Understanding the 'this' Keyword in JavaScript: A Guide to Execution Context and Binding Rules
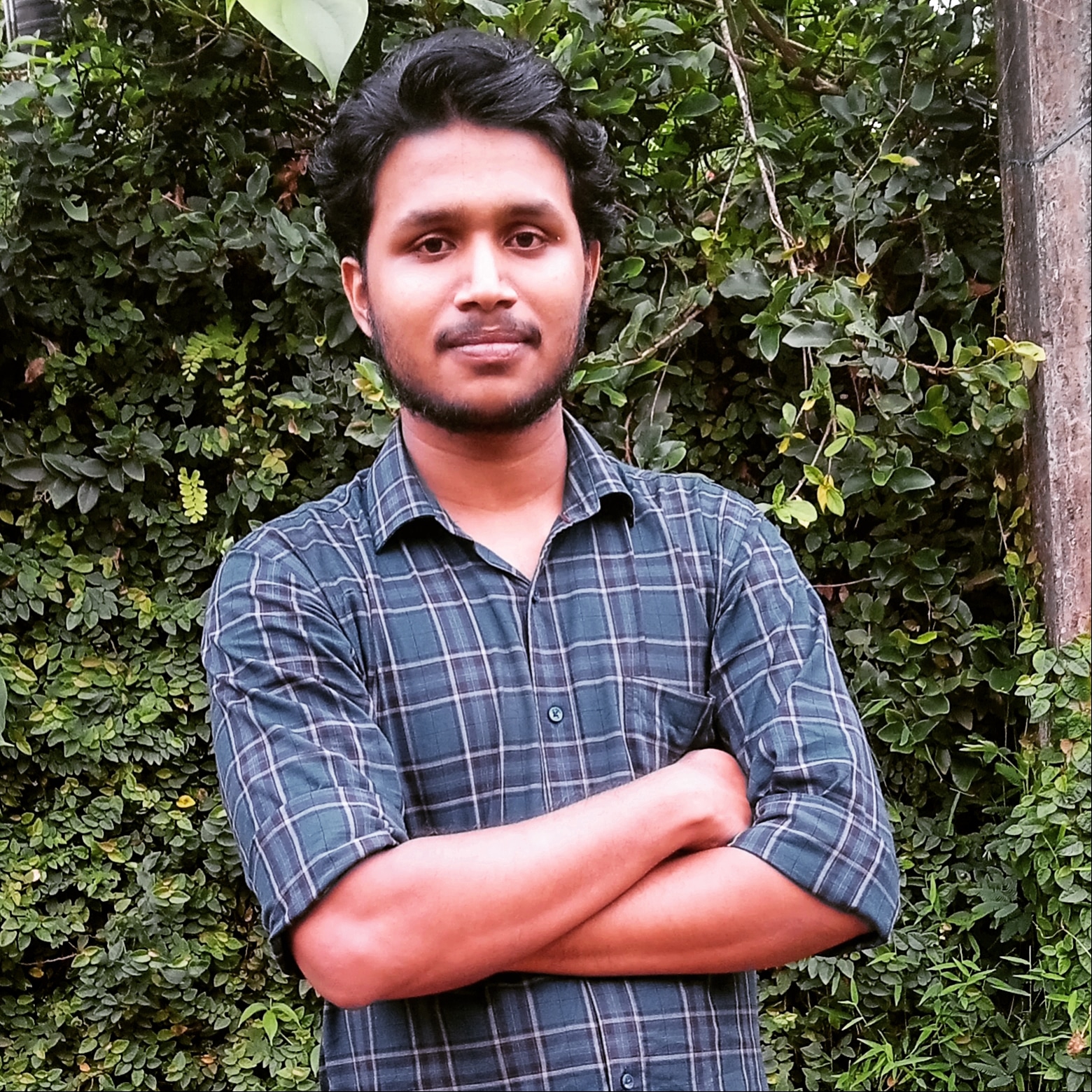
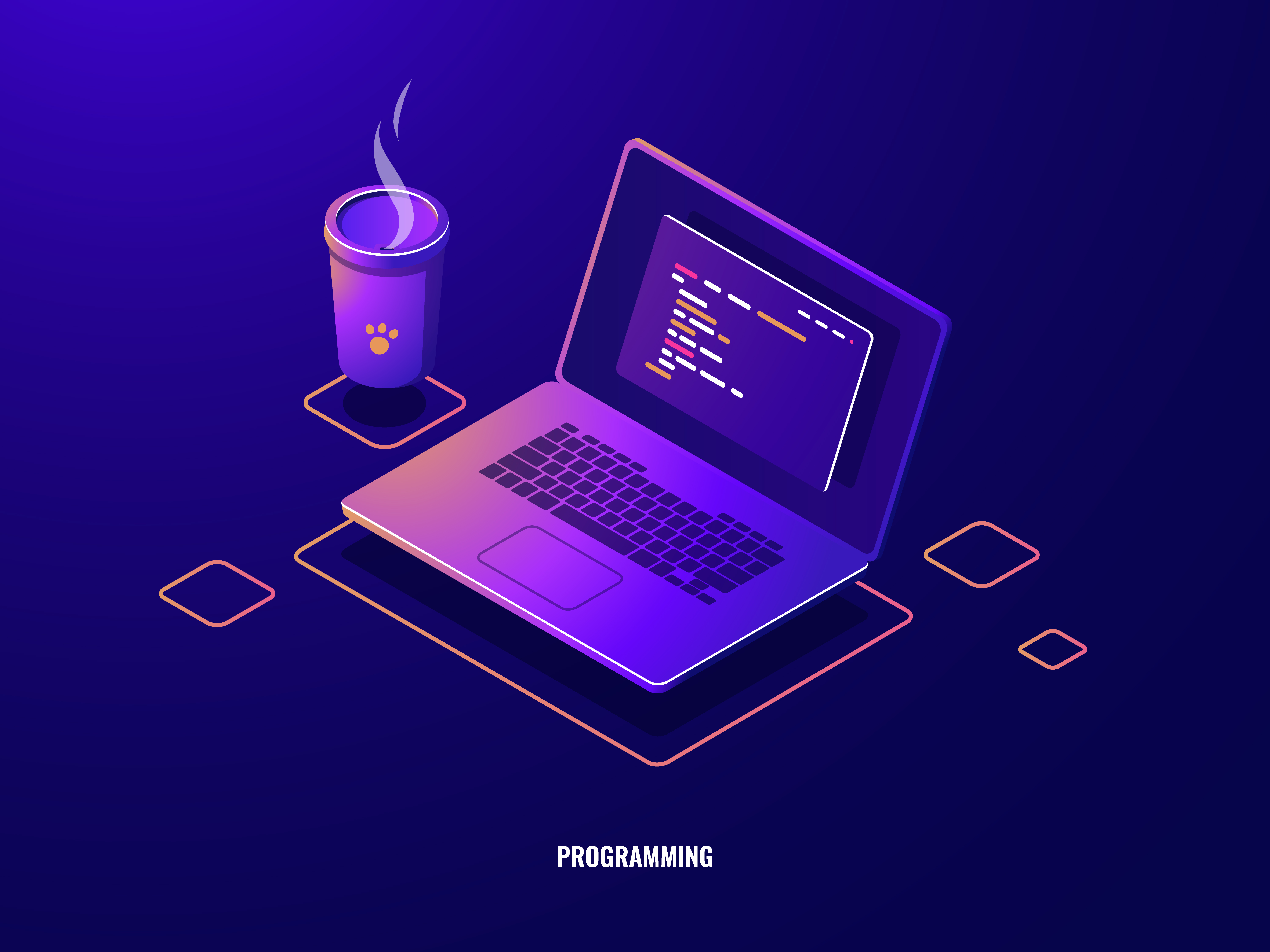
Introduction
In JavaScript, the 'this' keyword plays a crucial role in determining the context in which a function is executed or an object is accessed. It serves as a reference to an object and its value depends on the specific call-site or invocation context. Additionally, the concept of execution context encompasses the code that is currently running and all the elements required to execute it smoothly. In this blog, we will explore the execution context, delve into the intricacies of the 'this' keyword, and examine the various binding rules that govern its behavior.
Execution Context: The Foundation of Code Execution At its core, an execution context represents the environment in which a piece of code is executed. It includes the current code being executed and the surrounding state that helps run it. Think of it as a mini Lexical Environment where the code operates, holding variables, functions, and other resources necessary for execution.
The 'this' Keyword: An Invocation Context The 'this' keyword serves as a reference to an object within a function, and its value is determined by the call-site or invocation context. It encapsulates the object on which a function is invoked and enables access to its properties and methods. Consequently, 'this' is often referred to as an "Invocation Context" since it signifies the context of the function invocation.
Binding Rules: For establishing the Value of 'this' understanding how 'this' is bound to an object is crucial. Let's explore the different binding rules:
Implicit Binding: When a function is invoked from an object, 'this' is bound to that object. It allows the function to access and manipulate the object's properties and methods directly. From the example given below, 'foo' is invoked from 'obj' so 'this' is bound to 'obj'.
function foo() { console.log( this.a ); } var obj = { a: 3, foo: foo }; obj.foo(); // 3
Explicit Binding: By using methods like 'call()', 'apply()', or 'bind()', we can explicitly set the execution context for a function. 'call()' and 'apply()' immediately invoke the function with the specified context, while 'bind()' creates a new function with a permanently bound context.
function greet() { console.log(Hello, ${this.name}!); } const person = { name: 'Alice' }; greet.call(person); // Output: Hello, Alice!
New Binding: When a function is used as a constructor with the 'new' keyword, 'this' refers to the newly created instance of the object. It allows the constructor function to initialize the object's properties.
function foo(a) { this.a = a; } var bar = new foo( 2 ); console.log( bar.a ); // 2
Global Object Binding: If a function is called outside any object, it is considered a global function. In this case, 'this' is bound to the global object, typically 'window' in a browser environment. However, in strict mode, 'this' becomes undefined instead of defaulting to the global object. 'this' in the below code is bound to the global object which is the space outside every objects and functions.
function foo() { console.log( this.a ); } var a = 2; foo(); // 2
Event Handler Binding: In event handlers, such as those used in HTML elements, 'this' is bound to the element that receives the event. It allows the handler function to operate on the specific element.
Special Cases: Null or Undefined Context and Arrow Functions If you pass null or undefined as the context argument in 'call()', 'apply()', or 'bind()', the context is ignored, and 'this' is automatically set to the global binding. This can be helpful in certain scenarios.
Arrow functions behave differently when it comes to 'this'. They do not have their own 'this' binding. Instead, they inherit the value of 'this' lexically from the enclosing function or the global scope. This lexical scoping behavior makes arrow functions useful in callback functions, ensuring consistent access to the intended 'this' value.
Conclusion: Mastering the 'this' keyword and understanding the intricacies of execution context and binding rules is fundamental to writing robust and effective JavaScript code. By grasping how 'this' is bound in different situations, you can leverage its power to access and manipulate objects seamlessly. Remember to consider the invocation context and apply the appropriate binding rules to ensure proper functioning of your code. With this knowledge, you'll be better equipped to navigate the complexities of JavaScript's 'this' keyword.
Subscribe to my newsletter
Read articles from Ritvik Saran S directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
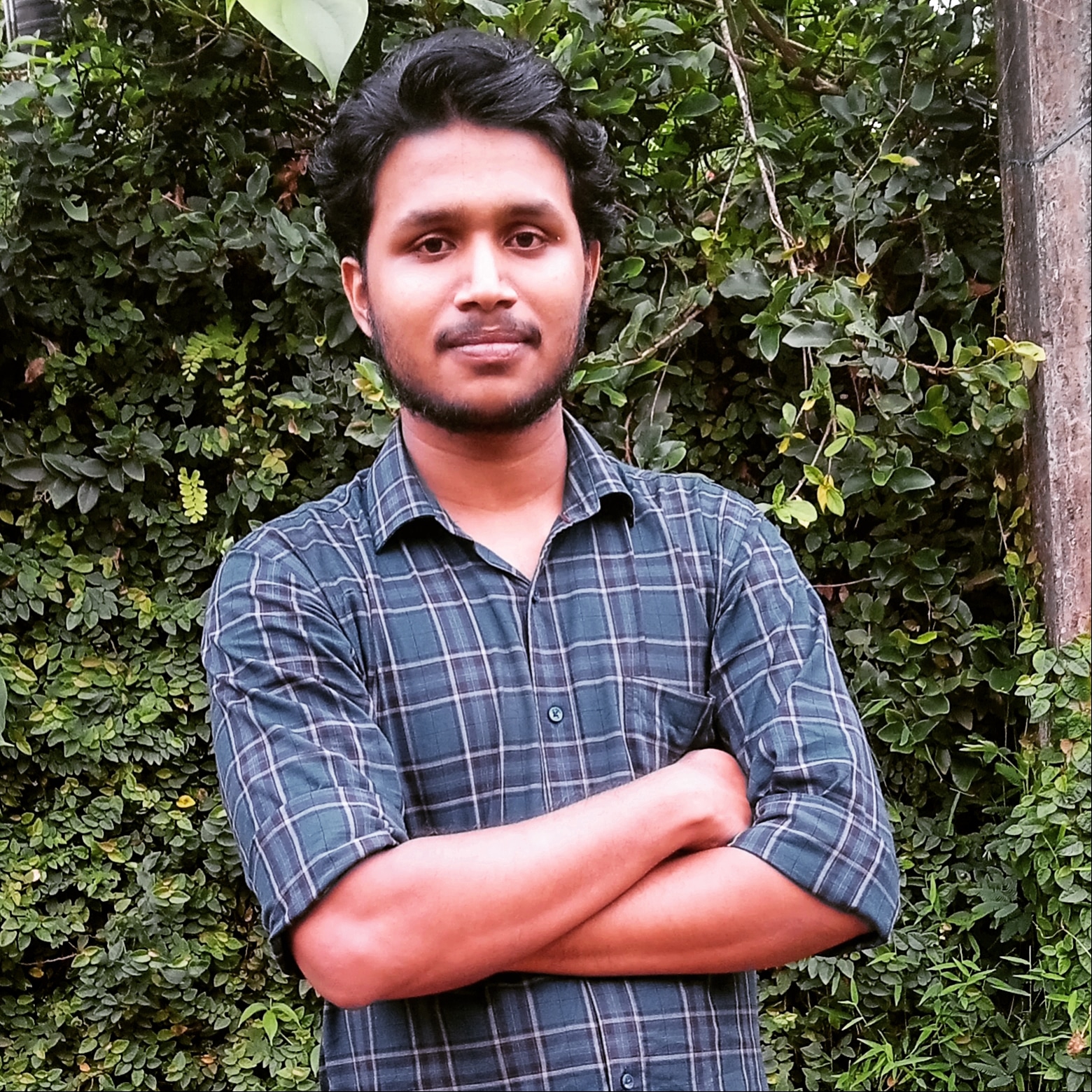
Ritvik Saran S
Ritvik Saran S
Self Taught Software Engineer at TeamLease Services Limited🌟 | Nitian 🎓 | Expanding My Tech Horizon and Sharing the Excitement 🔥