The Factory Method Design Pattern in Java
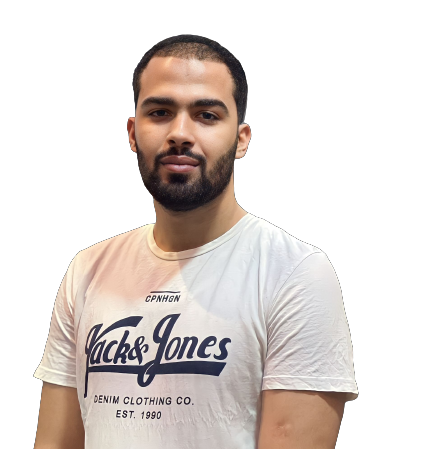
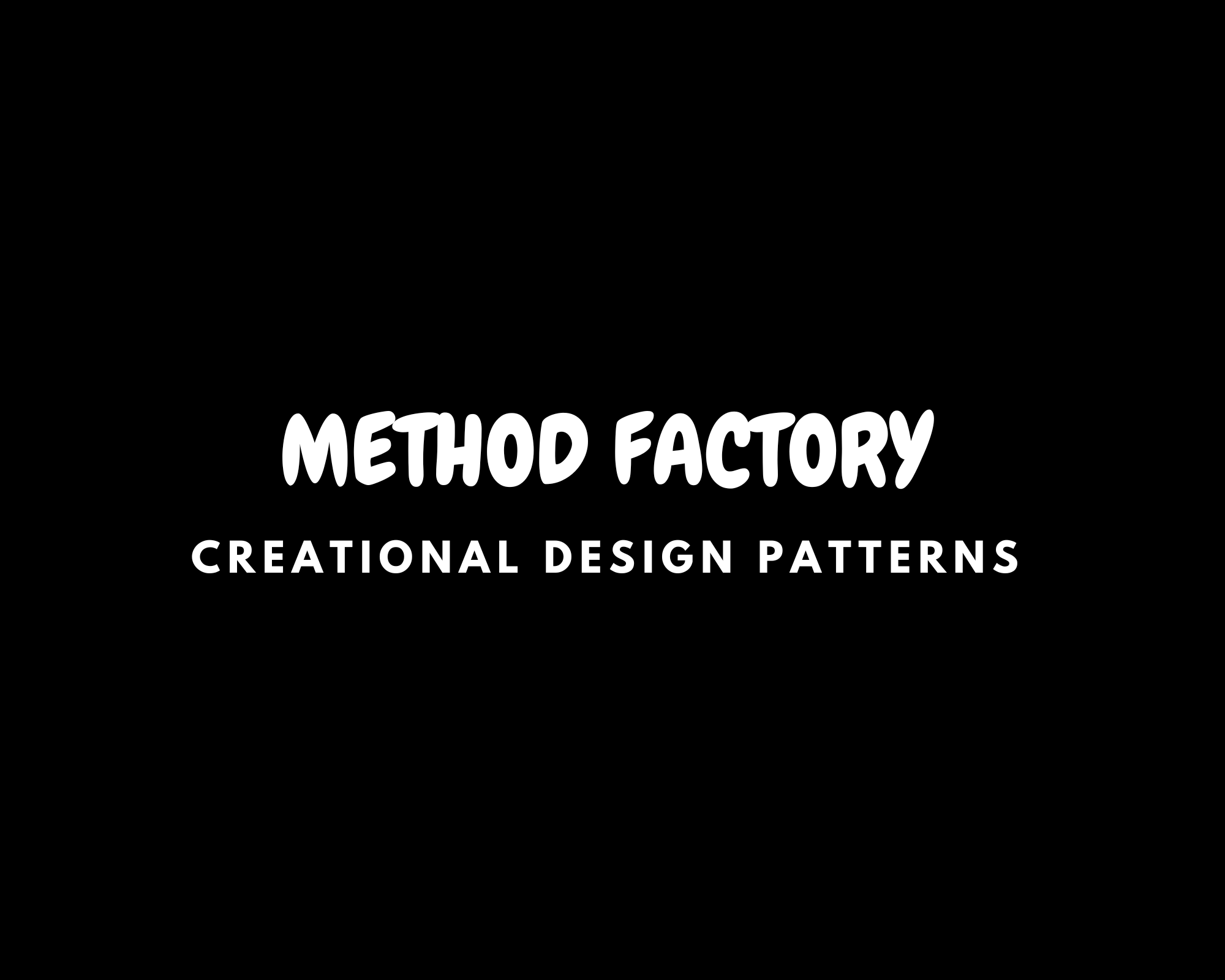
Introduction:
The Method Factory design pattern is a creational pattern that provides an interface for creating objects. It encapsulates the object creation logic within a method, allowing subclasses to decide which concrete class to instantiate. In this article, we will explore the implementation of the Method Factory pattern in Java, along with a step-by-step guide to creating a practical example.
Overview :
The Method Factory pattern promotes loose coupling between the creator and the products by introducing an abstract creator class that declares a factory method. Subclasses of the creator implement this method to create specific instances of the product. The creator class depends on abstractions, while the concrete subclasses handle the object creation details.
Key Principales:
✅ Product: This represents the abstract class or interface for the objects that the factories create.
✅ ConcreteProduct: These are the concrete implementations of the Product interface or class.
✅ Creator: The abstract class or interface that declares the factory method(s) for creating objects.
✅ ConcreteCreator: These are the concrete implementations of the Creator interface, which override the factory method(s) to produce specific ConcreteProduct instances.
- UML representation:
Example Implementation:**
Let's understand the Method Factory pattern with a simple example in Java. Suppose we have an abstract class called Vehicle, and we want to create two concrete implementations: Car and Motorcycle.
STEP 1️⃣:
STEP 2️⃣
USAGE
Why you should consider using the factory classes:
✅ Encapsulation and Abstraction: The Method Factory pattern promotes encapsulation by encapsulating the object creation logic within the factory classes. By creating objects through factory methods, you separate the client code from the specifics of object creation, providing a more abstract and higher-level interface.
✅ Loose Coupling: The pattern helps achieve loose coupling between the
client code and the concrete classes. When you directly create a Car
instance, the client code becomes tightly coupled to the Car
class. However, by using a factory, the client code only depends on the abstract VehicleFactory
or Vehicle
interface, reducing dependencies and enhancing flexibility.
✅ Code Extensibility: The Method Factory pattern allows for easy extensibility by providing a straightforward mechanism to add new concrete implementations of Vehicle
without modifying existing client code. If you decide to introduce a new type of vehicle, such as a Truck
, you can create a TruckFactory
without modifying the existing code that uses the VehicleFactory
.
✅Single Responsibility Principle: The pattern follows the Single Responsibility Principle by assigning the responsibility of object creation to the factory classes. Each factory has the sole responsibility of creating a specific type of object, making the codebase more modular and easier to maintain.
✅Testing and Mocking: Using factory classes simplifies testing and mocking in unit tests. By creating objects through factory methods, you can easily substitute real objects with mock objects during testing, allowing for more effective unit testing and isolating dependencies.
✅Centralized Configuration: Factory classes provide a centralized location for managing object creation. If, for example, you need to configure the creation of objects based on certain conditions or parameters, the factory can handle this logic, abstracting it away from the client code.
I'm Active in
Subscribe to my newsletter
Read articles from Ismail Harik directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
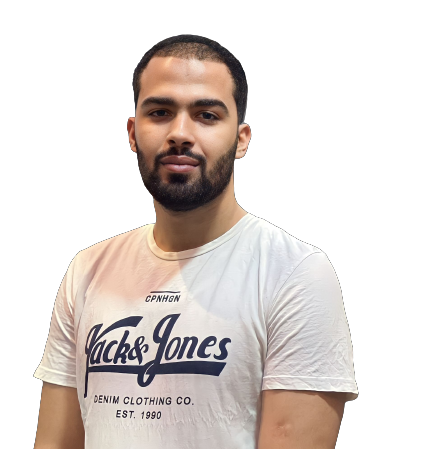
Ismail Harik
Ismail Harik
As a skilled computer science student and software developer, I have a strong passion for building innovative solutions and learning new technologies. With a solid foundation in Java programming, Spring Ecosystem, and Angular, I am well-equipped to design and develop robust and scalable applications that meet business requirements. I have hands-on experience in developing applications based on Microservices Architecture and utilizing technologies such as Spring Cloud, Docker, and Kubernetes.