Getting familiar with Closure in Javascript
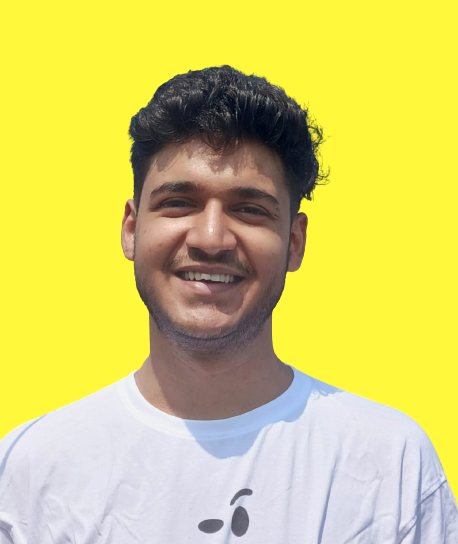
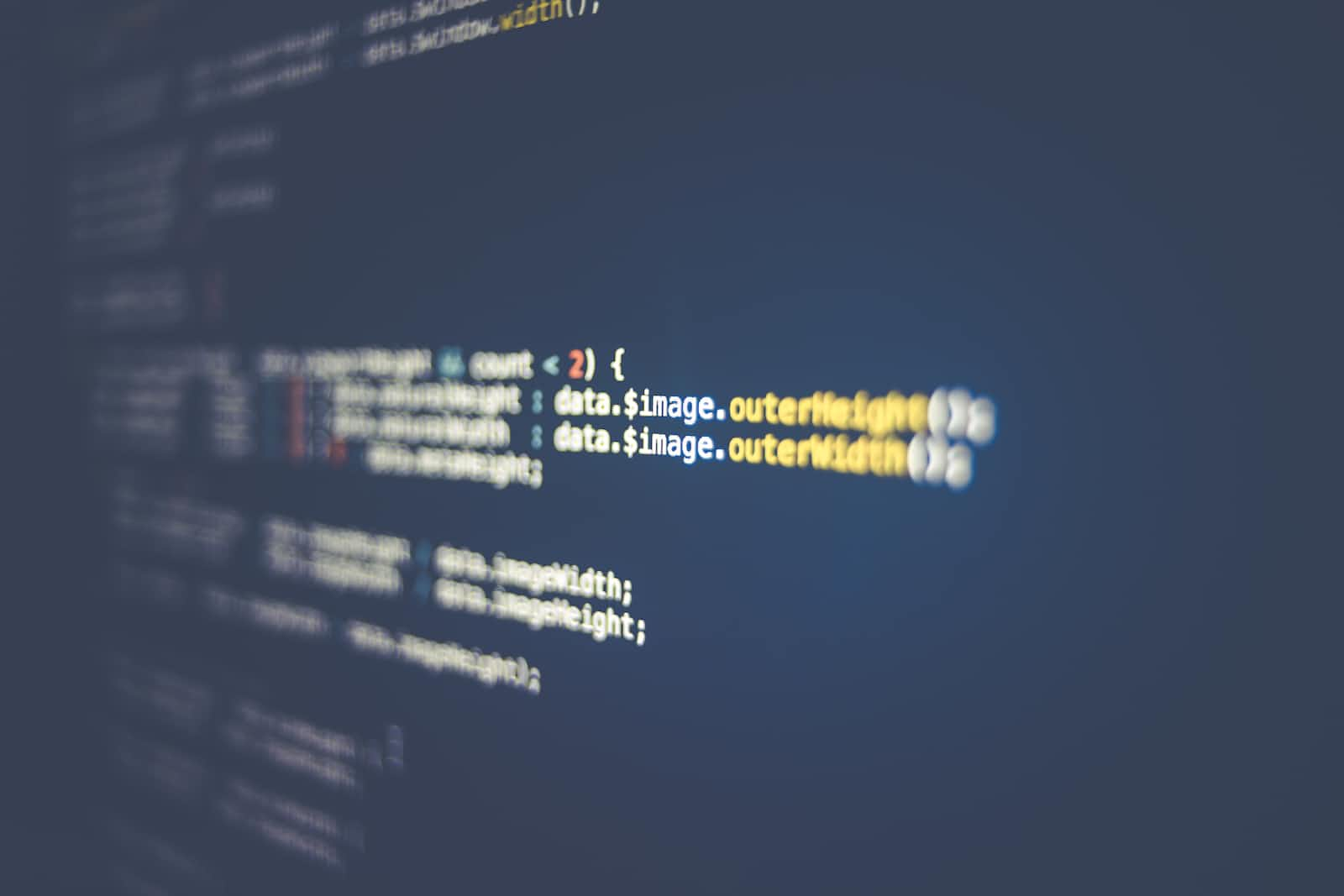
Closures are one of JavaScript's most useful features. This feature enables developers to write self-contained functions that have access to their surrounding context. Closures, when used correctly, can enhance code quality, encapsulate variables, and provide reusable code. You will understand how closures function and their advantages in JS code at the end of this post.
What are Closures?
It is the combination of a function and references to its surrounding state (the lexical environment). It basically allows you to access the scope of an outer function from an inner function. An inner function in a closure has access to the variables of the outer (enclosing) function — a scope chain.
Lexical Scope
It defines the scope of a variable according to the position of that variable declared in the source code.
When a function is defined inside another function, JavaScript employs lexical scoping to resolve variable names. It determines the parent scope of the function by looking at where the function was generated rather than where it was invoked.
How Closure Works?
Closures are functions that provide access to a function's outer scope from its inner function. This capability indicates that a closure can access variables in its surrounding environment, even if those variables are outside the defined limits of a function.
To make a closure, define a function within another function and return it. As a result, even after the external function has been closed, the returning function will be tied to the variables of the outer function. To further comprehend this behaviour, consider the following example:
function makeGreeting(name) {
// name is a variable in the outer scope
return function() {
// this inner function is a closure
console.log("Hello " + name);
};
}
let greetAlice = makeGreeting("Alice");
let greetBob = makeGreeting("Bob");
greetAlice(); // Hello Alice
greetBob(); // Hello Bob
MakeGreeting
is a function that takes a parameter name
and returns another function. The method returned is an inner function that logs "Hello" + name. When you use makeGreeting
with distinct arguments, two new closures are created: greetAlice
and greetBob
. From the outer scope, each closure has access to its own name
variable. Despite the fact that makeGreeting
has returned and its execution context has been removed, the inner functions retain their name
variables. They have attained this capability because they have closed over them.
Benefits of Closures
Closures offer various advantages to JS programming. Encapsulation, for example, allows you to hide implementation details and build self-contained functions. These functions can only expose the functionality that is required. As a result, encapsulation improves the readability, maintainability, and reusability of your code.
Closures can also be used in JavaScript to define private variables. You can establish private variables that cannot be accessed outside of a certain function by creating variables inside a function that returns another function. This procedure can assist you in avoiding unintentional changes to the variable's value and improving the security of your code. This functionality is illustrated by the following:
function counter() {
let count = 0;
function increment() {
count++;
console.log(count);
}
return increment;
}
const incrementCounter = counter();
incrementCounter(); // Output: 1
incrementCounter(); // Output: 2
incrementCounter(); // Output: 3
You define a counter
function with a private count
variable here. The counter
method returns another function called, which increments the count
variable and logs it to the console. You then attach the increment
function to a variable called incrementCounter
. When you call incrementCounter()
many times, it increments the count
variable and logs the new value to the console. The count
variable is private and cannot be accessed outside of the counter
function, which means you cannot unintentionally edit or read its value.
Note:
Closures are a powerful JavaScript feature that allows you to define self-contained functions that have access to their surrounding context. Closures offer a number of advantages, including encapsulation, private variables, and enhanced code quality. Closures allow you to write clearer, more readable, and more manageable code.
Subscribe to my newsletter
Read articles from Tushar Joshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
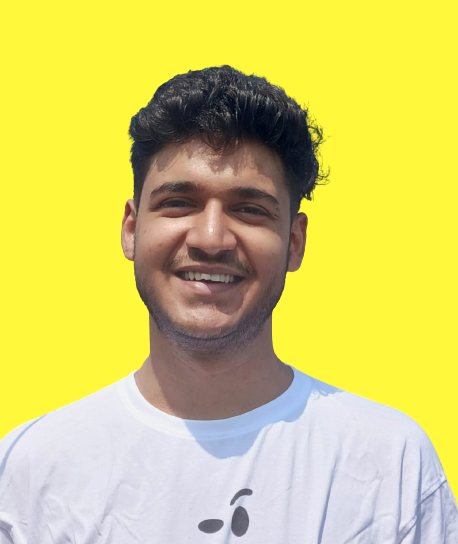