Abstract Factory Design Patterns in Java: Building Modular and Extensible Software Solutions
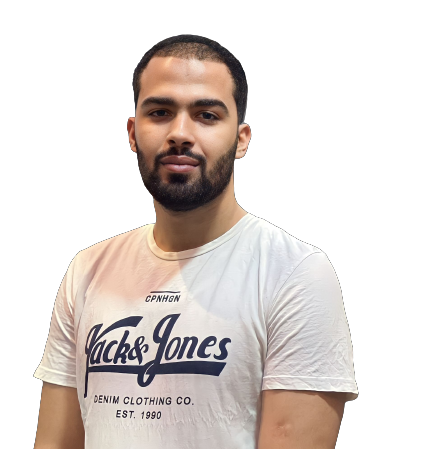
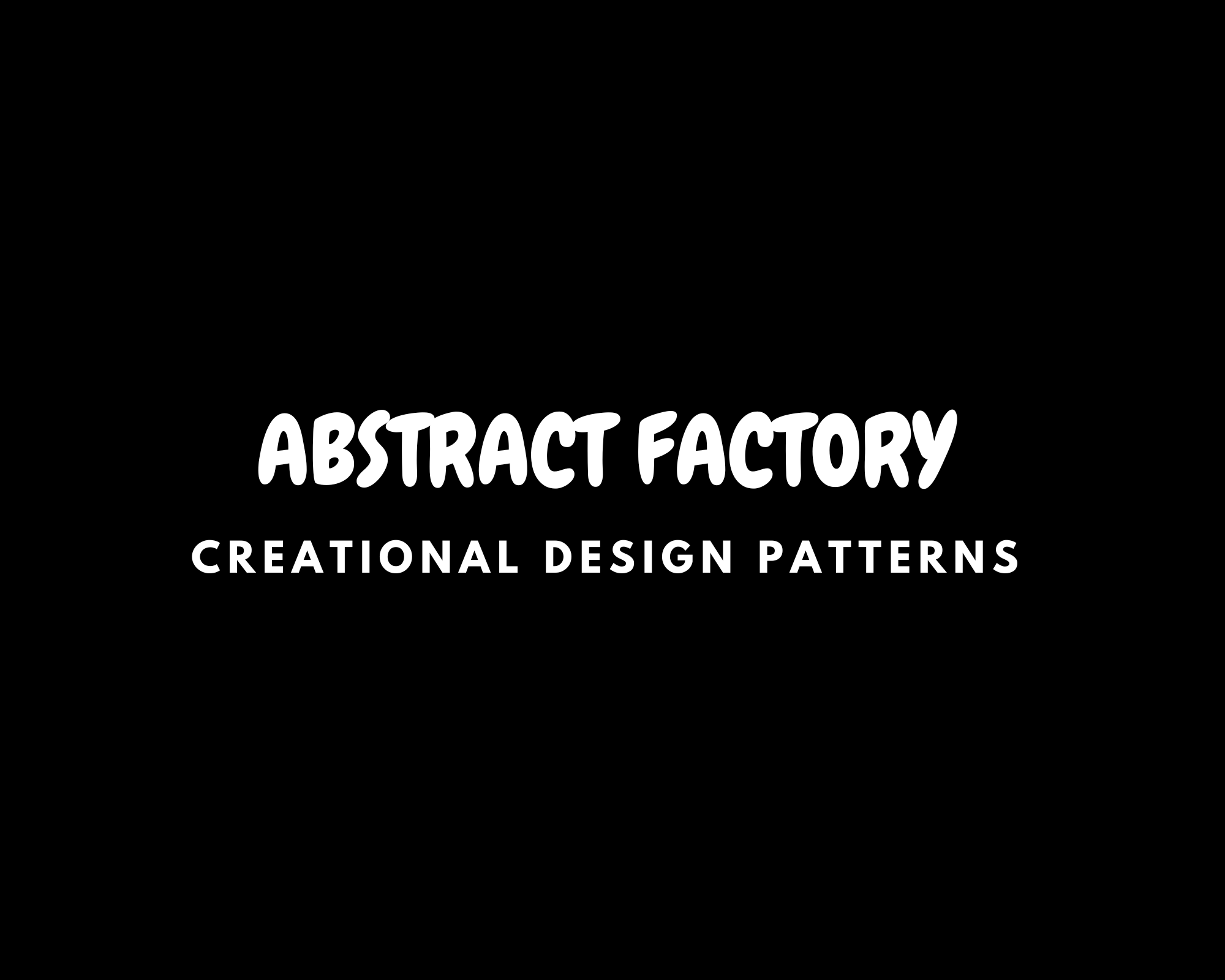
• Introduction:
In our previous article, we explored the Method Factory design pattern, which promotes loose coupling and extensibility. Now, we will enhance our example by incorporating the Abstract Factory design pattern. The Abstract Factory pattern allows us to create families of related objects such as different types of vehicles, without specifying their concrete classes, this pattern promotes the concept of "programming to an interface, not an implementation”. Let's dive into the integration of the Method Factory and Abstract Factory patterns using Java.
• Overview :
The Abstract Factory pattern extends the concepts of the Method Factory pattern by providing a way to create families of related objects. Instead of relying on a single factory method, the Abstract Factory pattern introduces an abstract factory interface or class that declares multiple factory methods for creating different types of related objects. Concrete factory implementations then provide the necessary logic to create specific objects from each family.
• Example Implementation:
Let's consider a scenario where we have two families of objects: shapes and colors. The shapes family includes Circle and Rectangle, while the colors family includes Red and Blue. We'll define an abstract factory interface, AbstractFactory, which declares the creation methods for shapes and colors. Concrete factories, ConcreteFactory1 and ConcreteFactory2, will provide specific implementations of these creation methods.
1️⃣ : define families:
2️⃣ Define abstract factory interface & Concrete factories:
The Abstract Factory design pattern offers several benefits in the code you have provided:
✅Encapsulation of object creation: The Abstract Factory pattern encapsulates the creation of related objects within a factory interface. This promotes a separation of concerns, as the client code does not need to know about the specific implementation details of creating objects. It can simply rely on the factory interface to create the desired objects.
✅Loose coupling: The client code depends on the AbstractFactory interface, which abstracts away the concrete implementations of shapes and colors. This loose coupling allows the client code to be independent of the specific classes being instantiated. It enhances flexibility and maintainability, as changes to the concrete classes can be made without affecting the client code.
✅Easy extensibility: The Abstract Factory pattern makes it easier to introduce new families of related objects. By implementing a new ConcreteFactory that conforms to the AbstractFactory interface, new sets of shapes and colors can be created without modifying the existing client code. This promotes scalability and allows for future expansions and modifications without breaking existing code.
✅Code reusability: The Abstract Factory pattern promotes code reusability by encapsulating the creation logic within the factory classes. Different client code modules can use the same AbstractFactory interface to create objects, facilitating code reuse across multiple parts of the application.
✅Simplified client code: The client code becomes simpler and more focused on its core functionality. It delegates the responsibility of object creation to the AbstractFactory and does not need to worry about the specific instantiation details. This leads to cleaner and more maintainable client code.
• Conclusion:
By integrating the Method Factory and Abstract Factory patterns, we enhance our software design by promoting loose coupling, extensibility, and the ability to create families of related objects. The Method Factory pattern handles the creation of individual objects, while the Abstract Factory pattern extends this concept to create families of related objects. This combination provides a powerful and flexible solution for object creation in Java and can be applied to various scenarios in software development.
I'm Active in
Subscribe to my newsletter
Read articles from Ismail Harik directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
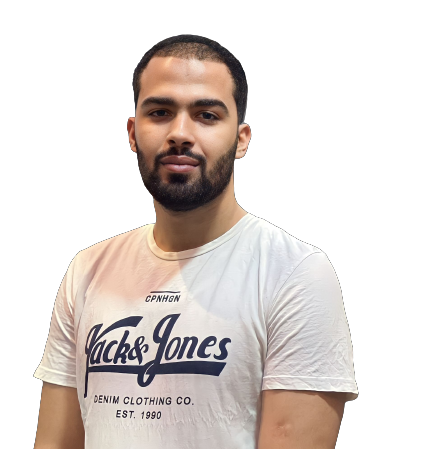
Ismail Harik
Ismail Harik
As a skilled computer science student and software developer, I have a strong passion for building innovative solutions and learning new technologies. With a solid foundation in Java programming, Spring Ecosystem, and Angular, I am well-equipped to design and develop robust and scalable applications that meet business requirements. I have hands-on experience in developing applications based on Microservices Architecture and utilizing technologies such as Spring Cloud, Docker, and Kubernetes.