Day 8 Of #30DaysOfJavaScript
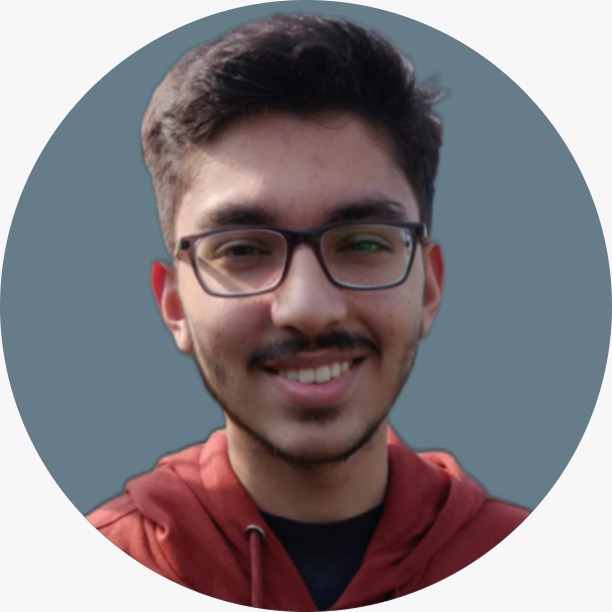
Table of contents
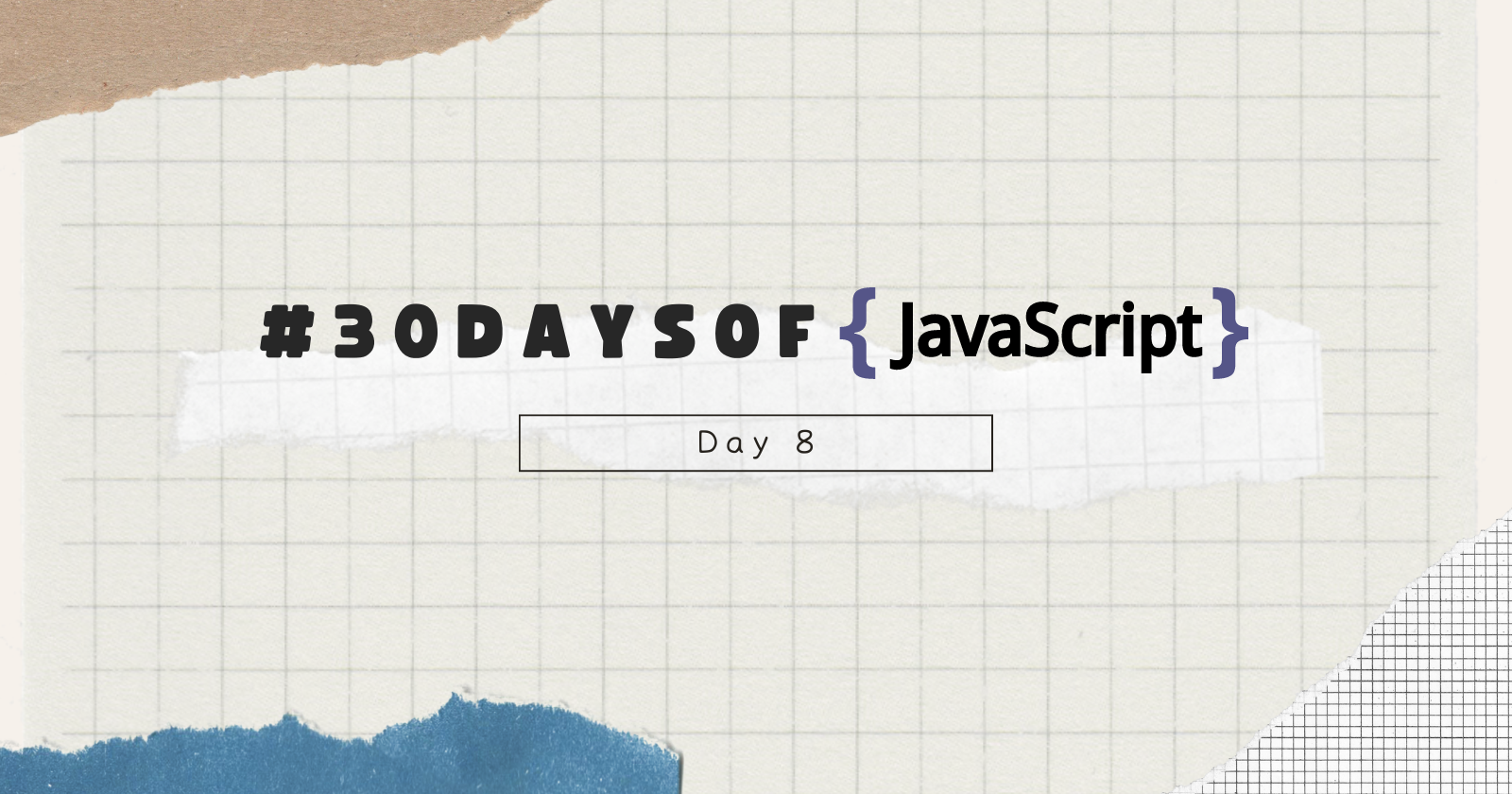
Introduction
Hey folks, I hope that you must have read my Day 7 Article on #30DaysOfJs. In case, if you haven't then make sure you give it a read by clicking here.
Scope
A variable is a fundamental part of JavaScript. We declare variables to store different data types. To declare a variable we use the keywords var, let and const. A variable can be declared at different scopes. In this section, we will see the scope variables, the scope of variables when we use var or let. Variables scopes can be:
Global
Local
These variables can be declared global or local scope. Anything declared without var, let, or const is scoped at the global level.
- Window Global Object: Without using console.log() open your browser and check, you will see the value of a and b. If you write a or b on the browser. That means a and b are already available in the window.
a = 'JavaScript' // Declaring a variable without let or const make it available in window object and this found anywhere.
b = 10 // This is a global scope variable and found in the window object.
function letsLearnScope() {
console.log(a, b);
if (true) {
console.log(a, b);
}
}
console.log(a, b); // Accessible.
- Global Scope: A globally declared variable can be accessed everywhere in the same file. But the term global is relative. It can be global to the file or it can be global relative to some block of codes.
let a = 'JavaScript' // Is a global scope it will be found anywhere in this file.
let b = 10 // Is a global scope it will be found anywhere in this file.
function letsLearnScope() {
console.log(a, b); // JavaScript 10, accessible.
if (true) {
let a = 'Python'
let b = 100
console.log(a, b); // Python 100.
}
console.log(a, b);
}
letsLearnScope()
console.log(a, b); // JavaScript 10, accessible.
- Local Scope: A variable declared as local can be accessed only in certain block codes.
Block Scope
Function Scope
let a = 'JavaScript' // Is a global scope it will be found anywhere in this file.
let b = 10 // Is a global scope it will be found anywhere in this file.
// Function scope.
function letsLearnScope() {
console.log(a, b); // JavaScript 10, accessible.
let value = false
// Block scope.
if (true) {
// We can access from the function and outside the function but
// variables declared inside the if will not be accessed outside the if block.
let a = 'Python'
let b = 20
let c = 30
let d = 40
value = !value
console.log(a, b, c, value); // Python 20 30 true.
}
// We can not access c because c's scope is only the if block.
console.log(a, b, value); // JavaScript 10 true.
}
letsLearnScope()
console.log(a, b); // JavaScript 10, accessible.
Now, you have an understanding of the scope. A variable declared with var only scoped to function but a variable declared with let or const is block scope(function block, if block, loop block, etc). Block in JavaScript is a code in between two curly brackets ({}).
function letsLearnScope() {
var gravity = 9.81
console.log(gravity);
}
// console.log(gravity), Uncaught ReferenceError: gravity is not defined.
if (true){
var gravity = 9.81
console.log(gravity); // 9.81
}
console.log(gravity); // 9.81
for(var i = 0; i < 3; i++){
console.log(i); // 0, 1, 2
}
console.log(i); // 3
In the below example, we'll use let instead of the var keyword.
function letsLearnScope() {
// You can use let or const, but gravity is constant I prefer to use const.
const gravity = 9.81
console.log(gravity)
}
// console.log(gravity), Uncaught ReferenceError: gravity is not defined.
if (true){
const gravity = 9.81
console.log(gravity) // 9.81
}
// console.log(gravity), Uncaught ReferenceError: gravity is not defined.
for(let i = 0; i < 3; i++){
console.log(i) // 0, 1, 2
}
// console.log(i), Uncaught ReferenceError: i is not defined.
The scope let and const are the same. The difference is only that we can not change or reassign the value of the const
variable. I would strongly recommend you use let and const, by using them one can write clean code and avoid mistakes.
Object
Objects have different properties in a key-value pair relationship. To create an object literal, we use two curly brackets.
Syntax:
const person = {}
Creating an Object With Values: Now, let us say that the person object has firstName, lastName, age, location, skills and isMarried properties. The value of properties or keys could be a string, number, boolean, null, undefined, or anything else.
Let us see some examples of objects. Each key has a value in the object.
const person = {
firstName: 'Shivank',
lastName: 'Kapur',
age: 18,
country: 'India',
city: 'New Delhi',
skills: [
'HTML',
'CSS',
'JavaScript',
'React',
'Node',
'MongoDB',
],
isMarried: false
}
console.log(person);
- Getting Values From an Object: The values inside an object can be accessed by the following methods:
- Dot Method:
const person = {
firstName: 'Shivank',
lastName: 'Kapur',
age: 18,
country: 'India',
city: 'New Delhi',
skills: [
'HTML',
'CSS',
'JavaScript',
'React',
'Node',
'MongoDB',
],
getFullName: function() {
return `${this.firstName}${this.lastName}`
},
'phone number': '+91xxxxxxxxxx'
}
// Accessing values using . (dot method)
console.log(person.firstName);
console.log(person.lastName);
console.log(person.age);
console.log(person.location); // undefined
- Square Bracket Method:
const person = {
firstName: 'Shivank',
lastName: 'Kapur',
age: 18,
country: 'India',
city: 'New Delhi',
skills: [
'HTML',
'CSS',
'JavaScript',
'React',
'Node',
'MongoDB',
],
getFullName: function() {
return `${this.firstName}${this.lastName}`
},
'phone number': '+91xxxxxxxxxx'
}
// Value can be accessed using square bracket and key name.
console.log(person['firstName']);
console.log(person['lastName']);
console.log(person['age']);
console.log(person['age']);
console.log(person['location']); // undefined
// For instance to access the phone number we'll only use the square bracket method.
console.log(person['phone number']);
- Creating Object Methods: getFullName is a function inside the person object and we call it in an object method. this keyword refers to the object itself. We can use it to access the values of different properties of the object.
For Example:
const person = {
firstName: 'Shivank',
lastName: 'Kapur',
age: 18,
country: 'India',
city: 'New Delhi',
skills: [
'HTML',
'CSS',
'JavaScript',
'React',
'Node',
'MongoDB',
],
getFullName: function() {
return `${this.firstName}${this.lastName}`
}
}
console.log(person.getFullName());
// Shivank Kapur
- Setting New Key For an Object: We can easily modify the Data inside an Object.
const person = {
firstName: 'Shivank',
lastName: 'Kapur',
age: 18,
country: 'India',
city: 'New Delhi',
skills: [
'HTML',
'CSS',
'JavaScript',
'React',
'Node',
'MongoDB',
],
getFullName: function() {
return `${this.firstName}${this.lastName}`
}
}
person.nationality = 'Indian'
person.country = 'India'
person.title = 'Student'
person.skills.push('Coding')
person.skills.push('Editing')
person.isMarried = false
person.getPersonInfo = function() {
let skillsWithoutLastSkill = this.skills
.splice(0, this.skills.length - 1)
.join(', ')
let lastSkill = this.skills.splice(this.skills.length - 1)[0]
let skills = `${skillsWithoutLastSkill}, and ${lastSkill}`
let fullName = this.getFullName()
let statement = `${fullName} is a ${this.title}.\nHe lives in ${this.country}.\nHe teaches ${skills}.`
return statement
}
console.log(person);
console.log(person.getPersonInfo());
There are different ways to manipulate an Object. Some of them are mentioned below:
- Object.assign: To copy an object without modifying the original object.
For Example:
const person = {
firstName: 'Shivank',
age: 18,
country: 'India',
city:'New Delhi',
skills: ['HTML', 'CSS', 'JS'],
title: 'Student',
address: {
street: 'xxxxx',
pobox: 2004,
city: 'xxxxx'
},
getPersonInfo: function() {
return `I am ${this.firstName} and I live in ${this.city}, ${this.country}. I am ${this.age}.`
}
}
// Object methods: Object.assign, Object.keys, Object.values, Object.entries.
const copyPerson = Object.assign({}, person);
console.log(copyPerson);
- Object.keys: To get the keys of an object as an array.
For Example:
const keys = Object.keys(copyPerson);
console.log(keys);
const address = Object.keys(copyPerson.address)
console.log(address);
- Object.values: To get the values of an object as an array.
For Example:
const values = Object.values(copyPerson);
console.log(values);
- Object.entries: To get the keys and values in an array.
For Example:
const entries = Object.entries(copyPerson);
console.log(entries);
I hope that you must have found this article quite helpful. If yes, then do give a read to some of my other articles!
Who knows you might become a great programmer ๐ค!
Subscribe to my newsletter
Read articles from Shivank Kapur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
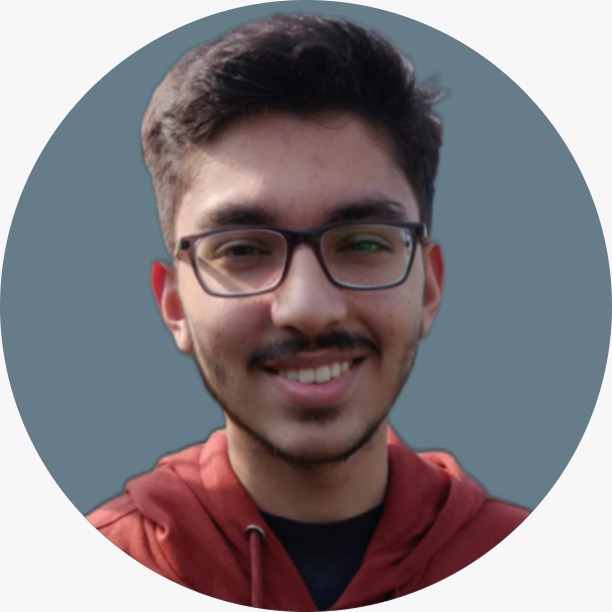
Shivank Kapur
Shivank Kapur
I'm a FullStack Developer currently working with Technologies like MERN Stack, BlockChain, GraphQL, Next.js, LLMs, and a plethora of other cutting-edge tools. I'm working as a Developer Relations Engineering intern at Router Protocol and also as a Contributor at SuperteamDAO. My journey also encompasses past experiences at Push Protocol, where I orchestrated seamless community management strategies. I help Communities by Bridging the Gap between Developers and Clients. Crafting, launching, and meticulously documenting products fuel my passion, infusing every endeavor with purpose and precision. Beyond the binary, I find solace in the pages of self-help and spirituality, honing both mind and spirit with each turn of the page. phewww... I love a cup of coffee โ while writing code. So let's connect and have a meet over something you wanna discuss. I'll be more than happy to have it. Drop me a line at shivankkapur2004@gmail.com