Export Django Model Schemas into Excel
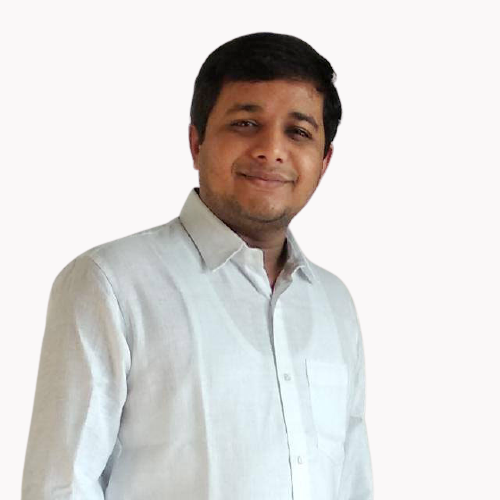

Hello Devs,
I have come across Scanario where I have too many models in my Django project and I have to export model schemas into an Excel file to audit the same.
I have found the solution and I wanted to share it here so it can help you as well.
To export the schemas of all the models in a Django project into an Excel file, you can follow these steps:
To run this solution we can create Django Command which loads all Django settings and models and allow us to get field information.
Retrieve all the models dynamically: You can use the
get_models()
function fromdjango.apps
to get all the models present in your Django project.Create an Excel file: You can use a library like
openpyxl
orxlsxwriter
to create a new Excel file.Iterate over each model: For each model, create a new sheet in the Excel file to represent the model.
Retrieve model fields: Use the
._meta.get_fields()
method to get all the fields of a model. You can filter out any unwanted fields like reverse relationships or internal Django fields.Write field information to the corresponding sheet: Iterate over the fields and write the field name, type, and any other desired information to the corresponding sheet.
Installation
pip install openpyxl
First of all, we have to create Django Custom Command as per below, create folder structure as displayed here
app_name/
management/
__init__.py
commands/
__init__.py
export_schemas.py <-- this is command file
Here is the content for export_schemas.py
import openpyxl
from django.apps import apps
from django.core.management.base import BaseCommand
def export_model_schemas_to_excel():
# Create a new workbook
workbook = openpyxl.Workbook()
# Retrieve all models in the Django project
models = apps.get_models()
# Iterate over each model
for model in models:
# Create a new sheet for the model
sheet = workbook.create_sheet(title=model._meta.model_name)
# Retrieve the fields of the model
fields = model._meta.get_fields()
# Write field information to the sheet
for index, field in enumerate(fields):
sheet.cell(row=index + 1, column=1, value=field.name)
sheet.cell(row=index + 1, column=2, value=str(type(field).__name__))
# Save the workbook as an Excel file
workbook.save('model_schemas.xlsx')
# this is command which we have to import from BaseCommand
class Command(BaseCommand):
help = 'exports all models schema into excel file'
def handle(self, *args, **kwargs):
export_model_schemas_to_excel()
You need to make sure to register your app_name
inside INSTALLED_APPS
in the Django Setting file.
Once the command and setting are finished, we can run the bellow command and it will generate an Excel file in the main Django directory
python3 manage.py export_schemas
After running this command successfully you will get a file generated with name model_schemas.xlsx
Here is the Sample output of exported models
Reference :
Subscribe to my newsletter
Read articles from Akash Senta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
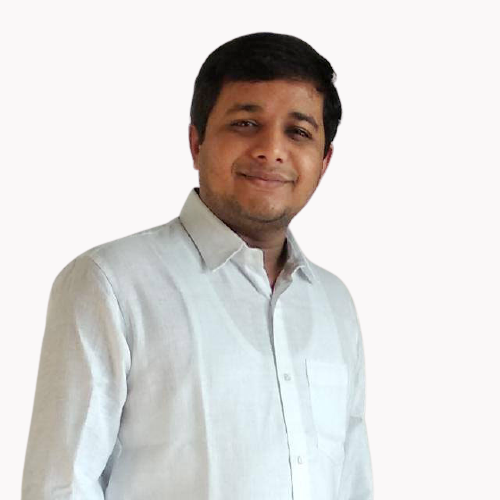
Akash Senta
Akash Senta
Python Developer | Exploring Machine Learning and AI | Former Co-founder at Spring Infosoft. • 5+ Years of experience in developing mobile/web applications using Java / PHP / Python. • Hands-on expertise on Rest API and API generation for Mobile Applications / Backend API Development. • Strong emphasis and in-depth knowledge of Java / Python (Strings, Exception Handling, OOPS concepts) • Worked as Technical Lead gathered requirements from clients, Developed products, Performed client interactions, Resolving issues, and Mentoring juniors. • Apart from Technical skills, my skills include Problem-solving attitude, Ownership, Communication Skills, and Team building skills. • My Skillset includes Python (Django, Django Rest Framework, Flask, REST API, Dialogflow), Java, Android, PHP, MySQL, ElasticSearch, MongoDB, AWS RDS, SQLAlchemy, Amazon EC2 / Other Cloud-based Servers.