Prototypal Inheritance in JavaScript
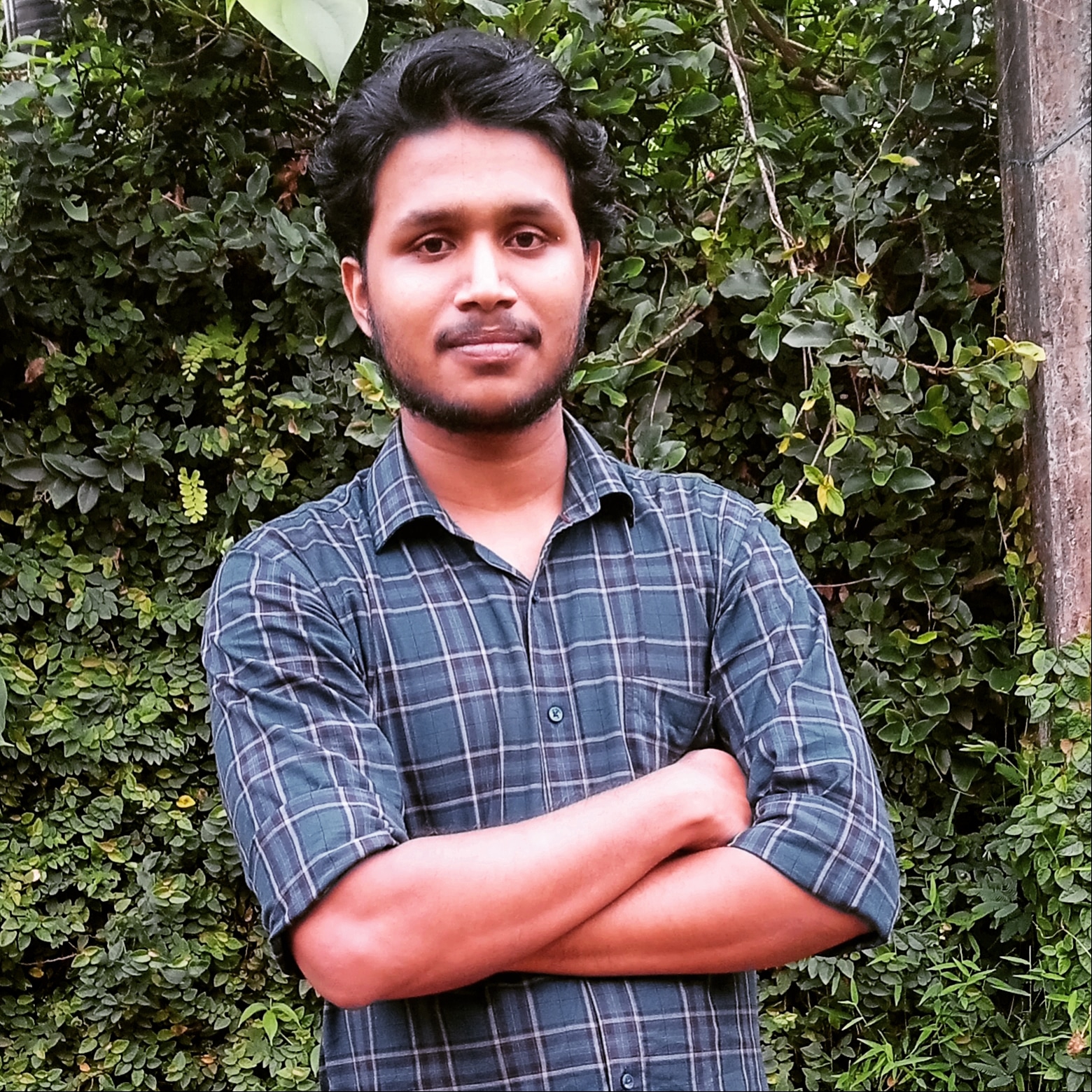
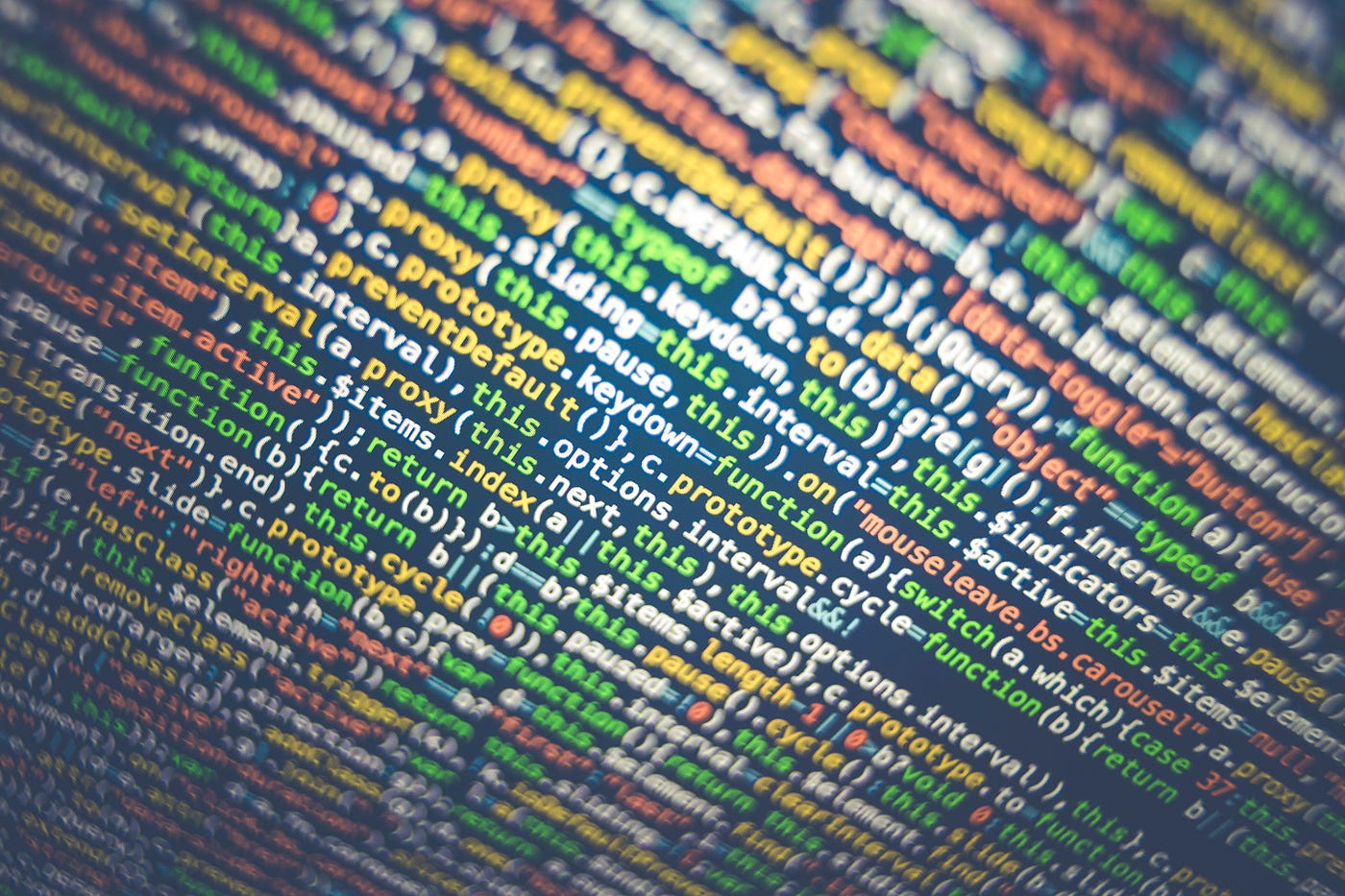
JavaScript prototype objects are a powerful feature that allows you to create reusable code and extend the functionality of existing objects.
What is a prototype object?
A prototype object is an internal property of a function that contains methods and properties that can be inherited by objects created by the function.
When an object is created using the
new
keyword, it will be linked to the prototype by a link called__proto__
. Since this prototype is also an object it will also have another prototype and it forms the prototype chain. When we try to access a property, first it checks in the constructor then it follows the prototype chain to find a match and if not found it returnsundefined
.This chain ends in
Object.prototype
which is the prototype object that serves as the base for all JavaScript objects. It is the ultimate prototype in the prototype chain for all objects created in JavaScript. So as you might have already guessed the__proto__
link ofObject.prototype
points tonull
. So normally an object is created by using another object as its prototype.let b = new Date(); b.__proto__ == Date.prototype b.constructor == Date Date.prototype._proto__ == Object.prototype Date.prototype.constructor == Date Object.prototype.constructor == Object Object.prototype._proto__ == null // Everything returns true
For literal objects, the prototype link is established as follows:
obj.__proto__ = Object.prototype
. For objects created using thenew Function()
syntax, the prototype link is set as:obj.__proto__ = Function.prototype
. There are other ways of creating an object, experiment with those and try to find how this applies there.Okay so if we wanted to add a property to a group of objects created from a prototype object what should we do? Yes, we add it to the prototype object.
function Person() {}
Person.prototype.greet = function() {
console.log('Hello!');}
const p = new Person();
p.greet(); // Hello!
- So we have seen that
Person.prototype
has the properties and methods that are available to all the instances created fromPerson
. What about the members in thePerson
itself and not the prototype? These are static members.
Conclusion
JavaScript prototype objects are a powerful feature that can be used to create reusable code, extend the functionality of existing objects, and implement a variety of different programming patterns. If you are new to JavaScript, I encourage you to learn more about prototype objects. They are valuable tools that can help you write better code.
Subscribe to my newsletter
Read articles from Ritvik Saran S directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
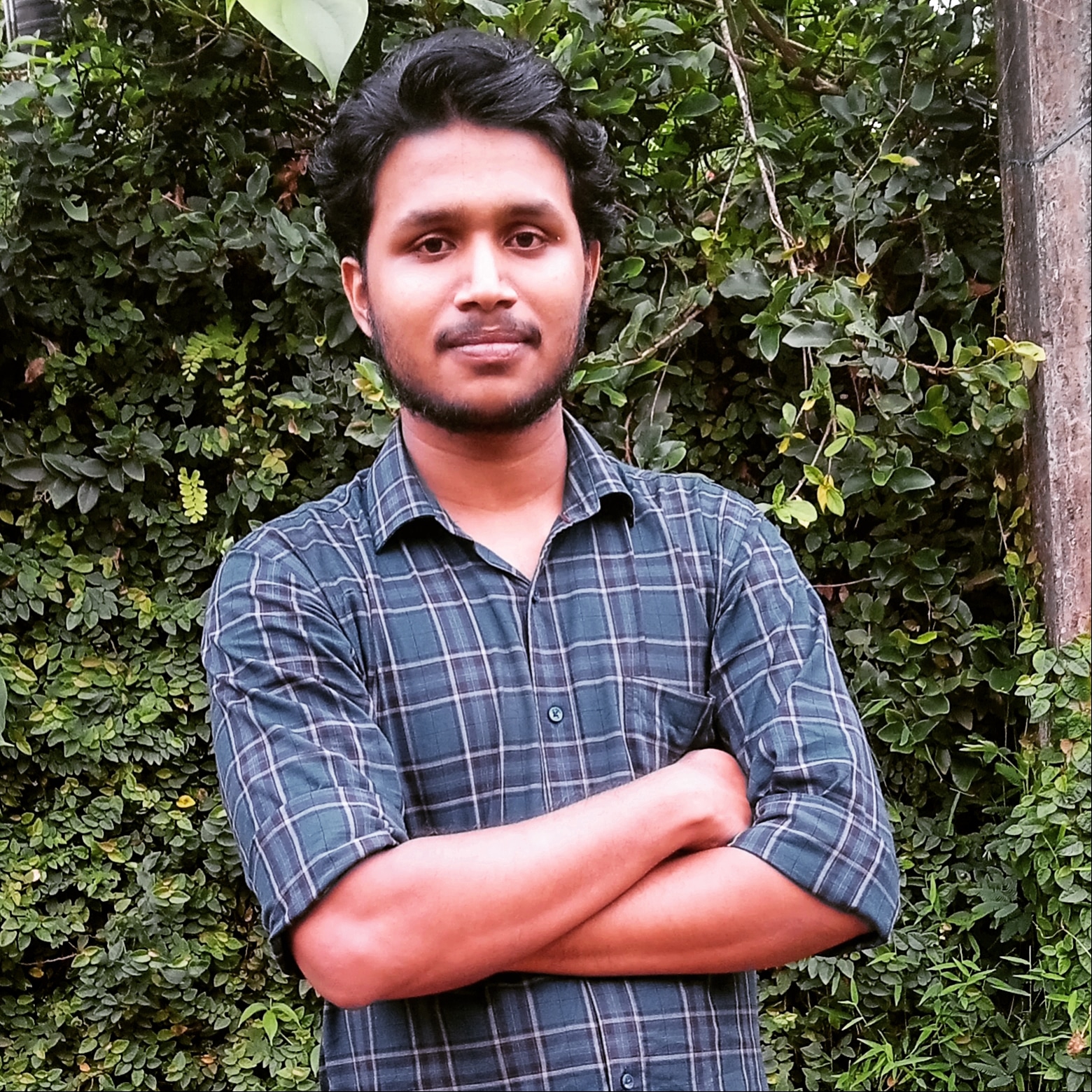
Ritvik Saran S
Ritvik Saran S
Self Taught Software Engineer at TeamLease Services Limited🌟 | Nitian 🎓 | Expanding My Tech Horizon and Sharing the Excitement 🔥