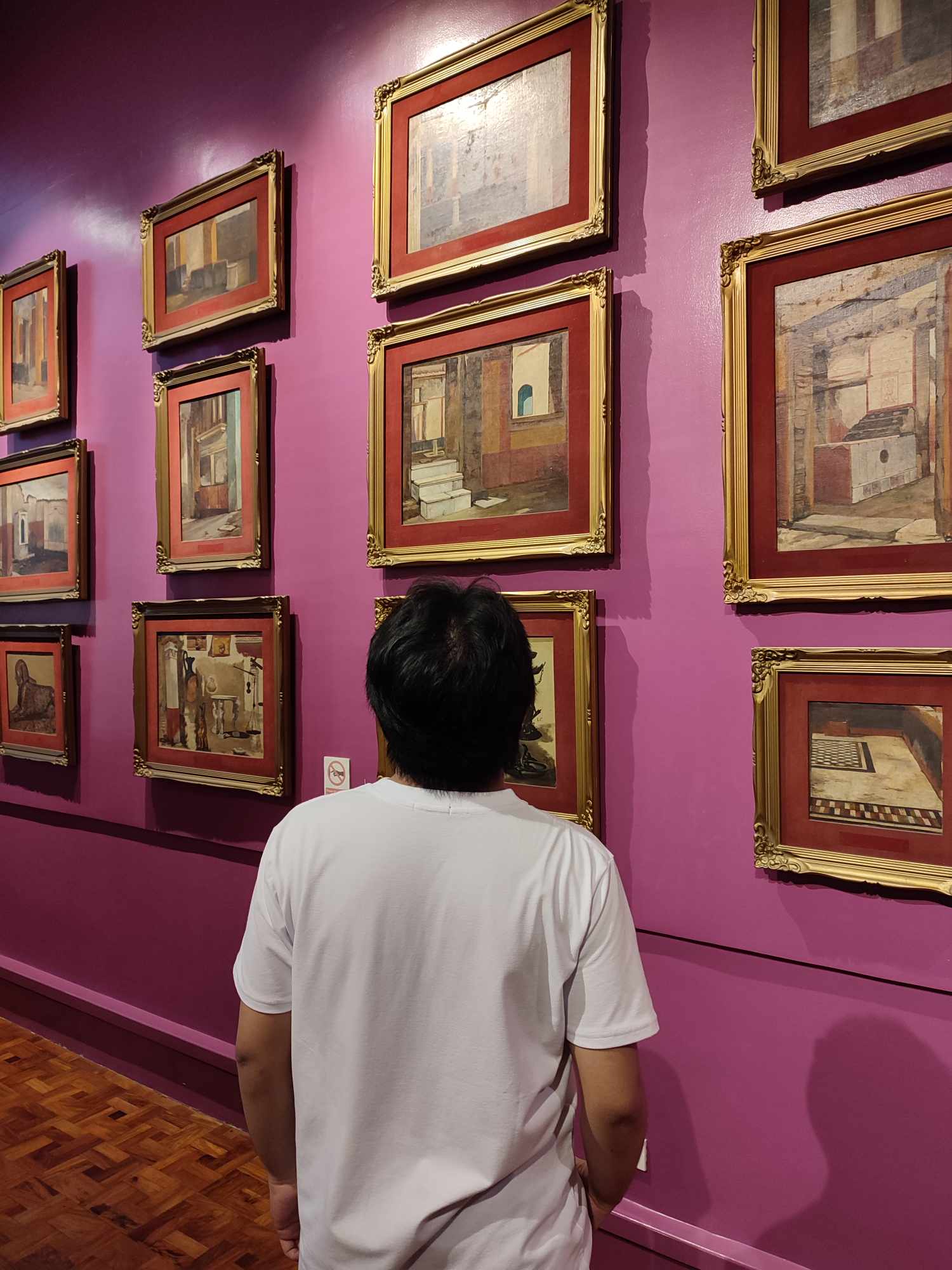
Nads
Nads
Hi! I'm Adrian, a Software Engineer specialized in Web Development. I want to share my experiences, knowledge, and expertise with you through my blogs. Feel free to message me if you have any questions regarding tech-related topics!