How Hoisting Works in JavaScipt
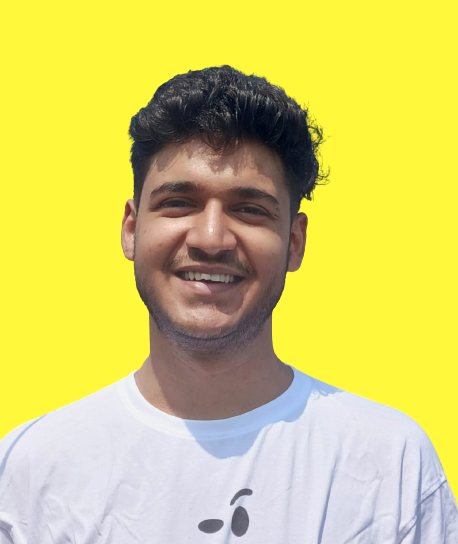
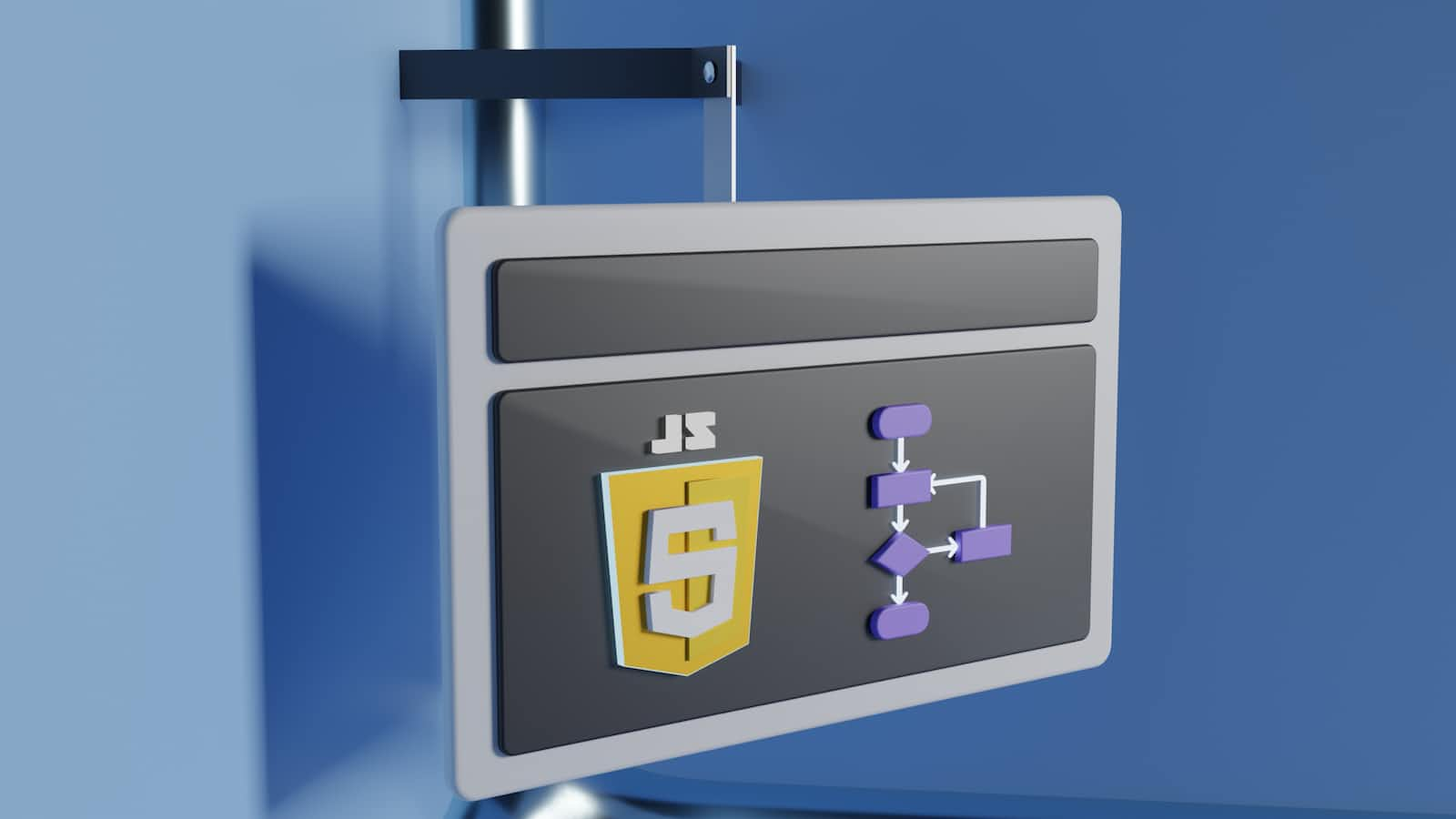
Before the code is executed, during the compilation step, variable and function declarations are moved to the top of their respective scopes. This behavior is referred to in JavaScript as hoisting. As a result, variables and functions can be defined after being utilized and yet be accepted as genuine.
Variable Hoisting
Variables in JavaScript can be declared using the var
, let
, and const
keywords. These variable declarations would be hoisted, but they would behave differently. Let's begin with var.
Hoisting var
variables
var
declared variables are hoisted, which means their declarations are "lifted" to the top of their function or global scope. However, just their declarations are hoisted, not their initializations. When a variable is accessed before it has been declared, the default value of undefined is assigned.
console.log('name is ', name);// name is undefined
var name;
name = 'tom';
console.log('name is ', name);// name is tom
What is Temporal Dead Zone (TDZ)
Before learning about how let
and const
variables are hoisted, we need to understand the concept of the Temporal Dead Zone.
The Temporal Dead Zone (TDZ) is the delay between declaring a variable using the let
or const
keywords and actually initializing it with a value. The variable is said to be in the TDZ during this time period.
The TDZ is useful because it prevents variables from being accessed or assigned values before they have been initialized. This helps to avoid code mistakes that can occur when variables are accessed before they are ready. When you declare a variable with let
or const
, it is automatically placed in the TDZ.
Hoisting let
and const
variables
Hoisting works differently with let
and const
. The variable is in a "temporal dead zone" (TDZ) even if the declarations have been hoisted. The time between hoisting a variable and actually declaring or initializing it is known as the TDZ. Accessing the variable at this time causes a ReferenceError
. It was added to ECMAScript 6 (ES6) along with let and const to make JavaScript more rigid and prevent potential issues brought on by accessing variables before they are specified. TDZ is applicable to block scope.
with let
:
console.log(name)
// ReferenceError: Cannot access 'name' before initialization
let name = "John Cena"
with const
:
console.log(fruit)
// ReferenceError: Cannot access 'name' before initialization
const fruit = "apple";
Function Hoisting
Unlike var declarations, which only hoist the declaration but not its value, function declarations are hoisted entirely — you can safely call the function anywhere in its scope before declaring it.
greet(); // Hi. there.
function greet() {
console.log('Hi, there.');
}
Read the highlighted sentence once again.
greet()// hello
name() // ReferenceError: printDillion is not defined
function greet() {
console.log('Hi, there')
function name() {
console.log("John Cena")
}
}
The greet()
function is defined in this code, and when it is used, it prints the "Hi, there" to the console. However, since name()
is not declared in the global scope, a ReferenceError
is raised when it is called. The reason for this is that greet()
is only accessible within the scope of greet()
because it is defined inside the name()
method. The scope of a function is illustrated here. Therefore, name()
is only accessible withingreet()
and not elsewhere. The hoisting name()
only extends to the top of the scope in which it was declared. In this instance, it is declared inside greet()
under a local scope. It would only be usable in the function as a result.
Only function declarations are hoisted, not function expressions, because they are assigned to variables.
greet(); // TypeError: greet is not a function
let greet = function() {
console.log('Hi, there.');
}
Note:
Though hoisting appears to move the declaration ahead in the programme, what actually happens is that the function and variable declarations are added to memory during the build step.
Subscribe to my newsletter
Read articles from Tushar Joshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
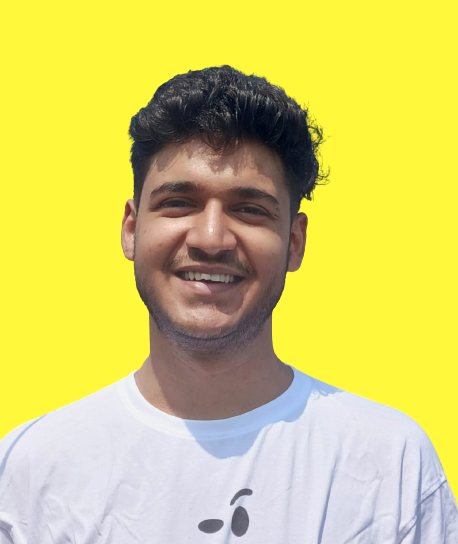