Best practices for integrating React with GraphQL

Table of contents
- Introduction
- Understanding React and GraphQL
- Example:
- Benefits of Integrating React with GraphQL
- Setting Up React and GraphQL
- Writing GraphQL Queries
- Mutations and Data Manipulation
- Handling GraphQL Errors
- Caching and Optimizing Performance
- Securing GraphQL Endpoints
- Testing and Debugging
- Scaling and Deployment
- Conclusion
- FAQs
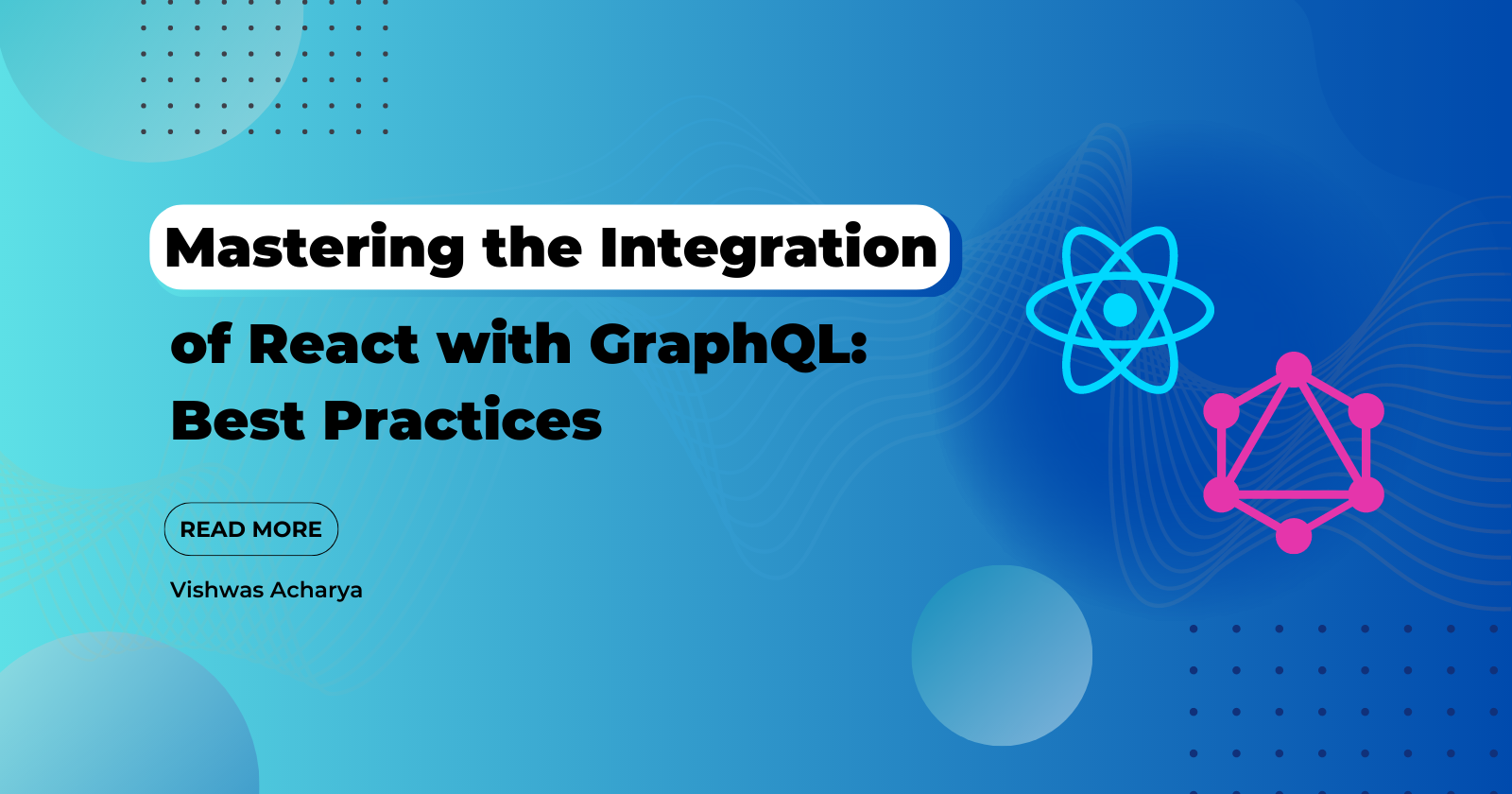
Introduction
In today's fast-paced web development landscape, React and GraphQL have emerged as powerful technologies that can greatly enhance the efficiency and performance of web applications. React, a popular JavaScript library for building user interfaces, and GraphQL, a query language for APIs, work seamlessly together to provide a flexible and efficient approach to data fetching and manipulation. This article explores the best practices for integrating React with GraphQL, enabling developers to leverage the benefits of both technologies and build robust applications.
Understanding React and GraphQL
Before diving into the integration practices, it's essential to have a clear understanding of React and GraphQL.
What is React?
React is a JavaScript library developed by Facebook for building interactive user interfaces. It allows developers to create reusable UI components and efficiently manage the application state, resulting in highly modular and maintainable codebases.
What is GraphQL?
GraphQL is an open-source query language and runtime that enables efficient data fetching and manipulation. It provides a flexible and intuitive syntax for querying APIs, allowing clients to request only the data they need and eliminating the problem of over-fetching.
Example:
Now Let's dive right into the example:
First, make sure you have the necessary dependencies installed. You will need
react
,react-dom
,@apollo/client
, andgraphql
.Set up your GraphQL server or use an existing GraphQL API endpoint.
In your React application, install the Apollo Client library by running the following command:
npm install @apollo/client graphql
- Create a new file called
ApolloProvider.js
to configure Apollo Client and provide it to your React application:
import React from 'react';
import { ApolloClient, InMemoryCache, ApolloProvider } from '@apollo/client';
const client = new ApolloClient({
uri: 'http://your-graphql-api-endpoint', // Replace with your GraphQL API endpoint
cache: new InMemoryCache(),
});
const ApolloAppProvider = ({ children }) => {
return <ApolloProvider client={client}>{children}</ApolloProvider>;
};
export default ApolloAppProvider;
- In your root
App.js
file, wrap your React application with theApolloAppProvider
component:
import React from 'react';
import ApolloAppProvider from './ApolloProvider';
const App = () => {
return (
<ApolloAppProvider>
{/* Your React application components */}
</ApolloAppProvider>
);
};
export default App;
- Now, you can start using GraphQL queries and mutations in your React components. Here's an example of fetching data using a GraphQL query:
import React from 'react';
import { gql, useQuery } from '@apollo/client';
const GET_USERS = gql`
query GetUsers {
users {
id
name
email
}
}
`;
const UserList = () => {
const { loading, error, data } = useQuery(GET_USERS);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error :(</p>;
return (
<ul>
{data.users.map((user) => (
<li key={user.id}>
{user.name} - {user.email}
</li>
))}
</ul>
);
};
export default UserList;
- In your React component where you want to display the list of users, import the
UserList
component and render it:
import React from 'react';
import UserList from './UserList';
const Home = () => {
return (
<div>
<h1>User List</h1>
<UserList />
</div>
);
};
export default Home;
That's it! You have successfully integrated a React application with GraphQL using Apollo Client. You can now fetch data from your GraphQL API and use it in your React components. Remember to replace the GraphQL API endpoint in the ApolloProvider.js
file with your actual endpoint.
Now Let's Dive into the theory for a deep understanding of this concept.
Benefits of Integrating React with GraphQL
Integrating React with GraphQL brings several benefits to the development process and application performance.
Efficient Data Fetching
By leveraging GraphQL's query optimization and selective data fetching capabilities, React applications can efficiently retrieve only the required data from the server, reducing unnecessary network overhead and improving performance.
Strong Typing and Validation
GraphQL's type system ensures that both the client and server have a shared understanding of the data schema. This enables early detection of data-related issues and provides a robust validation mechanism, reducing the chances of runtime errors.
Reduced Overfetching
One common issue with RESTful APIs is over-fetching, where the server sends more data than the client needs. GraphQL eliminates this problem by allowing clients to specify exactly what data they require, leading to more efficient network utilization.
Setting Up React and GraphQL
To start integrating React with GraphQL, certain steps need to be followed.
Installing Dependencies
First, ensure that the required dependencies, such as React and GraphQL libraries, are installed in the project. These dependencies can be easily added using package managers like npm or yarn.
Configuring GraphQL Server
Next, set up a GraphQL server to handle incoming queries and mutations. Tools like Apollo Server or Express GraphQL provide simple and powerful ways to configure and run a GraphQL server.
Creating React Components
Once the server is set up, create React components that will interact with the GraphQL server. These components can be functional or class-based, depending on the project requirements.
Writing GraphQL Queries
GraphQL queries are the primary means of fetching data from the server. Understanding the syntax and structure of GraphQL queries is crucial for effective integration with React.
Query Syntax and Structure
GraphQL queries consist of fields and arguments, allowing clients to specify the desired data and any necessary parameters. By structuring queries efficiently, developers can optimize data fetching and avoid unnecessary round trips to the server.
Querying Data in React
To execute GraphQL queries in React, utilize tools like Apollo Client, which seamlessly integrates with React components. Apollo Client provides hooks and components that simplify the process of fetching and managing GraphQL data within React.
Mutations and Data Manipulation
Apart from data fetching, GraphQL enables developers to perform mutations and manipulate data on the server. This allows for seamless data updates and modifications in React applications.
Modifying Data with Mutations
GraphQL mutations are used to modify data on the server. They follow a similar syntax to queries and can be executed from React components using Apollo Client.
Updating React Components
When a mutation modifies the data on the server, it's crucial to update the affected React components to reflect the changes. By utilizing React's state management capabilities, developers can ensure that the UI remains synchronized with the server-side data.
Handling GraphQL Errors
Error handling is an integral part of any application. When integrating React with GraphQL, it's essential to implement effective error-handling mechanisms.
Error Handling Best Practices
Implementing proper error-handling practices in GraphQL involves returning specific error messages and status codes from the server. This enables React components to display meaningful error messages and guide users through the troubleshooting process.
Displaying Error Messages
In React, displaying error messages can be achieved by utilizing conditional rendering and error state management. By providing clear and concise error messages, developers can enhance the user experience and facilitate issue resolution.
Caching and Optimizing Performance
Caching and performance optimization are crucial considerations when integrating React with GraphQL, as they can significantly improve application speed and responsiveness.
Using Apollo Client
Apollo Client provides built-in caching capabilities that can be leveraged to store and retrieve GraphQL query results. Caching eliminates redundant network requests and improves overall application performance.
Implementing Caching Strategies
Developers can implement various caching strategies, such as time-based invalidation or manual cache updates, based on the specific requirements of their React application. These strategies ensure that the UI remains up to date with the latest data while minimizing unnecessary data fetching.
Securing GraphQL Endpoints
Securing GraphQL endpoints is essential to protect sensitive data and prevent unauthorized access.
Authentication and Authorization
Implementing authentication and authorization mechanisms, such as
JWT (JSON Web Tokens) or OAuth, ensures that only authenticated and authorized users can access the GraphQL endpoints. This safeguards sensitive data and maintains the integrity of the application.
Protecting Sensitive Data
When integrating React with GraphQL, it's crucial to identify and secure any sensitive data transmitted between the client and server. Implementing encryption and following security best practices can help mitigate potential security risks.
Testing and Debugging
Testing and debugging are crucial steps in the development process to ensure the reliability and stability of React applications integrated with GraphQL.
Unit Testing React Components
Unit tests for React components verify their behavior and ensure they function as expected. Testing libraries like Jest and React Testing Library provide tools to write comprehensive unit tests for React components.
Testing GraphQL Queries and Mutations
GraphQL queries and mutations can be thoroughly tested using tools like Apollo Client's mock provider or testing libraries specifically designed for GraphQL, such as Apollo Server Testing.
Debugging React and GraphQL
During development, debugging tools like React DevTools and GraphQL Playground can be utilized to inspect component states, and query responses, and identify potential issues or performance bottlenecks.
Scaling and Deployment
As React applications integrated with GraphQL grow in complexity, it becomes essential to scale and deploy them efficiently.
Deploying React and GraphQL
Deploying React and GraphQL applications can be done using various hosting providers or cloud platforms. Services like Vercel, Netlify, or AWS provide straightforward deployment options for React applications, while platforms like Heroku or AWS App Runner can host GraphQL servers.
Scaling for Increased Traffic
To handle increased traffic and growing user bases, developers can scale the GraphQL server horizontally by utilizing load balancers, caching mechanisms, and optimizing database queries. Additionally, implementing performance monitoring tools can help identify and address performance bottlenecks.
Conclusion
Integrating React with GraphQL offers numerous benefits for web application development. By leveraging the efficiency of GraphQL's data fetching and manipulation capabilities and the flexibility of React's UI component model, developers can create powerful and performant applications. Following the best practices outlined in this article, from setting up React and GraphQL to securing endpoints and optimizing performance, will ensure a smooth integration process and enhance the overall development experience.
FAQs
Q: How does GraphQL differ from traditional RESTful APIs?
A: GraphQL differs from RESTful APIs in several ways. Unlike REST, which often requires multiple round trips to fetch related data, GraphQL allows clients to request exactly what data they need in a single query. Additionally, GraphQL's type system provides strong typing and validation, reducing the chances of errors and improving the development experience.
Q: Can I use React without integrating it with GraphQL?
A: Yes, React can be used independently without integrating it with GraphQL. React is a versatile library that can be used for building user interfaces in various contexts, including traditional RESTful APIs or even static websites.
Q: What are some popular libraries or frameworks for integrating React with GraphQL?
A: There are several popular libraries and frameworks for integrating React with GraphQL. Apollo Client, Relay, and Urql are widely used libraries that provide powerful tools and abstractions for fetching and managing GraphQL data in React applications.
Q: Is GraphQL suitable for all types of applications?
A: GraphQL is a versatile technology that can be used in various application contexts. However, its strengths are particularly beneficial in applications with complex data requirements, frequent data updates, or a need for precise control over data fetching.
Q: How can I learn more about React and GraphQL integration?
A: To learn more about integrating React with GraphQL, you can explore online tutorials, documentation, and resources provided by React and GraphQL communities. There are also numerous courses and books available that delve into the topic in detail, allowing you to deepen your understanding and gain hands-on experience.
By Vishwas Acharya 😉
Checkout my other content as well:
YouTube:
Podcast:
Book Recommendations:
Subscribe to my newsletter
Read articles from Vishwas Acharya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vishwas Acharya
Vishwas Acharya
Embark on a journey to turn dreams into digital reality with me, your trusted Full Stack Developer extraordinaire. With a passion for crafting innovative solutions, I specialize in transforming concepts into tangible, high-performing products that leave a lasting impact. Armed with a formidable arsenal of skills including JavaScript, React.js, Node.js, and more, I'm adept at breathing life into your visions. Whether it's designing sleek websites for businesses or engineering cutting-edge tech products, I bring a blend of creativity and technical prowess to every project. I thrive on overseeing every facet of development, ensuring excellence from inception to execution. My commitment to meticulous attention to detail leaves no room for mediocrity, guaranteeing scalable, performant, and intuitive outcomes every time. Let's collaborate and unleash the power of technology to create something truly extraordinary. Your dream, my expertise—let's make magic happen! Connect with me on LinkedIn/Twitter or explore my work on GitHub.